飞猪签到里程python
isFine = input('Are you mentally healthy, my friend?')if not isFine:
print("Please take care of yourself.Nothing in the
world is more important than you. Do things you like,
unless it's something crazy like f*cking a donkey.")continue
Python is one of the most popular languages on the planet. With its specialization in Machine Learning, Data Science, and Web Development, it’s used for almost all types of general purposes.
Python是地球上最受欢迎的语言之一。 凭借其在机器学习,数据科学和Web开发方面的专长,它几乎可以用于所有类型的通用用途。
When I was in my beginner phase of learning this language, I always used to wonder that when I’ll be able to call myself an intermediate programmer. So based on my experience, I’ll tell you about all the skills you could set as milestones in Python language. If you’re a beginner in programming, you can treat it as a guideline of topics and in what order you should learn them. And if you consider yourself Intermediate or Advanced, then maybe I’ll list some ideas that you haven’t heard of yet. And you could learn them to make your workflow much better.
当我处于学习该语言的初级阶段时,我总是想知道何时可以称自己为中级程序员。 因此,根据我的经验,我将向您介绍可以设置为Python语言里程碑的所有技能。 如果您是编程的初学者,可以将其视为主题指南,并以什么顺序学习它们。 如果您认为自己是中级或高级,那么也许我会列出一些您尚未听说的想法。 您可以学习它们以使您的工作流程变得更好。
On every topic, I’ll try to give a quick summary of it and what you should learn in them.
在每个主题上,我都将尝试对其进行简要总结,并介绍您应从中学到的知识。
First of all, let’s print ‘Hello World’ on the screen.
首先,让我们在屏幕上打印“ Hello World ”。
print("Hello World")>>> Hello World
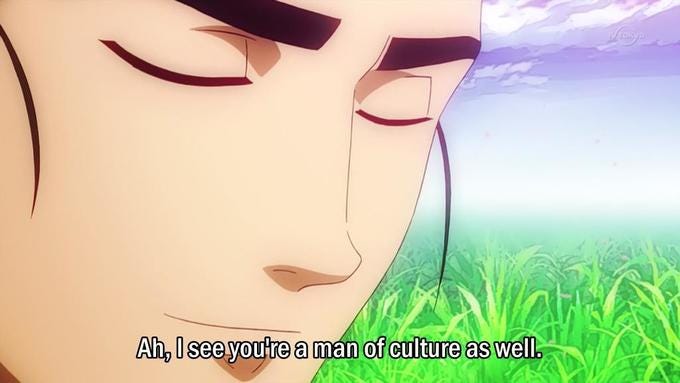
In this post, I’ll be using ‘ >>> ’ syntax to denote any kind of output. And in Python, ‘#’ is used to write comments which will not be read by the compiler. Now, without any further ado, let’s begin the master plan.
在本文中,我将使用' >>> '语法表示任何类型的输出。 在Python中,“#”用于编写注释,编译器不会读取。 现在,事不宜迟,让我们开始总体规划。
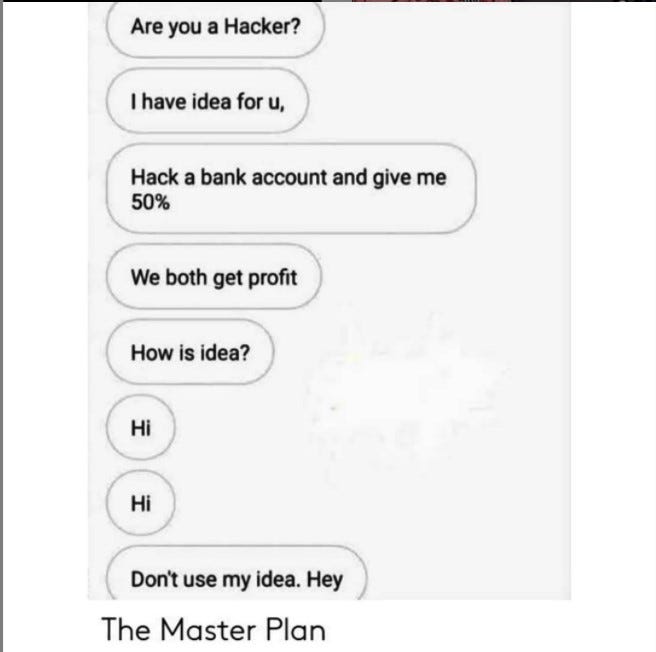
A.基本 (A. Basic)
This section is about fundamental programming topics and basic building blocks of the language. The core syntax to print something on the screen, define a variable, adding two numbers, conditions, loops, etc.
本节介绍语言的基本编程主题和基本构建块。 在屏幕上打印内容,定义变量,添加两个数字,条件,循环等的核心语法。
1.变量 (1. Variables)
You should learn what’s the need of using a variable and how do we define it in Python.
您应该了解使用变量的需求以及如何在Python中定义变量。
x = 3
y = x + 1
print(x * y)>>> 12
2.基本数据结构 (2. Basic Data Structures)
On this level, you need to know about strings, integers, floats, booleans, arrays, and dictionaries.
在此级别上,您需要了解字符串 , 整数 , 浮点数 , 布尔值 , 数组和字典 。
name = 'Chetan' # String
age = 16 # Integer
gpa = 9.7 # Float
lovesPython = True # Boolean
friends = ['Abhijeet', 'Mallick', 'Pranky'] # Arraydetails = {'color': 'white', height: '5 feet'} #dictionary
3.数学运算符 (3. Math Operators)
Exactly what we hated in primary school.
正是我们在小学时所讨厌的。
print(4 + 5) >>> 9
print(7 - 2) >>> 5print(5 * 4) >>> 20
print(7 / 2) >>> 3.5print(7 // 2) >>> 3 # Integer division (or quotient)
print(11 % 3) >>> 2 # Remainder
print(3 ** 4) >>> 81 # raised to the power
4.条件 (4. Conditions)
It’s just about true and false. You need to know how to compare two elements
这只是关于真假 。 您需要知道如何比较两个元素
print( 3 == 5 ) >>> False
print( 5 > 3 and 6 >= 6) >>> True
print( 4 < 3 or 5 > 3 ) >>> True
5.控制流程 (5. Control Flow)
It’s about making a decision based on a condition. You should know how to write ‘if’, ‘elif’, and ‘else’ statements.
这是关于根据条件做出决定的。 你应该知道如何写“ 如果”,“ELIF” 和 “ 其他”的语句。
num = -3if num > 0:
print('Number is Positive')
elif num < 0:
print('Number is Negative')
else:
print('Number is 0')>>> Number is negative
6.循环和可迭代 (6. Loops and Iterables)
You need to learn to use ‘for’ and ‘while’ loops. Also, how to iterate through iterables like arrays, string, dictionary, etc. using ‘in’ keyword.
您需要学习使用' for'和' while'循环。 另外,如何使用' in '关键字遍历数组,字符串,字典等可迭代对象。
for i in range(1, 4):
print(' Life sucks. ')>>> Life sucks.
>>> Life sucks.
>>> Life sucks.
7.功能 (7. Functions)
After learning all these basic building blocks of the language, the next thing I’d suggest you to look at is function. A function is a piece of code that does a specific task and can be used multiple times.
在学习了语言的所有这些基本组成部分之后,我建议您接下来看的是function 。 函数是执行特定任务的一段代码,可以多次使用。
def introduce(name, age):
print("You are"+ name + "and you're " + str(age) + "years old")introduce('Anshika Pattnaik', 17)
introduce('Navjeet', 16)>>> You are Anshika Pattnaik and you're 17 years old
>>> You are Navjeet and you're 16 years old
8.一些内置方法 (8. Some Built-In Methods)
Methods are a little bit different from functions. We’ll talk about them in the Intermediate section. There are some very commonly used methods already built in the language like converting a text into lowercase, sorting an array in ascending order, etc.
方法与功能略有不同。 我们将在“中间”部分中讨论它们。 语言已经内置了一些非常常用的方法,例如将文本转换为小写字母,按升序对数组进行排序等。
array = [ 4, 6, 2, 5 ]
array.sort()
print(array)>>> [ 2, 4, 5, 6 ]
9.用户输入和读取文件 (9. User Input and Reading a File)
Next, you need to learn about how to take inputs from the user or any file. You should also know how to open, read, write, and close a file using python.
接下来,您需要学习如何从用户或任何文件获取输入。 您还应该知道如何使用python打开,读取,写入和关闭文件。
name = input('Enter your name')file = open('filename.txt, 'r')
text = file.read()
file.close()
So, that’s it for the Basic section. If you are a beginner, these may sound a lot to you but trust me, these are elementary topics, and you would not take much time to master these. To learn these, I’d suggest Mike Dane’s Python Playlist. And to practice, try beginner problems on CodeChef.
所以,这就是“基本”部分。 如果您是初学者,这些内容对您可能听起来很多,但请相信我,这些都是基本主题,您不需要花费很多时间来掌握这些内容。 要学习这些内容,我建议Mike Dane的Python播放列表 。 要练习,请尝试在CodeChef上解决初学者的问题。
And if you already know all these, take a moment to appreciate yourself. Even now, you can do so many things with these skills. Like, making a height calculator or building a neural network model with 98.93% accuracy.
如果您已经了解所有这些,请花点时间欣赏一下自己。 即使是现在,您也可以使用这些技能来做很多事情。 例如,制作高度计算器或构建具有98.93%精度的神经网络模型。
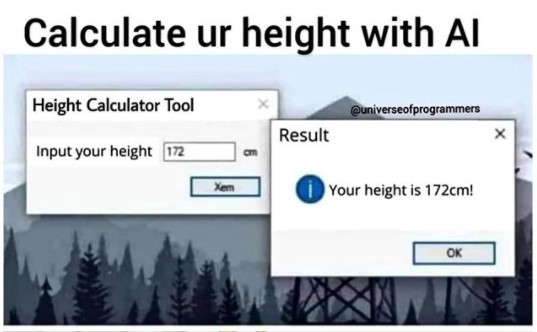
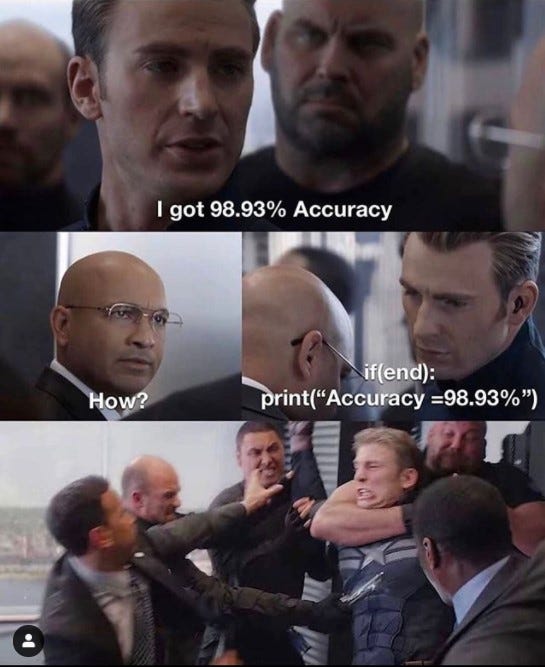
B.中级 (B. Intermediate)
Before moving ahead, it’s very important to practice everything in the beginner section as you’re going to use them at every step of your coding journey. With that said, it’s time to dive deeper into the language.
在继续之前,在初学者部分中练习所有内容非常重要,因为您将在编码过程的每个步骤中使用它们。 话虽如此,是时候深入了解该语言了。
1.面向对象的程序设计 (1. Object-Oriented Programming)
To understand anything above this level you need to have a good understanding of OOP concepts.
要了解此级别以上的任何内容,您需要对OOP概念有充分的了解。
class Student:
def __init__(self, name, class):
self.name = name
self.class = classdef changeSession(self):
self.class += 1
Here, ‘changeSession’ is a method that is kind of similar but not exactly a function. You need to learn how to define classes, objects, methods, and some basic design patterns of OOP.
在这里,“ changeSession ”是一种类似于但不完全是函数的方法。 您需要学习如何定义OOP的类,对象,方法和一些基本设计模式。
2.理解力 (2. Comprehensions)
List comprehension is an elegant way to define and create lists based on existing lists. List comprehension is generally more compact and faster than normal.
列表理解是一种基于现有列表定义和创建列表的优雅方法。 列表理解通常比普通方法更紧凑,更快速。
a = [i for i in range(1, 1000) if x % 7 == 0]b = [int(j) for j in input().split()]
3. Lambda函数 (3. Lambda Functions)
A lambda function is a small anonymous function. Using them with map method helps you to keep your code concise and clean.
Lambda函数是一个小的匿名函数。 将它们与map方法一起使用可帮助您保持代码简洁明了。
x = lambda a, b : a * b
print(x(4,5))>>> 20
4.继承 (4. Inheritance)
Inheritance allows us to define a class that inherits all the methods and properties of another class. For example, if you’re having an animal class and now you need to create a dog class then you could simply inherit all methods and properties of ‘Animal’ class into the new ‘Dog’ class as ‘Dog’ will be having all the properties of an animal.
继承允许我们定义一个类,该类继承另一个类的所有方法和属性。 例如,如果您有一个动物类,现在需要创建一个狗类,那么您可以简单地将“动物”类的所有方法和属性继承到新的“狗”类中,因为“狗”将具有所有动物的属性。
Here, we can inherit all properties from the ‘Student’ class built earlier to a new ‘FemaleStudent’ class.
在这里,我们可以继承从先前构建的“ 学生 ”类到新的“ 女 学生 ”类的所有属性。
class FemaleStudent(Student):
def __init__(self, name, class, cupSize):
super().__init__(name, class)
self.cupSize = cupSize
5.其他OOP概念 (5. Other OOP concepts)
You should also try to learn some advanced OOP concepts like Polymorphism, Data Abstraction, Dunder Methods, and Encapsulation. If you don’t get these in the very first attempt, don’t worry about it. These are some high-level concepts and understanding them completely will take time.
您还应该尝试学习一些高级OOP概念,例如多态 , 数据抽象 , Dunder方法和封装 。 如果您初次尝试时没有得到这些,请不要担心。 这些是一些高级概念,要完全理解它们需要时间。
6. Python模块和PIP (6. Python Modules and PIP)
I feel that this is the best thing about Python. Whenever I want to do something, a library exists out there, written for exactly what I need, and makes my task super easy.
我觉得这是Python最好的东西。 每当我想做某事时,就在那里存在一个图书馆,正是为我的需要而编写的,这使我的工作变得异常简单。
import mathprint(math.sqrt(9))
print(math.pi)>>> 3.0
>>> 3.141592653589793
PIP stands for ‘PIP Installs Packages’. You can use it to install third-party packages that could be of use. You should learn how to use PIP. You should also work with some libraries to get a feel for it. While beginning, I’d recommend trying BeautifulSoup (for web scraping), Requests(for sending HTTP requests), Pillow(for image processing), MoviePy(for video editing), and Selenium(for automating browsers).
PIP代表“ PIP安装软件包 ”。 您可以使用它来安装可能有用的第三方软件包。 您应该学习如何使用PIP。 您还应该使用一些库来感受一下。 开始时,建议您尝试使用BeautifulSoup (用于Web抓取), Requests (用于发送HTTP请求), Pillow (用于图像处理), MoviePy (用于视频编辑)和Selenium (用于自动执行浏览器)。
7.异步IO (7. Async IO)
Python reads and executes code line by line. Sometimes that’s not what you want. Async IO allows you to write programs that get more work done without waiting for independent tasks to finish.
Python逐行读取和执行代码。 有时那不是您想要的。 异步IO允许您编写可以完成更多工作的程序,而无需等待独立任务完成。
import asyncio
async def my_func():
await func()
8.算法和数据结构 (8. Algorithms and Data Structures)
In real-world tasks, often you get huge chunks of data and to process them efficiently you must have a good grasp of algorithms and data structures. Even huge tech giants ask most of the questions in their interviews about these. You should understand the time complexities of operations and big-O notation. To practice their problems, again you could head to Codechef or Codeforces. It’s not language compulsion but Learning these will give you an extra edge over other programmers.
在现实世界中的任务中,通常您会获得大量数据,并且要有效地处理它们,您必须对算法和数据结构有很好的了解。 甚至是大型科技巨头,在采访中也都会问这些问题。 您应该了解操作和big-O符号的时间复杂性。 要解决他们的问题,您可以再次前往Codechef或Codeforces 。 这不是语言强迫,但学习这些将使您比其他程序员有更多的优势。
9.环境 (9. Environments)
Virtual Environments are created to isolate interpreters, libraries, and script installed in a project from other virtual environments or systems. Consider a scenario in which you created an app that uses Django v2.2 without using any virtual environment. And later you updated Django installed in your system to v3.1. Then,
创建虚拟环境是为了将项目中安装的解释器,库和脚本与其他虚拟环境或系统隔离。 考虑一个场景,其中您创建了一个使用Django v2.2而不使用任何虚拟环境的应用程序。 之后,您将系统中安装的Django更新到v3.1。 然后,
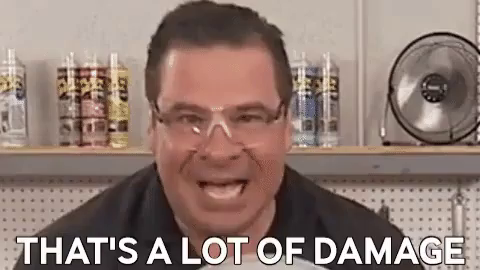
And this may cause your web app to misbehave. So, you should consider using a virtual environment.
这可能会导致您的Web应用程序行为异常。 因此,您应该考虑使用虚拟环境。
From my side, that’s it for the Intermediate section. Best way to learn these is by ‘googling’. For every topic, there are tons of tutorials and blogs out there on the internet. But don’t just watch the tutorials. Code along and also make changes to discover more possibilities.
从我这边来说,就是中级部分。 学习这些的最好方法是通过“搜索”。 对于每个主题,互联网上都有大量的教程和博客。 但是,不要只看教程。 进行编码,并进行更改以发现更多可能性。
After finishing the topics listed in the intermediate, now you’re good enough to start doing things of your interest. If you want to be a web developer, start learning Flask or Django. If you want to be a data scientist or machine learning engineer, go with NumPy, Pandas, matplotlib, sci-kit learn, TensorFlow, etc. and also start learning the math behind them.
在完成了中级列出的主题之后,现在您已经可以开始做自己感兴趣的事情了。 如果您想成为Web开发人员,请开始学习Flask或Django。 如果您想成为数据科学家或机器学习工程师,请使用NumPy,Pandas,matplotlib,sci-kit学习,TensorFlow等,然后开始学习它们背后的数学。
C.高级 (C. Advanced)
Now, you don’t necessarily need to learn everything listed in this section. You may do them as per the requirement. I only recently learned some of these. But yeah, learning is never wasted. So, consider having a look at them.
现在,您不必学习本节中列出的所有内容。 您可以按照要求进行操作。 我最近才学到其中一些。 但是,是的,学习永远不会浪费。 因此,考虑看看它们。
1. * args和** kwargs (1. *args and **kwargs)
*args and **kwargs are special keyword which allows a function to take variable-length arguments. They make a function very flexible.
* args和** kwargs是特殊的关键字,它允许函数采用可变长度的参数。 它们使功能非常灵活。
# *args
def add(*num):
sm = 0
for n in num:
sm = sm + n
return smprint(add(3,5))
print(add(4,5,6,7))>>> 8
>>> 22
2.发电机 (2. Generators)
Simply speaking, a generator is a function that returns an object (iterator) which we can iterate over (one value at a time). But, what’s new? Well, they are memory efficient and easy to implement.
简而言之,生成器是一个函数,它返回一个对象(迭代器),我们可以对其进行迭代(一次一个值)。 但是,有什么新消息? 好吧,它们具有高效的内存并且易于实现。
This function can generate all even numbers less than n very efficiently.
此函数可以非常有效地生成小于n的所有偶数。
def all_even(n):
i = 0
while n > i:
yield i
i += 2
3.装饰器 (3. Decorators)
In case you didn’t know functions can be passed as an argument to another function and even a function can return a function. So, decorators take in a function and change(decorate) it.
如果您不知道函数可以作为参数传递给另一个函数,甚至一个函数都可以返回一个函数。 因此,装饰器接受一个函数并对其进行更改(装饰)。
def decorate(func):
def final():
print("I got Decorated")
func()
return final@decorate
def normal():
print("I am Normal")normal()>>> I got Decorated
>>> I am Normal
4.元类 (4. MetaClass)
A metaclass in Python is a class of a class that defines how a class behaves. If you haven’t heard of it before, it may sound confusing as hell to you. Learning them will allow you to understand how exactly classes work. I’d recommend this YouTube playlist by ‘Tech with Tim’ to learn these concepts.
Python中的元类是定义类行为的类的类。 如果您以前从未听说过,它可能会让您感到困惑。 学习它们将使您了解类的工作原理。 我建议使用“与蒂姆合作”的YouTube播放列表来学习这些概念。
5.并发与并行 (5. Concurrency and Parallelism)
Both concurrency and parallelism are involved with performing multiple tasks simultaneously, but what sets them apart is the fact that while concurrency only takes place in one processor. Parallelism is achieved when multiple computations or operations are carried out at the same time or in parallel to speed up the computation process.
并发和并行性都涉及到同时执行多个任务,但是使它们与众不同的是,虽然并发仅在一个处理器中发生。 当同时或并行执行多个计算或操作以加快计算过程时,就可以实现并行。
6.正则表达式 (6. RegEx)
A Regular Expression (RegEx) is a sequence of characters that defines a search pattern. ^a…k$ is regex pattern of any 5-letter word starting with ‘a’ and ending with ‘k’. Python has a module named re to work with RegEx.
正则表达式(RegEx)是定义搜索模式的字符序列。 ^ a…k $是任何以'a'开头并以'k'结尾的5个字母的正则表达式。 Python有一个名为re的模块可与RegEx一起使用。
import repattern = '^a...k$'
test_string = 'aighk'
print(re.match(pattern, test_string))>>> True
7.测试 (7. Testing)
Once you’ve learned all of that, you’d like to get into testing. You should learn about unit testing, integration testing, pipelines, assert keyword in Python, etc.
一旦了解了所有这些,就想开始测试。 您应该了解单元测试,集成测试,管道,Python中的assert关键字等。
8. Cython (8. Cython)
One of the biggest reasons many people choose C, C++ over Python is the speed factor. Python is an interpreted programming language. This makes Python much slower. Cython solves this problem to a huge extent. Cython helps us to achieve C, C++ speed in Python. It is used widely in scientific and numerical computing projects. Several widely used scientific computing libraries for Python — NumPy and SciPy — are also written in Cython.
许多人选择Python而不是C,C ++的最大原因之一是速度因素。 Python是一种解释型编程语言。 这使Python慢得多。 Cython在很大程度上解决了这个问题。 Cython帮助我们在Python中实现C,C ++速度。 它广泛用于科学和数值计算项目。 Cython也编写了几个广泛使用的Python科学计算库-NumPy和SciPy。
So, that concludes the Advanced section. Man… That was a loottt. And, if you came reading all the way till here, appreciate yourself. That’s the dedication you need to learn all these, bruh. You deserve a meme( okay, I can’t control myself from sharing it ).
至此,高级部分结束。 伙计……那是个lo头。 而且,如果您一直阅读到这里,请多多关照。 这就是学习所有这些所需要的奉献,布鲁赫。 你应该得到一个模因(好吧,我无法控制自己分享它)。
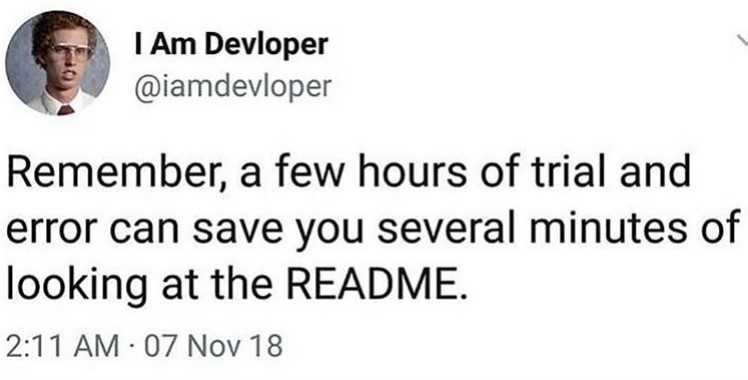
To learn many topics in the Advanced section, I’d recommend this playlist. Also, just google whatever you want to learn.
要学习“高级”部分中的许多主题,建议您使用此播放列表 。 另外,只要使用google即可学习任何内容。
D.专家 (D. Expert)
This section was very hard for me to come up with because, if you’ve completed everything mentioned in the beginner, intermediate, and advanced section, you can pretty much learn whatever you want. And at this point, you’re probably having an idea of what you want to use Python for. You don’t need me to tell you anymore.
这部分对我来说很难,因为如果您完成了初学者,中级和高级部分中提到的所有内容,那么您几乎可以学到任何内容。 至此,您可能已经对要使用Python的内容有所了解。 您不需要我再告诉您了。
But, according to me, now you should try to understand the extremely low-level features of Python. Like, how the interpreter, compiler works. How the language itself is built. Usually, most people don’t care about it. But learning these will always give you an extra edge above your fellow programmers.
但是,据我介绍,现在您应该尝试了解Python的极低级功能。 就像解释器,编译器的工作方式一样。 语言本身是如何构建的。 通常,大多数人不关心它。 但是学习这些知识总是会给您比其他程序员更高的优势。
But, the most important part is how’re you going to use these skills. Do you want to be a web developer, or a data scientist, or you want to make GUIs?How you can solve real-world problems with your code. From here, your path depends on what exactly you want to do.
但是,最重要的部分是您将如何使用这些技能。 您想成为Web开发人员或数据科学家,还是想制作GUI?如何解决代码中的实际问题。 从这里开始,您的路径取决于您实际要做什么。
该开始了! (Time to Get Started!)
I hope, you found some useful information here.
希望您在这里找到了一些有用的信息。
If you have anything to share about your progress — I’m looking forward to seeing the results.
如果您有任何可分享的进展情况,我们非常期待看到结果。
Happy Python!
快乐的Python!
飞猪签到里程python