aqi插件
Working in a Global Security Operations Center usually involves monitoring current events and especially abnormal weather. Abnormal weather can cause facility closures, and a good operation will be constantly monitoring any changes to abnormal weather. Currently, we are suffering from poor air quality in the Bay Area due to fires throughout the state of California. As a result, most physical security operations are probably monitoring air quality.
在全球安全运营中心W¯¯平时工作会有涉及监视当前事件,特别是异常天气。 天气异常会导致设施关闭,良好的运营将不断监控异常天气的任何变化。 目前,由于整个加利福尼亚州的大火,我们在湾区空气质量欠佳。 结果,大多数物理安全操作可能正在监视空气质量。
Rather than manually update my team hourly, I used a slack bot that grabs the AQI from the air now API every hour. So I thought I’d share how I did it, and maybe if you use G Suite and Slack in your workplace you could set one up too.
我不是使用每小时200分钟从空中API获取AQI的Slack机器人,而不是每小时手动更新团队。 所以我想我将分享我的工作方式,也许如果您在工作场所使用G Suite和Slack,也可以设置一个。
First, I’d recommend checking out this article on how to set up the slack bot with api.slack.com and Google Apps Script. It’s well written and gets straight to the point.
首先,建议您阅读这篇文章,了解如何使用api.slack.com和Google Apps Script设置Slack机器人。 它写得很好,直截了当。
Once you’re able to send out your first message with the slack bot based on the previous article using the giphy API — check back here to learn how to set up a slack bot that will update the air quality for your team.
一旦您能够使用giphy API根据上一篇文章通过Slack机器人发出第一条消息后,请回到此处以了解如何设置Slack机器人来更新团队的空气质量。
设置立即播送API帐户 (Set Up Air Now API Account)
Go to the following website and set up a free account. When you sign up you’ll be able to retrieve an API key. If you click File > Project properties in your apps script file. You can set the API key to a value in your project properties.
请访问以下网站并设置一个免费帐户。 注册后,您将可以检索API密钥。 如果您在应用程序脚本文件中单击文件>项目属性。 您可以在项目属性中将API密钥设置为一个值。
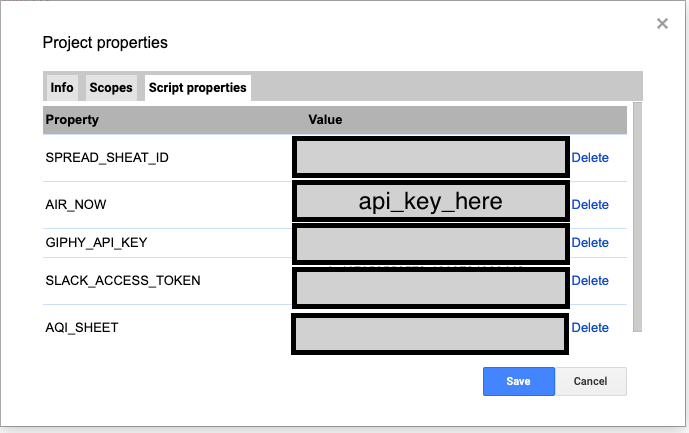
提取AQI (Fetch the AQI)
First, we have to put together a URL to fetch some data. And the airnow API website makes this easy for developers because they have a query tool that will generate the request URL for you, including your API key last.
首先,我们必须将URL组合在一起以获取一些数据。 而且airnow API网站使开发人员很容易做到这一点,因为他们有一个查询工具,它将为您生成请求URL,包括最后一个API密钥。
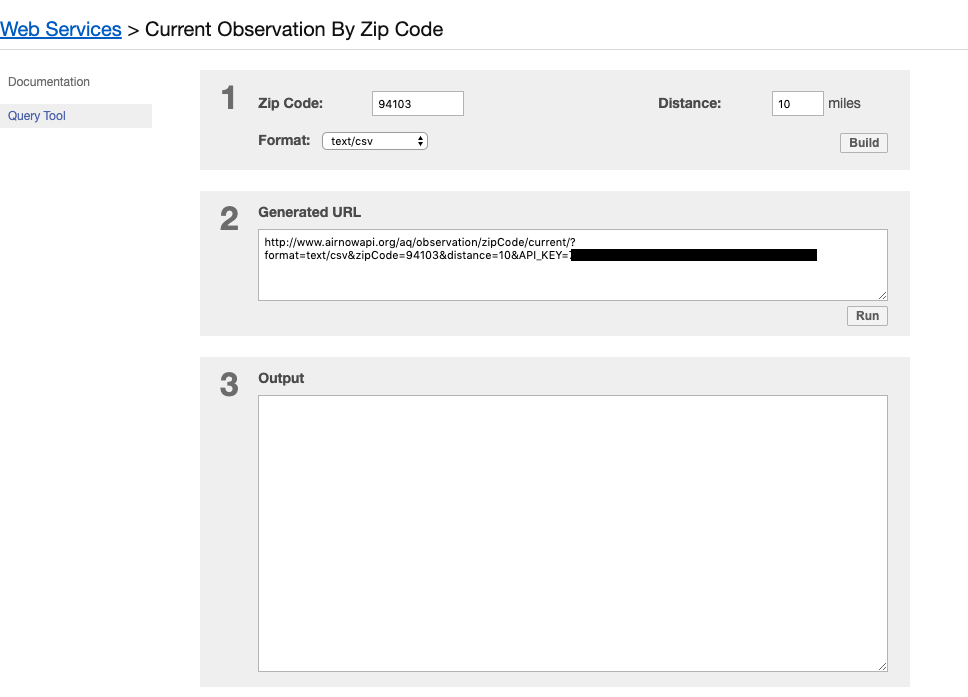
The Query tool makes getting a request URL extremely simple, just type in the zip code and distance you’ll get a request that will provide you some data containing the current air quality. At the top of my apps script file, I placed this URL in a constant called AIR_NOW_BASE and appended the API Key using the project property that I set.
查询工具使获取请求URL非常简单,只需键入邮政编码和距离,您就会获得一个请求,该请求将为您提供一些包含当前空气质量的数据。 在我的应用程序脚本文件的顶部,我将此URL放置在名为AIR_NOW_BASE的常量中,并使用设置的项目属性附加了API密钥。
//retrieve API Key from properties service
var AIR_NOW = PropertiesService
.getScriptProperties()
.getProperty('AIR_NOW');
// generate base url request for current conditions with in
//50 miles of the zip code 94103var AIR_NOW_BASE = "http://www.airnowapi.org/aq/observation/zipCode/current/?format=application" +
"/json&zipCode=94103&distance=50&API_KEY=" + AIR_NOWfunction airQuality()
{
var datatry {var response = UrlFetchApp.fetch(AIR_NOW_BASE);
data = JSON.parse(response)} catch(error) {return "error retrieving AQI"
}return data[1].AQI}
Awesome, now we have a method for retrieving the current AQI. And if it fails we will return an error message.
太好了,现在我们有了一种检索当前AQI的方法。 如果失败,我们将返回错误消息。
将AQI发送到频道 (Send the AQI to the channel)
Slack provides a lot of options for sending messages through a bot. Bots can be made to be quite interactive. But, all we are doing here is sending off a message periodically. No need for too much razzle-dazzle, just some good ol’ straight forward code.
Slack提供了许多通过机器人发送消息的选项。 可以使机器人变得非常互动。 但是,我们在这里所做的只是定期发送消息。 不需要太多的眼花just乱,只需要一些很好的简单代码即可。
I created a method called updateChannelAQI() that puts together a message that has a bit of formatting and returns a custom message depending on where the AQI is.
我创建了一个名为updateChannelAQI()的方法,该方法将一条消息进行了格式化,并根据AQI的位置返回了一条自定义消息。
function updateChannelAQI()
{
var channel = "your_channel"
var aqi = airQuality()
var status = "Good"
// depending on where the AQI - the status will be updatedswitch(true)
{
case aqi < 51: status = "Green"; break;
case aqi < 101: status = "Yellow"; break;
case aqi < 151: status = "Orange"; break;
case aqi < 201: status = "Red"; break;
case aqi < 301: status = "Purple"; break;
case aqi < 500: status = "Maroon"; break;
default: status = "Over 500..."
}
var block = [
{
"type": "header",
"text": {
"type": "plain_text",
"text": "AQI Update :airwatch: ",
"emoji": true
}
},
{
"type": "divider"
},
{
"type": "section",
"text": {
"type": "mrkdwn",
//throw in the aqi and the status in the return text"text": "AQI currently at " + aqi + " (*"+ status +"*)"
}
},
{
"type": "divider"
}
]
var blocks = JSON.stringify(block)
var payload = {token:SLACK_ACCESS_TOKEN, channel: channel, text:"",blocks: blocks};
fetchLogSuccess(payload)
}var POST_MESSAGE_ENDPOINT = 'https://slack.com/api/chat.postMessage';function fetchLogSuccess(payload)
{
try{
var val = UrlFetchApp.fetch(POST_MESSAGE_ENDPOINT, {method: 'post', payload:payload});
var result = JSON.parse(val.getContentText())
if(result.ok)
{
console.log("success")
} else {
console.log(result.error)
}
} catch (e) {
console.log("failed to send message to slack")
console.log(e)
}
}
/**
If you’d like to learn more about using blocks check out the documentation. Otherwise, just replace the block that I’ve set with your custom title or message.
如果您想了解有关使用块的更多信息,请查阅文档。 否则,只需用您的自定义标题或消息替换我设置的块。
The fetchLogSuccess() method posts the message to the Slack bot. I have it separated in its method so that if I choose to give this bot some more features I won’t be repeating code. I can just pass it a payload that gets generated.
fetchLogSuccess()方法将消息发布到Slack机器人。 我将其方法分开,这样,如果我选择为该机器人提供更多功能,就不会重复代码。 我可以将生成的有效负载传递给它。
To test it out you can run the method in the editor and you should see a message like this pop into the channel that you selected.
要对其进行测试,可以在编辑器中运行该方法,并且应该看到一条类似此消息的消息弹出到所选的通道中。
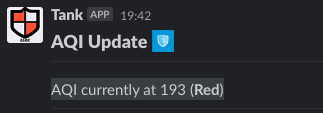
设置触发器 (Set up the Trigger)
Having to manually go back to the Apps Script editor every hour to have the slack bot send a message would defeat the purpose of making the bot work on its own. So to do this we can set up a trigger in a google apps script to run the updateChannelAQI() method hourly.
必须每小时每小时手动返回到Apps Script编辑器,以使松弛的bot发送消息,这将使使bot自行工作的目的无法实现。 因此,为此,我们可以在google apps脚本中设置触发器,以每小时运行一次updateChannelAQI()方法。
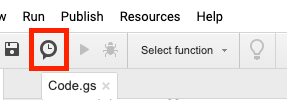
Clicking on the button displayed above will open up a window that will allow you to see the triggers for the project. Select the Add Trigger button on the bottom right-hand side of the page.
单击上面显示的按钮将打开一个窗口,您可以在其中查看项目的触发器。 选择页面右下角的添加触发器按钮。
From there you can select which function to run, and how often to run it. You can even choose how often you should be notified of any failures. Here you’d select the method that you’d like to run and how often you’d like to run it. Here’s what the trigger I set up looks like.
您可以从那里选择要运行的功能以及运行频率。 您甚至可以选择多长时间通知一次失败。 在这里,您可以选择要运行的方法以及运行频率。 这是我设置的触发器的样子。
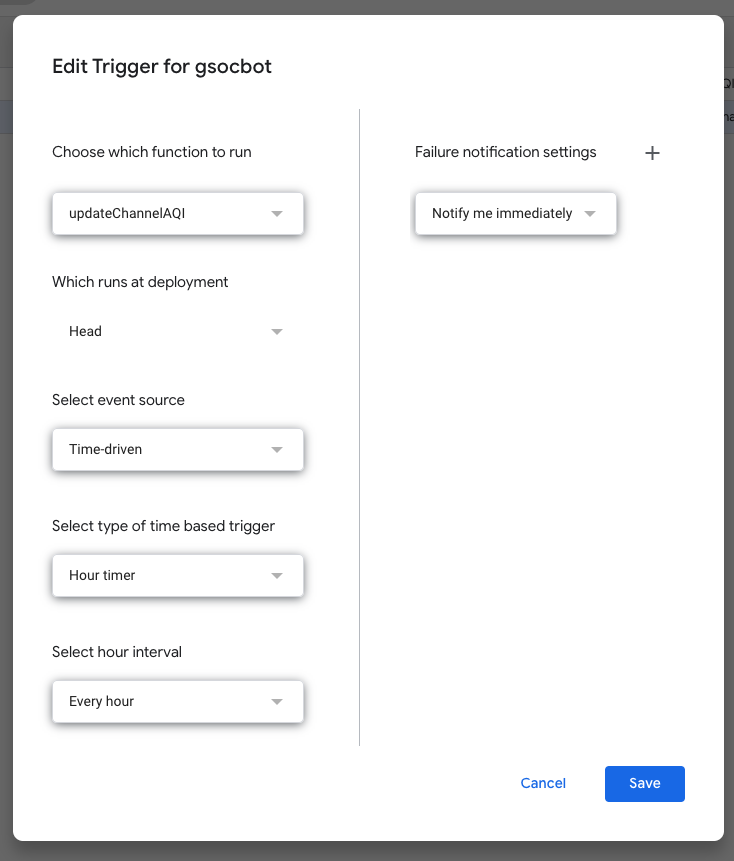
Now if you’ve made it this far and followed along Congratulations! You have successfully set up a Slack bot that will update the AQI into your slack channel every hour. This will save you and your teammates the time from having to collect the AQI hourly. I don’t know about you, but I’m not fond of tedious tasks that we can make scripts do for us.
现在,如果您已经做到了这一点,那么恭喜您! 您已经成功设置了一个Slack机器人,该机器人会每小时将AQI更新到您的Slack频道中。 这样可以节省您和您的队友每小时必须收集AQI的时间。 我不了解您,但是我不喜欢我们可以让脚本为我们做的乏味任务。
And there is so much potential for improvement here. Maybe you only want the message to send when the AQI is above 150? Maybe you want to send a custom message to certain stakeholders when the AQI reaches over 200? Depending on the circumstances you want, you can write some code in Apps Script to get you there.
而且这里还有很大的改进潜力。 也许您只想在AQI高于150时发送消息? 当AQI超过200时,您是否想向某些利益相关者发送自定义消息? 根据您想要的情况,您可以在Apps脚本中编写一些代码以达到目标。
翻译自: https://medium.com/christopher-holmes/google-apps-script-aqi-slack-bot-19f352dcb9f1
aqi插件