When encounter the problem about finding the largest sum of contiguous subarray within an array, the optimized solution for now is Kandane’s algorithm. To understand the Kandane’s algorithm, first we need to learn about dynamic programming.
当遇到在阵列中找到最大连续子阵列之和的问题时,目前的最佳解决方案是Kandane算法 。 要了解坎丹算法,首先我们需要学习动态编程。
Dynamic programming is a problem solving method for solving an optimization problem by breaking it down into simpler sub-problems. Don’t get intimidated by the theory, it is mostly just a matter of taking a recursive algorithm and finding the overlapping sub-problems. One of the simplest examples of dynamic programming is the classic nth Fibonacci number. There are two key attributes that a problem must have in order for dynamic programming to be applicable: optimal substructure and overlapping sub-problems.
动态编程是一种通过将优化问题分解为更简单的子问题来解决问题的方法。 不要被理论所吓倒,这主要只是采用递归算法并找到重叠的子问题。 动态编程最简单的例子之一就是经典的第n个斐波那契数 。 为了使动态编程适用,问题必须具有两个关键属性: 最优子结构和重叠子问题 。
Dynamic programming has two methodologies: top-down & bottom-up. Top-down approach starts to solve the bigger problem by recursively finding the solution to smaller sub-problems. While dealing with the sub-problems, we cache those results for future recursive calls. This way of storing the results of already solved sub-problems is called memoization. Contrary to memoization, bottom-up approach starts to solve the subproblems first, and iteratively generating solutions to bigger and bigger sub-problems by using the solutions to small sub-problems. The way to cumulating answers to the top is called tabulation.
动态编程有两种方法:自顶向下和自底向上 。 自上而下的方法开始通过递归地找到较小的子问题的解决方案来解决更大的问题。 在处理子问题时,我们将这些结果缓存起来,以供将来进行递归调用。 存储已经解决的子问题的结果的这种方式称为备忘录。 与记忆相反, 自下而上的方法首先开始解决子问题,然后通过使用较小的子问题的解决方案来迭代生成越来越大的子问题的解决方案。 将答案累积到顶部的方法称为制表。
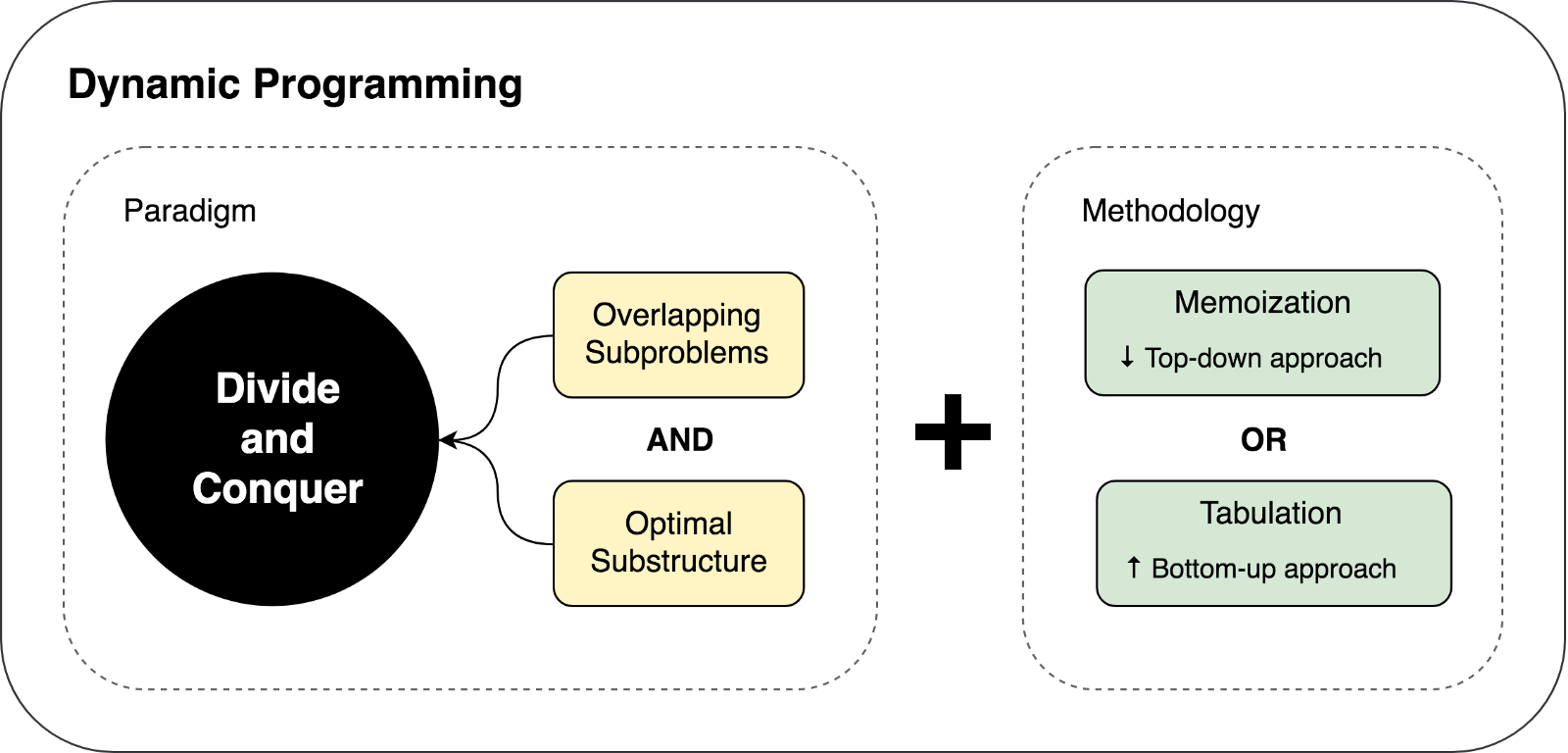
How Kandane’s algorithm falls under dynamic programming paradigm? Let’s see an example(link).
Kandane的算法如何属于动态编程范式? 让我们看一个例子( link )。
Given an integer array Example:
Input: [-2,1,-3,4,-1,2,1,-5,4],Output: 6Explanation: [4,-1,2,1] has the largest sum = 6.
With Kandane’s algorithm we search the given array nums[1…n] from left to right. In the ith step, it calculate the subarray with the largest sum ending at i, this sum is maintained in currentSum. Moreover, it calculates the subarray with the largest sum anywhere in nums[1…n], maintained in maxSum and easily obtained as the maximum of all values of currentSum.
使用Kandane算法,我们搜索给定的数组nums [1…n] 从左到右。 在第i步中,它计算以i结尾的最大和的子数组,该和保持在currentSum中。 而且,它可以计算出子数组,其子数组的总和最大为nums [1…n],并保持在maxSum中,并且很容易获得为currentSum所有值的最大值。
The Kandane’s algorithm uses optimal substructures and overlapping sub-problems with bottom-up approach by tabulation. Because we loop through the array once, the time complexity is O(n).
Kandane的算法使用最佳子结构和重叠的子问题,并通过列表采用自底向上的方法。 因为我们循环遍历数组一次,所以时间复杂度为O(n)。
To understand the solution better, you can test it out with this repl link.
为了更好地理解该解决方案,您可以使用此repl 链接对其进行测试。
Next time, I hope you could use Kandane’s algorithm to solve similar problems. ;)
下次,我希望您可以使用Kandane算法来解决类似的问题。 ;)
翻译自: https://medium.com/swlh/maximum-subarray-sum-in-javascript-eee107d6c3d6