python3.5 新功能
重点 (Top highlight)
这个通告 (The Announcement)
On the 25th of March Apple announced that the release process for Swift 5.3 has begun, it’s a major change since it will improve the overall language quality and performance, and will make Swift available for multiple platforms as Windows and Linux.But let’s see in detail what’s new.
苹果在3月25日宣布,Swift 5.3的发布过程已经开始,这是一个重大变化,因为它将提高整体语言的质量和性能,并使Swift可以在Windows和Linux等多个平台上使用。什么是新的。
列举案例作为协议证人 (Enum Cases as Protocol Witnesses)
Currently a class extending a protocol needs to match exactly the protocol’s requirements, for example if we write static requirements within a protocol:
当前,扩展协议的类需要完全符合协议的要求,例如,如果我们在协议中编写静态要求:
And then we try to conform an enum to our protocol, we’ll face the following error:
然后我们尝试使枚举符合我们的协议,我们将面临以下错误:
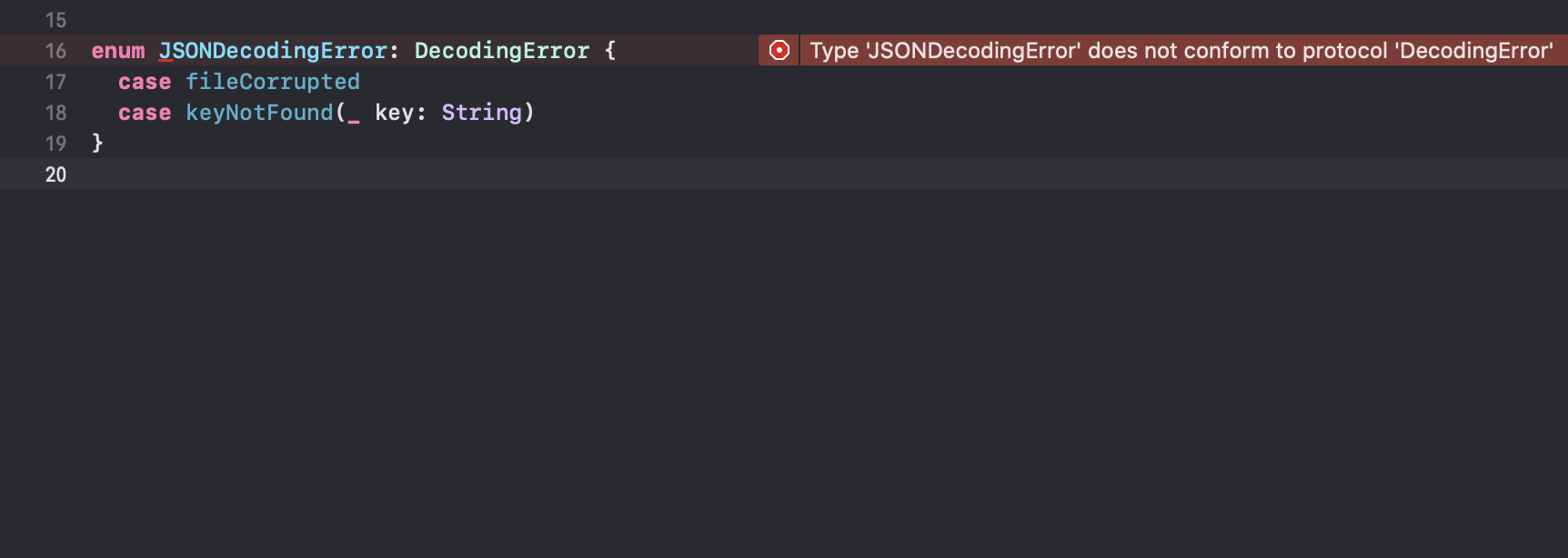
With SE-0280 this will no longer cause a compiling error.
使用SE-0280,将不再导致编译错误。
多个尾随闭包 (Multiple Trailing Closures)
Swift 5.3 finally introduced the possibility to use multiple trailing closures.
Swift 5.3最终引入了使用多个尾随闭包的可能性。
一些背景 (Some Background)
First of all for those who don’t know what a trailing closure is, it’s a simple syntactic sugar that we can use when we have a closure parameter in a function:
首先,对于那些不知道尾随闭包是什么的人,它是一个简单的语法糖,当我们在函数中具有闭包参数时可以使用它:
and we can simply call the function as follows:
我们可以简单地如下调用该函数:
问题 (The problem)
This is an amazing feature and allows us to write cleaner and easier to read code, however it’s currently limited only to the last parameter of a function as we can see in the following example:
这是一个了不起的功能,使我们能够编写更清晰,更容易阅读的代码,但是,目前仅限于函数的最后一个参数,如以下示例所示:
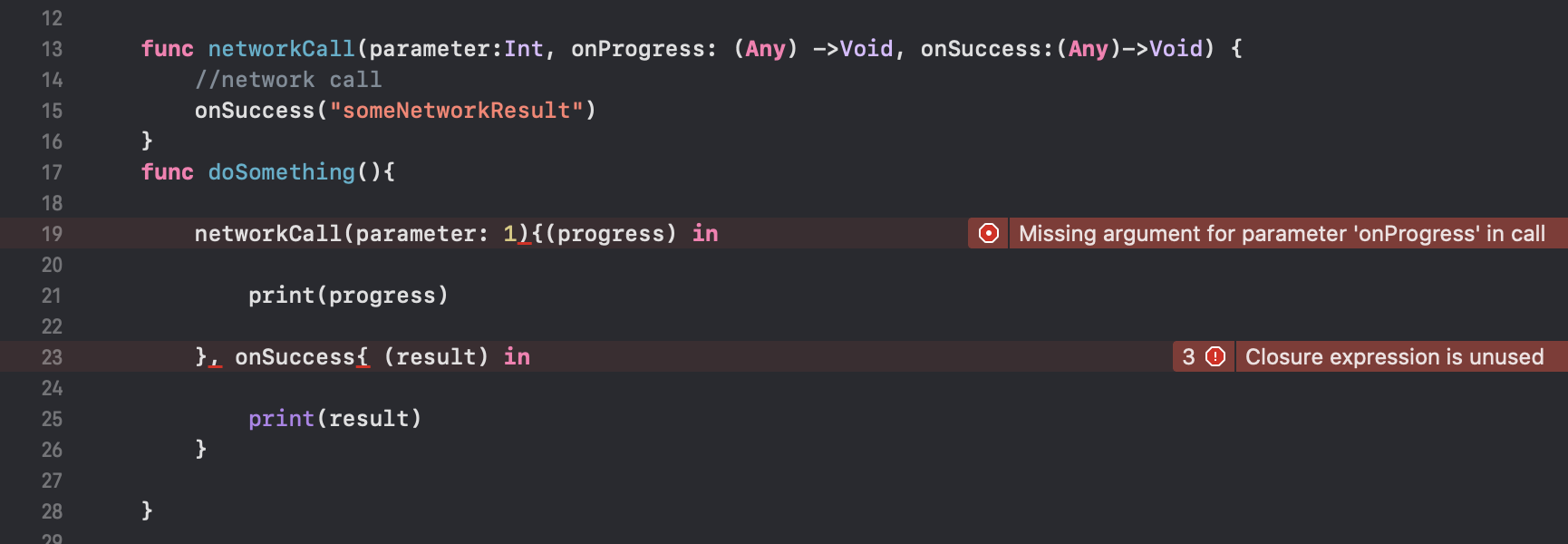
拟议的解决方案 (The Proposed Solution)
With SE-0279 we will be allowed to insert extra trailing closures, just by adding extra labels, therefore the following code will compile.
使用SE-0279,我们可以通过添加额外的标签来插入额外的结尾闭包,因此将编译以下代码。
一种新的Float16类型 (A New Float16 Type)
This is pretty straight forward, I’ll report the motivation explained by apple:
这很简单,我将报道苹果解释的动机:
The last decade has seen a dramatic increase in the use of floating-point types smaller than (32-bit)
Float
. The most widely implemented isFloat16
, which is used extensively on mobile GPUs for computation, as a pixel format for HDR images, and as a compressed format for weights in ML applications.在过去的十年中,使用小于(32位)
Float
类型的使用急剧增加。 实现最广泛的是Float16
,它在移动GPU上广泛用于计算,作为HDR图像的像素格式以及作为ML应用程序中权重的压缩格式。Introducing the type to Swift is especially important for interoperability with shader-language programs; users frequently need to set up data structures on the CPU to pass to their GPU programs. Without the type available in Swift, they are forced to use unsafe mechanisms to create these structures.
将类型引入Swift对于与着色器语言程序的互操作性尤为重要。 用户经常需要在CPU上设置数据结构以传递到其GPU程序。 如果没有Swift中可用的类型,他们将被迫使用不安全的机制来创建这些结构。
You can find additional informations on SE-0277.
您可以在SE-0277上找到更多信息。
多模式捕获条款 (Multi-Pattern Catch Clauses)
Currently swift allows only a single error type for each catch clause, that means that if we define the following enum
目前swift仅允许每个catch子句使用单一错误类型,这意味着如果我们定义以下枚举
Assuming someNetworkCall is a function that throws a NetworkError, and we want to handle both errors, we will need to write two separate catch clauses:
假设someNetworkCall是一个引发NetworkError的函数,并且我们要处理这两个错误,我们将需要编写两个单独的catch子句:
With SE-0276 we will be allowed to handle both errors on a single catch clause, with the following code:
使用SE-0276,我们可以使用以下代码在单个catch子句中处理这两个错误:
在非连续元素上添加收集操作 (Add Collection Operations on Noncontiguous Elements)
Currently we can use Range<Index> to refer to multiple consecutive positions in a Collection, but there’s not a simple way to do this for discontiguous positions, based on some condition.
当前,我们可以使用Range <Index>来引用Collection中的多个连续位置,但是根据某些条件,没有简单的方法可以对不连续的位置执行此操作。
For example if we have an array of numbers from 0 to 15 and we want to get a sub range of the Array, let’s say with only even numbers, the language doesn’t provide any way to get it, or even to get a sub Array with elements with non contiguous positions, but we can only select a range of contiguous elements:
例如,如果我们有一个从0到15的数字数组,并且想要获得Array的子范围,那么假设只有偶数,则该语言不提供任何方式来获取它,甚至无法获得子对象具有不连续位置的元素的数组,但是我们只能选择一系列连续元素:

SE-0270 adds a super useful “RangeSet” Method that will allow us to get a subRange of all the indexes of the elements that satisfy a specific condition, therefore, if we want to get only even numbers from the previous array, the following code will work:
SE-0270添加了超级有用的“ RangeSet”方法,该方法将使我们能够获得满足特定条件的元素的所有索引的subRange,因此,如果我们仅想从前一个数组中获得偶数,则以下代码将工作:
当引用周期不太可能发生时,提高@转义闭包中隐式自我的可用性 (Increase Availability of Implicit self in @escaping Closures when Reference Cycles are Unlikely to Occur)
Currently in closures we need to use explicit self even in some cases where reference cycles are unlikely to happen, for example because we already captured self in the current closure:
当前,在闭包中,即使在不太可能发生参考周期的某些情况下,我们也需要使用显式self,例如,因为我们已经在当前闭包中捕获了self:
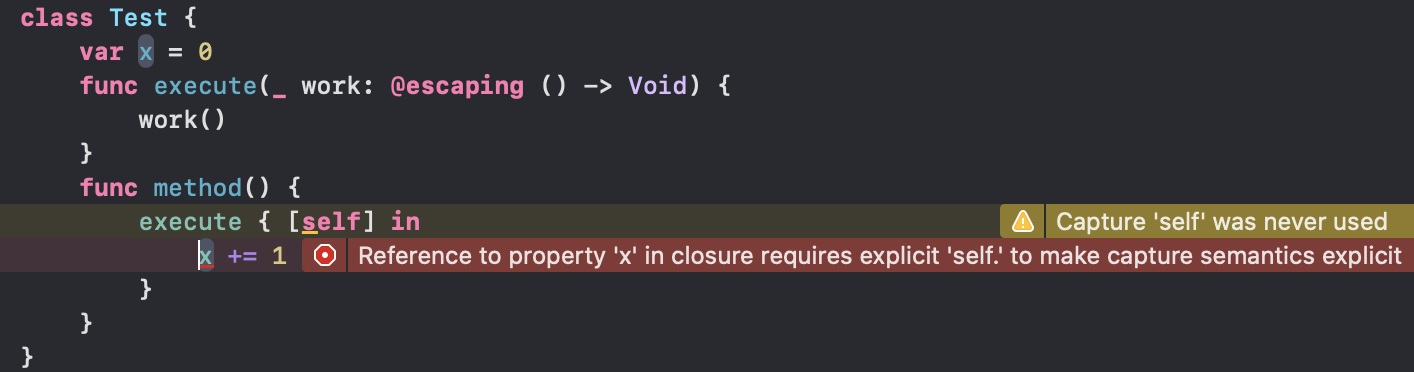
To make our code compile we need to add explicit self to x in order to reference it.
为了使我们的代码编译,我们需要向x添加显式self以便对其进行引用。
Another example is with structs, where self is a value type, therefore can’t cause a reference cycle:
另一个例子是structs,其中self是一个值类型,因此不会引起引用循环:
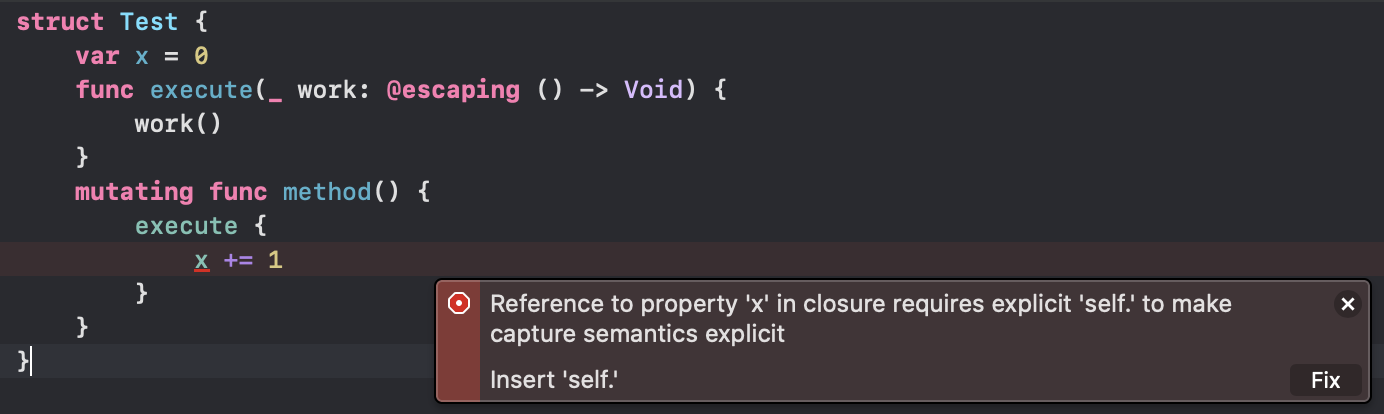
With SE-0269 we won’t need to use explicit anymore in the previous cases.
使用SE-0269 ,在先前的情况下我们将不再需要使用显式的。
didSet
语义 (Refine didSet
Semantics)
Currently, if we have a property with a “didSet” observer, the getter to get the oldValue is always called, even if observer doesn’t contain any reference to the oldValue in its body, for example the following code
当前,如果我们有一个带有“ didSet”观察者的属性,则即使观察者的主体中不包含对oldValue的任何引用,也会始终调用获取oldValue的吸气剂,例如以下代码
creates 100 copies of the array in the memory to provide the oldValue
, even though they're not used at all, causing performance issues.
在内存中创建数组的100个副本以提供oldValue
,即使根本不使用它们也会导致性能问题。
With SE-0268 the property getters will be no longer called if they’re not accessed within the didSet resulting in a performance boost
如果未在didSet中访问SE-0268 ,则将不再调用属性获取器,从而提高了性能
Where
具有内容的通用声明条款 (Where
Clauses on Contextually Generic Declarations)
Currently a where clause on a member declaration can be used only by placing the member inside a specific extension, but this can sound confusing, let’s make an example.
当前,只能通过将成员放在特定扩展名内来使用成员声明上的where子句,但这听起来可能会令人困惑,让我们举个例子。
Let’s say we want to extend the Array implementation to sort element of 3 different types, for which we want to define a different sorting logic.Currently we would need to extend Array 3 times:
假设我们要扩展Array实现以对3种不同类型的元素进行排序,为此我们要定义不同的排序逻辑,当前我们需要将Array扩展3次:
With SE-0267 we can implement that logic by simply adding the where clause to the function in a single extension:
使用SE-0267,我们可以通过在单个扩展中简单地将where子句添加到函数中来实现该逻辑:
枚举类型的综合Comparable
一致性 (Synthesized Comparable
Conformance for Enum Types)
Let’s say we want to define an Enum whose cases have an obvious semantic order, for example:
假设我们要定义一个枚举,其枚举具有明显的语义顺序,例如:
If we try to compare its values we got the following error:
如果我们尝试比较其值,则会出现以下错误:

怎么了 (What’s wrong here?)
In order to compare two Objects, they need to conform the Comparable protocol:
为了比较两个对象,它们需要遵循Comparable协议:
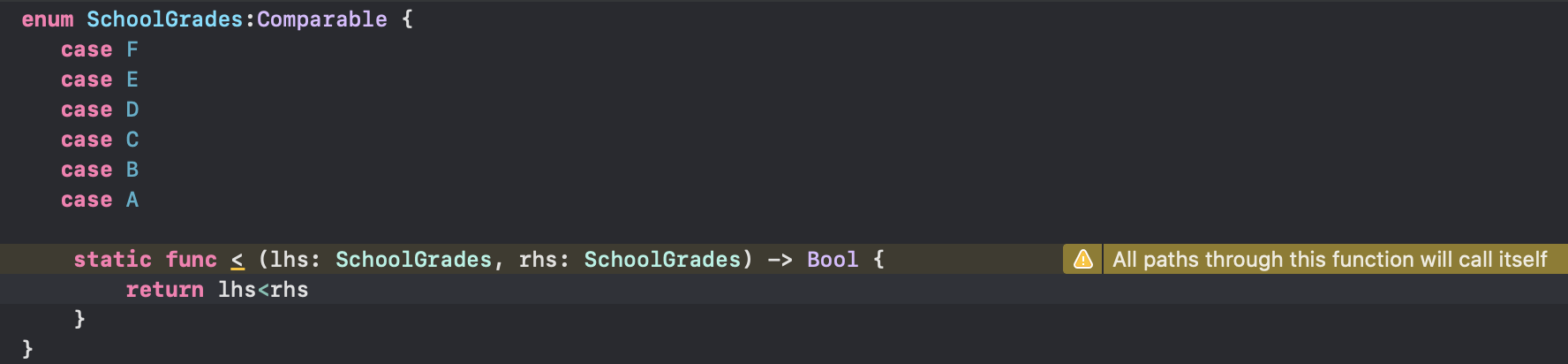
looking at the warning this will generate a loop, since the < function will be calling itself, therefore we need to compare the rawValues so that we can finally compare our grades
查看警告会生成一个循环,因为<函数将调用自身,因此我们需要比较rawValues以便最终比较等级
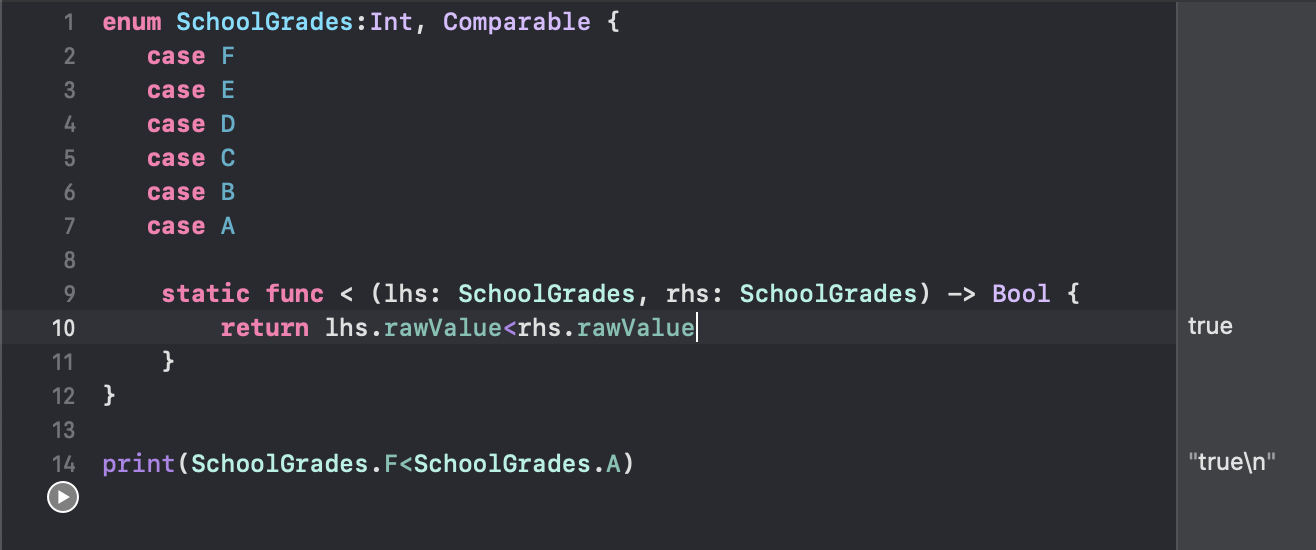
This, is just a simple case, and it adds a lot of boilerplate code.
这只是一个简单的案例,它增加了许多样板代码。
With SE-0266 this will not be necessary and we don’t need any additional code to compare Enums.
使用SE-0266,这将不是必需的,并且我们不需要任何其他代码即可比较枚举。
套件管理员变更 (Package Manager Changes)
With next Swift release there will be also several major improvements to Swift Package Manager.
在下一个Swift版本中,Swift Package Manager也将进行几项重大改进。
包管理器资源 (Package Manager Resources)
SE-0271 introduces support to resources as images and data files inside Swift Packages and makes them easily accessible, SE-0278 adds localization support for resources.
SE-0271在Swift软件包中引入了对资源的支持,例如图像和数据文件,并使其易于访问, SE-0278添加了对资源的本地化支持。
程序包管理器的条件目标依赖项 (Package Manager Conditional Target Dependencies)
SE-0273 adds the possibility for package authors to add specific dependencies differentiated by platform.
SE-0273为程序包作者添加了添加特定的依赖关系(按平台区分)的可能性。
程序包管理器二进制依赖项 (Package Manager Binary Dependencies)
Swift Package Manager currently supports only source packages, SE-0272 adds support for Binary Packages as GoogleAnalytics and many more, and this will make the Swift Package Manager adoption move faster.
Swift Package Manager当前仅支持源软件包, SE-0272增加了对Binary Packages的支持,如GoogleAnalytics等等,这将使Swift Package Manager的采用速度更快。
Let me know what’s the most useful addition in your opinion and thanks for Reading!
让我知道您认为最有用的补充是什么,感谢您的阅读!
翻译自: https://medium.com/swlh/whats-new-in-swift-5-3-ae135c9c259f
python3.5 新功能