python字节流串联
Python中的串联和重复: (Concatenation and Repetition in Python:)
In python, concatenations and repetitions are supported by sequence data types both mutable(list) and immutable(tuple, strings). Sequence types like range objects do not support concatenation and repetition. Furthermore, Python containers that are non-sequence data types (such as sets or dictionaries) do not support concatenation and repetition operators. In this article, we will take a detailed look at concatenations and repetitions that are supported by sequence data types such as list, tuple, and strings.
在python中,序列数据类型支持mucate(list)和immutable(tuple,strings)串联和重复。 诸如范围对象之类的序列类型不支持串联和重复。 此外,非序列数据类型(例如集合或字典)的Python容器不支持串联和重复运算符。 在本文中,我们将详细研究序列数据类型(如列表,元组和字符串)所支持的串联和重复。
Topics covered in this story:
这个故事涉及的主题:
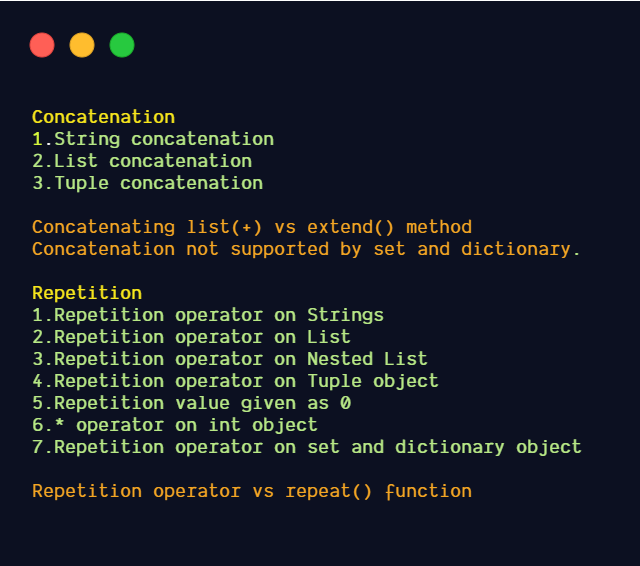
级联: (Concatenation:)
Concatenation is done by +
operator. Concatenation is supported by sequence data types(string, list, tuple). Concatenation is done between the same data types only.
串联由+
运算符完成。 序列数据类型(字符串,列表,元组)支持串联。 只能在相同的数据类型之间进行串联。
Syntax:
句法:
a+b
a+b
字符串串联: (String Concatenation:)
The string is an immutable sequence data type. Concatenating immutable sequence data types always results in a new object.
字符串是不可变序列数据类型。 串联不可变序列数据类型总是会产生一个新对象。
Example 1: Strings are concatenated as they are, and no space is added between the strings.
示例1:字符串按原样连接,并且在字符串之间不添加空格。
s1="Welcome"s2="to"s3="python"s4=s1+s2+s3
print (s4)#Output:Welcometopython
Example 2: If we want space between two strings while concatenating, we either have to include a space in the string itself or concatenate the space.
示例2:如果在连接时希望两个字符串之间有空格,我们要么必须在字符串本身中包含一个空格,要么将其串联。
Method 1:
方法1:
s1="Welcome "s2="to "s3="python"s4=s1+s2+s3
print (s4)#Output:Welcome to python
Method 2:
方法2:
s1="Welcome"s2="to"s3="python"s4=s1+" " + s2+" "+ s3
print (s4)#Output:Welcome to python
Example 3: Concatenation between different data types.
示例3:不同数据类型之间的串联。
This will raise TypeError
.
这将引发TypeError
。
s1="Welcome"
s2="to"
s3="python"
s4=[3.8]
s5=s1+s2+s3+s4
#Output:TypeError: can only concatenate str (not "list") to str
Example 4:Using +
operator results in and addition to int objects and concatenation in strings.
示例4:使用 +
运算符可在int对象和字符串连接中添加结果。
s=1+2
print (s)#Output:3print (type(s))#Output:<class 'int'>s1='1'+'2'print (s1)#Output:12print (type(s1))#Output:<class 'str'>
Example 5: If the strings are placed next to each other without +
operator this will result in a concatenation.
示例5:如果不使用 +
运算符将 字符串彼此相邻放置, 则将导致串联。
s='Welcome''to''Python'print (s)#Output:WelcometoPythons1='Welcome'+'to'+'Python'print (s)#Output:WelcometoPython
Example 6: Apply methods to strings while concatenating.
示例6:在连接时将方法应用于字符串。
s1="Welcome"s2="TO"s3="Python"s4=s1+s2.lower()+s3
print (s4)#Output:WelcometoPython
连接元组对象: (Concatenating tuple objects:)
The tuple is an immutable sequence data type. Concatenating immutable sequence data types always results in a new object.
元组是不可变序列数据类型。 串联不可变序列数据类型总是会产生一个新对象。
Example 1: Concatenating two tuple objects
示例1:连接两个元组对象
t1=(1,2)
t2=(3,4)
print (t1+t2)#Output:(1, 2, 3, 4)
Example 2: Concatenating between different data types
示例2:在不同数据类型之间进行串联
t1=(1,2)
t2=[3,4]
print (t1+t2)#Output:TypeError: can only concatenate tuple (not "list") to tuple
Concatenating list objects:
串联列表对象:
Example 1: Concatenating two list objects
示例1:连接两个列表对象
l1=[1,2]
l2=[3,4]
print (l1+l2)#Output:[1, 2, 3, 4]
Example 2: Applying sorted() functions to list while concatenating
示例2:在连接时将sorted()函数应用于列表
l1=[1,2]
l2=[5,3,4]
print (l1+sorted(l2))#Output:[1, 2, 3, 4, 5]
串联列表与调用列表中的extend方法之间的区别: (The difference between concatenating lists and calling extend method in the list:)
Concatenating two lists will result in a new list object, but calling extend method will update the original list itself. Its return type is None
.
连接两个列表将产生一个新的列表对象,但是调用extend方法将更新原始列表本身。 其返回类型为None
。
l1=[1,2]
l2=[3,4]
l3=l1+l2
print (l3)#Output:[1,2,3,4]l1=[1,2]
l2=[3,4]
print (l1.extend(l2))#Output:Noneprint (l1)#Output:[1,2,3,4]
set和dictionary不支持串联。 (Concatenation not supported by set and dictionary.)
This will raise TypeError.
这将引发TypeError 。
l1={1,2}
l2={3,4}#print (l1+(l2))
#Output:TypeError: unsupported operand type(s) for +: 'set' and 'set'd1={'1':'a','2':'b'}
d2={'3':'c'}
print (d1+d2)#Output:TypeError: unsupported operand type(s) for +: 'dict' and 'dict'
重复: (Repetition:)
Sequences datatypes (both mutable and immutable) support a repetition operator *
The repetition operator *
will make multiple copies of that particular object and combines them together. When * is used with an integer it performs multiplication but with list, tuple or strings it performs a repetition
序列数据类型(可变和不可变的)都支持重复运算符*
重复运算符*
会制作该特定对象的多个副本并将它们组合在一起。 当*与整数一起使用时,它执行乘法,但与列表,元组或字符串一起时,它执行repetition
Syntax:
句法:
a*b
a*b
Example 1: Repetition operator on Strings
示例1:字符串上的重复运算符
s1="python"print (s1*3)#Output:pythonpythonpython
Example 2: Repetition operator on List
示例2:列表上的重复运算符
Items in the sequences are not copied but are referenced multiple times.
序列s中的项目不会被复制,但会被多次引用。
l1=[1,2,3]
print (l1 * 3)#Output:[1, 2, 3, 1, 2, 3, 1, 2, 3]
Example 3: Repetition operator on a nested list
示例3:嵌套列表上的重复运算符
Items in the sequences are not copied, they are referenced multiple times.l1=[[2]]l2=l1*2
序列s中的项目不会被复制,它们会被多次引用。 l1=[[2]]l2=l1*2
Elements in list l2 are referring to the same element in list l1. So, modifying any of the elements of list l1 will modify list l2 as well.
列表l2中的元素引用列表l1中的相同元素。 因此,修改列表l1的任何元素也将修改列表l2。
l1=[[2]]
l2=l1*2
print (l2)#Output:[[2], [2]]l1[0][0]=99
print (l1)#Output:[[99]]print (l2)#Output:[[99], [99]]

Example 4: Repetition operator on a tuple object
示例4:元组对象上的重复运算符
t=(1,2)
print (t*3)#Output:(1, 2, 1, 2, 1, 2)
Example 5: Repetition value is given as 0.
示例5:重复值为0。
When a value less than or equal to 0 is given, it will return an empty sequence of the same type.
当给出的值小于或等于0时 ,它将返回相同类型的空序列。
l1=[1,2,3]
print (l1 * 0)#Output:[]t=(1,2)
print (t*0)#Output:()
Example 6: *
operator on int object
示例6: *
int对象上的运算符
It will perform the multiplication operation for int object.
它将对int对象执行乘法运算。
print (2*3)#Output:6
Example 7:Repetition operator on set and dictionary object
示例7:集合和字典对象上的重复运算符
It will raise TypeError
.
它将引发TypeError
。
s1={1,2}#print (s1*2)
#Output:TypeError: unsupported operand type(s) for *: 'set' and 'int'd1={'a':1}
print (d1*2)#Output:TypeError: unsupported operand type(s) for *: 'dict' and 'int'
重复运算符vs repeat()函数 (Repetition operator vs repeat() function)
Repeat()This makes an iterator that returns object over again and again, and runs indefinitely unless the times argument is mentioned. If the times argument is not mentioned, it will return an infinite iterator. Set, dictionary , and data types are also supported in repeat() function. This method is supported by the itertools module.
Repeat()这使得迭代器可以一次又一次返回对象,并且可以无限期地运行,除非提到了times参数。 如果未提及times参数,它将返回一个无限迭代器。 Set(),dictionary和data类型也受repeat()函数支持。 itertools模块支持此方法。
import itertools#dict is used as an argumentl1=itertools.repeat({'a':1},times=3)
print (list(l1))#string is used as an argument.times argument is mentioned as 10.It will repeat the string 10 times.l2=itertools.repeat("hello",times=3)
print (list(l2))#Output:['hello', 'hello', 'hello']#list is used as argumentl3=itertools.repeat([1,2],times=2)
print (list(l3))#Output:[[1, 2], [1, 2]]#tuple is used as an argumentl4=itertools.repeat(('red','blue'),times=2)
print (list(l4))#Output:[('red', 'blue'), ('red', 'blue')]#set is used as an argumentl5=itertools.repeat({1,2},times=2)
print (list(l5))#Output:[{1, 2}, {1, 2}]
结论: (Conclusion:)
The concatenation and repetition operators are supported only by sequence datatypes except for when a range object is present. Both concatenation and repetition always result in a new object. Concatenation is done only between the same datatypes, and the difference between the concatenating list and the extend method is that concatenation results in a new list object where extend() will update the original list. Repetition can also be performed by using a repeat() method and this will return an iterator. Set and dictionary data types also supported in repeat() function.
序列数据类型仅支持串联和重复运算符,但存在范围对象时除外。 串联和重复总是产生一个新的对象 。 级联仅在相同的数据类型之间完成,并且级联列表和extend方法之间的区别在于级联会产生一个新的列表对象,其中extend()将更新原始列表。 也可以使用repeat()方法执行重复操作,这将返回一个迭代器 。 Set和字典数据类型在repeat()函数中也受支持。
I hope that you have found this article helpful, thanks for reading!
希望本文对您有所帮助,感谢您的阅读!
翻译自: https://codeburst.io/concatenation-and-repetition-in-python-d3f843a683c8
python字节流串联