aws 自定义ip
Using AWS CloudFormation or CDK, you might find yourself needing to create a resource that is not available under CloudFormation resource types.
使用AWS CloudFormation或CDK,您可能会发现自己需要创建CloudFormation资源类型下不可用的资源。
In this post, you will learn how to use CDK to create an S3 object with AWSCustomResource and walk you through the life-cycle of your resource. We will also take a look at some pitfalls and gotchas that I encountered working with CDK AWSCustomResource.
在本文中,您将学习如何使用CDK和AWSCustomResource创建S3对象,并逐步介绍资源的生命周期。 我们还将看一下使用CDK AWSCustomResource时遇到的一些陷阱和陷阱。
I assume that you are somewhat familiar with CDK, but if not, I highly recommend giving it a try. You can go through the CDK Getting started guide from AWS to get going.
我认为您对CDK有点熟悉,但是如果没有,我强烈建议您尝试一下。 您可以阅读AWS的CDK入门指南 ,以继续学习。
If you just want to see how it works, head to the working example in GitHub and simply follow the instructions in README.MD.
如果只想看看它是如何工作的,请转到GitHub中的工作示例,然后按照README.MD中的说明进行操作。
什么是AWS自定义资源? (What are AWS Custom Resources?)
Custom Resources allow you to write custom logic in your CloudFormation deployment. You implement the creation, update, and deletion logic to define the custom resource deployment.
自定义资源允许您在CloudFormation部署中编写自定义逻辑。 您可以实现创建,更新和删除逻辑来定义定制资源部署。
CDK Implements the AWSCustomResource — a lambda-backed custom resource that uses the AWS SDK to provision your resources. This means that the CDK stack deploys a “provisioning lambda” which, upon deployment, calls the AWS SDK APIs that you defined for the resource lifecycle (create, update and delete).
CDK实施AWSCustomResource-一种由lambda支持的自定义资源,该资源使用AWS SDK来供应资源。 这意味着CDK堆栈会部署一个“配置lambda”,该lambda在部署后会调用您为资源生命周期(创建,更新和删除)定义的AWS开发工具包API。
问题 (The Problem)
Assume you are deploying your cloud stack which includes an S3 bucket. Being a CloudFormation resource type, the bucket is deployed, updated, and destroyed naturally. Now, for our example, assume that you also want to create some objects in your S3 bucket for a system configuration. How will you do that? You could naively create them using boto3, but this means that they will not be destroyed with your stack. Furthermore, they could prevent your stack from being destroyed when needed.
假设您正在部署包括S3存储桶的云堆栈。 作为CloudFormation资源类型,存储桶是自然部署,更新和销毁的。 现在,对于我们的示例,假设您还想在S3存储桶中创建一些对象以进行系统配置。 你会怎么做? 您可以使用boto3天真地创建它们,但这意味着它们不会被您的堆栈破坏。 此外,它们可以防止您的堆栈在需要时被破坏。
Custom Resources to the rescue!
自定义资源救援!
先决条件 (Prerequisites)
Access to an AWS account in which you can deploy your custom resources. Don’t forget to destroy your resources when you are done!
访问可以在其中部署自定义资源的AWS账户。 完成后,别忘了破坏资源!
A clean CDK project (you can do this) — I will use Python in this example.
一个干净的CDK项目(您可以执行此操作 )—在本示例中,我将使用Python。
- Ability to work with a CDK Python project in a virtual environment. 能够在虚拟环境中使用CDK Python项目。
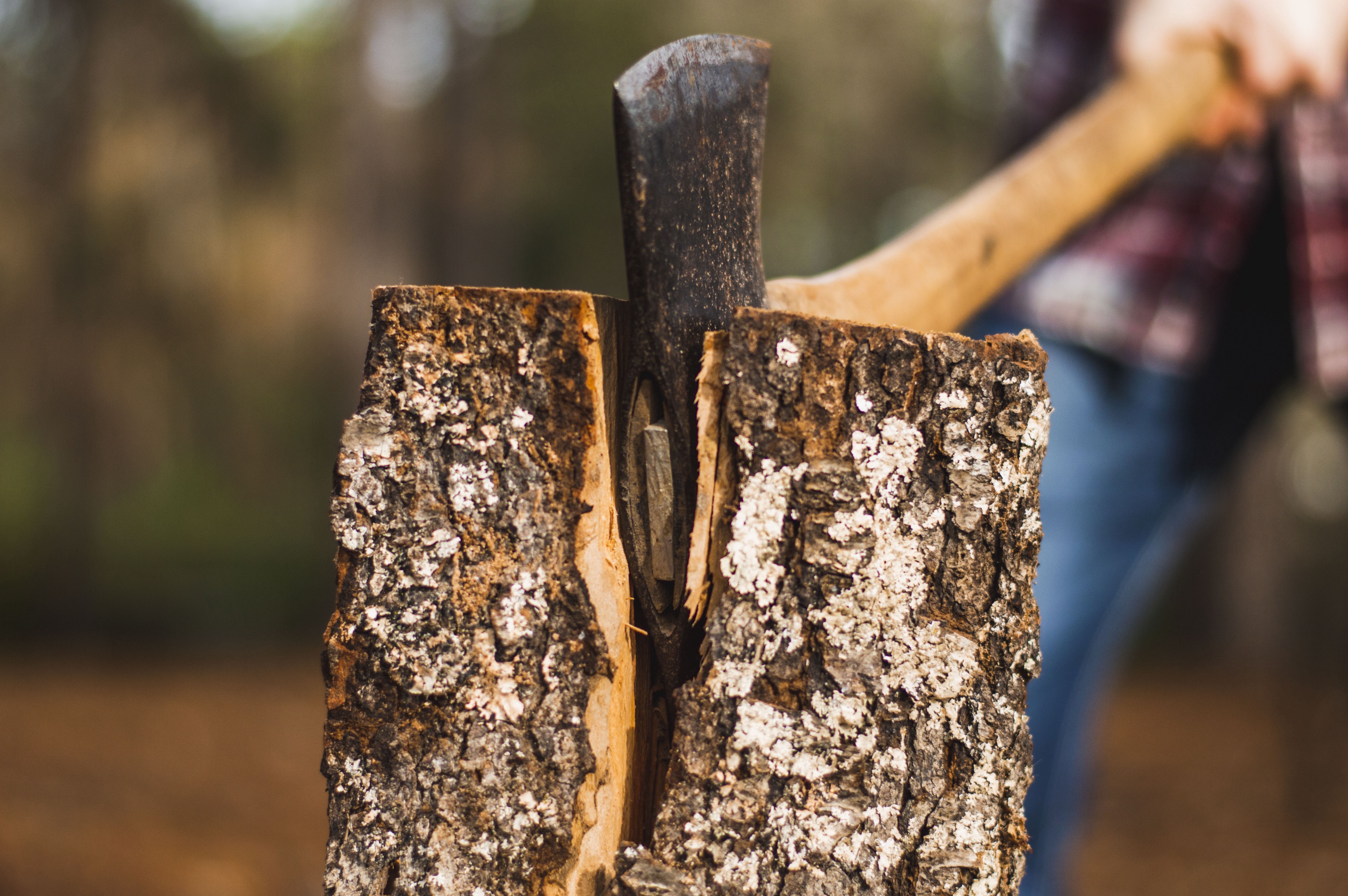
上班吧 (Let’s get to work)
We will start by creating a nice construct to host all this custom goodness, some of the imports will be used later.
我们将首先创建一个很好的构造来承载所有这些自定义优点,稍后将使用某些导入。
We created our own construct to wrap the creation of the custom object and make it friendlier. I exposed the log_retention of the custom resource, but it is totally optional and you can decide what you want to expose in AWSCustomResource.
我们创建了自己的构造,以包装自定义对象的创建并使其更友好。 我公开了自定义资源的log_retention,但它是完全可选的,您可以决定要在AWSCustomResource中公开的内容。
We also referenced the bucket from its bucket name so we can get its arn. You can of course simply pass the arn, but this way you can reference external buckets by name.
我们还从存储桶名称中引用了存储桶,以便获取其信息。 您当然可以简单地传递arn,但是通过这种方式,您可以按名称引用外部存储桶。
Moving on, we need to define the creation, update, and deletion of our resources. In the case of the S3 object, “update” is merely uploading a new object, so create and update are the same.
接下来,我们需要定义资源的创建,更新和删除。 对于S3对象,“更新”仅是上载新对象,因此创建和更新相同。
This code goes in the construct init function:
这段代码进入了构造函数init:
And the implementation is in the following member functions:
实现在以下成员函数中:
To understand how to implement the lifecycle events above, you need to turn to the AWS SDK API Reference. Since we want to create, update, and delete an S3 object, we need to look at the docs for S3.putObject and S3.deleteObject, where you can find the description of the parameters that you need to pass to it along with the API name (putObject/deleteObject) and the service name (S3).
要了解如何实现上述生命周期事件,您需要转到AWS开发工具包API参考。 由于我们要创建,更新和删除S3对象,因此需要查看S3.putObject和S3.deleteObject的文档,您可以在其中找到与API一起传递给它的参数的描述。名称(putObject / deleteObject)和服务名称(S3)。
IMPORTANT: For the physical resource id, you need to use something that will not change over deployments. I simply used the object full name which is unique and unchanging. If your physical id changes your resource will be deleted on an update, since CloudFormation considers these different resources.
重要信息 :对于物理资源ID,您需要使用在部署过程中不会改变的内容。 我只是简单地使用了对象的全名,它是唯一且不变的。 如果您的物理ID发生更改,则由于CloudFormation会考虑这些不同的资源,因此资源将在更新时被删除。
Next, we will create the policy, role, and the actual custom resource:
接下来,我们将创建策略,角色和实际的自定义资源:
Let’s break this down:
让我们分解一下:
First, we define the policy that will be added to the provisioning lambda role. cdk docs are a little unclear about this saying:
首先,我们定义将添加到预配置lambda角色的策略。 cdk文档对此说法尚不清楚:
The policy to apply to the resource.
应用于资源的策略。
This policy will actually be attached to the provisioning lambda role. A documentation issue was created and I expect the docs to be updated soon.
该策略实际上将附加到配置lambda角色。 创建了一个文档问题,我希望文档会尽快更新。
We let CDK decide what permissions are needed for the SDK API, by calling the from_sdk_calls
, and set the resource to the exact object name which is just what we need.
通过调用from_sdk_calls
,让CDK决定SDK API需要什么权限,并将资源设置为正是我们所需要的确切对象名。
Next, we create the role of the provisioning lambda, specifying lambda as the service principal, and attaching the managed policy for basic lambda execution. This will allow this lambda to write logs that can help us troubleshoot deployment issues. You can pass None as the role, but then you get no logs.
接下来,我们创建供应lambda的角色,将lambda指定为服务主体,并附加托管策略以执行基本lambda。 这将允许该lambda编写日志,以帮助我们解决部署问题。 您可以将None用作角色,但随后不会获得任何日志。
And finally, we create our custom resource:
最后,我们创建自定义资源:
I passed the default timeout just to stress that it’s there, if your resource requires, you can pass something else.
我通过默认超时只是为了强调它的存在,如果您的资源需要,您可以传递其他内容。
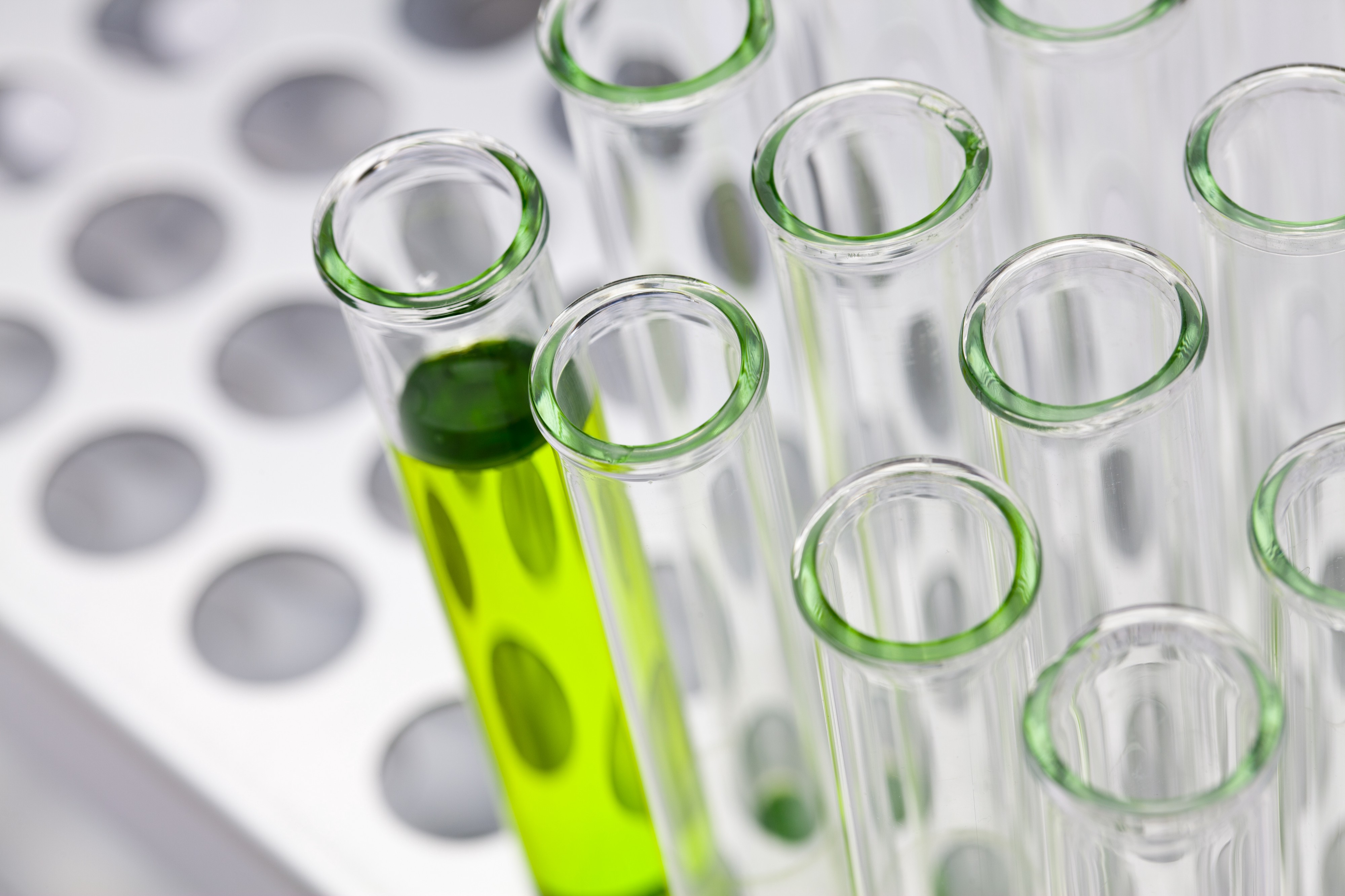
进行测试 (Putting it to the Test)
You are now ready to deploy, update, and destroy your stack. You can follow the instructions here, and make sure to check out your S3 object, as it is created, updated, and eventually deleted.
现在您可以部署,更新和销毁堆栈了。 您可以按照此处的说明进行操作,并确保在创建,更新并最终删除它时签出S3对象。
失败堆栈 (Failing Stacks)
If for some reason, your deployment is failing, you might experience a hanging stack deployment. This can occur if you have a problem with your lifecycle events like using the wrong API (create) for the on_delete event. this means that CloudFormation cannot remove this resource and will hang there for a long time.
如果由于某种原因部署失败,则可能会遇到挂起的堆栈部署。 如果您的生命周期事件有问题,例如对on_delete事件使用错误的API(创建),则会发生这种情况。 这意味着CloudFormation无法删除该资源,并且将在该资源上悬挂很长时间。
You can get rid of it manually by following this AWS guide, but I was also able to delete the stack by clicking the delete button, waiting for the stack to fail, and then delete again, checking the “Retain resource” which will allow the stack to be deleted. You can later manually delete the remaining resources.
您可以按照此AWS指南手动删除它,但是我也能够通过单击Delete按钮删除堆栈,等待堆栈失败,然后再次删除,然后选中“保留资源”要删除的堆栈。 您以后可以手动删除剩余资源。
结语 (Wrapping Up)
In a world of automation and infrastructure-as-code, we must make sure that the resources we deploy have a well-defined life-cycle.
在自动化和基础架构即代码的世界中,我们必须确保部署的资源具有明确定义的生命周期。
AWS Custom Resources can be used to deploy resources with CloudFormation that are not supported otherwise. This will keep your deployment consistent and without “rogue” resources, which management can later cost you a lot of time.
AWS Custom Resources可用于通过CloudFormation部署否则不支持的资源。 这将使您的部署保持一致,并且没有“流氓”资源,这以后的管理可能会花费您很多时间。
With CDK, you can use the example above as the basis for creating your own custom resources as part of your CloudFormation deployment.
借助CDK,您可以将以上示例用作创建自己的自定义资源(作为CloudFormation部署的一部分)的基础。
翻译自: https://medium.com/cyberark-engineering/custom-resources-with-aws-cdk-d9a8fad6b673
aws 自定义ip