内心宁静
Serenity/JS 2.16.0 is out and brings two new exciting features to Questions, one of the five building blocks of the Screenplay Pattern.
Serenity / JS 2.16.0发布了,它为Questions带来了两个令人兴奋的新功能,这是Screenplay Pattern的五个构建块之一。
This latest version of the framework will enable you to:
该框架的最新版本将使您能够:
perform synchronous and asynchronous data transformation operations using Question#map
使用Question#map执行同步和异步数据转换操作
adjust descriptions of complex questions — via Question#describedAs
调整复杂问题的描述-通过Question#describeAs
There’s also a new Questions Design Guide, several implementation examples, and 11 new mapping functions.
还有一个新的《问题设计指南》 ,几个实现示例和11个新的映射功能。
OK, there’s a lot to go through, so let me show you a practical example of how you can use those new features in your tests and why they’re so great.
好的,还有很多事情要做,所以让我向您展示一个实际的示例,说明如何在测试中使用这些新功能以及它们为何如此出色。
Imagine that you needed to write an acceptance test that interacted with a web app that served quiz questions like the one below:
想象一下,您需要编写一个验收测试,该验收测试与提供以下测验问题的Web应用程序交互:
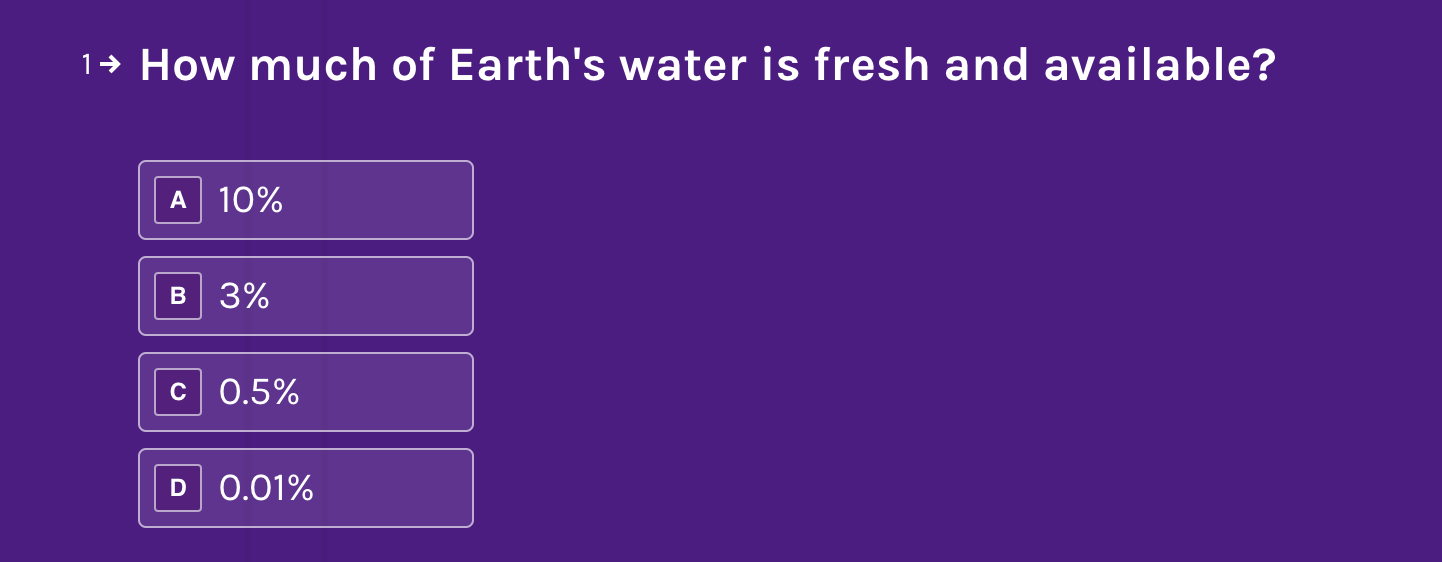
So our test scenario should:
因此,我们的测试方案应为:
verify that the available answers are 10%, 3%, 0.5% and 0.01%
验证可用答案是10%,3%,0.5%和0.01%
How would we do it?
我们将如何做?
OK, so to keep things simple, let’s say that the HTML behind our web form looks like this:
好的,为简单起见,我们假设Web表单后面HTML如下所示:
<p>How much of Earth's water is fresh and available?</p>
<ul>
<li data-test="answer">
<label for="a"><input type="radio" name="answer" id="a" />
10%
</label>
</li>
<li data-test="answer">
<label for="b"><input type="radio" name="answer" id="b" />
3%
</label>
</li>
<li data-test="answer">
<label for="c"><input type="radio" name="answer" id="c" />
0.5%
</label>
</li>
<li data-test="answer">
<label for="d"><input type="radio" name="answer" id="d" />
0.01%
</label>
</li>
</ul>
We start with defining a Lean Page Object describing the structure of our component:
import { Target } from '@serenity-js/protractor';
import { by } from 'protractor';class Quiz {
static Answers =
Target.all('quiz answers')
.located(by.css('[data-test="answer"]'));}
… and define a question that will allow our actors to retrieve the text of all the answers available in the quiz:
…并定义一个问题,该问题将使我们的演员可以检索测验中所有可用答案的文本:
import { Text } from '@serenity-js/protractor';const Answers =
Text.ofAll(Quiz.Answers);
With the above in place, we can write an assertion (which we’d invoke in a Cucumber step definition or a Jasmine or Mocha test):
完成上述操作后,我们可以编写一个断言(在Cucumber步骤定义或Jasmine或Mocha测试中调用该断言):
import { actorInTheSpotlight } from '@serenity-js/core';
import { Ensure, equals } from '@serenity-js/assertions';
actorInTheSpotlight().attemptsTo(
// ... other interactions Ensure.that(Answers, equals([
'10%',
'3%',
'0.5%',
'0.01%',
])),
));
Nice and easy. So let’s increase the level of difficulty a bit, shall we?
好,易于。 因此,让我们稍微增加一点难度吧?
Now imagine that there’s a new business rule that our test scenario should accommodate.
现在想象一下,我们的测试场景应该适应一个新的业务规则。
The new rule states that instead of providing canned answers to our quiz question, the app now needs to generate the values of all the incorrect answers at random.
新规则规定,该应用程序现在需要随机生成所有错误答案的值,而不是为我们的测验问题提供固定答案。
Let’s also throw in another requirement that neither one of those randomly generated answers should ever exceed the value of 15%.
我们还提出另一个要求,即那些随机生成的答案中的任何一个都不应该超过15%的值。
All this means is that our test should:
所有这些意味着我们的测试应该:
verify that there’s a correct answer available in the list,
验证列表中是否有正确答案,
verify that none of the answers in the list exceeds the value of 15%.
验证列表中的答案均未超过15%的值。
Needless to say, an explicit equals check will no longer cut it since we can’t predict what values the answers are going to have.
不用说,显式的等式检查将不再有效,因为我们无法预测答案将具有什么值。
What we can do instead, though, is to improve our implementation to take advantage of the new Serenity/JS 2.16.0 mapping functions and turn the text-based data into numbers:
但是,我们可以做的是改进我们的实现,以利用新的Serenity / JS 2.16.0映射功能,并将基于文本的数据转换为数字:
import { trim, replace, toNumber } from '@serenity-js/core';
import { Text } from '@serenity-js/protractor';const Answers =
Text.ofAll(Quiz.Answers)
.map(trim()) // remove whitespace
.map(replace('%', '')) // remove the '%' character
.map(toNumber()); // convert to numbers// resolves to [ 10, 3, 0.5, 0.01 ]
Now that our question resolves to a list of numbers, we can improve the assertion:
现在我们的问题解决了一个数字列表,我们可以改善断言:
import { actorInTheSpotlight } from '@serenity-js/core';import {
Ensure, equals,
containAtLeastOneItemThat, containItemsWhereEachItem,
isLessThan
} from '@serenity-js/assertions';actorInTheSpotlight().attemptsTo(
// ... other interactionsEnsure.that(Answers, and(
containAtLeastOneItemThat(equals(0.5)),
containItemsWhereEachItem(isLessThan(15)),
)),
));
The great thing about the Question#map and all the mapping functions is that they work with any Question, not just the Web-specific ones. You could, for example, use this technique to extract interesting bits from an API response.
关于Question#map和所有映射功能的妙处在于,它们可以与任何Question一起使用,而不仅限于特定于Web的问题。 例如,您可以使用此技术从API响应中提取有趣的位。
Another great thing is that you can use the same syntax with questions returning a single value or a list of values.
另一个很棒的事情是,您可以对返回单个值或值列表的问题使用相同的语法。
There’s one more thing left to do in our little tutorial, though.
不过,在我们的小教程中还有另外一件事要做。
To avoid the below question getting reported as "text of all quiz answers"
:
为了避免以下问题被报告为"text of all quiz answers"
:
const Answers =
Text.ofAll(Quiz.Answers)
.map(trim())
.map(replace('%', ''))
.map(toNumber())
we can take advantage of the second new feature I mentioned in the beginning — Question#describedAs, which will help us customise the description:
我们可以利用我一开始提到的第二个新功能-Question#describeAs ,它将帮助我们自定义描述:
import { trim, replace, toNumber } from '@serenity-js/core';
import { Text } from '@serenity-js/protractor';const Answers =
Text.ofAll(Quiz.Answers)
.map(trim())
.map(replace('%', ''))
.map(toNumber())
.describedAs('available answers');
And voila! Now you know how to perform data transformations on your Serenity/JS questions!
瞧! 现在,您知道如何对Serenity / JS问题执行数据转换!
To learn more check out the new Questions Design Guide and remember to let me know what you think in the comments!
要了解更多信息,请查看新的“问题设计指南”,并记住让我知道您在评论中的想法!
Enjoy Serenity!
享受宁静!
Jan
一月
翻译自: https://janmolak.com/new-features-in-serenity-js-2-16-0-2097b09e4302
内心宁静