python二次方程求解
Oh, sure — you can do a quick Google search and find a bunch of quadratic equation solvers online. But wouldn’t it be more fun to just build one yourself? Yup. I’m going to show you how to do that.
哦,可以-您可以快速进行Google搜索,并在线找到一堆二次方程求解器。 但是,自己动手建造不是更有趣吗? 对。 我将向您展示如何做到这一点。
What is the Quadratic Equation?
什么是二次方程式?
Suppose you have a some quadratic function. Maybe it looks like this.
假设您有一些二次函数。 也许看起来像这样。
Now, you want to find the values where f(x) = 0, so you get the following.
现在,您要查找f(x)= 0的值,因此得到以下结果。
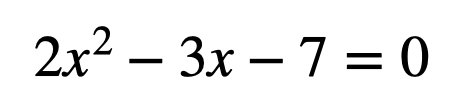
What do you do next? Well, you could try to factor this to isolate x, but sometimes that just doesn’t work out so well. Another option is to just plot the function and see where it crosses the x-axis.
下一步你要怎么做? 好吧,您可以尝试将其分解为x,但有时效果并不理想。 另一种选择是仅绘制函数并查看其与x轴的交点。
Solution by Graphing
图解法
Here’s what that would look like (code here in python if you want it).
这就是它的样子( 如果需要,可以在python中找到代码 )。
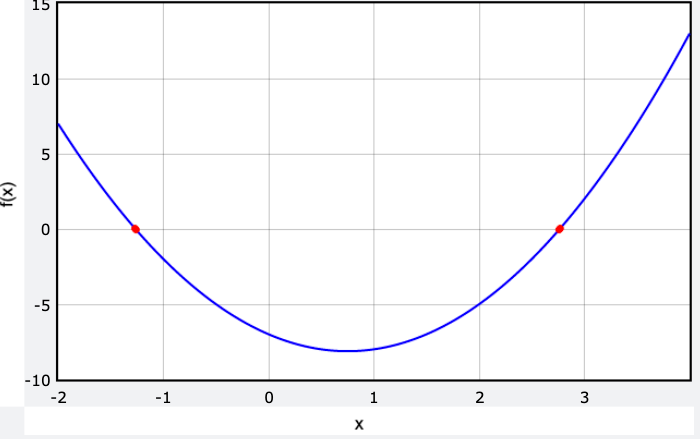
I put two red points where the function crosses the x-axis. Since I’m just getting the values by inspecting this graph — it’s not perfect. But this gives two x-values at about -1.27 and 2.76. That’s not too bad.
我在函数与x轴交叉处放置了两个红色的点。 由于我只是通过检查此图来获取值的,所以它不是完美的。 但这给出了两个x值,分别约为-1.27和2.76。 还算不错
The Quadratic Equation
二次方程
Actually, the quadratic equation isn’t something new. It’s just a generic solution to factoring a second order polynomial. Suppose your basic function looks like this.
实际上,二次方程式并不是什么新鲜事物。 这只是分解二阶多项式的通用解决方案。 假设您的基本功能如下所示。
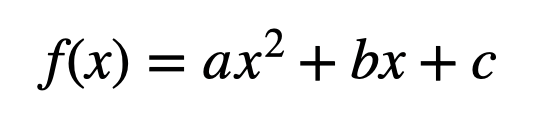
If you set this function equal to zero, you can factor to get the following two solutions when f(x) = 0:
如果将此函数设置为零,则在f(x)= 0时可以分解为以下两个解:
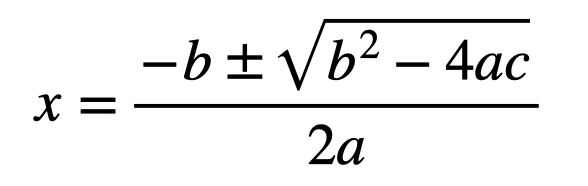
Yup. That’s the quadratic equation. You can put in your values for a, b, and c and you get two solutions. For the example above: a = 2, b = -3, c = -7.
对。 那是二次方程。 您可以输入a,b和c的值,然后得到两个解。 对于上面的示例:a = 2,b = -3,c = -7。
But let’s build a python program so that we can enter whatever values we want for pretty much any second order polynomial.
但是让我们构建一个python程序,以便我们可以输入几乎任何二阶多项式所需的任何值。
Step 1: Read Values of a, b, c.
步骤1:读取a,b,c的值。
I’m going to be using python 2 on trinket.io (because anyone can use that for free). Of course the simplest thing would be to just define a, b, and c as variables in your code. But I don’t want to do that. Instead, I am going to have it as a “user input”. Yes, you can actually do this in Glowscript also — but it just looks nicer in python 2 (works in python 3 just fine).
我将在trinket.io上使用python 2 (因为任何人都可以免费使用它)。 当然,最简单的事情就是在代码中将a,b和c定义为变量。 但是我不想那样做。 相反,我将其作为“用户输入”。 是的,您实际上也可以在Glowscript中执行此操作-但在python 2中看起来更好(在python 3中正常工作)。
Here is what that looks like (full code here).
看起来像这样( 完整代码在这里 )。
from numpy import sqrt
print("You have an equation ax^2 +bx+c = 0")
a=float(input("a ="))
b=float(input("b ="))
c=float(input("c ="))
Now for some comments.
现在发表一些评论。
- The quadratic equation requires a square root — this is not automatically in normal python (but it is in Glowscript). This means that I need to import this sqrt function from the numpy module. That’s what is going on in that first line. 二次方程需要平方根-在正常的python中这不是自动的(但是在Glowscript中)。 这意味着我需要从numpy模块导入此sqrt函数。 那就是第一行的内容。
- The second line just prints out some stuff so that it’s clear to the user what values should be entered for “a”, “b”, and “c”. 第二行只是打印出一些东西,以便用户清楚应为“ a”,“ b”和“ c”输入什么值。
The last three lines are the actual text input. The
a=float()
means it forces the input into a non-integer number and assigns this to the variable “a”. Theinput(“a =”)
prints out the “a =” part and then collects from the user.最后三行是实际的文本输入。
a=float()
表示将输入强制为非整数,并将其分配给变量“ a”。input(“a =”)
打印出“ a =”部分,然后从用户处收集。- Yes, if the user wants to be funny and input a non-number this doesn’t work (we can fix that below). 是的,如果用户想取笑并输入一个非数字,则此操作无效(我们可以在下面进行修复)。
Now for the rest of the program with all the calculations.
现在,对程序的其余部分进行所有计算。
if a==0:
print("What the what?? This is not a quadratic equation.")
b=0
c=0
a=1x1=(-b+sqrt(b**2-4*a*c))/(2*a)
x2=(-b-sqrt(b**2-4*a*c))/(2*a)print("x1 = "+str(x1))
print("x2 = "+str(x2))
Let me point out some things.
让我指出一些事情。
- That first line checks to make sure the value of a is not equal to zero. If a WAS zero, you would cause a problem by dividing by zero. So, I just give “alternative” values. 第一行检查以确保a的值不等于零。 如果WAS为零,则除以零会引起问题。 因此,我只给出“替代”值。
You have to calculate the roots twice. One for a “plus” and one for a “minus”. Remember that to square the value of b, you would use
b**2
.您必须计算两次根。 一个代表“加号”,另一个代表“减号”。 请记住,要对b的值求平方,可以使用
b**2
。
This works. Using the same values for a, b, and c as in the previous example I get the following output.
这可行。 使用与上一个示例相同的a,b和c值,将得到以下输出。
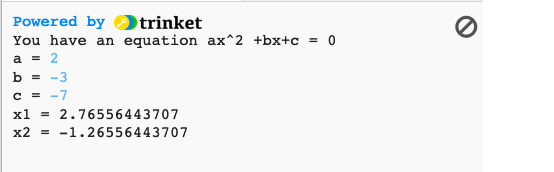
Let’s Make It Even Better
让我们变得更好
Just for fun, let’s fix this up. There are a couple of big problems with the simple code.
只是为了好玩,让我们对其进行修复。 简单的代码有两个大问题。
- What can you do about fools entering non-numbers? 傻瓜输入非数字怎么办?
- What if there are no real value roots to the polynomial? 如果多项式没有实数值根怎么办?
Here is a bit of code to handle user input from bad people. OK, they aren’t bad people — just people with bad actions.
这里有一些代码可以处理不良用户的输入。 好吧,他们不是坏人-只是有不良行为的人。
while 1:
try:
a=int(raw_input("a = "))
break
except ValueError:
print("Enter a number")
continue
Let’s go through this stuff.
让我们看一下这些东西。
The
while 1
just makes an infinite loop (but there is an escape hatch).while 1
只是无限循环(但是有一个逃生舱口)。The
try:
just executes the same input line as before, if it works with no error, you get to use the loop escape hatch.try:
仅执行与以前相同的输入行,如果它没有错误,则可以使用循环转义填充。The
except ValueError:
tells the code what to do if the user doesn’t want to follow social norms.except ValueError:
告诉代码如果用户不想遵循社会规范该怎么办。Of course, I’m not a real programmer — so I found some help on stackoverflow.com.
当然,我不是一个真正的程序员-因此我在stackoverflow.com上找到了一些帮助。
Now, what about the non-real roots stuff? Here’s what I have.
现在,那些非真实的根源呢? 这就是我所拥有的。
if a==0:
print("What the what?? This is not a quadratic equation.")
if b==0:
print("There is no x")
else:
x1=-b/c
print("x1 = "+str(x1))
else:
if b**2-4*a*c<0:
print("No Real Roots")
else:
x1=(-b+sqrt(b**2-4*a*c))/(2*a)
x2=(-b-sqrt(b**2-4*a*c))/(2*a)
print("x1 = "+str(x1))
print("x2 = "+str(x2))
- In this version, if a is equal to zero I just go ahead and solve the non-quadratic equation. 在此版本中,如果a等于零,我将继续求解非二次方程。
- If a IS equal to zero, the first thing I do is to check the stuff in the square root. If this has a value that is less than zero, then it’s negative and there are no real roots. 如果IS等于零,那么我要做的第一件事就是检查平方根中的内容。 如果该值小于零,则它为负,并且没有实际根。
- The rest of the calculation is the same. 其余计算相同。
Here is the full code for the improved calculator. Wasn’t that fun to build?
这是改进的计算器的完整代码 。 建立起来不是很有趣吗?
翻译自: https://medium.com/swlh/build-your-own-quadratic-equation-solver-in-python-4f70e48d2ca4
python二次方程求解