Unlike Java, where we need to write everything, a Kotlin compiler can understand the code and write the boilerplate code under the hood. For example, it can infer types in variable declarations. This increases productivity and saves time.
与Java需要编写所有内容的Java不同,Kotlin编译器可以理解代码并在后台编写样板代码。 例如,它可以推断变量声明中的类型。 这样可以提高生产率并节省时间。
If you search the web, you’ll find tons of ways in which Kotlin solved many of Java’s pain points and how features of Kotlin aim to make development more fun.
如果您在网络上搜索,您将找到大量的方式来解决Kotlin的许多Java难题,以及Kotlin的功能旨在使开发变得更加有趣的目的。
本文的重点 (Takeaway from the article)
With cool features and the sexy syntax of Kotlin, it’s easy to get lost in the process and use them more desperately in a way they aren’t intended to use (it might not be a mistake, but it’s definitely not going to be ideal code). This might lead your team to severe problems in the long run. So in this article, you’re going to learn how to use Kotlin-exclusive features in a better way.
凭借出色的功能和Kotlin的性感语法,很容易在过程中迷失方向,并以一种不希望使用的方式更加绝望地使用它们(这可能不是一个错误,但绝对不是理想的代码)。 从长远来看,这可能会导致您的团队遇到严重问题。 因此,在本文中,您将学习如何更好地使用Kotlin专有功能。
种类 (Types)
Let’s start with basic things like val
and var
. If you’ve previously been a Java developer, you might feel better using val
and var
instead of mentioning data types all of the time.
让我们从val
和var
类的基本内容开始。 如果您以前是Java开发人员,则最好使用val
和var
而不是总是提到数据类型。

Wouldn’t it be better if we could see the type of the variable listOfTvShows
? We can do this by enabling hints in Android Studio.
如果我们可以看到变量listOfTvShows
的类型,那会更好吗? 我们可以通过在Android Studio中启用提示来做到这一点。
Navigate as follows: Settings > Editor > General > Appearance — you need to check “Show parameter name hints.”
导航如下:设置>编辑器>常规>外观-您需要选中“显示参数名称提示”。

After that, you’ll see the hints of the variable that wasn’t declared with data types. Have a look at the listOfTvShows
variable after enabling the hint option.
之后,您将看到未使用数据类型声明的变量提示。 启用提示选项后,看看listOfTvShows
变量。

Well, this is useful and handy in many situations. But what if someone in your team added an integer to the list. Have a look.
好吧,这在许多情况下都是有用且方便的。 但是,如果您团队中的某人向列表中添加了整数,该怎么办。 看一看。

Now it won’t show any error, but it’ll change the type of the list from String
to Any
, and you don’t have any control over it. So use val
and var
without data types only for simple declarations, but when it comes to situations like this, it’s better to take time and mention the data type.
现在,它不会显示任何错误,但是会将列表的类型从String
更改为Any
,并且您无法对其进行任何控制。 因此,仅对简单声明使用不带数据类型的val
和var
,但是在遇到这种情况时,最好花些时间并提及数据类型。

Kotlin also provides functions with lambdas, where you can write the body of the function and return the result without mentioning what the return type is. Have a look.
Kotlin还提供带有lambda的函数,您可以在其中编写函数的主体并返回结果,而无需提及返回类型是什么。 看一看。

This is good but not for complex functions; you’ve got to keep in check of the context and choose an appropriate format to use. These types of Kotlin features are designed to ease your development, not to manipulate us in the long run.
这很好,但不适用于复杂的功能。 您必须检查上下文并选择合适的格式来使用。 这些类型的Kotlin功能旨在简化您的开发,从长远来看不会操纵我们。
Keep in mind the code should speak for itself, so I suggest you take a moment and explicitly specify the type. There are times when the tool won’t be there to show you hints — like in the PR review.
请记住,代码应该说明一切,因此我建议您花一点时间并明确指定类型。 有时,该工具不会在那里向您显示提示-就像在PR评论中一样。
它很棒,但是重命名它会很棒 (`it` Is Great, But Renaming ‘it’ Will Be Awesome)
Functions are first-class citizens in Kotlin; you can use a function as a parameter, use it as a higher-order function to carry a set of code and execute them somewhere else, you can return a value, and a hundred other things.
职能是Kotlin的一等公民; 您可以将函数用作参数,将其用作高阶函数来携带一组代码并在其他地方执行它们,可以返回值以及一百种其他东西。
To help you a little bit in this world of handling functions, the creators thought of simplifying single-parameter lambda functions by providing the parameter it
so you don’t need to mention it explicitly. Have a look.
为了帮助您在这个世界上的处理函数一点点,创作者通过提供参数想到简化单参数lambda函数it
,所以你并不需要明确地提到它。 看一看。

It’s a nice feature, and I recommend its use. But you should remember it won’t be helpful all the time. Have a look at the following code.
这是一个不错的功能,我建议使用它。 但是您应该记住,它不会一直有用。 看下面的代码。
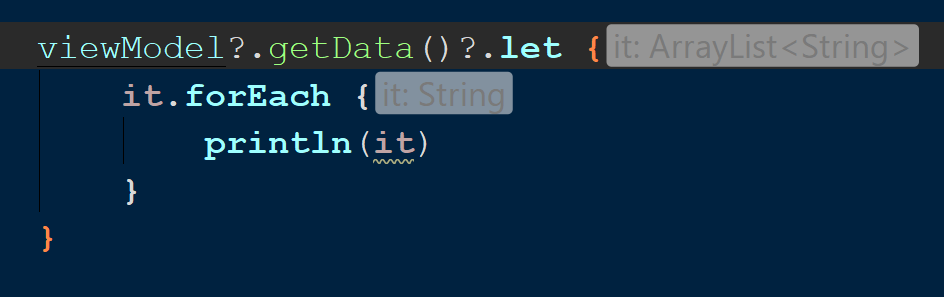
In this piece of code, inside let
, it
is an ArrayList
, but inside the for
loop, it’s a String
. It’s a bit confusing, right? Now, let’s see the same code without hints.
在这一段代码,里面let
, it
是一个ArrayList
,但里面for
循环,这是一个String
。 这有点令人困惑,对吧? 现在,让我们看看没有提示的相同代码。
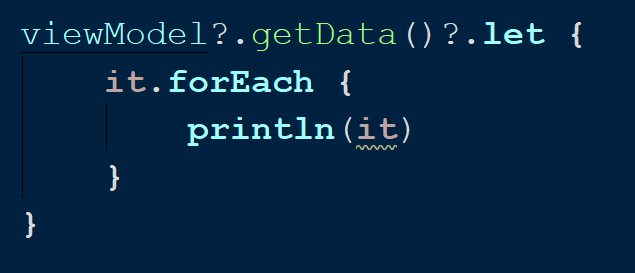
It’s insane, right? There’s no readability. This is what happens when you’re desperate about using Kotlin too much.
疯了吧? 没有可读性。 当您非常渴望使用Kotlin时,会发生这种情况。
Maintainability and readability are two of the main aspects of code, so be aware of them. To improvise the code, we have to rename it
with an appropriate name. Have a look.
可维护性和可读性是代码的两个主要方面,因此请注意它们。 为了临时编写代码,我们必须使用适当的名称it
进行重命名。 看一看。
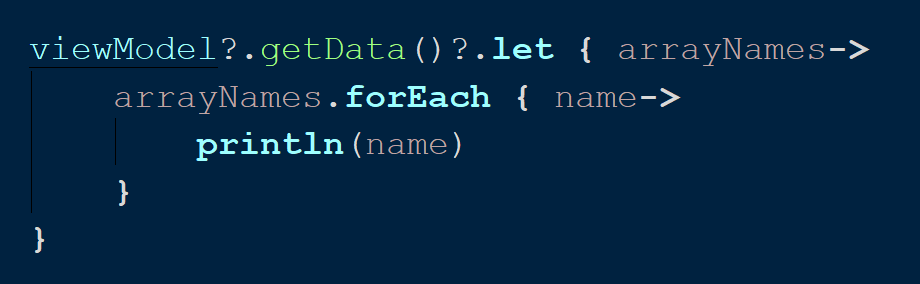
Again, you don’t need to rename it
inside of the forEach
loop, but it’s your choice. Don’t get caught up with too much clarity or clutter. This type of coding will help your fellow team members understand the context a little bit faster.
同样,您不需要在forEach
循环中重命名it
,但这是您的选择。 不要被太多的清晰度或混乱所困扰。 这种编码将帮助您的团队成员更快地了解上下文。
范围功能 (Scope Functions)
Scope functions in Kotlin are another super sexy feature — you can use them to get a functional-programming look to your code. They’re also handy in situations like let
for the null check, apply
in viewholders
, and more. I’m not going to discuss the most controversial subject — where to use a specific scope function; rather, I’d like to talk about how not to use them.
Kotlin中的作用域函数是另一个超级性感的功能-您可以使用它们来使代码具有功能编程的外观。 在let
进行null检查,在viewholders
apply
viewholders
情况下,它们也很方便。 我不会讨论最有争议的主题-在哪里使用特定范围的函数; 相反,我想谈谈如何不使用它们。
Let’s take a piece of code in which we don’t use any of our Kotlin nature. Have a look.
让我们来看一段不使用任何Kotlin性质的代码。 看一看。
This is nice — maybe something like we’d do in Java a couple of years ago with all the repetitive code and stuff. Let’s jazz it up with our Kotlin nature. Have a look.
很好-也许像几年前我们在Java中所做的那样,使用了所有重复的代码和东西。 让我们以Kotlin的性质来爵士吧。 看一看。
There’s always a good intention behind writing this type of code, like scoping things and minimizing the boilerplate code. But nesting the scope function isn’t one of the Kotlin-style guidelines. Even in my enterprise-level coding, I write nested scopes because of my desire to write Kotlin-style code.
编写此类代码的背后总是有一个良好的意图,例如确定范围并最小化样板代码。 但是嵌套范围功能并不是Kotlin风格的准则之一。 即使是在企业级编码中,由于我想编写Kotlin风格的代码,所以我也会写嵌套作用域。
However, we need to control the urge to use scope functions extremely. Have a look at this rewritten code with simple Kotlin features.
但是,我们需要控制使用范围函数的冲动。 看看这些具有简单Kotlin功能的重写代码。
This might not be the most concise code, but, in my opinion, it’s the best way to go for now without nesting any scope functions. Writing code in Kotlin doesn’t mean you have to use Kotlin features all the time.
这可能不是最简洁的代码,但是在我看来,这是暂时不嵌套任何作用域函数的最佳方法。 用Kotlin编写代码并不意味着您必须一直使用Kotlin功能。
扩展功能很棒,但不要过度扩展 (Extension Functions Are Awesome, But Don’t Overextend Them)
Extension functions in Kotlin were great when I first heard of them in a demo; it’s like I was in some magical land where I could write my style of code almost on anything I’m working on. But I was wrong — let’s see why.
当我第一次在演示中听说Kotlin的扩展功能时,它们很棒。 就像我在一个神奇的地方一样,我几乎可以在任何工作中编写自己的代码风格。 但是我错了-让我们看看为什么。
“Kotlin provides the ability to extend a class with new functionality without having to inherit from the class or use design patterns. Such functions are available for calling in the usual way as if they were methods of the original class. This mechanism is called extension functions.” — Creators of Kotlin
“ Kotlin提供了使用新功能扩展类的能力,而不必从类中继承或使用设计模式。 这些函数可以按常规方式调用,就好像它们是原始类的方法一样。 这种机制称为扩展功能。” -Kotlin的创造者
Turns out extension functions aren’t meant to jazz up your syntax (doesn’t mean it’s wrong to do so), but the primary intention is to implement additional functionality on a class to implement features without creating a new class.
事实证明,扩展功能并不是要弄乱您的语法(这并不意味着这样做是错误的),但是主要目的是在类上实现其他功能以实现功能而不创建新类。
It’s easy to get caught up with extension functions in the sense of writing more concise code; I do this, too. Sometimes I make all the functions as extension functions in the process of converting a remote model to a local database model, and I don’t realize it at the moment.
从编写更简洁的代码的意义上来说,很容易被扩展函数所困扰。 我也是。 有时,在将远程模型转换为本地数据库模型的过程中,我会将所有功能都用作扩展功能,但目前我还没有意识到。
Somehow I came across a talk by Huyen Tue Dao at KotlinConf 2019, which I mention at the end of this article. She showed me how far I went with the obsession of using extensions effectively.
不知何故,我在2019年KotlinConf上遇到了Huyen Tue Dao的演讲,我在本文末尾提到。 她向我展示了我对有效使用扩展的痴迷程度。
奖金 (Bonus)
To learn more about Kotlin, read the previous parts of this Advanced Programming With Kotlin series:
要了解有关Kotlin的更多信息,请阅读“使用Kotlin进行高级编程”系列的先前部分:
To learn more about Kotlin coroutines and other advanced features of Kotlin, read the following articles:
要了解有关Kotlin协程和Kotlin的其他高级功能的更多信息,请阅读以下文章:
结论 (Conclusion)
Before we wrap up, I’d like to thank Huyen Tue Dao. I’ve learned many of the concepts I mentioned here from the talk she gave at KotlinConfig 2019. If you’re interested, have a look:
在结束之前,我要感谢Huyen Tue Dao。 我从她在KotlinConfig 2019上的演讲中学到了很多我在这里提到的概念。 如果您有兴趣,请看一下:
Thank you for reading.
感谢您的阅读。
翻译自: https://medium.com/better-programming/advanced-android-programing-in-kotlin-part-4-187b88fea048