vue ui库开发
Heads up: this article assumes at least a basic understanding of HTML, CSS, and JavaScript. If you don’t have that, no worries, you can find a really great crash course on these technologies here. You can also find more information about Vue here.
注意:本文假设至少对HTML,CSS和JavaScript有基本的了解。 如果没有这些,不用担心,您可以在这里找到有关这些技术的非常不错的速成课程。 您还可以在此处找到有关Vue的更多信息。
什么是Vue实例? (What is the Vue instance?)
The Vue instance is simply the way in which the Vue object is instantiated on your page by calling ‘new Vue({…}).’
Vue实例只是通过调用“ new Vue({…})”在页面上实例化Vue对象的方式。
Within the object, you have all of your associated data, methods, etc. that can be used to store your data and manipulate your DOM.
在对象内,您具有所有关联的数据,方法等,可用于存储数据和操作DOM。
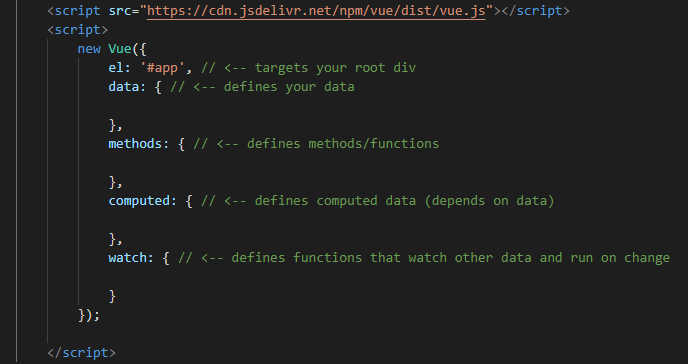
Vue实例如何创建? (How is the Vue instance created?)
When you call the Vue constructor function from the Vue library, and instantiate it into a new object on your page, Vue looks for properties within that object and uses that information to paint/modify the DOM, or to perform specified calculations in the background.
当您从Vue库调用Vue构造函数并将其实例化到页面上的新对象时,Vue会在该对象中查找属性,并使用该信息来绘制/修改DOM或在后台执行指定的计算。
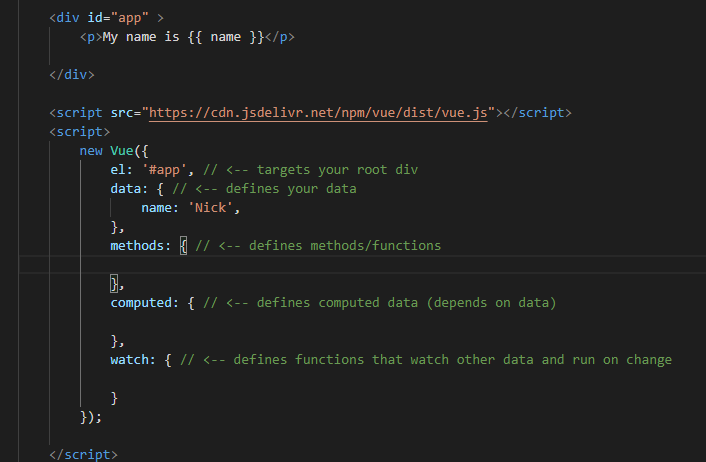
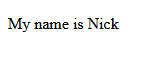
Vue实例的常见片段 (Common pieces of the Vue instance)
The common pieces that you are going to run into are as follows:
您将遇到的常见部分如下:
艾尔 (El)
The ‘el,’ is short for ‘element,’ and it is used to specify which element you are targeting on your page as the root of your Vue instance for DOM manipulation and data interpolation.
“ el”是“ element”的缩写,用于指定要在页面上定位的元素作为DOM操作和数据插值的Vue实例的根。
数据 (Data)
The data property is where you can store all variables that you want the Vue instance to be able to access on the fly, and will stay in scope with the ‘this’ keyword, in relation to the Vue instance itself.
在data属性中,您可以存储希望Vue实例能够即时访问的所有变量,并且相对于Vue实例本身,将使用'this'关键字保留在范围内。
方法 (Methods)
The methods property is where you can store any functions that you want to be able to call on your data to manipulate it, handle interactions, or otherwise encapsulate functionality.
在methods属性中,您可以存储要调用的任何函数,以对数据进行操作,处理交互或封装功能。
已计算 (Computed)
The computed property is where you can store any data that is dependent on other data, or that needs to be computed with a more complex function before it can be displayed.
您可以在计算属性中存储依赖于其他数据的任何数据,或者需要使用更复杂的函数进行计算才能显示这些数据。
Note that computed properties can be interpolated just like values from the data property, but they are cached so they may not re-run every time different parts of the page are updated.
请注意,可以像计算数据属性中的值一样对计算的属性进行插值,但是它们会被缓存,因此它们可能不会在每次更新页面的不同部分时重新运行。
看 (Watch)
The watch property specifies functions that run when a particular data value is changed. These functions are not cached and will run every single time there is a change regardless (though I believe you can stop them manually with a stopPropagation command, or by using the v-once directive).
watch属性指定在更改特定数据值时运行的函数。 这些函数不会被缓存,并且无论是否发生更改都将每次运行(尽管我相信您可以使用stopPropagation命令或使用v-once指令手动停止它们)。
使用多个Vue实例 (Working with multiple Vue instances)
It is totally legal and fine to have multiple Vue instances on your page controlling different parts and pieces of it. In fact, you can even have your instances communicate with each other by storing them in variables within the global namespace.
在页面上具有多个Vue实例来控制它的不同部分是完全合法的。 实际上,您甚至可以通过将实例存储在全局名称空间内的变量中来使它们彼此通信。
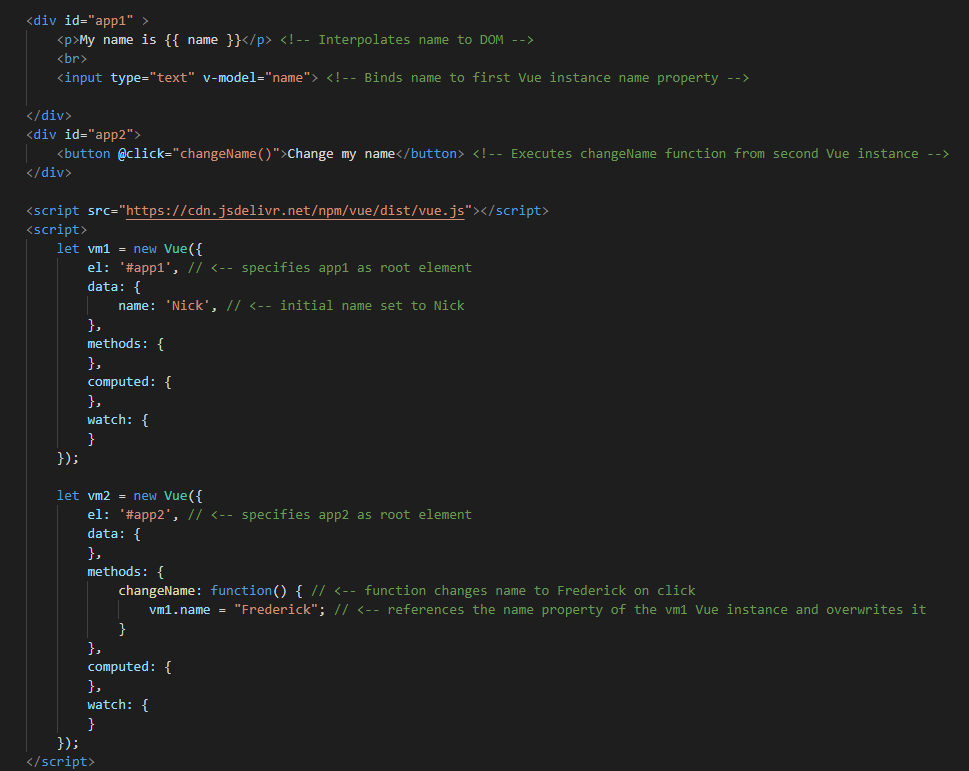
When the button is clicked, the name is changed to ‘Frederick’ from outside of the first Vue instance where the property is held.
单击该按钮后,名称将从保存该属性的第一个Vue实例外部更改为“ Frederick”。
This can have massive implications if you have multiple widgets handled by Vue on your page, that need to talk with each other, share, and manipulate data based on user interaction.
如果您的页面上有多个由Vue处理的小部件,这些小部件需要彼此交谈,共享和基于用户交互操作数据,那么这可能会产生巨大的影响。
我还能做其他事情吗? (Is there other stuff I can do?)
Absolutely, there’s a lot more you can do with Vue, including mounting templates, creating components, and working with lifecycle hooks.
绝对,您可以使用Vue做更多的事情,包括安装模板,创建组件以及使用生命周期挂钩。
I would go into all of that here, but its probably overkill for what you need, and requires much more explanation. If you find you do need this functionality, you can find an excellent free tutorial for Vue here.
我将在这里介绍所有这些内容,但是它可能对您所需的内容过于夸张,并且需要更多说明。 如果您确实需要此功能,可以在此处找到有关Vue的出色免费教程。
Additionally, while you can use Vue off a CDN for simple DOM control, if you need advanced functionality, you will wan to use the Vue CLI, which the above tutorial goes over as well.
另外,虽然您可以将CD之外的Vue用于简单的DOM控制,但如果需要高级功能,则可以使用Vue CLI,上面的教程也对此进行了介绍。
底线 (The bottom line)
Vue can do a lot for you when it comes to managing your data and manipulating your DOM quickly and easily.
在管理数据和快速轻松地操作DOM方面,Vue可以为您做很多事情。
By learning more about the Vue instance, you have taken the first steps to empowering yourself when developing your UI with Vue.
通过了解有关Vue实例的更多信息,您已迈出了使用Vue开发UI时增强自身能力的第一步。
翻译自: https://medium.com/design-bootcamp/ui-ux-development-master-the-vue-instance-18aa5e708abd
vue ui库开发