程式设计 (Programming)
This is the first part of “Understanding Python” wherein we would be learning the foremost 5 fundamentals in python. They are,
这是“ Python理解”的第一部分,我们将学习python最重要的5个基础知识。 他们是,
- Variables and their assignment 变量及其赋值
- Print function 打印功能
- User input() function 用户输入()函数
- How to find information using help() 如何使用help()查找信息
- Built-in functions 内建功能
Let us jump right into understanding each of them so that at the end of your reading, you will have basic know-how on working with python.
让我们直接理解它们,以便在阅读结束时,您将拥有使用python的基本知识。
Note: All the coding examples provided in this blog are done in the Jupyter notebook which is a web-based platform to work on python. It is highly recommended for learners who want to start with a few lines of code.
注意:此博客中提供的所有编码示例均在Jupyter笔记本中完成,该笔记本是基于Web的平台,可在python上工作。 强烈建议想从几行代码开始的学习者。
1.Variable
1.变量
Python is an object-oriented language and every object is stored in the memory whenever it is instantiated. The variable in python is a label given to the memory location where the object is stored. In simple words, whenever you assign a variable a value, the value gets stored in the memory and the name of the variable is just a view to the object(value). Let us understand this with an example.
Python是一种面向对象的语言,每个对象在实例化时都存储在内存中。 变量 python中的标签是给对象存储位置的标签。 简而言之,只要为变量分配一个值,该值就会存储在内存中,并且变量的名称只是对象(值)的视图。 让我们通过一个例子来理解这一点。
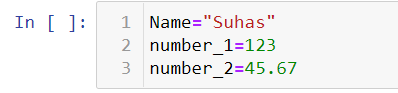
Figure[1] shows the variable assignment with different types of objects. Here, Line 1 represents the assignment of a string(“Suhas”) to the variable “Name”. In other words, a string object “Suhas” is stored in memory and the variable “Name” is the label given to it. Similarly, line 2 and line 3 represent int and float type objects getting assigned to the variables number_1 and number_2 respectively.
图[1]显示了具有不同类型对象的变量分配。 在这里,第1行表示将字符串 (“ Suhas”)分配给变量“ Name”。 换句话说,字符串对象“ Suhas”存储在内存中,变量“ Name”是为其赋予的标签。 类似地,第2行和第3行表示分别分配给变量number_1和number_2的 int和float类型对象。
What are string, int, and float?
什么是字符串,整数和浮点数?
They are the data types available in python. For now, let us say string is a sequence of characters, an int is an integer and float is a fraction number.
它们是python中可用的数据类型。 现在,让我们说字符串是一个字符序列,一个整数是一个整数,而浮点数是一个小数。
Multiple variables assignments:
多变量分配:
Till now, we have seen how a single variable assignment works. Let us see how we can assign multiple variables.
到目前为止,我们已经看到了单个变量分配的工作方式。 让我们看看如何分配多个变量。
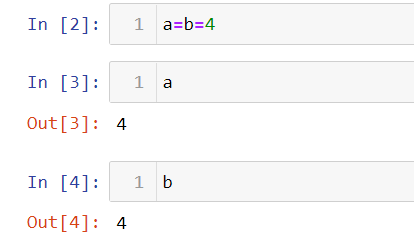
In the above figure, we have assigned value 4 to variables a and b. Hence, their output is 4 accordingly.
在上图中,我们已将值4分配给变量a和b 。 因此,它们的输出相应为4。

In Figure[3], we have assigned different types of values to variables x,y, and z. Integer value 12 is assigned to x, the string “Suhas” is assigned to y and float value 56.78 is assigned to z respectively.
在图[3]中,我们为变量x,y和z分配了不同类型的值。 整数值12分配给x, 字符串 “ Suhas”分配给y, 浮点值56.78分配给z。
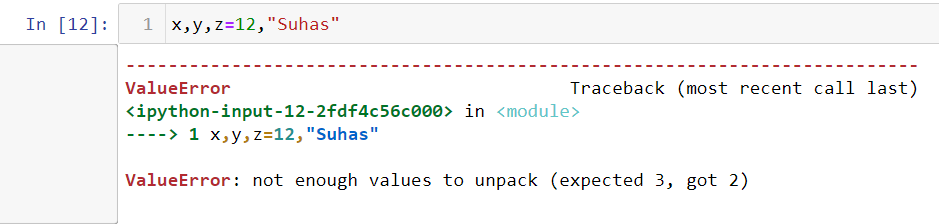
One thing to be mindful of while assigning value to multiple variables in a single line is that the number of values assigned must be equal to the number of variables. Figure[4] shows a ValueError when we are assigning only 2 values to 3 variables x,y, and z.
在一行中为多个变量赋值时要注意的一件事是,赋值的数量必须等于变量的数量。 图[4]显示了当我们仅向3个变量x,y和z分配2个值时的ValueError 。
2. Print function
2.打印功能
The print function (denoted by print()) prints the data passed into it. There are different ways to use print() as it is very flexible and subtly helps your code.
打印功能(由print()表示) 打印传递给它的数据。 有多种使用print()的方法,因为它非常灵活并且可以巧妙地帮助您的代码。
For most of the examples, the usage is direct and self-explanatory. You can refer to my comment on each line of the code for better understanding.
对于大多数示例,用法是直接且不言自明的。 您可以参考我对代码的每一行的评论,以更好地理解。
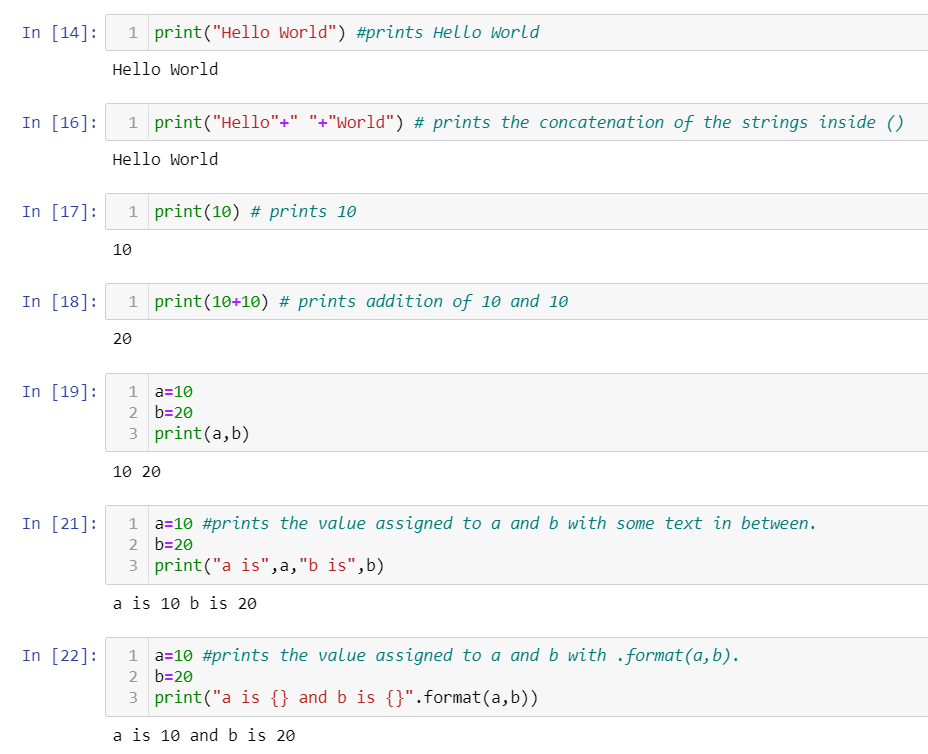

Figure[6] prints the value assigned to a and b with .format(a,b).Here,0 is the first variable a, and 1 is the second variable b.
图[6]用.format(a,b)打印分配给a和b的值。这里,0是第一个变量a,1是第二个变量b。
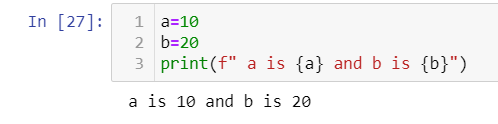
In Figure[7], the values of a and b are passed inside {} and then printed.
在图[7]中,a和b的值在{}内传递,然后打印。
带有“ end”和“ sep”参数的print() (print( ) with “end” and “sep” parameters)
By default, python’s print() function ends with a newline. A parameter called ‘end’ decides how the print should end. The default value of this parameter is ‘\n,’ i.e., the new line character. You can end a print statement with any character or string using this parameter. Refer to Figure[8].
默认情况下,python的print()函数以换行符结尾。 名为“ end”的参数决定打印的结束方式。 此参数的默认值为'\ n',即换行符。 您可以使用此参数以任何字符或字符串结束打印语句。 参见图[8]。
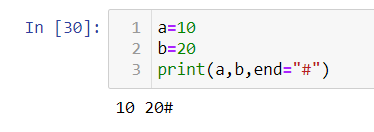
To separate the print of objects, the “sep” parameter is used. Refer Figure[9].
要分隔对象的打印,使用“ sep ”参数。 参见图[9]。
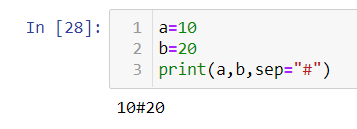
3. User input()
3.用户输入()
Suppose you want your code to take inputs from the user and perform some function. Python allows you to have a dialog box pop up for the user to enter the value of their choosing.
假设您希望代码从用户那里获取输入并执行某些功能。 Python允许您弹出一个对话框供用户输入其选择的值。

Note: By default, the input( ) function returns a string. Appropriate, type conversion to numerical datatypes(int, float) has to be done prior to performing a numerical calculation. If not, it throws an error. Refer Figure[11] and Figure[12].
注意:默认情况下,input()函数返回一个string 。 在执行数值计算之前,必须进行适当的类型转换为数值数据类型(int,float)。 如果不是,则抛出错误。 参见图[11]和图[12]。
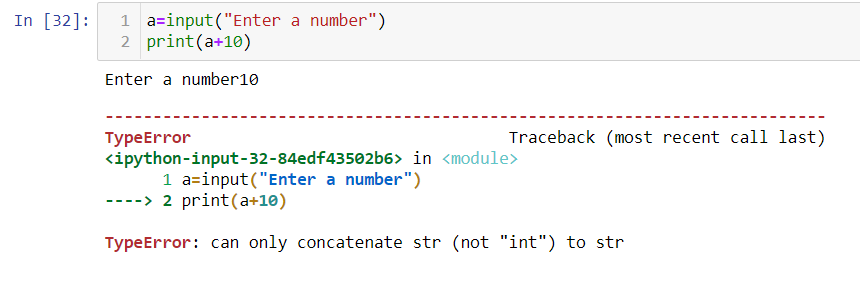
Perform type conversion of a to int and then print(a+10)
执行a到int的类型转换,然后打印(a + 10)
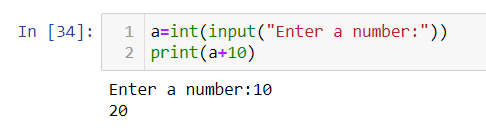
4. Finding information using help( )
4.使用help()查找信息
Learning Python in Jupyter is very intuitive and easy. It allows you to explore and find information on your own. One such way is by using the built-in help() function. Let us see what information we can extract on print using help and it is self-explanatory. Let us see what are the parameters of the print() function.
在Jupyter中学习Python非常直观且容易。 它使您可以自己探索和查找信息。 一种方法是使用内置的help()函数。 让我们看看我们可以使用帮助从印刷中提取哪些信息,这些信息是不言自明的。 让我们看看print()函数的参数是什么。
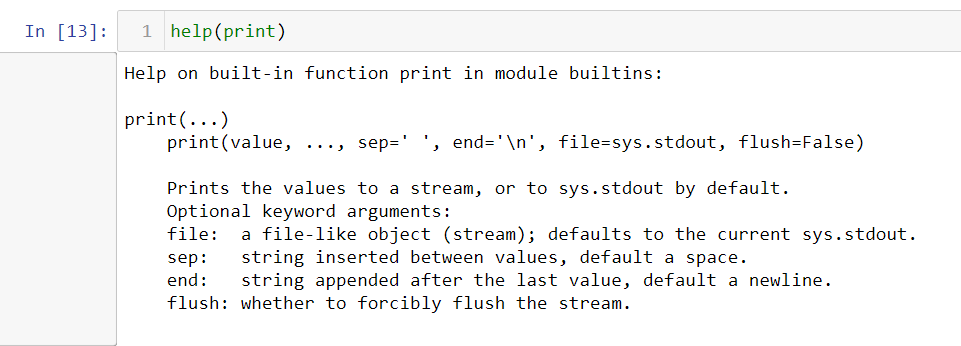
5. Built-in Functions
5.内置功能
Functions are a piece of reusable code that allows us to write a program to perform a certain activity. This is called user-defined functions. For now, let us concentrate on the built-in Functions of python. It is a one-line piece of code available for the users at a higher level to adapt in their computations. We will now explore some built-in functions.
函数是一段可重用的代码,它使我们可以编写程序来执行特定的活动。 这称为用户定义函数 。 现在,让我们集中讨论python的内置函数。 它是单行代码,可供更高级别的用户使用以适应他们的计算。 现在,我们将探索一些内置功能。
type( ) function:
type()函数:
If you would want to know the data type of a variable, all you have to do is write type(variable name). The type( ) returns the data type of the variable passed. Refer Figure[14].
如果您想知道变量的数据类型,您所要做的就是写类型(变量名)。 type()返回所传递的变量的数据类型。 参见图[14] 。

id( ) function:
id()函数:
Whenever an object is created in memory, a unique identity will be given to it. id( ) function returns the identity of the object. In other words, we can use the id ( ) function when we need to get the memory location of an object.
每当在内存中创建对象时,都会为其赋予唯一标识。 id()函数返回对象的身份。 换句话说,当我们需要获取对象的内存位置时,可以使用id()函数。
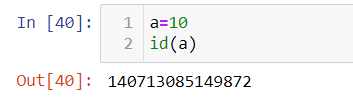
Object value 10 assigned to variable a is at the memory location 14071308514982
分配给变量a的对象值10在存储器位置14071308514982
Type conversion functions:
类型转换功能:
str(), int(), float(), bool( ) are the most commonly used built-in conversion functions. Let us see what each one of them does.
str(),int(),float(),bool()是最常用的内置转换函数。 让我们看看他们每个人都在做什么。
str( )
str()
The string function converts the value to a string value. Refer the Figure[16], where a float value 25.456 is converted to a string ‘25.456’.
字符串函数将值转换为字符串值。 参见图[16],其中浮点值25.456被转换为字符串'25 .456'。
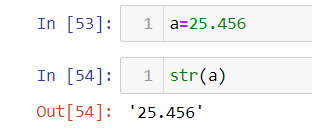
Similarly bool(),float(),int() converts the value inside the paranthesis to a bool, float and integer values respectively. Let us see an example for each in the Figure[17].
类似地,bool(),float(),int()会将括号内的值分别转换为bool,float和整数值。 让我们在图[17]中看到每个示例。

round( )
圆()
The round() function rounds off a floating number to a certain digit after the decimal point suggested by the user. Figure[18] shows a number with 3 places after the decimal point. It is rounded off to 2 places after the decimal point using the round function. Accordingly, 25.456 becomes 25.46
round()函数将浮点数四舍五入为用户建议的小数点后的某个数字。 图[18]显示了一个小数点后3位的数字。 使用舍入函数将其舍入到小数点后的2位。 因此,25.456变为25.46
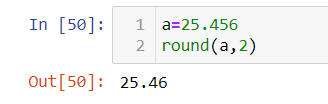
len( ) function
len()函数
The len() function returns the number of elements in a collection or a sequence of characters. For now, let us consider an example to find out the number of letters in a word. The “Python” word has 6 letters in it.
len()函数返回集合或字符序列中的元素数。 现在,让我们考虑一个示例来找出单词中字母的数量。 “ Python”一词中有6个字母。
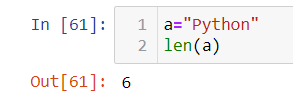
If you ever wonder how to see all the available built-in function in python in Jupyter platform, just write dir(__builtin__) and this will do the trick.
如果您想知道如何在Jupyter平台中查看python中所有可用的内置函数,只需编写dir(__ builtin__) ,即可解决问题。
You would be wondering, are these concepts sufficient enough to have a grasp on python? The answer is “Not really” to be honest, but they introduce you to the world of python. Since this being the first part of our learning journey, I have tried to keep things simple. I will continue to write more as we move on.
您会想知道,这些概念是否足以掌握python? 说实话,答案是“不是真的”,但是它们向您介绍了python的世界。 由于这是我们学习旅程的第一部分,因此我尝试使事情变得简单。 随着我们的前进,我将继续写更多。
If you are new to programming and want something to make you feel comfortable to start learning with python, please read my other blog “Python is Fun!!!”.
如果您是编程的新手,并且想要某种让您开始使用python学习感到自在的方法,请阅读我的其他博客“ Python is Fun !!!”。
翻译自: https://medium.com/towards-artificial-intelligence/understanding-python-part-1-651575f5d3b4