c语言实现堆栈
A stack is a set of items stored using a last-in first-out or LIFO policy. This means that the last thing that is inserted to the stack is the first thing that is removed from the stack. Often, we might think of a stack like a stack of dinner plates. When we place a plate onto the stack, we will place it on the top, and in turn when we want to take a plate off the stack, we also remove it from the top. This is the same sort of structure we wish to implement with a programming stack.
堆栈是使用后进 先出或LIFO策略存储的一组项目。 这意味着插入到堆栈中的最后一个内容是从堆栈中删除的第一个内容。 通常,我们可能会像一堆餐盘那样想到一堆。 当我们将盘子放到堆垛上时,我们会将其放在顶部,反过来,当我们想从堆垛上取下盘子时,我们也会从顶部卸下它。 这与我们希望通过编程堆栈实现的结构相同。
Let’s start by having a look at a visual example of a stack. Suppose I insert three values onto my stack, which are 5, 6, and 7. If they are inserted in the order specified, our stack will look like below.
让我们先来看一个可视的堆栈示例。 假设我在堆栈中插入了三个值,分别是5、6和7。如果按指定的顺序插入它们,则我们的堆栈将如下所示。
The last thing that was inserted, which is 7, is the top item on the stack, and the first thing inserted, which is 5, is the bottom item on the stack. When we work with stacks, we typically implement three methods to manipulate the data on them. These methods are push, pop, and peek. Push will insert a new value on top of the stack, pop will remove the top value from the stack, and peek will return the value of the top of the stack.
插入的最后一个东西(即7)是堆栈中的第一项,插入的第一件事(即5)是堆栈中的底部。 当使用堆栈时,通常会实现三种方法来处理堆栈上的数据。 这些方法是推,弹出和窥视。 Push将在堆栈顶部插入一个新值,pop将从堆栈中删除顶部值,而peek将返回堆栈顶部的值。
Using our example, suppose we wanted to now push the value 8 onto the stack. When we do this, 8 becomes the new top of the stack, and the item directly below it was the previous top, 7.
使用我们的示例,假设我们现在想将值8压入堆栈。 当我们执行此操作时,8将成为堆栈的新顶部,而其正下方的项目是前一个顶部7。

If we wanted to then pop from this stack, it would remove the current top, and update the top to be the thing that comes next in the stack. In this case, 8 would be removed and returned to the user, and 7 would become the top of the stack.
如果我们想从堆栈中弹出,它将删除当前顶部,并将顶部更新为堆栈中的下一个对象。 在这种情况下,将删除8个并返回给用户,而7个将成为堆栈的顶部。

Finally, if we wanted to peek on this stack, it would simply tell us the top value, without modifying the stack at all. In this case, it would return 7, and the stack would remain the same.
最后,如果我们想偷看这个堆栈,它只会告诉我们最高价值,而根本不需要修改堆栈。 在这种情况下,它将返回7,并且堆栈将保持不变。
When we implement a stack in C, we typically use two structs. The first struct is called a Node, and it keeps track of a single element, as well as a pointer to the next element in the stack. The second struct is called the Stack, which stores the top element as a node. Since the node has a pointer to the next element in the stack, if we ever need to get the element below the top, we can use the pointer to find it.
当我们用C实现堆栈时,通常使用两个结构。 第一个结构称为节点,它跟踪单个元素以及指向堆栈中下一个元素的指针。 第二个结构称为堆栈,该堆栈将顶部元素存储为节点。 由于节点具有指向堆栈中下一个元素的指针,因此,如果我们需要使该元素位于顶部之下,则可以使用该指针来查找它。
So, in our stack example, if 7 is the top of the stack, it will have a pointer to 6, which is the next element in the stack. The node for 6 will have a pointer to 5, since 5 comes after 6. Finally, 5 will have a pointer to NULL, since there are no values that follow it. The following code shows how these two structs can be implemented in C.
因此,在我们的堆栈示例中,如果7是堆栈的顶部,它将有一个指向6的指针,这是堆栈中的下一个元素。 6的节点将有一个指向5的指针,因为5紧随6之后。最后,5的指针将指向NULL,因为没有紧随其后的值。 以下代码显示了如何在C中实现这两个结构。
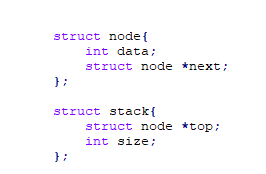
In this implementation, our node keeps track of its value using the data attribute. It additionally has a pointer attribute called next, that points to the node that directly follows it. Our stack tracks the node that is at the top through the top attribute, and also keeps track of its size in the size attribute.
在此实现中,我们的节点使用data属性跟踪其值。 此外,它还有一个名为next的指针属性,该属性指向直接跟随它的节点。 我们的堆栈通过top属性跟踪位于顶部的节点,并在size属性中跟踪其大小。
When we first create a stack, we will want to initialize its attributes appropriately. To do so, we will use an initialization function, which will take in our stack struct, and set the top to NULL, and the size to 0. Doing so will ensure that when we insert our first value, it points to NULL as its next entry, rather than garbage data.
首次创建堆栈时,我们将需要适当地初始化其属性。 为此,我们将使用一个初始化函数,该函数将接收我们的堆栈结构,并将top设置为NULL,并将大小设置为0。这样做将确保当我们插入第一个值时,它指向NULL作为其值。下一个条目,而不是垃圾数据。

Now that our stack is complete, we will begin to look at how we can implement our push, pop, and peek operations. We will also create a method to display the data currently on the stack, to be able to verify that our operations are working correctly.
现在我们的堆栈已完成,我们将开始研究如何实现推送,弹出和查看操作。 我们还将创建一种方法来显示堆栈上当前的数据,以便能够验证我们的操作是否正常运行。
For the push operation, we will take in any integer value the user wishes to push onto the stack. We will start by creating a new node to store the data provided by the user. Once we have added the data to the node, we will set the node’s next value to the current top of the stack and set the current top of the stack equal to the node we are pushing. Once this is completed, we increase the size of the stack by 1 so that it matches the stack after the insert is completed.
对于推入操作,我们将接受用户希望推入堆栈的任何整数值。 我们将首先创建一个新节点来存储用户提供的数据。 将数据添加到节点后,将节点的下一个值设置为堆栈的当前顶部,并将堆栈的当前顶部设置为与要推送的节点相等。 完成此操作后,我们将堆栈的大小增加1,以使其在插入完成后与堆栈匹配。

For the pop operation, our first step will be to make sure that there is at least one item on the stack that can be removed. To do this, we can look at the size of the stack to check if it is 0. If the size of the stack is not 0, we proceed to pop an item off the stack. Otherwise, we will print an error message, and end the program. When popping from the stack, we start by storing the value of the top element on the stack. We then update the top of the stack to be the next available element, which is the one that the top’s next attribute points to. Finally, we decrease the size of the stack by 1, and return the value that was at the top of the stack to the user.
对于弹出操作,我们的第一步将是确保堆栈上至少有一个可以删除的项目。 为此,我们可以查看堆栈的大小以检查它是否为0。如果堆栈的大小不为0,则继续从堆栈中弹出一个项目。 否则,我们将打印一条错误消息,并结束程序。 从堆栈中弹出时,我们首先将top元素的值存储在堆栈中。 然后,我们将堆栈的顶部更新为下一个可用元素,这是顶部的next属性所指向的元素。 最后,我们将堆栈的大小减小1,然后将堆栈顶部的值返回给用户。

The peek operation is basically a simplified version of the pop operation. We do the same steps, aside from updating the top and size of the stack.
窥视操作基本上是弹出操作的简化版本。 除了更新堆栈的顶部和大小以外,我们执行相同的步骤。

With these operations implemented, we now have a fully functional stack. To help with testing our stack, I will implement one additional method called print, which will print the elements inside of the stack. For this function, we will create a temporary node, and set it equal to the top of the stack. From here, we will print the temporary node, then move it to the next link, until we reach the end of the stack, which is a NULL node.
实施这些操作后,我们现在有了一个功能齐全的堆栈。 为了帮助测试我们的堆栈,我将实现一种称为print的附加方法,该方法将打印堆栈内部的元素。 对于此功能,我们将创建一个临时节点,并将其设置为等于堆栈的顶部。 从这里,我们将打印临时节点,然后将其移动到下一个链接,直到到达堆栈的末尾,这是一个NULL节点。

To test this implementation, we can create a main function, and run through each of our methods.
为了测试该实现,我们可以创建一个主要功能,并运行我们的每种方法。

There are a few things to note in how we setup our stack to be used. First, when we declare our stack struct, we malloc for the size of the stack, since it needs to be initialized in memory before we can use it. After this, we run the init function first to setup our stack with the proper initial values. Once this is done, we can run push, pop, peek, and print operations in any sequence to see what results we get. Running this sequence, I get the following output.
我们如何设置要使用的堆栈时要注意几件事。 首先,当我们声明堆栈结构时,我们为堆栈的大小分配了malloc,因为在使用它之前需要先在内存中对其进行初始化。 此后,我们首先运行init函数以使用正确的初始值设置堆栈。 完成此操作后,我们可以按任意顺序运行推入,弹出,查看和打印操作,以查看得到的结果。 运行此序列,我得到以下输出。
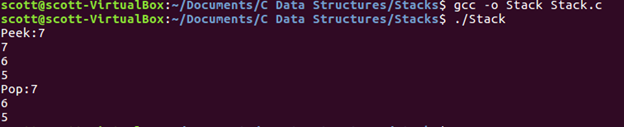
The full C code for this stack implementation is available at: https://github.com/scprogramming/The-Complete-Guide-to-C-Data-Structures-and-Algorithms/tree/master/Stacks
有关此堆栈实现的完整C代码,请访问: https : //github.com/scprogramming/The-Complete-Guide-to-C-Data-Structures-and-Algorithms/tree/master/Stacks
翻译自: https://medium.com/swlh/how-to-implement-a-lstack-in-c-4c8b29fdad11
c语言实现堆栈