flutter 单例
应用程序中如何使用服务? (How are Services used in Applications?)
It works like a drive-through. The Service is always there like the person that hands you your food in the fast food restaurant of your choice. The UI requests the data from the Service whenever it needs data.
它的工作原理就像是开车驶过 。 服务始终像在您选择的快餐店中将食物交给您的人一样。 UI在需要数据时从服务请求数据。
Like you, when you go to the drive through when you are hungry. Then the waiter receives your order and requests your order in the kitchen.
像你一样,当你饿着肚子去开车的时候。 然后服务员收到您的订单,并在厨房里要求您下订单。
In this case the kitchen is your Backend, the Waiter (Service) is then “serving” the food(data) to you (UI). Receiving your menu choice is like getting the parsed data into the frontend.
在这种情况下,厨房就是您的后端 ,然后服务员(服务)将食物(数据)“提供”给您( 用户界面 )。 接收菜单选项就像将解析的数据放入前端一样。
Using Google Firebase makes it very easy to build smartphone applications from scratch because Google will handle the authentication and a basic database service for you.
使用Google Firebase可以非常轻松地从头开始构建智能手机应用程序,因为Google会为您处理身份验证和基本数据库服务。
The following article will describe how to create a Singleton Service which can be accessed from everywhere in your application handling your Firebase dependent data.
以下文章将介绍如何创建一个Singleton服务,可以从处理Firebase依赖数据的应用程序中的任何位置访问该服务。
如何将Google Firebase集成到Flutter项目中: (How to integrate Google Firebase into your Flutter Project:)
I will not deep dive into that topic because Google already has a really good explanation.
我不会深入探讨该主题,因为Google已经有了一个很好的解释 。
Just make sure that the following dependencies are in your pubspec.yaml-file:
只要确保您的pubspec.yaml文件中存在以下依赖项:
firebase_auth will enable the Firebase Authentication API for your application e.g. Basic Auth, Sign In with Google etc. The Firebase Service will later access the Firebase Realtime database. For that usage we will use the firebase_database package. firebase_core enables the Firebase Core API, which enables multi Core Apps from Firebase itself. For the basic implementation we will not call any of the Core Functions. But depending on the Application you develop it might be helpful to use some of those API’s.
firebase_auth将为您的应用程序启用Firebase身份验证API,例如基本身份验证,通过Google登录等。Firebase服务稍后将访问Firebase Realtime数据库。 对于这种用法,我们将使用firebase_database包。 firebase_core启用Firebase Core API,该API从Firebase本身启用多个Core Apps。 对于基本实现,我们将不调用任何核心功能。 但是根据您开发的应用程序,使用其中一些API可能会有所帮助。
The FirebaseService will be a Singleton. For the Singleton Service I decided to use the package get_it. The Singleton design pattern is a part of creational patterns and basically means that only one object of the class exists.
FirebaseService将是一个Singleton。 对于Singleton Service,我决定使用包get_it 。 Singleton 设计 模式是创作 模式的一部分 和 基本上意味着该类仅存在一个对象。
为什么我们要创建FirebaseService? (Why do we create a FirebaseService?)
In general, while developing you will come to the point when the logic of your application growths.
通常,在开发过程中,您会遇到应用程序逻辑增长的问题。
Thinking about good programming, the way to go is the separation of UI (widgets) and logic. This will prevent failures and will also keep the logic consistent all over the application. This is also called Separation of Concerns.
考虑好的编程,要走的路是将UI(小部件)和逻辑分离。 这将防止出现故障,并使整个应用程序中的逻辑保持一致。 这也称为关注点分离 。
Speaking explicitly of the Service we want to create here it would look like that:
明确地说我们要在此处创建的服务看起来像这样:
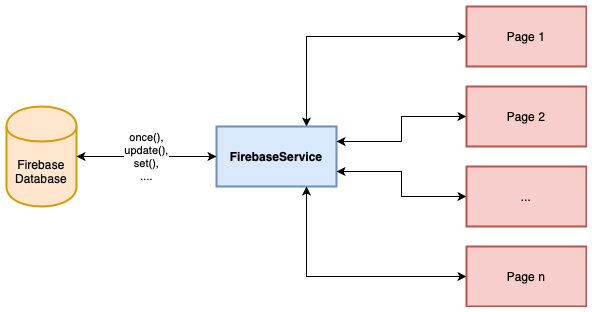
The Separation of Concerns leads a bit into the topic Software Quality:
关注点分离使主题进入软件质量 :
“Quality is never an accident; it is always the result of intelligent effort.”
“质量绝非偶然; 这始终是明智努力的结果。”
John Ruskin
约翰·罗斯金
Being a good software developer is also thinking about Software Quality. Two out of four measurement techniques for good software quality are Maintainability and Reliability.
做一名优秀的软件开发人员还要考虑软件质量 。 为了获得良好的软件质量,四分之二的测量技术是可维护性和可靠性 。
For the achievement of Maintainability and Reliability the Separation of Concerns is very important.
为了实现可维护性和可靠性 ,关注点的分离非常重要。
For example, updating your Firebase database paths could happen to your project. First, maybe one of the keys for your data is named: lists.
例如,更新Firebase数据库路径可能会发生在您的项目中。 首先,也许您的数据键之一被命名为: lists 。
Calling the data for that key from the Flutter application would look like that:
从Flutter应用程序调用该键的数据应如下所示:
But then you decide that lists are maybe not the best name and rename it to: grocery_lists. So you would also have to rename any occurences in the frontend like this:
但随后您确定列表可能不是最好的名称,然后将其重命名为: 杂货清单 。 因此,您还必须像这样重命名前端中的所有事件:
Without the separation of logic and widget you would need to check your whole application, rename every reference you are using and recheck the whole functionality.
如果没有逻辑和小部件的分离,您将需要检查整个应用程序,重命名您正在使用的每个引用,然后重新检查整个功能。
Having it all in one service leading to just one file that has to be adapted enables code Maintainability!
将所有功能整合到一个服务中,只需要修改一个文件就可以实现代码的可维护性 !
设置FirebaseService (Setting up the FirebaseService)
After a bit of explanation we will now create our FirebaseService.
经过一番解释,我们现在将创建FirebaseService。

I really like to separate my code and give my project a clear folder structure.
我真的很想分离我的代码,并给我的项目一个清晰的文件夹结构。
So all widget files are placed in the package pages, our FirebaseService will be placed in the package service. I would also recommend to create a model package and place all your data model classes in there.
因此,所有小部件文件都放置在软件包页面中 ,我们的FirebaseService将放置在软件包服务中 。 我还建议创建一个模型包,并将所有数据模型类放在其中。
Create a new dart file in this package and name it “firebase_service.dart”.
在此程序包中创建一个新的dart文件,并将其命名为“ firebase_service.dart” 。
Add the code below to the dart-File:
将以下代码添加到dart文件中:
FirebaseAuth is going to handle your way of authentication which will later be shown in a coding example for sign in etc.
FirebaseAuth将处理您的身份验证方式,稍后将在代码示例中显示登录方式等。
The FirebaseDatabase Instance will handle your data access to your Firebase database itself.
FirebaseDatabase实例将处理您对Firebase数据库本身的数据访问。
Now we switch to the main.dart-file. This is where our library get_it kicks in. Add the code below to your main.dart-file. Any other Singleton Service that you are going to create while coding can be registered here as well. Just do it the same way as with the FirebaseService.
现在,我们切换到main.dart -file。 这是我们的库get_it插入的位置。将下面的代码添加到main.dart -file中。 您还将在此处注册编码时要创建的任何其他Singleton服务。 只需执行与FirebaseService相同的方法即可。
Firebase中的身份验证 (Authentication in Firebase)
Speaking about authentication and Firebase there are many options:
说到身份验证和Firebase,有很多选择:

In this article signing in with E-Mail will be explained a bit. We will now extend the FirebaseService and add the signIn, signUp and signOut — methods:
在本文中,将对使用电子邮件登录进行一些说明。 现在,我们将延长FirebaseService并添加签到 , 注册和signOut -方法:
After adding this methods either extend your login — page in the page package or create a login — page:
添加此方法后可以扩展您的登录 -页面在页面包或创建一个登录-页面:
Add the FirebaseService to your login — page and get the running instance with get_it. Then create or extend your signIn, signUp — method with the calls to the FirebaseService.
将FirebaseService添加到您的登录页面,并使用get_it获取正在运行的实例。 然后使用对FirebaseService的调用来创建或扩展您的signIn , signUp —方法。
使用Firebase获取数据 (Getting data with Firebase)
If you already have a page where you want to call the FirebaseService from, move to that page. Otherwise create a new page like the one below:
如果您已经有了要从其调用FirebaseService的页面,请移至该页面。 否则,创建一个新页面,如下所示:
First things first. In this step we add the FirebaseService to the page and call the Singleton instance with get_it. I also added the MyExampleObject to later assign the response from our FirebaseService.
首先是第一件事。 在这一步中,我们将FirebaseService添加到页面中,并使用get_it调用Singleton实例。 我还添加了MyExampleObject,以便以后从FirebaseService分配响应。
But how do we get the data from the Service? Before we continue on the page we have to switch back to the FirebaseService. Below, our Authentication methods like signIn etc. add the following method:
但是,我们如何从服务中获取数据? 在继续浏览页面之前,我们必须切换回FirebaseService 。 在下面,我们的身份验证方法(如signIn等)添加以下方法:
Use the child() — Method to setup the correct database call for your project. This function will return a DataSnapshot, which is probably not the object that we want to access on our page. In my Application I wrote a factory that is parsing the DataSnapshot in the correct shape of the MyExampleObject. This approach looks like that:
使用child() -方法为您的项目设置正确的数据库调用。 此函数将返回一个DataSnapshot,它可能不是我们要在页面上访问的对象。 在我的应用程序中,我编写了一个工厂 ,该工厂以MyExampleObject的正确形状解析DataSnapshot 。 这种方法看起来像这样:
If you choose this approach you can include the fromJson and toJson — methods as well. Therefore add the following dependencies to your pubspec.yaml:
如果选择这种方法,则可以同时包含fromJson和toJson方法。 因此,将以下依赖项添加到pubspec.yaml中 :
I also have an article describing how to use the fromJson and toJson — methods with the Shared Preferences on your phone.
我也有一篇文章介绍如何将fromJson和toJson —方法与手机上的“ 共享首选项”一起使用。
Switch back to the page that you want to fill with data from the database. Before integrating the data into our view, create a function to call the backend:
切换回要用数据库中的数据填充的页面。 在将数据集成到我们的视图之前,创建一个函数来调用后端:
As already explained Firebase itself returns the data type DataSnapshot which has to be parsed in a class in the frontend. Of course you could also work with the value of the DataSnapshot which has the type Map<dynamic, dynamic>, but to be honest this would destroy the approach of good software quality. As we are working with Futures because of the async calls to the backend we have to add a FutureBuilder to the creation of the body part from our widget. Using the FutureBuilder will enable our widget to already set up the view. When the async call is finished our values that we receive from the backend will be set to the MyExampleObject. If we would use this without the FutureBuilder our MyExampleObject would be null on the first call leading to an error in the widget creation.
如前所述,Firebase本身返回数据类型DataSnapshot ,该数据类型必须在前端的类中进行解析。 当然,您也可以使用类型为 Map <dynamic,dynamic>的DataSnapshot的值,但是说实话,这会破坏良好软件质量的方法。 由于与后端的异步调用,我们在与Futures一起工作时,我们必须在小部件的主体部分的创建中添加FutureBuilder 。 使用FutureBuilder将使我们的小部件已经设置好视图。 当异步调用完成时,我们从后端收到的值将设置为MyExampleObject 。 如果我们不使用FutureBuilder就使用它 我们的MyExampleObject在第一次调用时将为null,从而导致小部件创建错误。
The coding example below shows how to use the FutureBuilder and also how to display an loading indicator while the async calls are pending.
下面的编码示例显示了如何使用FutureBuilder,以及如何在异步调用挂起时显示加载指示器。
结论 (Conclusion)
This article outlined how the design principle Separation of Concerns can be easily used to separate calls to the backend(logic) from the widgets(UI) which is also providing Software Quality to a project.
本文概述了如何轻松地使用设计原则“关注点分离” 将对后端(逻辑)的调用与小部件(UI)分开,这也为项目提供了软件质量 。
Besides that it was shown how to use the library get_it to create a Singleton Service, this approach can be used for all services in the Application.
除了显示了如何使用库get_it创建Singleton Service之外,该方法还可以用于Application中的所有服务。
All this knowledge was used to built a Singleton Service for simplyfying data access to Backend Services from your Flutter Application.
所有这些知识都用于构建Singleton Service,以简化从Flutter应用程序对后端服务的数据访问。
翻译自: https://medium.com/swlh/how-to-create-a-singleton-firebase-service-in-flutter-15d7a5ed596b
flutter 单例