没有直接的Apple API (No Direct Apple API)
I am sure we all have struggled at some point in customizing small UI tweaks for UISearchBar
.
我确信我们在为UISearchBar
自定义小的UI调整时都UISearchBar
。
We know that Apple API’s are not flexible to customize the UISearchBar
like accessing UITextField
, It was in iOS 13 Apple has made this searchTextField
API available but it was not easy in previous iOS 10– 12 versions and there are many apps which support this iOS versions.
我们知道Apple API不能像访问UITextField
那样灵活地自定义UISearchBar
,这是在iOS 13中。Apple已经提供了searchTextField
API,但在以前的iOS 10至12版本中并不容易,并且有很多应用程序都支持此iOS版本。
Changing Placeholder color, Changing Background color or change UITextField
rightView the list is very long.
更改占位符颜色,更改背景颜色或更改UITextField
rightView列表非常长。
I have wasted so many hours every time to tweak different parts of UISearchBar
so thought of listing them to one place and sharing it to our iOS community.
我每次都浪费了很多时间来调整UISearchBar
不同部分,所以想到将它们列出到一个地方并分享给我们的iOS社区。
Here is the list of tweak we will implement today
这是我们今天将要实施的调整列表
Accessing
UITextField
reference w.r.t to all iOS versions访问所有iOS版本的
UITextField
参考- Adding Left Padding from Search Icon 从搜索图标添加左填充
Changing
UITextField
Left View (Search Icon)更改
UITextField
左视图(搜索图标)- Changing Placeholder Color 更改占位符颜色
Showing Loader in
UISearchBar
在
UISearchBar
显示加载程序Changing
UITextField
Right View(Bookmark Icon) inUISearchBar
在
UISearchBar
更改UITextField
右视图(书签图标)Consistent
UISearchBar
UI一致的
UISearchBar
UI
让我们从UITextField
开始 (Let’s start with UITextField
)
We know that Apple has made not easy for us to access the UITextField
reference on different OS. So here is the code to help u guys for safe access of UITextField
across all iOS versions.
我们知道Apple让我们不容易在不同的操作系统上访问UITextField
引用。 因此,以下代码可帮助您跨所有iOS版本安全访问UITextField
。
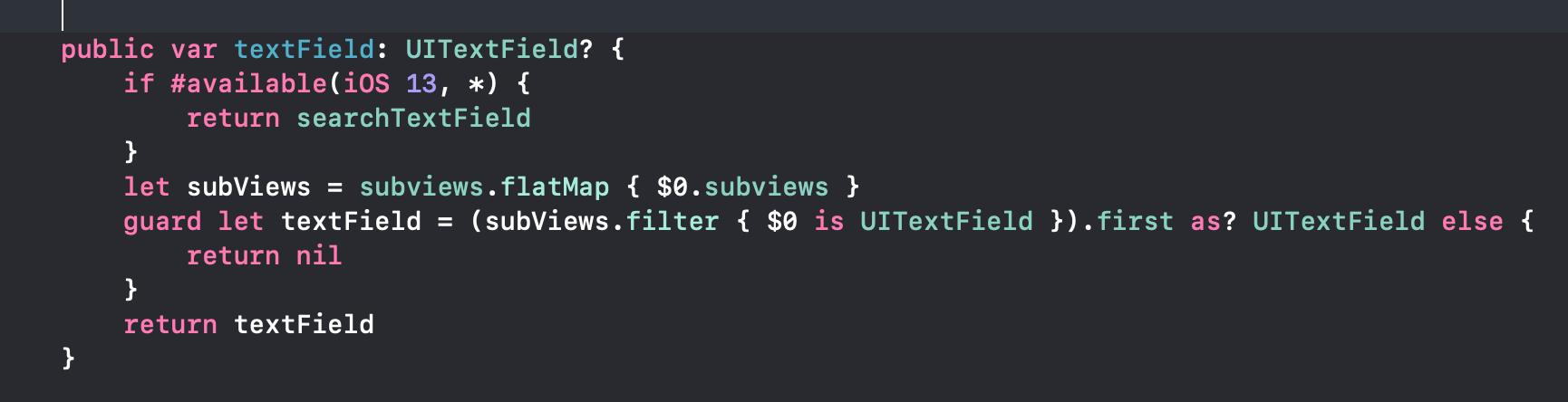
This will help us to customize the font, Background color, Search icon of UITextField
and many more, Below is example snippet
这将帮助我们自定义字体,背景色, UITextField
搜索”图标等等,以下是示例片段
searchBar.textField?.textColor = UIColor.gray
searchBar.textField?.font = // Your font
UISearchBar中的左填充 (Left Padding in UISearchBar)

Now with one line of code we can customize left icon of UISearchBar
and also add padding to it.
现在,只需一行代码,我们就可以自定义UISearchBar
左侧图标,并为其添加填充。
searchBar.setLeftImage(UIImage(named: "dark mode")!, with: 20)


更改占位符颜色 (Change Placeholder Color)
Note: This will not work if you call in viewDidLoad
, call it in viewDidAppear
注意 :如果您在viewDidLoad
中调用,在viewDidAppear
调用它,则将无法正常工作
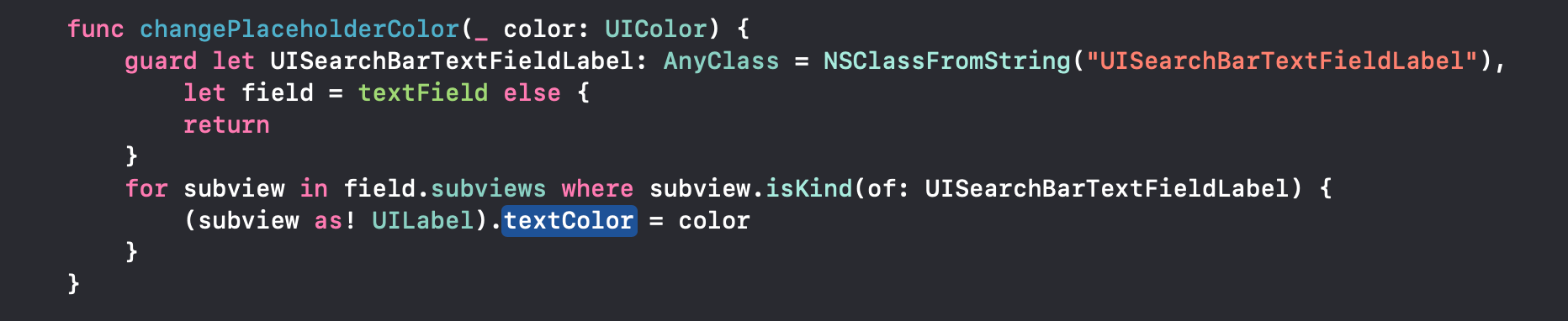
Now with just single line of code you can change UISearchBar
placeholder color. Note: This call doesn’t work in viewDidLoad
call this above method in viewDidAppear
现在只需一行代码,您就可以更改UISearchBar
占位符的颜色。 注意:此调用在viewDidLoad
不起作用在viewDidAppear
调用上述方法
searchBar.changePlaceholderColor(UIColor.orange)

UISearchBar中的UIActivityIndicatorView (UIActivityIndicatorView in UISearchBar)

searchBar.isLoading = true // show loader
searchBar.isLoading = false // hide loader

UISearchBar(UITextField)右视图图像 (UISearchBar(UITextField) Right View Image)
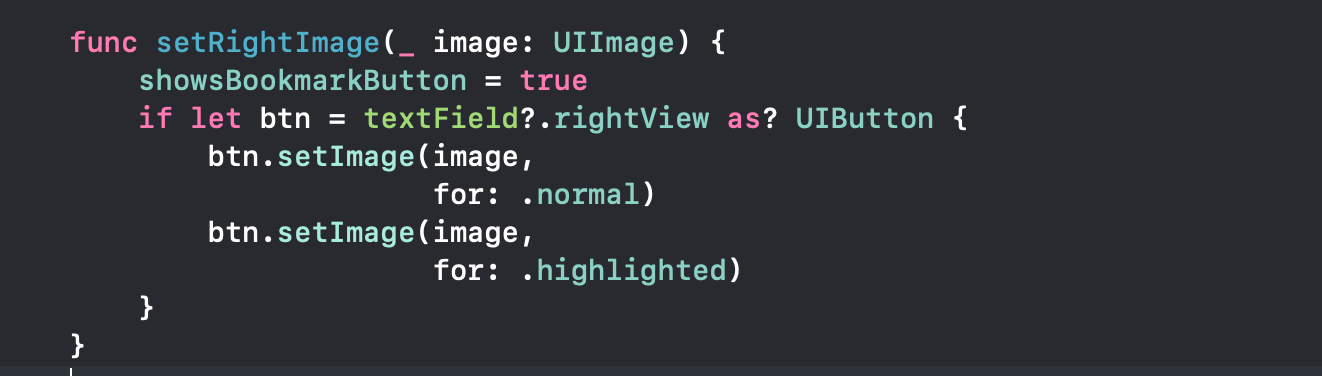
Now with just single line of code you can change UISearchBar
right view icon. Note: This call doesn’t work in viewDidLoad
call this above method in viewDidAppear
现在只需一行代码,您就可以更改UISearchBar
右视图图标。 注意:此调用在viewDidLoad
不起作用在viewDidAppear
调用上述方法

To invoke your method on click of Right View of UISearchBar
override below method of UISearchBarDelegate
要单击UISearchBar
的右视图,请单击下面的UISearchBarDelegate
方法来调用您的方法
// Set Custom Right View
searchBar.setRightImage(normalImage: UIImage(named: “filter”)!,
highLightedImage: UIImage(named: “filter_selected”)!)// Override method
func searchBarBookmarkButtonClicked(_ searchBar: UISearchBar) {
// Filter Action
tappedFilter()
}
UISearchBar UI一致性 (UISearchBar UI Consistency)

Actually I debugged UISearchBar
so much because I need custom UI for Dark mode and Light mode. So this is final piece which helped me to accomplish the result, Basically keep the UISearchBar
consistent across all iOS versions for any mode.
实际上,我对UISearchBar
很多调试,因为我需要针对暗模式和亮模式的自定义UI。 因此,这是帮助我完成结果的最后一部分,基本上可以在任何模式下的所有iOS版本中保持UISearchBar
一致性。




Here is the code of UISeachBar extension to customize it according to your requirement. I know this extension still has scope of improvements this is just for reference, I am sure you may have to customise it for your project needs.
这是UISeachBar扩展的代码,可根据您的要求对其进行自定义。 我知道此扩展程序仍有改进的范围,仅供参考,我相信您可能必须根据项目需要对其进行自定义。
感谢您的阅读 (Thank you for reading)
I hope this article helps you in customizing different UI properties of UISearchBar
. Do share your valuable feedback.
我希望本文能帮助您自定义UISearchBar
不同UI属性。 分享您的宝贵意见。
学分 (Credits)
- StackOverflow Community StackOverflow社区