node deno
Deno is Ryan Dahl’s (yeah, you guessed it right, the guy who created Node.js) latest venture.
D eno是Ryan Dahl的最新创举 (是的,您猜对了,这是创建Node.js的人 )。
But it isn’t just another JavaScript Engine. It also supports TypeScript — JavaScript’s strictly typed cousin — out of the box.
但这不仅仅是另一个JavaScript引擎 。 它还支持开箱即用的TypeScript (JavaScript的严格表亲)。
安装Deno (Installing Deno)
On macOS, you can install Deno using Homebrew — the open-source software package manager for macOS:
在macOS上,您可以使用Homebrew (用于macOS的开源软件包管理器)安装Deno:
brew install deno
Here’s a GIF to give you a better idea:
这是GIF,可让您有更好的主意:
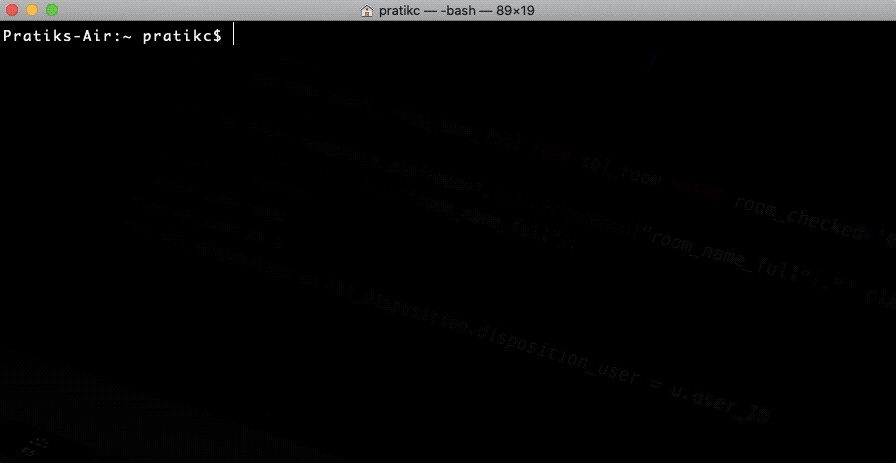
On Windows, Chocolately serves an alternative to macOS’s Homebrew:
在Windows上, Chocolately提供了macOS Homebrew的替代产品:
choco install deno
On Linux, good ol’ curl will do the job:
在Linux上,良好的卷曲将完成此工作:
curl -fsSL https://deno.land/x/install/install.sh | sh
Once Deno is installed, one can run the following command to see all the command-line options that are available:
安装了Deno后,可以运行以下命令来查看所有可用的命令行选项:
deno --help
The output of the above command will look something like below:
上面命令的输出如下所示:
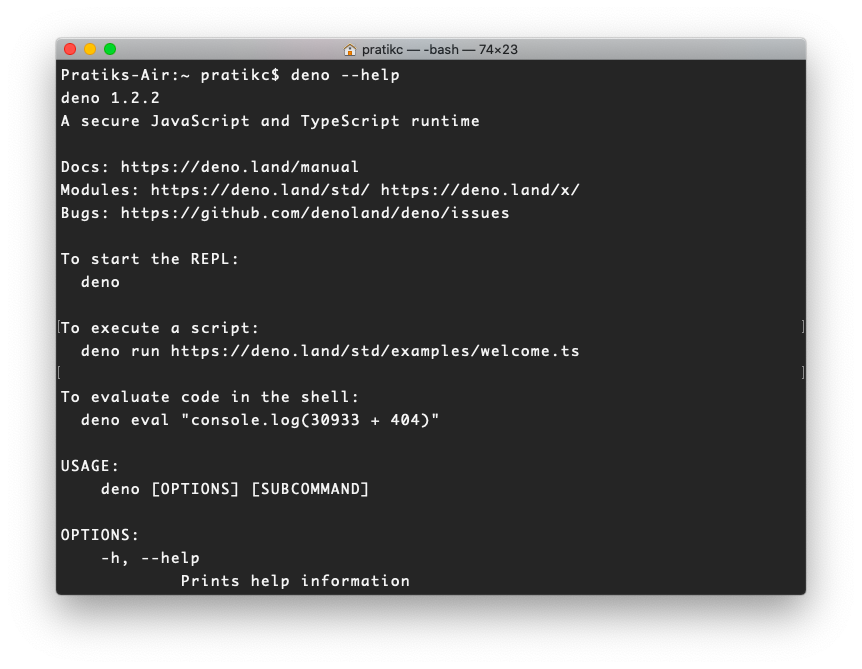
哈De,迪诺! (Hello, Deno!)
You can run Deno in REPL (Read-Eval-Print Loop) mode by simply executing the following command in the Terminal/Powershell/Shell:
您只需在Terminal / Powershell / Shell中执行以下命令,即可在REPL(读取-评估-打印循环)模式下运行Deno:
deno
Once the REPL is active, type the following code on the prompt and press the Enter/Return key:
REPL激活后,在提示符下键入以下代码,然后按Enter / Return键:
console.log('Hello, Deno!')
Here’s what you’ll see:
这是您将看到的内容:
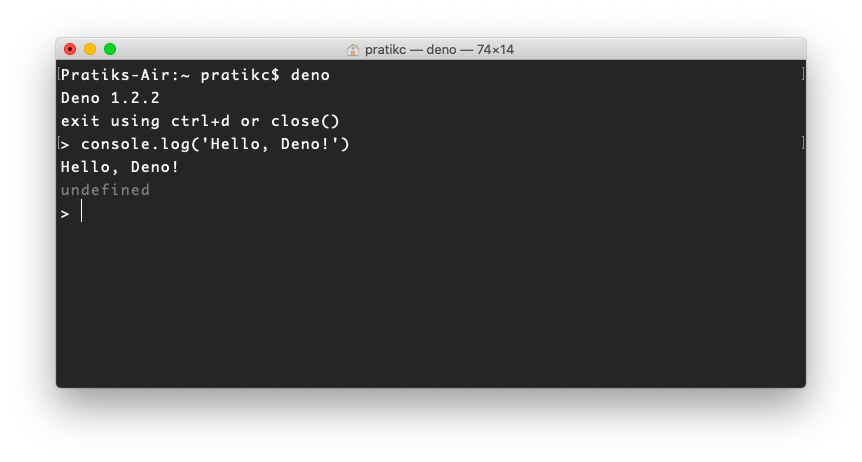
This is all good and fine. But it’s just like all other “Hello World!” programs out there!
一切都很好。 但这就像所有其他“ Hello World!”一样 程序在那里!
Let’s have some real fun.
让我们玩得开心。
Let’s see if we can build a web server in Deno!
让我们看看是否可以在Deno中构建Web服务器!
使用第三方/外部软件包 (Using third-party/external packages)
In Node.js, if you want to (or rather, need to) use a third-party library in your code, you first need to install it using npm, like so:
在Node.js中,如果要(或需要)在代码中使用第三方库,则首先需要使用npm进行安装,如下所示:
npm i express
And then you import it into your code using this:
然后使用以下命令将其导入代码中:
require('express')
But Deno allows you to import such packages directly. You just need to specify the package’s URL in your code. Let’s see how:
但是,Deno允许您直接导入此类软件包。 您只需要在代码中指定包的URL。 让我们看看如何:
Fire up the nano editor and add the following code to it:
启动nano编辑器,并向其中添加以下代码:
import { serve } from "https://deno.land/std@0.63.0/http/server.ts";const s = serve({ port: 8000 });console.log("http://localhost:8000/");for await (const req of s) {req.respond({ body: "Hello World\n" });}
Save this in a file named server.ts
.
将此保存在名为server.ts
的文件中。
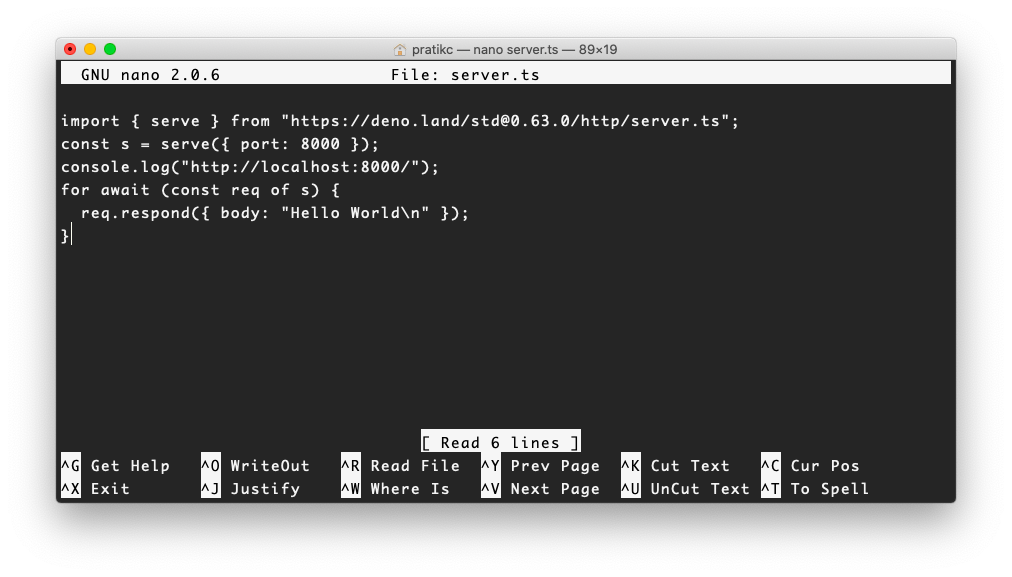
Exit the nano editor or open a new terminal and execute the following command:
退出nano编辑器或打开新终端并执行以下命令:
deno run server.ts
With the above command, the server should start listening over port 8000
.
使用以上命令,服务器应开始通过端口8000
侦听。
But what we get instead is this:
但是我们得到的是:
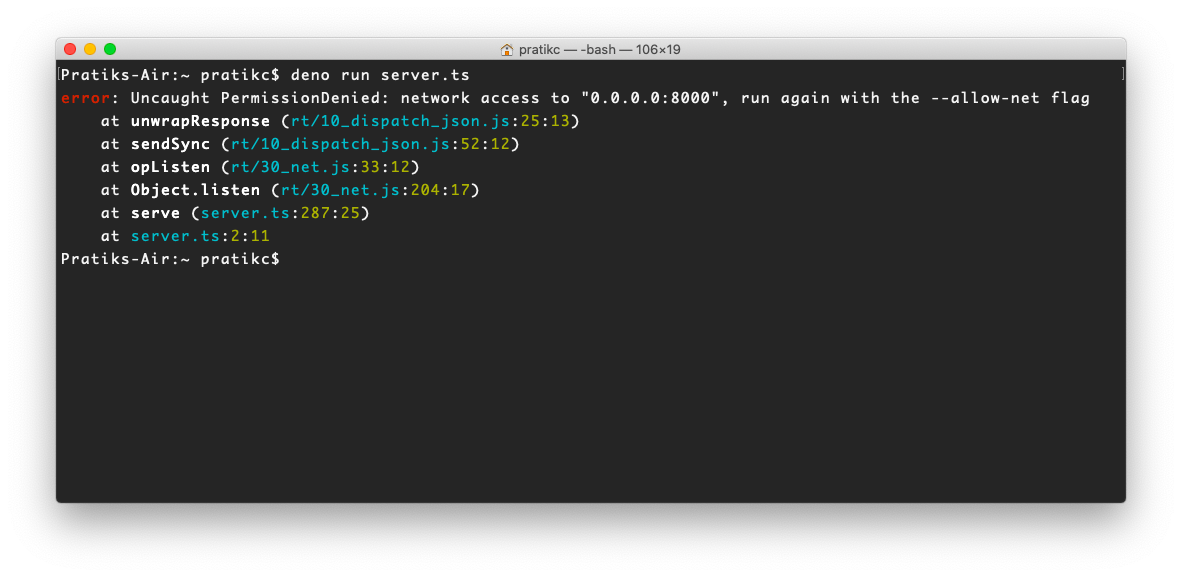
What does the following error in the above screenshot mean?
以上屏幕截图中的以下错误是什么意思?
error: Uncaught PermissionDenied: network access to "0.0.0.0:8000", run again with the --allow-net flag
You see, you need to explicitly grant Deno the permission to access the network. This is one of the many security features that are built into Deno (that’s why they call it a secure runtime).
您会看到,您需要显式授予Deno访问网络的权限。 这是Deno内置的许多安全功能之一(这就是为什么他们将其称为安全运行时)。
You can allow Deno to access the network by specifying the command line flag --allow-net
您可以通过指定命令行标志--allow-net
来允许Deno访问网络。
deno run --allow-net server.ts
And voila! The server starts listening for requests on port 8000.
瞧! 服务器开始在端口8000.
上侦听请求8000.
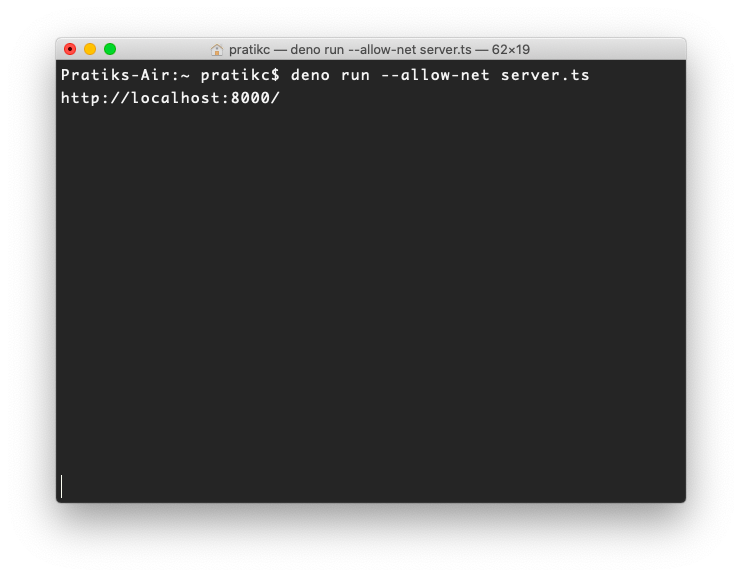
If you look at the following line of code, you’ll notice that we have specified the full URL of theserver.ts
file which contains the serve()
function that we use in our code to listen & serve requests on port 8000
.
如果看下面的代码行,您会注意到我们已经指定了server.ts
文件的完整URL,该文件包含了我们在代码中使用的serve()
函数,以侦听和服务端口8000
上的请求。
import { serve } from "https://deno.land/std@0.63.0/http/server.ts"
We didn’t have to install it using npm
beforehand. Deno automatically downloads it while executing the above code. Deno also downloads any other packages that might be required by server.ts
i.e., it also takes care of the transitive dependencies.
我们不必事先使用npm
安装它。 在执行上述代码时,Deno自动下载它。 Deno还会下载server.ts
可能需要的任何其他软件包,即,它还会处理传递依赖项。
This saves us the trouble of executing npm install
before running our programs.
这npm install
了我们在运行程序之前执行npm install
的麻烦。
This is something that’s new and unique to Deno (apart from the need for explicit permissions, that we saw before)and makes it stand apart from Node.js.
这是Deno的新功能和独特功能(除了对显式权限的需求(我们之前已经看到)),并使它与Node.js脱颖而出。
结束语 (Closing Comments)
So far, Deno seems to be a good alternative to Node.js.
到目前为止,Deno似乎是Node.js的不错选择。
But will it be able to take its place someday?
但是,有一天它能代替它吗?
Only time will tell.
只有时间证明一切。
翻译自: https://medium.com/swlh/meet-deno-the-new-javascript-typescript-runtime-639783ad832e
node deno