ios 后台任务
React Native background tasks can be useful in a number of ways, from aiding in the user experience to managing authentication mechanisms and analytics data. Background tasks are triggered behind the scenes even if the app is not running. What is impressive, and extremely useful, is the ability to tie these tasks into React Native code, giving the developer the ability to do things like contact remote servers through fetch
requests, and update AsyncStorage
values (or other database solutions you may have such as SQLLite or Realm).
从帮助用户体验到管理身份验证机制和分析数据,React Native后台任务在许多方面都非常有用。 即使应用未运行,后台任务也会在后台触发。 令人印象深刻且极其有用的功能是将这些任务绑定到React Native代码中的能力,使开发人员能够执行诸如通过fetch
请求联系远程服务器,更新AsyncStorage
值(或您可能拥有的其他数据库解决方案,例如SQLLite或Realm )。
Background tasks are also a useful way to tackle syncing issues, by allowing an app to do housekeeping while the app is not running in the foreground. In an ideal world, an app should display the most up to date app state from the moment it is opened. This problem is magnified when apps go multi-platform, whereby users may be using a web or desktop version of a service before jumping back into the native app — expecting those updates to have taken effect.
后台任务也是解决同步问题的一种有用方法,它允许应用程序在前台不运行时进行内务处理。 在理想的世界中,应用程序应从打开之日起显示最新的应用程序状态。 当应用程序进入多平台时,此问题会放大,从而使用户在跳回本机应用程序之前可能正在使用服务的Web或桌面版本-希望这些更新已生效。
There are a few packages available for background task management in React Native, and this article will showcase the most reliable of them. These packages support both Android and iOS, but will be demoed around iOS throughout this piece.
React Native中有一些可用于后台任务管理的软件包,本文将展示其中最可靠的软件包。 这些软件包同时支持Android和iOS,但在整篇文章中将围绕iOS进行演示。
后台任务的局限性 (Limitations of background tasks)
It is important to note that the implementation of background processes differ between iOS and Android, and iOS in-particular comes with strict limitations in terms of background task usage.
重要的是要注意,iOS和Android之间后台进程的实现有所不同,特别是iOS在后台任务使用方面受到严格限制。
For example, iOS invokes a minimum waiting period of 15 minutes before background tasks are triggered, the timing of which is also dependent on battery drain and frequency of app usage. Because of this, it could be hours before the next background task is fired. Because of this inconsistency and unpredictability, background tasks should not be mission critical, and instead should be used to supplement foreground processes. Apple discuss in more detail about how background tasks are executed here.
例如,iOS会在触发后台任务之前调用最少15分钟的等待时间,该等待时间还取决于电池消耗和 应用使用频率。 因此,下一个后台任务可能要花几个小时才能启动。 由于这种不一致和不可预测性,后台任务不应成为关键任务,而应被用来补充前台流程。 苹果公司在这里详细讨论如何执行后台任务。
整合后台任务 (Integrating Background Tasks)
This section documents some of the background task managers that have been implemented for React Native, and dives deeper with background task capabilities and behaviour.
本节介绍了已为React Native实施的一些后台任务管理器,并深入介绍了后台任务功能和行为。
There are a few background task packages one could adopt to start taking advantage of background functionality within React Native. The one this piece explores is react-native-background-fetch
due to its comprehensive configurable options, ease of use and the fact it is actively maintained.
可以采用一些后台任务包来开始利用React Native中的后台功能。 由于其全面的可配置选项,易用性以及积极维护的事实,本文探讨的是“ react-native-background-fetch
。
Other packages have sprung up in the past. react-native-background-task
provides a slightly simpler API, but has not been updated in 3 years at the time of writing. It actually relies on react-native-background-fetch
as a dependency and builds its functionality around it. Because of the lack of maintenance, this package cannot be recommended.
过去出现了其他软件包。 react-native-background-task
提供了稍微简单的API,但在撰写本文时已3年未更新。 它实际上依赖于react-native-background-fetch
作为依赖关系,并围绕它构建功能。 由于缺乏维护,因此不建议使用此软件包。
React本机后台计时器 (React Native Background Timer)
Another package that has gained strong adoption and is regularly maintained is react-native-background-timer
. This package has opted for a start-stop approach for triggering background tasks, where the code logic is wrapped between these two function calls. Unsurprisingly, the package inherits the same limitations as react-native-background-fetch
, as the same underlying Objective-C APIs are used behind the JavaScript wrappers. This means that implementing setInterval
on iOS will not work after a period of time — less than a minute. setTimout
also operates under the same duration constraints.
另一个获得广泛采用并定期维护的软件包是react-native-background-timer
。 该软件包选择了一种用于触发后台任务的起止方法,其中代码逻辑包含在这两个函数调用之间。 毫不奇怪,该软件包继承了与react-native-background-fetch
相同的限制,因为在JavaScript包装器后面使用了相同的基础Objective-C API。 这意味着在一段时间(不到一分钟)后,无法在iOS上实现setInterval
。 setTimout
也可以在相同的持续时间约束下运行。
This behaviour is universal to all background task managers. To test this yourself with react-native-background-timer
, go ahead and install the package, remembering to link the package to CocoaPods if your version of React Native does not support auto-linking:
此行为对于所有后台任务管理器都是通用的。 要使用react-native-background-timer
自己进行测试,请继续安装该软件包,如果您的React Native版本不支持自动链接,请记住将该软件包链接到CocoaPods:
// install and link react-native-background-timeryarn add react-native-background-timer
react-native link react-native-background-timer
cd ios && pod install
Within your React Native application, attempt to code a setInterval
function within a timer:
在您的React Native应用程序中,尝试在计时器中编写setInterval
函数的代码:
// testing how long a setInterval background task runsexport const App = (props) => { // start the timerconsole.log('starting background timer');
BackgroundTimer.start(); // start interval loop
let i = 0;
setInterval(function () {
i++;
console.log('timer is now at increment ' + i); // manually stop the process after one minute
if (i === 20) {
BackgroundTimer.stop();
}
}, 3000); return(<Text>Testing Background Timer</Text>);
}
Now if you run this test on your device, and close the app immediately, you will notice the code continues to execute — that is, until it doesn’t. The loop typically lasts around 30 seconds, and never surpasses 60 seconds from my tests; the BackgroundTimer.stop()
line from above will never be executed.
现在,如果您在设备上运行该测试,然后立即关闭该应用程序,您将注意到代码继续执行-直到没有执行。 循环通常持续约30秒,从测试开始不会超过60秒; 上面的BackgroundTimer.stop()
行将永远不会执行。
Instead, what you will witness is iOS’s expiry handler at work, ending prolonged background tasks. Instead of relying on this mechanism — to continuously run an open task until the expiry handler closes it — and putting future background task time into jeopardy in the process, always end your background tasks in good time.
相反,您将看到的是iOS的到期处理程序正在工作,从而结束了长时间的后台任务。 不要依赖这种机制(要连续运行一个打开的任务,直到到期处理程序将其关闭),而要使将来的后台任务时间陷入危险之中,请始终按时结束您的后台任务。
The amount of background processing time your tasks get is exclusive to your app, not each background task. Therefore, it is important that all your background tasks are ended in a satisfactory time.
您的任务获得的后台处理时间是应用程序专有的,而不是每个后台任务专有的。 因此,重要的是 您的 所有 后台任务必须在令人满意的时间内结束。
总是结束后台任务 (Always end background tasks)
Concretely, developers are required to end a background task, otherwise iOS’s expiry handler will eventually end the task itself that will consequently limit future background tasks, either by shortening their execution time or suspending them completely. react-native-background-timer
does this via its BackgroundTimer.stop()
API. react-native-background-fetch
does so in a slightly different way, that we will explore further down.
具体来说,要求开发人员结束后台任务,否则,iOS的到期处理程序将最终结束任务本身,从而通过缩短执行时间或完全暂停它们来限制将来的后台任务。 react-native-background-timer
通过其BackgroundTimer.stop()
API进行此操作。 react-native-background-fetch
执行方式略有不同,我们将进一步探讨。
Now, if we open the above app again into the foreground, the background task continues. If we then return to the device home screen, the background task stops immediately. This pattern also happens with setTimeout
, where the timeout duration is more than the allotted background task time.
现在,如果我们再次将上述应用程序打开到前台,则后台任务将继续。 如果然后返回设备主屏幕,则后台任务将立即停止。 setTimeout
也会发生这种模式,其中超时时间长于分配的后台任务时间。
Background tasks are not executed on the iOS Simulator, and can only be tested on physical devices.
后台任务不会在iOS模拟器上执行,只能在物理设备上进行测试。
iOS will wait a period of time before this task will run once again in the background, that could range from 15 minutes to a number of hours depending on the factors mentioned earlier. If the app is open and living in memory, there will be a higher likelihood background tasks will fire more frequently. In any case, both setInterval
and setTimeout
are not ideal for running background tasks, and will not prolong the task on iOS.
iOS将等待一段时间,然后此任务才能在后台再次运行,具体时间可能从15分钟到几个小时不等,具体取决于前面提到的因素。 如果该应用程序已打开并存在于内存中,则后台任务将更频繁地触发。 无论如何, setInterval
和setTimeout
都不适合运行后台任务,并且不会延长iOS上的任务。
在XCode中启用后台模式 (Enabling background modes in XCode)
Your app’s background capabilities are dependant on which features you enable within Xcode. There are a few background modes that can be toggled, ranging from networking (fetch) capabilities, to using GPS, external accessory communication, as well as accessing audio, AirPlay and Picture in Picture:
应用程序的后台功能取决于您在Xcode中启用的功能。 可以切换几种背景模式,从联网(获取)功能到使用GPS,外部附件通信以及访问音频,AirPlay和画中画:

Apple have documented all background modes inside the documentation archive here, minus the Background processing option that has since been picked up on Stack Overflow. Background fetch will be the most commonly used option, as networking is required to communicate with external servers to do things like authentication checks, app state updates, etc.
Apple已在此处的文档档案中记录了所有后台模式,减去了从Stack Overflow上获取的“ 后台处理”选项。 后台获取将是最常用的选项,因为需要与网络进行通信以与外部服务器进行通信以执行身份验证检查,应用程序状态更新等操作。
Let’s next demonstrate react-native-background-fetch
with some real-world use cases.
接下来让我们用一些实际的用例来演示react-native-background-fetch
。
React Native后台获取的后台任务 (Background Tasks with React Native Background Fetch)
react-native-background-fetch
handles periodic background tasks under the same constraints react-native-background-timer
does, but also allows you to schedule one-off tasks that can be invoked in the foreground of the app.
react-native-background-fetch
在相同的约束下处理周期性的后台任务, react-native-background-timer
这样做,但是还允许您安排可以在应用程序前台调用的一次性任务。
It is important to note that on iOS, the scheduleTask
method responsible for these one-off background tasks only works when the device in question is plugged into a power source. This rules out most use cases, although it is a fair assumption to make that these tasks will fire at least once every few days when the user charges the device.
重要的是要注意,在iOS上, 负责这些一次性背景任务 的 scheduleTask
方法仅在有问题的设备插入电源时有效。 这可以排除大多数用例,尽管可以公平地假设,当用户为设备充电时,这些任务至少每隔几天就会触发一次。
As we did to react-native-background-timer
, the package needs to be installed and linked to CocoaPods:
正如我们对react-native-background-timer
所做的那样,需要安装该软件包并将其链接到CocoaPods:
// install react-native-background-fetchyarn add react-native-background-fetch
react-native link react-native-background-fetch
cd ios && pod install
Once installed, the BackgroundFetch
object can be imported into a component where your background tasks can then be configured.
安装完成后,可以将BackgroundFetch
对象导入组件,然后在其中配置您的后台任务。
配置后台任务 (Configuring Background Tasks)
The Example section of the official documentation lays all the options at your disposal, most of which are targeted towards Android devices. The only configuration option iOS users have is the minimumFetchInterval
field, where the minimum interval is 15 minutes.
官方文档的“ 示例”部分列出了所有可用的选项,其中大多数是针对Android设备的。 iOS用户拥有的唯一配置选项是minimumFetchInterval
字段,其中最小间隔为15分钟。
This following example contains the bare-bones boilerplate of enabling background task management. The component, named BackgroundTasks
, is designed to wrap your application. E.g., within your top-most App component.
下面的示例包含启用后台任务管理的基本样板。 名为BackgroundTasks
的组件旨在包装您的应用程序。 例如,在您最顶层的App组件中。
You could also opt to simply include the component somewhere in your component tree without wrapping any components, with <BackgroundTasks />
.
您还可以选择使用 <BackgroundTasks />
将组件简单地包含在组件树中的某个位置而不包装任何组件 。
What we need to initialise periodic background tasks is theBackgroundFetch.configure()
method, and provide a callback function as its second argument to handle the task logic:
我们需要初始化周期性的后台任务是BackgroundFetch.configure()
方法,并提供一个回调函数作为其第二个参数来处理任务逻辑:
import React, { useEffect } from 'react'
import BackgroundFetch from 'react-native-background-fetch'
export const BackgroundTasks = (props) => {
useEffect(() => {
BackgroundFetch.configure({
minimumFetchInterval: 15,
}, async (taskId) => {
console.log("Received background-fetch event: " + taskId);
/* process background tasks */
BackgroundFetch.finish(taskId);
}, (error) => {
console.log("RNBackgroundFetch failed to start");
});
}, []);
return (<>
{props.children}
</>);
}
A couple of important points about this setup:
关于此设置的几个重要点:
BackgroundFetch
is configured within auseEffect
hook, that will only be executed once straight after the component renders. This is due to the empty array[]
in its re-render dependents, that does not giveuseEffect
any opportunities to re-render during the component lifecycle.在
useEffect
挂钩中配置BackgroundFetch
,该挂钩仅在组件呈现后立即执行一次。 这是由于其重新渲染依赖项中的空数组[]
,在组件生命周期内没有为useEffect
任何重新渲染的机会。The
minimumFetchInterval
field of15
(minutes) is by no means a guaranteed interval, instead acting as the minimum duration at which the task can be executed.minimumFetchInterval
字段为15
(分钟)绝不是保证间隔,而是充当可以执行任务的最小持续时间。BackgroundFetch.finish()
is the all important call to end the background task process. If this is not called, iOS will eventually kill the task itself and will likely assign battery-blame to your app.BackgroundFetch.finish()
是结束后台任务过程的所有重要调用。 如果不调用此选项,iOS最终将终止任务本身,并可能为应用程序分配电池负担。
BackgroundFetch.finish()
is the equivalent of BackgroundTimer.stop()
from the react-native-background-timer
package we discussed earlier.
BackgroundFetch.finish()
与 我们前面讨论 的 react-native-background-timer
包中 的 BackgroundTimer.stop()
等效 。
After
useEffect()
is defined, the component simply renders its child components, enablingBackgroundTasks
to wrap any subset of components within your app.定义
useEffect()
之后,该组件将仅呈现其子组件,从而使BackgroundTasks
可以将应用程序中组件的任何子集包装起来。
实施后台任务 (Implementing background tasks)
Background task logic can now be coded within configure()
’s callback function. There are a few scenarios where background tasks become very useful:
现在可以在configure()
的回调函数中编码后台任务逻辑。 在某些情况下,后台任务变得非常有用:
- Downloading content for offline access, populating your app’s database in the process. 下载内容以供离线访问,并在此过程中填充应用程序的数据库。
- API calls to external services, such as distributing content to 3rd party platforms. This is useful for analysing app usage. 通过API调用外部服务,例如将内容分发到第三方平台。 这对于分析应用使用情况很有用。
- Where content is regularly updated such as in large online shops or social media apps, “cache warming” can be done via a background process, whereby the app is prepping content before the user opens the app back into the foreground. 在定期更新内容(例如在大型在线商店或社交媒体应用程序中)的地方,可以通过后台过程来完成“缓存预热”,即在用户将应用程序打开回到前台之前,应用程序正在准备内容。
- Authentication and security measures, such as clearing authentication data on device after a certain period, forcing the user to sign in to the service again. This could be coincided with token expiry on the server-side. 身份验证和安全措施,例如在一定时间后清除设备上的身份验证数据,迫使用户再次登录该服务。 这可能与服务器端的令牌到期同时发生。
Perhaps the simplest way of integrating tasks — especially in the event that there are many tasks to be processed — is with a queuing system. This can be achieved though AsyncStorage
whereby various tasks, represented as stringified JSON objects, can be queued up accordingly:
集成任务的最简单方法(尤其是在要处理的任务很多的情况下)可能是使用排队系统。 这可以通过AsyncStorage
来实现, AsyncStorage
,各种任务(以字符串化的 JSON对象表示)可以相应地排队:

Now when the next background task is executed, you can send all your tasks from the AsyncStorage
queue to your remote server in one request, where those tasks can then be processed.
现在,当执行下一个后台任务时,您可以在一个请求AsyncStorage
所有任务从AsyncStorage
队列发送到远程服务器,然后可以在其中处理这些任务。
检查后台任务状态 (Checking background task status)
In the event background tasks are denied or restricted (that could also be a result of earlier iOS devices not supporting multi-tasking — very rare these days), it is good practice to check the app’s background task authorization status.
如果后台任务被拒绝或限制(这也可能是较早的iOS设备不支持多任务处理的结果-如今非常罕见),那么最好检查应用程序的后台任务授权状态 。
This is useful for debugging purposes, where you could log events on your backend servers in the event background tasks are being denied, to track whether such occurrences are widespread throughout your install base.
这对于调试目的很有用,在后台任务被拒绝的情况下,您可以在后端服务器上记录事件,以跟踪此类事件是否在整个安装基础中普遍存在。
The authorization status can be invoked with the BackgroundFetch.status(callback)
method. If we dive into the response codes of this method, we can see exactly what each code represents:
可以使用BackgroundFetch.status(callback)
方法调用授权状态。 如果深入研究此方法的响应代码,我们可以确切地看到每个代码代表什么:
// taken from react-native-background-fetch type definitions* | BackgroundFetch.STATUS_RESTRICTED | Background fetch updates are unavailable and the user cannot enable them again. For example, this status can occur when parental controls are in effect for the current user. |* | BackgroundFetch.STATUS_DENIED | The user explicitly disabled background behavior for this app or for the whole system. |* | BackgroundFetch.STATUS_AVAILABLE | Background fetch is available and enabled. |
These statuses are enumerated, so the actual result will be either 0
, 1
or 2
. We will be wanting the 2
result in an ideal case.
这些状态被枚举,所以实际结果可能是0
, 1
或2
。 我们将希望在理想情况下获得2
结果。
Note that parental controls can also affect background task execution. In more severe cases, the user may disable background tasks altogether. These scenarios further highlight that background tasks should supplement an app, rather than act as a dependency.
请注意,家长控制也会影响后台任务的执行。 在更严重的情况下,用户可能会完全禁用后台任务。 这些场景进一步强调了后台任务应该补充应用程序,而不是充当依赖项。
The official documentation simply demonstrates a switch statement to handle the status result, that can be useful for the logging use case mentioned earlier. Another way to use BackgroundFetch.status()
is within a Promise, that other components can then import and utilise to handle the resulting status.
官方文档仅演示了用于处理状态结果的switch语句,这对于前面提到的日志记录用例很有用。 使用BackgroundFetch.status()
另一种方法是在Promise中,其他组件随后可以导入并利用其处理结果状态。
This is what that Promise may look like:
这就是Promise的样子:
// BackgroundTasks.jsximport BackgroundFetch from 'react-native-background-fetch'export const backgroundFetchStatus = () =>
new Promise((resolve) => {
BackgroundFetch.status((status) => {
resolve(status);
})
});
With backgroundFetchStatus
defined, any component could import and use the method accordingly, such as disabling background task settings if the status returns disabled or restricted:
定义了backgroundFetchStatus
,任何组件都可以相应地导入和使用该方法,例如,如果状态返回禁用或受限,则禁用后台任务设置:
// BackgroundSettings.jsx
import React, { useEffect, useState } from 'react'
import { backgroundFetchStatus } from './BackgroundTasks'
const AppSettings = (props) => {
const [backgroundTasksRestricted, setBackgroundTasksRestricted] = useState(false);
const checkBackgroundAuthStatus = async () => {
const res = backgroundFetchStatus();
setBackgroundTasksRestricted(
res === 2 ? false : true
);
}
useEffect(() => {
checkBackgroundAuthStatus();
}, []);
return(
<>
{!backgroundTasksRestricted &&
// Background Settings UI
}
</>
);
}
在服务器端处理后台任务 (Handling background tasks server-side)
Now, when designing background tasks that interact with your server-side API, it is very important to not assume that a result will be successfully returned.
现在,在设计与服务器端API交互的后台任务时,非常重要的一点是,不要假设将成功返回结果。
Say a background task wishes to manipulate your server-side database, perhaps to update usage statistics for a particular user. The app needs to be sure the task successfully completed, and mark that task as completed locally on the device. The problem lies in that the background task may be killed or finished before the response is received from the server. This means the result will not be handled, and the task will not mark as completed. The next time the background task fires, the same job will be processed on the server-side.
假设有一个后台任务希望操纵您的服务器端数据库,也许是为了更新特定用户的使用情况统计信息。 应用程序需要确保任务成功完成,并将该任务标记为在设备上本地完成。 问题在于, 在从服务器收到响应之前,后台任务可能会被终止或完成 。 这意味着将不处理结果,并且该任务也不会标记为已完成。 下次启动后台任务时,将在服务器端处理同一作业。
Here is a timeline of that process, where the task is already finished before the server returns a response:
这是该过程的时间轴,在服务器返回响应之前任务已经完成:
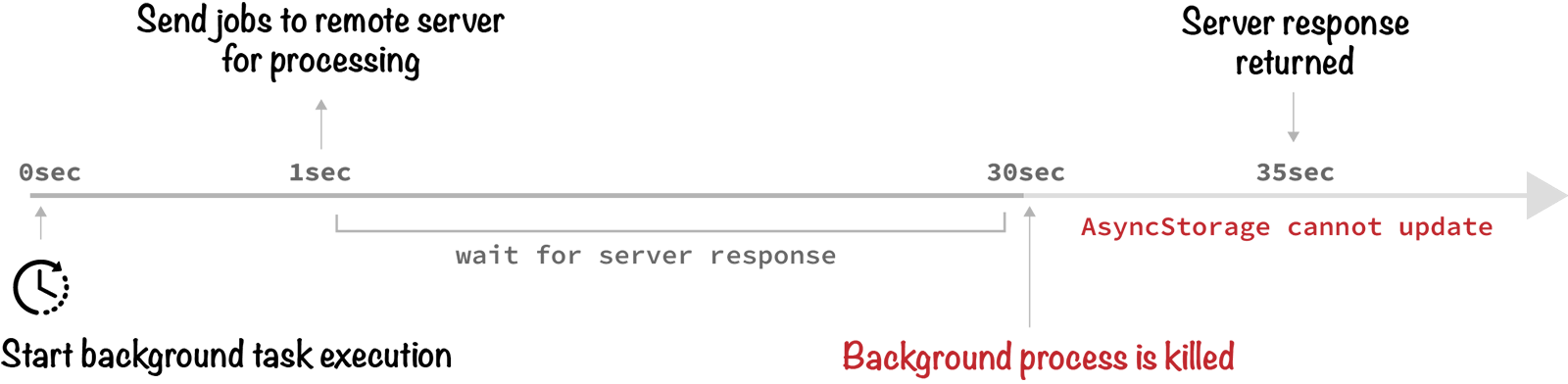
What can we do to prevent this? One way is to introduce the concept of a task manager on your server side, that tracks whether a particular task has been initiated
, completed
, or other states suitable for the task in question.
我们怎么做才能防止这种情况? 一种方法是在服务器端引入任务管理器的概念,该概念可跟踪特定任务是否已initiated
, completed
或适合于所讨论任务的其他状态。
A task status could simply be represented in your database as a status
field within background_tasks
table or collection.
任务状态可以简单地在数据库中表示为 background_tasks
表或集合中 的 status
字段 。
Now when your background task initiates a server-side task, the API can check whether the task has already been initiated, in which case no further processing will take place. In addition, completed tasks can be returned to the client without further processing:
现在,当您的后台任务启动服务器端任务时,API可以检查任务是否已经启动,在这种情况下,将不会进行进一步的处理。 此外,已完成的任务无需进一步处理即可返回给客户端:
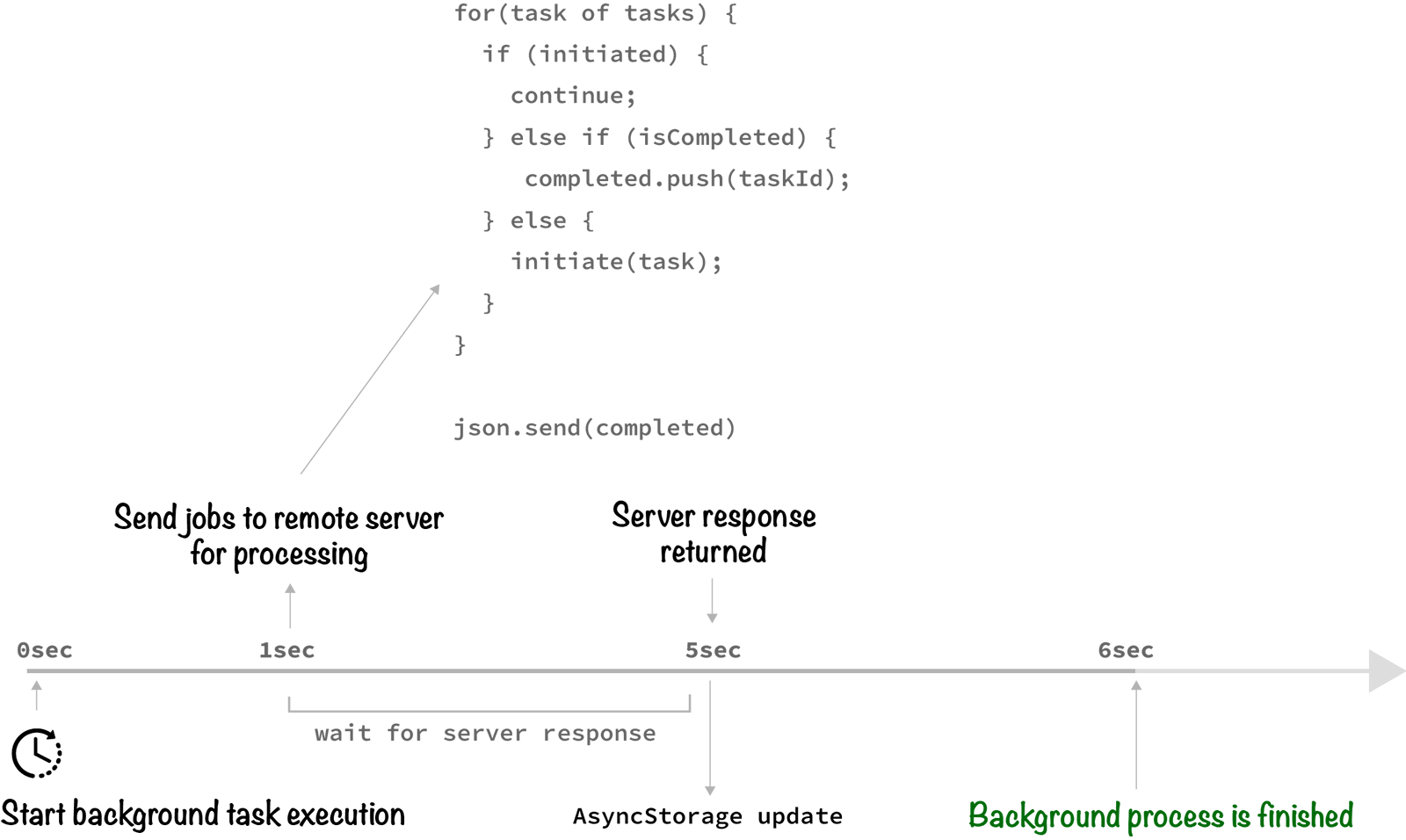
Because this API does not wait for processes to finish executing, the response is returned much quicker. This approach resolves two issues:
由于此API不会等待进程完成执行,因此可以更快地返回响应。 此方法解决了两个问题:
- The task will not be processed on the server more than once, even if the server is contacted upon subsequent background tasks 即使在后续的后台任务上联系了服务器,该任务也不会在服务器上多次处理
A failed server response delivery will not result in duplicate task processing. Subsequent requests containing the same task will simply be ignored by the server. Once the server successfully sends a response back to the background task,
AsyncStorage
tasks can be amended.服务器响应传递失败将不会导致重复的任务处理。 服务器将忽略包含相同任务的后续请求。 一旦服务器成功将响应发送回后台任务,就可以修改
AsyncStorage
任务。
This is quite an elegant, lightweight solution when processing tasks, where every second counts.
当处理任务时,这是一个非常优雅,轻巧的解决方案,每一秒钟都很重要。
权限更改也是后台过程 (Permission Changes are also Background Processes)
Although a subject of another article, permission changes can also be considered background tasks, and apps must react to these changes accordingly too.
尽管是另一篇文章的主题,但是权限更改也可以视为后台任务,应用程序也必须相应地对这些更改做出React。
For example, jumping into the Settings of your device and turning off notifications happens in the background (in relation to the app), or on the OS level of the device, that your app needs to then react to in order to stay consistent with OS level settings.
例如,跳入设备的设置并关闭通知是在后台(与应用程序有关)或设备的操作系统级别上发生的,然后您的应用程序需要做出React才能与操作系统保持一致级别设置。
A common scenario of doing this is when the camera is disabled, or when an app becomes too notification-happy, prompting a user to turn them off completely. In these cases, UX within the app must be updated to reflect the disabled feature, preferably with an explanation to the user, and even provide a link back to Settings to turn on that feature again.
常见的情况是禁用相机,或者当应用变得过于满足通知要求时,提示用户完全将其关闭。 在这些情况下,必须更新应用程序内的UX以反映禁用的功能,最好向用户说明,甚至提供指向“设置”的链接以再次启用该功能。
Simple solutions can be adopted to keep your app UX up to date pertaining to event listeners on app state that, upon changes in that state, can update your UX accordingly. To obtain permissions in a straight-forward API, the react-native-permissions
package can be used.
可以采用简单的解决方案来使您的应用UX与事件监听器相关的应用状态保持最新,一旦状态发生变化,便可以相应地更新您的UX。 要在简单的API中获得许可,可以使用react-native-permissions
包。
综上所述 (In Summary)
This article has outlined React Native specific tooling to implement background task management with your apps, and has outlined their benefits and limitations. iOS has strict background task limitations that were undoubtedly introduced to manage device resources and battery drain. Because of this, background tasks must be designed to act quick, while ensuring the task itself is finished promptly to prevent restrictions imposed by iOS on your app.
本文概述了使用本程序实现后台任务管理的特定于React Native的工具,并概述了它们的优点和局限性。 iOS具有严格的后台任务限制,无疑是引入来管理设备资源和电池消耗的。 因此,必须将后台任务设计为快速行动,同时确保任务本身Swift完成,以防止iOS对您的应用施加限制。
We also briefly looked at a potential issue on the server side, where a background task is killed before a server response is delivered — this is particularly common on devices with poor network connectivity, and can happen through no fault of the developer’s design choices. A task manager on the server side can prevent duplicate processing of background tasks, keeping your data consistent.
我们还简要地研究了服务器端的潜在问题,即在交付服务器响应之前杀死了后台任务-这在网络连接性较差的设备上尤为常见,并且不会因开发人员的设计选择错误而发生。 服务器端的任务管理器可以防止重复执行后台任务,从而使数据保持一致。
翻译自: https://medium.com/@rossbulat/react-native-background-task-management-in-ios-d0f05ae53cc5
ios 后台任务