swift 加载动画
Difficulty: Beginner | Easy | Normal | Challenging
难度:初学者| 容易| 普通 | 具有挑战性的
This article has been developed using Xcode 11.4.1, and Swift 5.2.2
本文是使用Xcode 11.4.1和Swift 5.2.2开发的。
Want to display a loading screen that can appear when you’re doing some heavyweight operations? Perhaps you want this with when you are producing your App with a Storyboard, perhaps you want to do this programatically. Either way, this article has you covered.
是否想要显示在执行某些重量级操作时可能出现的加载屏幕? 在使用情节提要制作应用程序时,也许您希望这样做,也许您希望以编程方式进行此操作。 无论哪种方式,本文都涵盖了您。
Oh, and there are versions using CABasicAnimation
and UIView.animate
all nestled rather nicely in the attached Repo.
哦,有一些使用CABasicAnimation
和UIView.animate
版本都很好地UIView.animate
在附加的Repo中 。
先决条件: (Prerequisites:)
You will be expected to be aware how to make a Single View Application() in Swift.
您将被期望知道如何在Swift中制作Single View Application ()。
This article uses storyboard constraints and loadview
术语: (Terminology:)
Storyboard: A visual representation of the User Interface of an Application
故事板:应用程序用户界面的直观表示
目标 (The goal)
This article is about producing a rather wonderful loading screen, with an infinitely scrolling background and a tree moving in parallax.
本文是关于制作一个非常漂亮的加载屏幕,它具有无限滚动的背景和一棵视差移动的树。
How is it possible to do this, when using the Storyboard
?
使用Storyboard
时如何做到这一点?
故事板版本 (The Storyboard version)
A new UIViewController
can be created that will have two background images side-by-side.
可以创建一个新的UIViewController
,它将并排具有两个背景图像。
Now for this to adequately work, the background images must be at least double the width of the devices screen you wish to cover.
现在,要使其正常工作,背景图像必须至少是要覆盖的设备屏幕宽度的两倍。
The Storyboard
is created as below with constraints to keep the images horizontally centered in the screen.
Storyboard
的创建如下所示,并具有一些约束条件,以使图像在屏幕中水平居中。
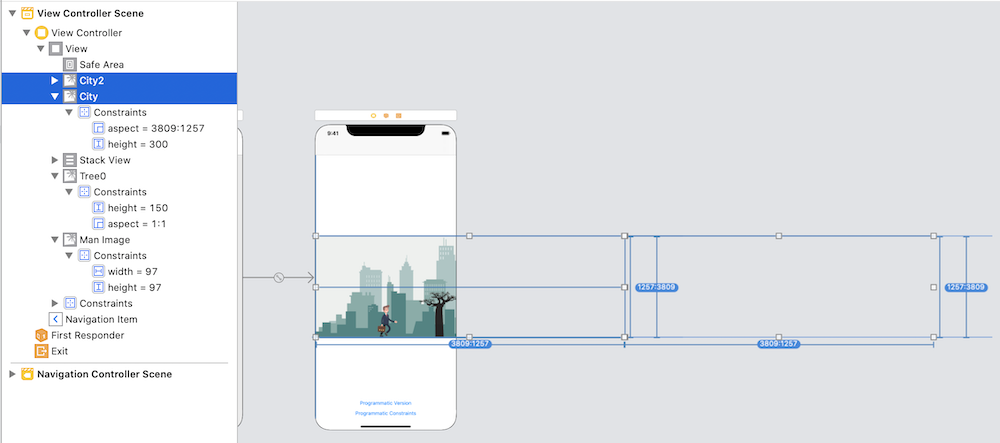
The man has a single static image (we will animate walking this in code), as does the tree and both are in static positions on the screen that can be moved later.
这个人只有一个静态图像(我们将以动画方式在代码中进行遍历),树也是如此,并且两者都位于屏幕上的静态位置,可以稍后移动。
Animating the man
动画的人
Within viewDidLoad()
we can animate the man walking (albeit on the spot), appending images from the asset catalog.
在viewDidLoad()
我们可以为步行的人(尽管是当场)设置动画,并添加资产目录中的图像。
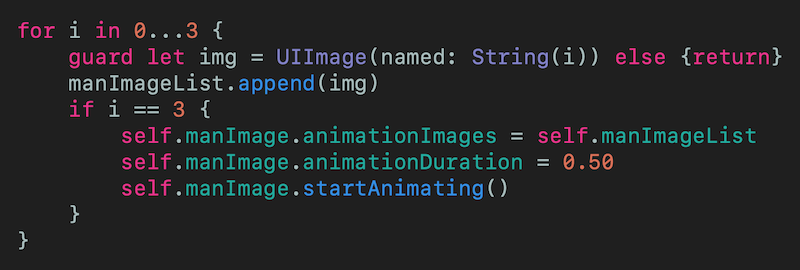
Fading in and out nicely
淡入淡出
Within the Storyboard
I set the man, the tree and the two backgrounds as invisible — the alpha as 0.0
for each of them.
在Storyboard
我将人,树和两个背景设置为不可见-每个背景的Alpha设置为0.0
。
Then before we animate we have the following code, using .curveEaseOut
to weight the animation towards the beginning of the animation.
然后,在制作动画之前,我们需要使用以下代码,使用.curveEaseOut
将动画权重设置为动画的开始。

with a similar animation to make them fade out just before the screen disappears. viewWillDisappear
is called just before the view disappears from the screen, that is when we press one of the buttons to see the other implementation variants of this code!
并使用类似的动画使它们在屏幕消失之前淡出。 viewWillDisappear
在视图从屏幕消失之前被调用,也就是说,当我们按下其中一个按钮以查看此代码的其他实现变体时!
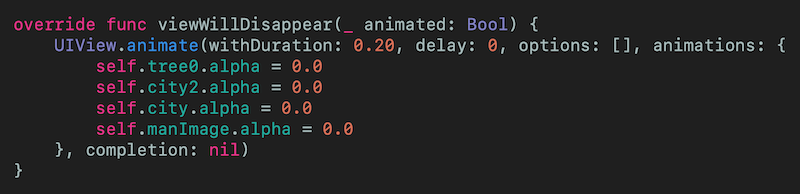
The animation
动画
Here I am using CABasicAnimation
— the tricky part here is to make sure that the first city city
and the second city city2
move at the same pace across the screen, here working through the self.city.frame.size.width
.
在这里,我使用的是CABasicAnimation
这里最棘手的部分是要确保第一个城市city
和第二个城市city2
在屏幕上以相同的速度移动,这是通过self.city.frame.size.width
。
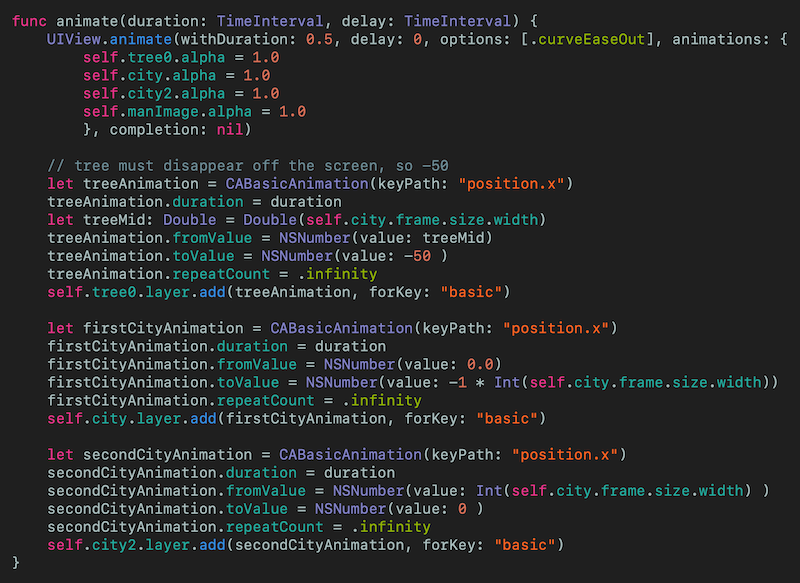
This animate function is called from viewDidAppear
, a good place to start the animation as the constraints are set (everything is in position) and this is called just before the view is visible to the user.
这个动画函数从viewDidAppear
调用,这是设置了约束(一切都就位)的开始动画的好地方,并且在用户对视图可见之前调用它。
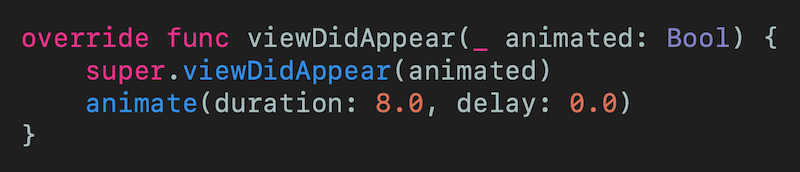
程序版本 (The programmatic version)
The ProgrammaticViewController
displays the items programmatically, but animating the man is done as before!
ProgrammaticViewController
显示项目,但是像以前一样对人进行动画处理!
**Load the view**
** 加载视图 **
Nothing much to see here, but this is much like my guide to programmatically create a view with loadview.
这里没什么可看的,但这很像我的指南,以编程方式使用loadview创建视图。
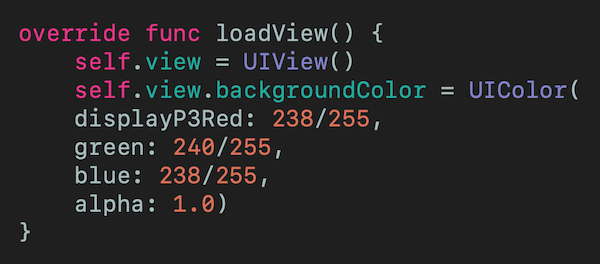
Adding the objects
添加对象
I’ve added the objects in viewWillAppear
since the frame of the UIView
is set at this point, but it is before the user will see the screen.
由于已在此处设置UIView
的框架,因此已在viewWillAppear
添加了对象,但这是在用户看到屏幕之前。
Here is the code:
这是代码:
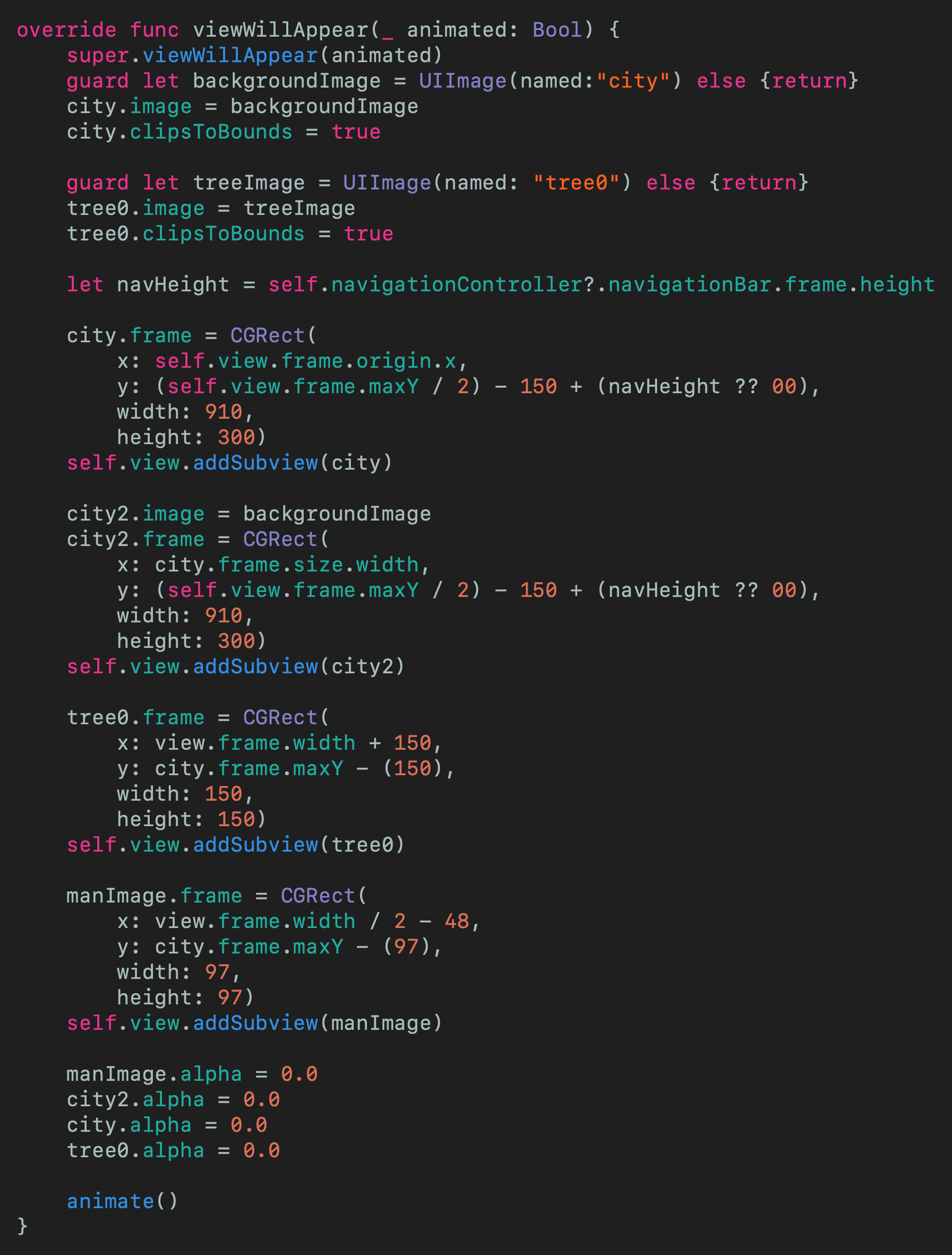
The animation
动画
Called once again from viewWillAppear
however I’ve put a little delay to make this seem like a nicer transition in my NavigationBar
implementation here.
从viewWillAppear
再次调用,但是在这里的我的NavigationBar
实现中,我稍加延迟以使其看起来更好。
Here I’m using UIView.animate
, and this means the animation is working in just a few lines!
在这里,我使用的是UIView.animate
,这意味着动画仅用几行就能工作!
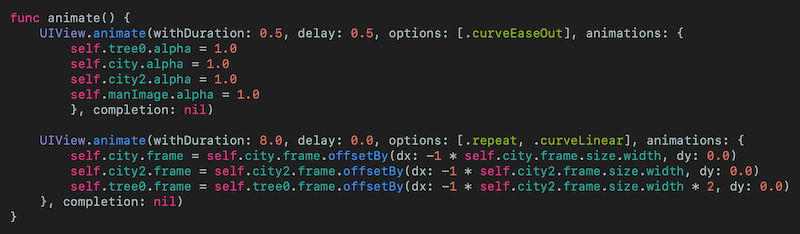
BUT by not using constraints…what happens when we rotate a device? Bad things happen! Of course I could do some various fixes for this, but why not just use constraints?
但是通过不使用约束...当我们旋转设备时会发生什么? 坏事发生了! 当然,我可以为此做一些修复,但是为什么不只使用约束呢?
使用约束的程序版本 (The programmatic version using constraints)
Animating the man is done as before, when we choose to load in views it doesn’t matter if we have constraints
or not.
对该人进行动画处理与以前一样,当我们选择加载视图时,是否有constraints
没有关系。
In fact, all the animation is done in much the same way.
实际上,所有动画的制作方法都差不多。
Load the view
加载视图
Now this is where we are creating the subviews in code. The constraints
here mirror those set up in the Storyboard
version detailed above.
现在,这是我们在代码中创建子视图的地方。 这里的constraints
与上面详细说明的Storyboard
版本中设置的constraints
。
*Interesting* parts here might be considered to be `clipsToBounds` which keeps the UIImage
within the bounds, and of course translatesAutoresizingMaskIntoConstraints
to let the compiler know that we are taking care of the constraints
here.
*有趣的 *部分可能被认为是`clipsToBounds`,它使UIImage
保持在边界之内,当然, translatesAutoresizingMaskIntoConstraints
可以使编译器知道我们在这里处理constraints
。
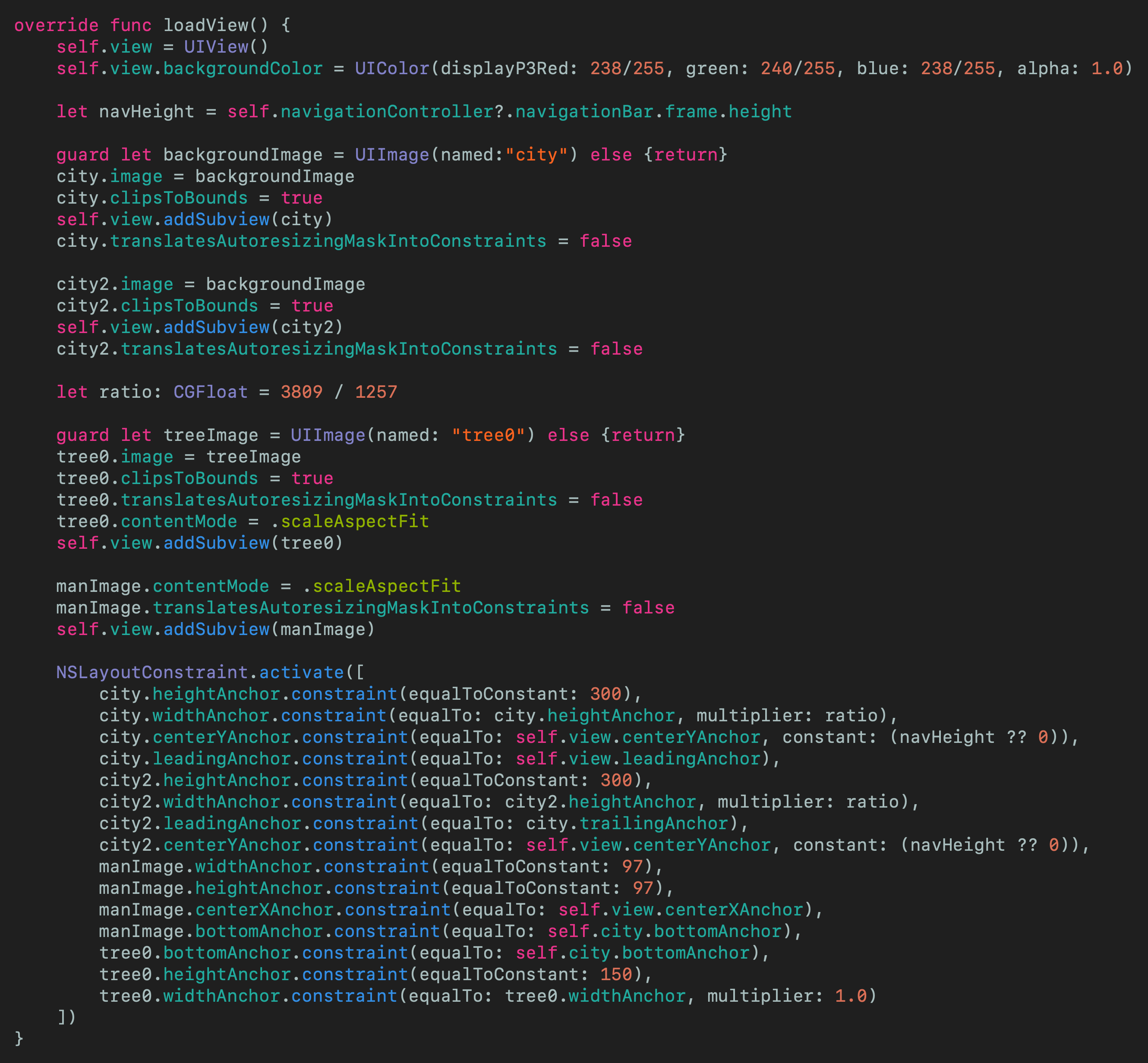
结论 (Conclusion)
This article is useful to help you with the difference between Storyboard
and constraints
.
本文对于帮助您区分Storyboard
和constraints
之间的区别很有用。
My take on this is that the version using constraints
programatically is not only easier to write, but easier to maintain and change in the future. If you’re not that familiar with walking away from the storyboard
you might enjoy my storyboard technical article.
我对此的看法是,以编程方式使用constraints
的版本不仅易于编写,而且将来也易于维护和更改。 如果您不熟悉离开storyboard
您可能会喜欢我的情节提要技术文章。
It is rather complex in the description, but rather easy in the implementation. Take a look at the attached Repo. It is a rather wonderful thing.
它的描述相当复杂,但实现起来却很容易。 看看所附的Repo 。 这是一件相当美妙的事情。
If you’ve any questions, comments or suggestions please hit me up on Twitter
如果您有任何疑问,意见或建议,请在Twitter上打我
翻译自: https://medium.com/@stevenpcurtis.sc/create-a-loading-animation-in-swift-75788a488fdb
swift 加载动画