什么是接口
The ‘this’ keyword in Javascript is one among the most commonly misunderstood concepts because its behaviour differs based on how and where it was called.
Javascript中的' this '关键字是最常被误解的概念之一,因为其行为因调用方式和调用位置而异。
The ‘this’ keyword refers to the current object/function/method that they are working with and it’s not peculiar to Javascript, it’s also available in other languages as well, but here in Javascript it behaves differently compared to those languages. In Javascript, the context of ‘this’ differs based on how you call, and whether it’s been executed in strict mode or non-strict mode.
“ this”关键字是指他们正在使用的当前对象/函数/方法,它不是Javascript特有的,它也可以在其他语言中使用,但是在Javascript中,它的行为与那些语言不同。 在Javascript中,“ this”的上下文取决于您的调用方式以及是以严格模式还是非严格模式执行的。
浏览器和Node.js: (Browser & Node.js:)
In the browser console if you type ‘this’ then it will print the window object for you, and in node.js REPL if you type this it will print the globalThis.
在浏览器控制台中,如果键入“ this ”,它将为您打印窗口对象;在node.js REPL中,如果键入此对象,则将打印globalThis 。
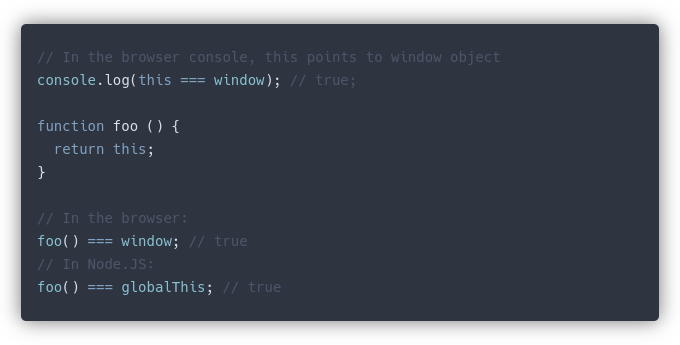
call(),apply()和bind() (call(), apply() & bind())
As already mentioned the ‘this’ keywords context differs based on where and how it’s been called. When called in strict mode, the context of ‘this’ will be undefined. But you can set the context for ‘this’ using either call() or apply() or bind() functions.
如前所述,“ this ”关键字上下文根据在何处以及如何调用而有所不同。 在严格模式下调用时,“ this ”的上下文将是不确定的。 但是您可以使用call()或apply()或bind()函数为“ this”设置上下文。
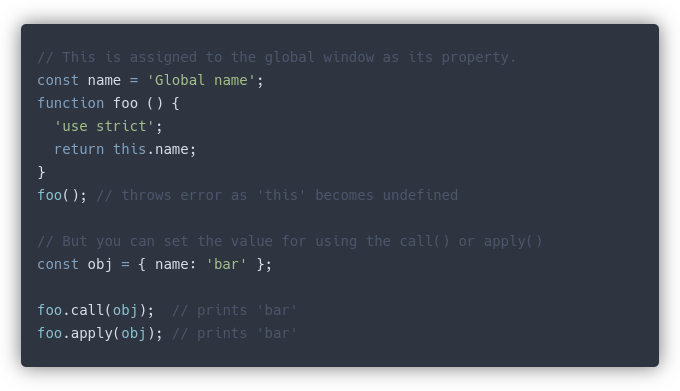
In the above example, the function foo() uses strict mode, and thus the value of ‘this’ becomes undefined and throws an error when we try to access a property from it. But if we have tried the same function without the ‘use strict’ then ‘this’ would have pointed to the global context and ‘this.name’ would have printed ‘Global name’.
在上面的示例中,函数foo()使用严格模式,因此,当我们尝试从中访问属性时,' this '的值变为未定义,并引发错误。 但是,如果我们在不使用“ use strict ”的情况下尝试了相同的功能,则“ this ”将指向全局上下文,而“ this.name ”将显示“ Global name ”。
Also as you could see on the next line we have created an object(obj), and after that when we did foo.call(obj) and foo.apply(obj) we got the output of ‘bar’, that is because both the call() & apply() function sets the value of ‘this’ into whatever we pass as the first argument to them.
就像您在下一行看到的那样,我们创建了一个object(obj),然后在执行foo.call(obj) 和 foo.apply(obj)之后,我们得到了“ bar ”的输出,这是因为call()和apply()函数将“ this”的值设置为我们传递给它们的第一个参数的值。
The difference between the call() and apply() is that, call() expects the parameters to passed as individual arguments, but in apply() the arguments are provided as an array.
call()和apply()之间的区别在于,call()期望将参数作为单独的参数传递,但是在apply()中,参数作为数组提供。
call:
foo.call(obj, a, b);apply:
foo.apply(obj, [a, b])
There is one more way to achieve the same which is .bind() and the difference between bind() and the other two is that when we use .bind() it returns a new function and does not execute the function right away like call() and apply().
还有另一种方法可以实现.bind() ,bind()与其他两种方法的区别在于,当我们使用.bind()时,它将返回一个新函数,并且不会像调用那样立即执行该函数()和apply()。
const bar = foo.bind(obj)
bar();
功能与方法: (Functions & methods:)
When a function is defined within the scope of an object or class then it is called as a method. Though they may appear like there are only syntactical differences but, there are some behavioral differences as well. One to start with is the behavior of ‘this’ inside a function and a method.
如果在对象或类的范围内定义函数,则将其称为方法。 尽管它们看起来似乎只有句法上的差异,但行为上也有一些差异。 首先是函数和方法中“ this ”的行为。
Within a method: ‘this’ refers to the current object/owner the method belongs.
在方法内: “ this”是指该方法所属的当前对象/所有者。
Within a function: By default, the ‘this’ points to the global object. When invoked using the call() or apply() or bind() function then it refers to whatever argument we have passes to them. When a function is called as a constructor using the new, then the ‘this’ refers to the new instance.
在函数内:默认情况下,“ this ”指向全局对象。 当使用call()或apply()或bind()函数调用时,它将引用我们传递给它们的任何参数。 当使用新函数将函数作为构造函数调用时,则“ this”指的是新实例。
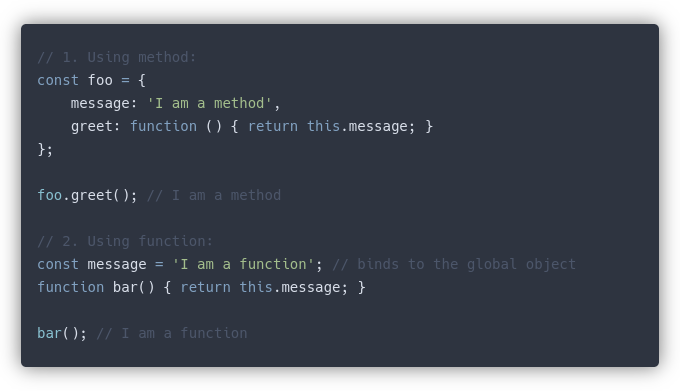
方法: (Method:)
As you could see in the first case greet() is a method inside the object, and when we call greet it returns ‘I am a method’ because the ‘this’ inside greet(method) refers to the current object/owner which is here foo.
如您在第一种情况下所见, greet ()是对象内部的方法 ,当我们调用greet时,它返回“我是方法”,因为greet(method)内的“ this ”是指当前对象/所有者。这里foo 。
功能: (Function:)
In the second case bar() is a function and the ‘this’ was not set while calling the function so the ‘this’ inside it points to the global object, and since the constant message was set in the global object, bar() prints ‘I am a function’.
在第二种情况下, bar()是一个函数,并且在调用函数时未设置“ this ”,因此其内部的“ this”指向全局对象,并且由于常量消息已在全局对象中设置,因此bar()打印“我是功能”。
箭头功能: (Arrow Function:)
Arrow function is the new addition to the Javascript family ever since the release of ES6, and this made the traditional functions look more cleaner and easy to visualize, but unlike a normal function the arrow function has a different behavior of ‘this’.
自ES6发行以来,箭头功能是Java脚本家族的新增功能,这使传统功能看起来更简洁,更易于可视化,但是与普通功能不同,箭头功能的行为不同于“此”。
The arrow functions have lexical scoping and thus the ‘this’ inside an arrow function points to the closest function/method scope.
箭头函数具有词法作用域,因此箭头函数内部的“ this”指向最接近的函数/方法范围。
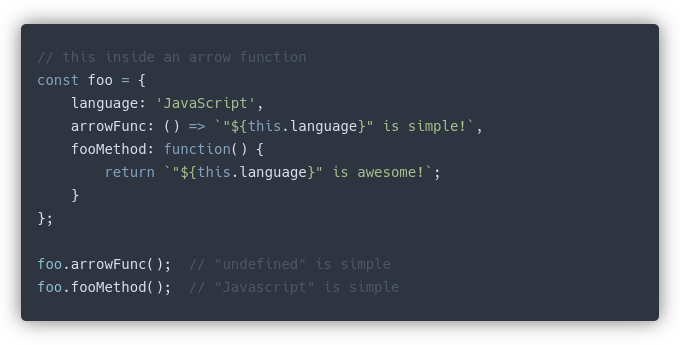
Here foo is an object with a method named fooMethod() and another method named arrowFunc() which uses the ES6 arrow functions. If you see the result of both the cases the ‘this.language’ rendered different results.
此处foo是一个对象,该对象具有一个名为fooMethod()的方法和另一个名为ESF的方法,该方法使用ES6箭头函数。 如果您看到这两种情况的结果,那么“ this.language”将呈现不同的结果。
In arrowFunc() ‘this.language’ returns undefined but in fooMethod(), it returned ‘JavaScript’.
在arrowFunc()中, “ this.language”返回未定义,但在fooMethod()中 ,其返回“ JavaScript”。
As mentioned earlier, arrow function has lexical scoping and ‘this’ points to the nearby function scope, here foo is an object and not a function, and there are no other functions wrapping foo so ‘this’ points to the global object and we haven’t set any key in the global object as language. so the arrowFunc() printed undefined when we tried ‘this.language’.
如前所述,箭头函数具有词法作用域,“ this”指向附近的函数范围,此处foo是一个对象而非函数,并且没有其他包装foo的函数,因此“ this”指向全局对象,我们没有请勿将全局对象中的任何键设置为语言。 因此当我们尝试 “ this.language” 时 , arrowFunc()的输出 未定义 。
Whereas for fooMethod() the ‘this’ points to the current working object(foo) and thus it prints ‘JavaScript’. Now let’s try the same example with an arrow function declared within a normal function.
而对于fooMethod()来说 ,“ this”指向当前的工作对象(foo),从而显示“ JavaScript”。 现在,让我们尝试在普通函数中声明箭头函数的示例。
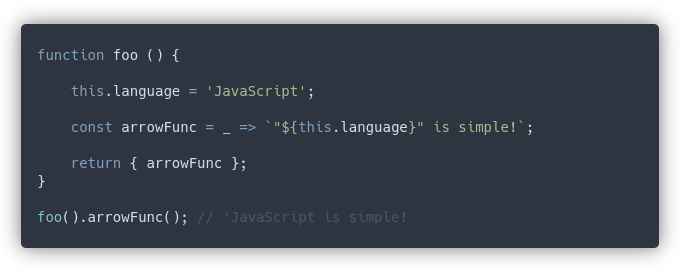
arrowFunc(): Here the ‘this’ points to the nearby function scope which is foo(), which has a ‘this’ pointing to the global object and we have appended a key ‘language’ to the ‘this’ of foo(). So when we try ‘this.language’ in the arrowFunc() it prints ‘JavaScript’ is simple.
arrowFunc ():此处的“ this ”指向附近的函数范围foo (),该函数范围具有指向全局对象的“ this”,并且我们在foo()的“ this”后面附加了键“ language” 。 因此,当我们在arrowFunc()中尝试使用“ this.language”时,其输出的“ JavaScript”非常简单。
‘this’ for a normal function is determined based on the below steps:
正常功能的“ this ”是根据以下步骤确定的:
- If it was explicitly set using call(), apply() or bind() then the values set in the argument is used as ‘this’. 如果使用call(),apply()或bind()进行了显式设置,则参数中设置的值将用作“ this”。
- If the function is called as a constructor then it points to the new instance values provided 如果该函数被称为构造函数,则它将指向提供的新实例值
- If there is no explicit value provided then it defaults to the global object 如果没有提供显式值,则默认为全局对象
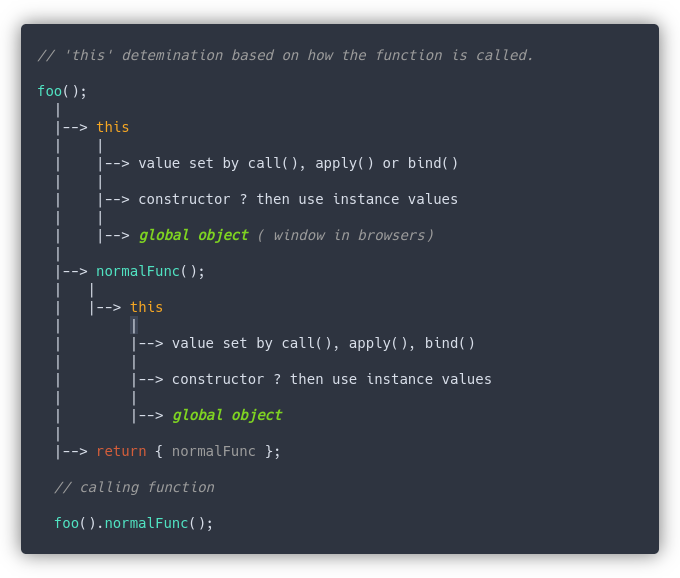
‘this’ in JavaScript could be so confusing some times, but it’s not much complicated as rocket science, we have to clear on where and how we use it.
JavaScript中的“ this ”有时可能会令人困惑,但是它并不像火箭科学那样复杂,我们必须弄清楚我们在哪里以及如何使用它。
Happy coding!
编码愉快!
翻译自: https://medium.com/swlh/what-is-this-keyword-in-javascript-and-how-it-behaves-4929689bd114
什么是接口