flutter ui
When used correctly, the Text widget is a powerful widget that easily allows the styling and displaying of text. Here are some questions you are likely to ask yourself when dealing with texts
如果正确使用,“文本”窗口小部件是一个功能强大的窗口小部件,可以轻松地样式化和显示文本。 这是您在处理文字时可能会问自己的一些问题
如何更改文字字体? (How do I change the font of my text?)

The Text widget has a parameter called style. This takes in a TextStyle
widget that allows you to add multiple styles to a widget’s font. As you can see below, it takes in multiple arguments, including fontSize
, fontWeight
, fontFamily
and fontStyle
.
文本小部件具有称为样式的参数。 这包含一个TextStyle
小部件,该部件允许您向小部件的字体添加多种样式。 如下所示,它接受多个参数,包括fontSize
, fontWeight
, fontFamily
和fontStyle
。
The font size takes in a double value for the size. The font weight takes in a class FontWeight that contains a static fields with various levels of thickness, ranging from FontWeight.w100
to FontWeight.w900
— the latter being the thickest. The font family, as shown on line 15, takes in a string of the name of the font you wish to use.
字体大小采用双精度值。 字体粗细包含一个FontWeight类,该类包含具有各种粗细级别(从FontWeight. w100
不等)的静态字段FontWeight. w100
FontWeight. w100
为 FontWeight. w900
FontWeight. w900
后者是最厚的。 如第15行所示,字体系列输入您要使用的字体名称的字符串。
如何在文本小部件中使用自定义字体? (How do I use custom fonts with the text widget?)
To use a custom font, you must first download the font and include the font’s .ttf
file in your project. You then make reference to the asset in your pubspec.yaml
file.
若要使用自定义字体,您必须首先下载该字体并将该字体的.ttf
文件包含在您的项目中。 然后,您pubspec.yaml
在pubspec.yaml
文件中引用资产。
In my project, I have created a fonts folder in the project root and placed in a font called Vogue.ttf
. The name you give it, as shown in line 8, can be anything. This is what you use pass as a string to your method. So, in this instance, in my Text widget, I would set the fontFamily
as ‘Vogue’.
在我的项目中,我在项目根目录中创建了一个fonts文件夹,并放置了一个名为Vogue.ttf
的字体。 如第8行所示,您提供的名称可以是任何名称。 这就是使用pass作为方法的字符串。 因此,在这种情况下,在“文本”小部件中,我将fontFamily
设置为“ Vogue”。
如何在文本中添加填充? (How do I add padding to text?)
To add padding to text, you wrap your text widget in a Padding
widget. Every padding widget, must give a value to the padding value (as shown in line 6).
要向文本中添加填充,您可以将文本小部件包装在“ Padding
小部件中。 每个padding小部件都必须为padding值赋一个值(如第6行所示)。
The value of padding
, however, must be of typeEdgeInsetsGeometry
. This determines the amount of space by which to create distance around the child widget. EdgeInsets comes with a few options to make life easier.
但是padding
的值必须为EdgeInsetsGeometry
类型。 这确定了在子窗口小部件周围创建距离的空间量。 EdgeInsets带有一些选项,可简化生活。
EdgeInset.all(8)
— adds padding to all sides of a widgetEdgeInset.all(8)
-在小部件的所有侧面添加填充EdgeInset.only(left: 8)
— adds padding to only one sideEdgeInset.only(left: 8)
-仅向一侧添加填充EdgeInset.symmetric(vertical: 8, horizontal: 0)
— adds same amount of padding to opposite sides.EdgeInset.symmetric(vertical: 8, horizontal: 0)
—在相对的两侧添加相同量的填充。
如何使文字可点击? (How do I make text clickable?)
On its own, the Text widget does not provide a way to be clickable. However, this can be solved by wrapping it with GestureDetector
or InkWell
.
就其本身而言,“文本”小部件不提供可单击的方式。 但是,可以通过用GestureDetector
或InkWell
包装它来解决。
The GestureDetector allows your text to respond to taps along side a multitude of other touches, including onTapUp
, onTapCancel
, onHorizontalDragDown
, onSecondaryLongPressUp
and onLongPressStart
. As shown on line 6, it takes in a function that allows you to determine what change you wish to occur when your text is clicked.
GestureDetector允许您的文本响应多种其他onTapUp
,包括onTapUp
, onTapCancel
, onHorizontalDragDown
, onSecondaryLongPressUp
和onLongPressStart
。 如第6行所示,它具有一个函数,可让您确定单击文本时希望进行的更改。
如何使我的文字适合空间? (How do I make my text fit inside a space?)
This is super simple. To make sure, your text does not extend infinitely, we use something that Text widget provides called softWrap
. By default, this is set to true in the default DefaultTextStyle
.
这非常简单。 为确保您的文本不会无限扩展,我们使用了文本小部件提供的称为softWrap
。 默认情况下,在默认的DefaultTextStyle
中将其设置为true。

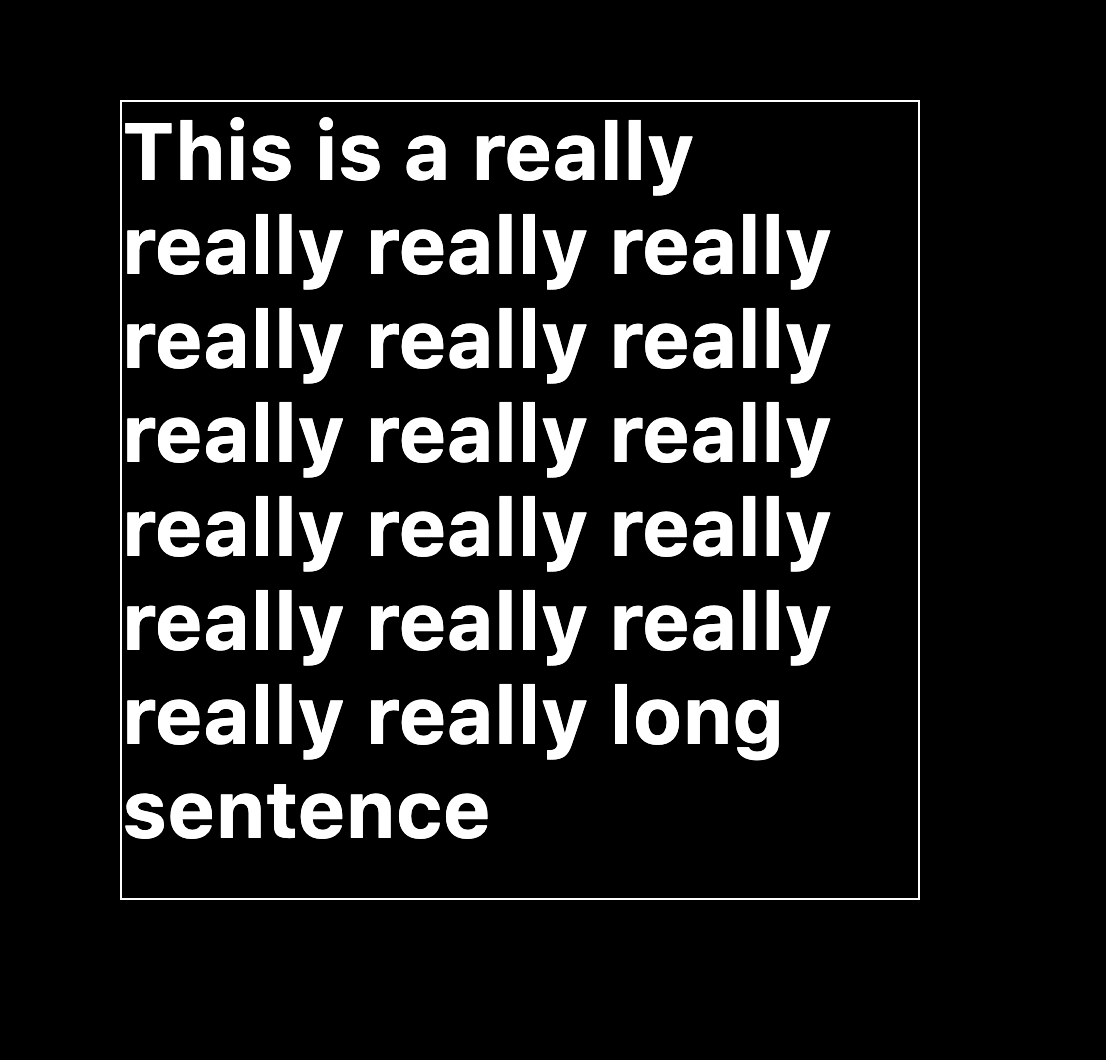
The code below shows how the image above was created.
下面的代码显示了上面的图像是如何创建的。
如何使文本成为链接? (How do I make a text a link?)
At the moment, the easiest way to do so is by using a plugin. I recommend url_launcher. Like before, we use the gesture detector to allow us to assert a response to a touch behaviour. So, onTap
we call the _launchURL() that allows us to launch the url from a compatible browser on our device. However, if you do set forceWebView: true
on an android device, it opens a web view inside the app.
目前,最简单的方法是使用插件。 我推荐url_launcher 。 和以前一样,我们使用手势检测器来声明对触摸行为的响应。 因此,在onTap
我们调用_launchURL(),它使我们能够从设备上的兼容浏览器启动url。 但是,如果您forceWebView: true
在Android设备forceWebView: true
设置forceWebView: true
,则会在应用程序内打开Web视图。
如何允许用户选择和复制文本? (How can I allow users to select and copy text?)

By default, you cannot select and copy text with the Text
widget. Luckily, Flutter has the SelectableText
widget, which allows you to grab and copy text. It’s similar to the Text widget, but allows more interactive behaviour. For example, you can set the cursorWidth
, cursorHeight
, cursorColor
and dragStartBehavior.
默认情况下,您无法使用“ Text
小部件选择和复制文本。 幸运的是,Flutter具有SelectableText
小部件,该部件可让您获取和复制文本。 它类似于“文本”小部件,但允许更多的交互行为。 例如,您可以设置cursorWidth
, cursorHeight
, cursorColor
和dragStartBehavior.
如何在文本中添加变量? (How do I add variables in my text?)
String concatenation is rather straight forward in Dart. All you need to do, as shown in line 14, is to add a $
before adding the variable in curly braces.
在Dart中,字符串连接相当简单。 如第14行所示,您所需要做的就是在花括号中添加变量之前添加$
。
如何为文本的不同部分设置样式? (How do I style different parts of my Text?)

From the image above, we have defined a new style specifically for the player’s name. As shown in the snippet below, Text.rich
allows you to break your inline text into singular components that you can then work on individually. The style in line 23, defines the base text style. However, we override this for the player’s name.
从上面的图片中,我们为玩家的名称定义了一种新样式。 如下面的代码片段所示, Text.rich
允许您将内联文本分成单个组件,然后可以单独进行处理。 第23行中的样式定义了基础文本样式。 但是,我们会将其替换为玩家的名字。
如何使文字垂直? (How do I make my text vertical?)

To achieve the above, we first break down our string into an array of strings and create a text widget for each one. We then stack them on top of each other, like Lego blocks using Wrap
. The critical point in this is line 14, where we set the direction of our our widget — this is vertical in our case.
为了达到上述目的,我们首先将字符串分解为字符串数组,然后为每个字符串创建一个文本小部件。 然后,我们将它们堆叠在一起,例如使用Wrap
来构建乐高积木。 关键点是第14行,我们在其中设置小部件的方向-在我们的例子中这是垂直的。
The end.
结束。
I hope this has been somewhat helpful!
我希望这会有所帮助!
翻译自: https://medium.com/swlh/flutter-ui-series-stuff-you-should-know-about-working-with-text-e2f0fb8201ae
flutter ui