开发react用什么软件
React (or any other front-end framework) is an extremely powerful tool to master. When it comes to building front-end interfaces, React is hands down a huge time-saver for development teams and individuals.
React(或任何其他前端框架)是一个非常强大的工具。 在构建前端接口时,React为开发团队和个人节省了大量时间。
npm packages are paramount when it comes to developer productivity and happiness. Why spend weeks reinventing the wheel when you can save time? Chances are high someone has already solved the problem you’re facing with an npm package.
对于开发人员的生产力和幸福感,npm软件包至关重要。 为了节省时间,为什么要花数周的时间重新设计轮子? 很有可能有人已经使用npm软件包解决了您面临的问题。
Without further ado, here’s a list of packages I believe every React developer should know. These packages are big time-savers — use them when you can, and often!
事不宜迟,这里是我相信每个React开发人员都应该知道的软件包列表。 这些软件包可节省大量时间,请经常使用它们!
1. React测试库 (1. React Testing Library)
The React Testing Library is a lightweight solution for testing React components. The library provides light utility functions on top of react-dom
and react-dom/test-utils
.
React Testing库是用于测试React组件的轻量级解决方案。 该库在react-dom
和react-dom/test-utils
之上提供了轻量级的实用程序功能。

安装 (Installation)
This module is distributed via npm packages.
该模块通过npm软件包分发。
yarn add --dev @testing-library/react
The library has peerDependencies
listings for react
and react-dom
— make sure you have them both installed.
该库包含有react
和react-dom
peerDependencies
列表-确保同时安装了它们。
快速示例 (Quick example)
Here’s a React component that displays a message based on its internal state. You can toggle the message on and off.
这是一个React组件,它根据其内部状态显示一条消息。 您可以打开和关闭消息。
And here’s how you can test the HiddenMessage
React component with react-testing-library
.
这是您可以使用react-testing-library
测试HiddenMessage
React组件的方法。
Check out the official documentation for more complex examples.
查看官方文档以获取更复杂的示例。
2.成帧器运动 (2. Framer Motion)
Framer Motion is a production-ready motion library for React. The motions and animations are powered by the Framer library.
Framer Motion是React的可量产的运动库。 动作和动画由Framer库提供动力。
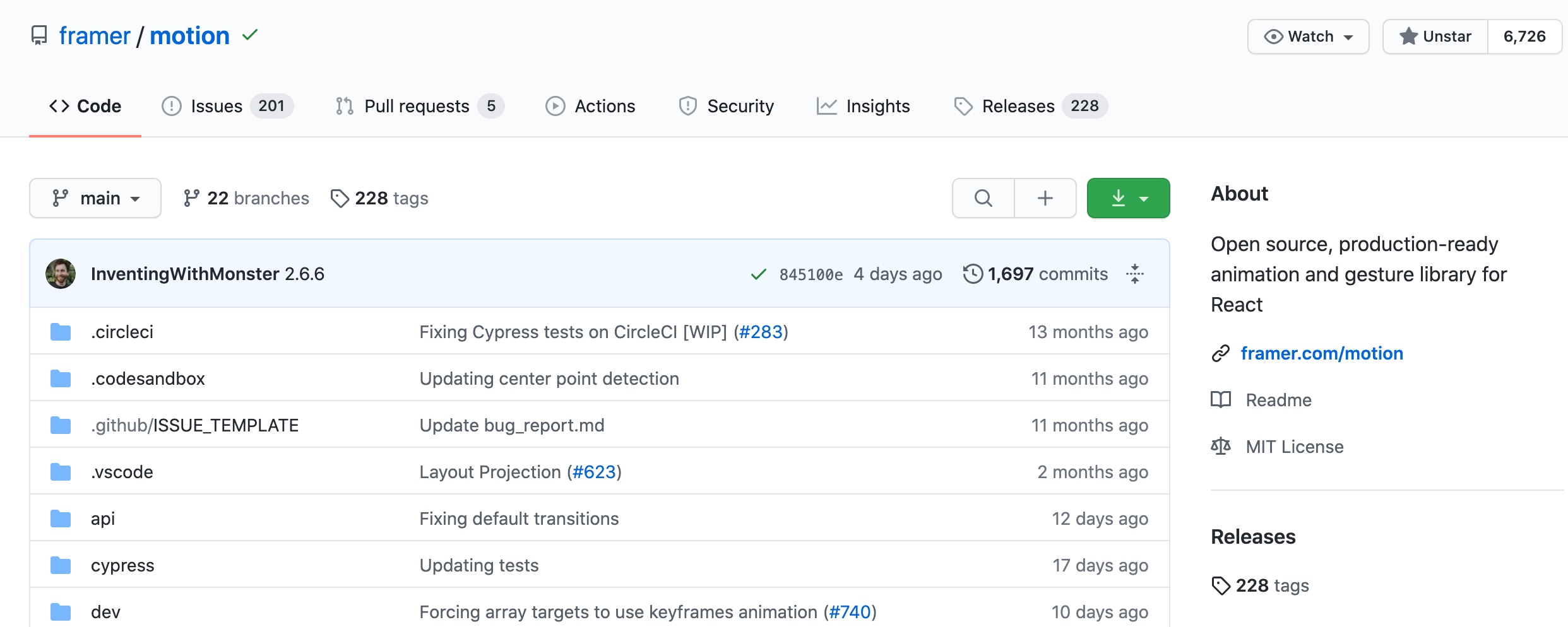
Framer Motion includes the following:
成帧器运动包括以下内容:
- Spring animations Spring动画
- Simple keyframes syntax 简单的关键帧语法
- Gestures (drag/tap/hover) 手势(拖动/点击/悬停)
- Layout and shared layout animations 布局和共享布局动画
- SVG paths SVG路径
- Exit animations 退出动画
- Server-side rendering 服务器端渲染
- Variants for orchestrating animations across components 跨组件编排动画的变体
- CSS variables CSS变量

入门 (Getting started)
Install it via Yarn:
通过Yarn安装:
yarn add framer-motion
And here’s how you use Framer Motion inside your React components.
这就是在React组件中使用Framer Motion的方法。
import { motion } from "framer-motion"export const MyComponent = ({ isVisible }) => (
<motion.div animate={
{ opacity: isVisible ? 1 : 0 }} />
)
If you’re curious for more examples, check out the article I wrote earlier about Framer Motion. You can also check out more examples for inspiration.
如果您想了解更多示例, 请查看我之前写的有关Framer Motion的文章 。 您也可以查看更多示例以获取灵感。
Read the documentation for guides and a full API reference.
阅读文档以获取指南和完整的API参考。
3.样式化的组件 (3. styled-components)
styled-components allows you to write actual CSS code to style your React components. It also removes the mapping between components and styles. Using components as a low-level styling construct could not be easier.
styled-components允许您编写实际CSS代码来设置React组件的样式。 它还删除了组件和样式之间的映射。 使用组件作为低级样式构造并不容易。

快速示例 (Quick example)
Here’s a React component styled with the styled-components library.
这是一个用styled-components库样式化的React组件。
import React from 'react';
import styled from 'styled-components';
// Create a <Title> react component that renders an <h1> which is
// centered, palevioletred and sized at 1.5em
const Title = styled.h1`
font-size: 1.5em;
text-align: center;
color: palevioletred;
`;
// Create a <Wrapper> react component that renders a <section> with
// some padding and a papayawhip background
const Wrapper = styled.section`
padding: 4em;
background: papayawhip;
`;
// Use them like any other React component – except they're styled!
<Wrapper>
<Title>Hello World, this is my first styled component!</Title>
</Wrapper>
And what you’ll see in your browser:
以及您将在浏览器中看到的内容:
Utilizing tagged template literals (a recent addition to JavaScript) and the power of CSS, we can write the following code.
利用带标签的模板文字 (JavaScript的最新功能)和CSS的强大功能 ,我们可以编写以下代码。
const Button = styled.button`
color: grey;
`;
Alternatively, you may use style objects. This allows for easy porting of CSS from inline styles, while still supporting the more advanced styled-components capabilities like component selectors and media queries.
或者,您可以使用样式对象 。 这样可以轻松地从内联样式中移植CSS,同时仍支持组件选择器和媒体查询等更高级的样式化组件功能。
const Button = styled.button({
color: 'grey',
});
Equivalent to:
相当于:
const Button = styled.button`
color: grey;
`;
See the documentation at styled-components.com/docs for more information about using styled-components.
有关使用样式化组件的更多信息,请参见styled-components.com/docs上的文档。
4.柏树 (4. Cypress)
Cypress is a fast, easy, and reliable testing library for anything that runs in a browser. Cypress solves the key pain points developers and QA engineers face when testing modern applications.
赛普拉斯是一个快速,简便,可靠的测试库,适用于在浏览器中运行的所有程序。 赛普拉斯解决了开发人员和质量保证工程师在测试现代应用程序时面临的关键难题。

Cypress makes it possible to:
赛普拉斯可以: