java扎实书籍
Though I am a researcher at the moment, I find myself being a better research/scientist/whatever whenever I use my software engineering knowledge to develop my algorithms. In this article, I am going to share my first ever software development/programming encounter. This was the project that we had to complete during the first semester in the Department of Computer Science and Engineering at my undergrad university (University of Moratuwa, Sri Lanka).
尽管我目前是一名研究人员,但是无论我何时使用我的软件工程知识来开发算法,我都会成为一名更好的研究人员/科学家。 在本文中,我将分享我的第一次软件开发/编程经验。 这是我们本科生大学(斯里兰卡莫拉图瓦大学)计算机科学与工程系第一学期必须完成的项目。
This was indeed a very challenging exercise given that I very poor knowledge of JAVA (which I did the project in). Choice of programming language was free, however, JAVA was my go-to-choice since it seemed easy compared to C/C++. Moreover, NetBeans being was an easy to use GUI development tool. I believe these naive choices may have helped apprentice myself to master programming with much less effort. I must thank Dr. (Mrs.) Surangika Ranathunga (sole course convenor/lecturer) for injecting such a huge amount of knowledge to many new students in just 4 months (1 Semester). It was difficult but the outcome was amazing. Anything that comes helpful out of this article must reflect her capability of lecturing and mentoring newbies. Let’s go through the project!
考虑到我对JAVA的了解非常少(我在该项目中从事过这项工作),所以这确实是一个非常具有挑战性的练习。 编程语言的选择是免费的,但是,JAVA是我的首选,因为与C / C ++相比,它似乎很容易。 而且,NetBeans是一个易于使用的GUI开发工具。 我相信这些天真的选择可能有助于徒弟们轻松地精通编程。 我必须感谢Surangika Ranathunga博士(唯一的课程召集人/讲师)在短短4个月(1个学期)内为许多新生提供了如此丰富的知识。 很难,但结果令人惊讶。 从本文中得出的任何帮助都必须反映出她讲授和指导新手的能力。 让我们来看一下这个项目!
大纲 (Outline)
The objective is to develop a spreadsheet program with basic data types, cell referencing and ability to tokenize cell content into datatypes. The complete implementation must follow OOP concepts (Inheritance, Abstraction, Encapsulation and Polymorphism) whereas applicable.
目的是开发一种具有基本数据类型,单元格引用和将单元格内容标记为数据类型的能力的电子表格程序。 完整的实现必须遵循OOP概念(继承,抽象,封装和多态性)(如果适用)。
第一个任务字符串标记器 (First Task-String Tokenizer)
One of the best ways to learn a programming language is by using the syntax in practice. Also, being a programmer one must know how to use REGEX (Regular Expressions) and Algorithms (though that is a completely different course in university) one must do a good project. I believe implementing a simple tokenizer and an algorithm to simplify mathematical strings could be one such way of learning them at once.
学习编程语言的最好方法之一是在实践中使用语法。 另外,作为一名程序员,必须知道如何使用REGEX(正则表达式)和算法(尽管在大学中这是一门完全不同的课程),但必须做一个好的项目。 我相信实现简单的标记器和简化数学字符串的算法可能是一次学习它们的一种方式。
- The tokenizer should identify datatypes; Integer, Float, String, Date/Time, Currency. 分词器应标识数据类型; 整数,浮点数,字符串,日期/时间,货币。
If the string is a mathematical expression like in excel
=500*10/5
the value must be evaluated to1000
.如果字符串是一个数学表达式,例如excel
=500*10/5
10/5,则该值必须计算为1000
。
Switching between the above cases can be done using the prefix =
in the string. The regexes I used for case 1 are as follows.
可以使用字符串中的prefix =
在上述两种情况之间切换。 我在情况1中使用的正则表达式如下。
booltype = ".*?(((=?[<>])|(!=)|([<>]=?)|<>|><)|==|=).*?";
currency = "[A-Za-z$]{1,2}[ .]?[0-9][0-9]*[.]?[0-9]*";time12hr = "(1[012]|[1-9]):[0-5][0-9]?[:]?[0-5][0-9]?(am|AM|pm|PM)"; time24hr = "(2[0-4]|1[0-9]|[0-9]):[0-5][0-9]?[:]?[0-5][0-9]?"; integer = "[-]?[0-9]*";
floating = "[-]?[0-9]*[.][0-9]*";dateformatSL = "[0-9]{2,4}[/-](1[012]|0?[0-9])[/-](1[0-9]|0?[0-9]|2[0-9]|3[01])";dateformatINTL = "(1[0-9]|0?[0-9]|2[0-9]|3[01])[/-](1[012]|0?[0-9])[/-][0-9]{2,4}";
I have my naive implementation here. However, in simple terms, one can have a static class to hold functions that could parse the string and return parsed sections (type, formatted/evaluated content, original content). Note that after parsing we must retain original content similar to excel.
我在这里有幼稚的实现。 但是,简单来说,可以有一个静态类来容纳可以解析字符串并返回已解析部分(类型,格式化/评估内容,原始内容)的函数。 请注意,解析后,我们必须保留类似于excel的原始内容。
For case 2, one must implement the algorithm; Shunting-yard. This simply pushes open brackets into a stack and solve by backtracking when a closing bracket is met. If you have an equation like 12*(5+6)
you push to stack until you find the second closing bracket. Then pop until the first open bracket is out, then simplify 5+6
and push again into the stack. Finally, the stack will be brackets free and you can use BODMAS to simplify the final stack. You can have a look at my implementation here.
对于情况2,必须实现该算法; 调车场 。 这简单地将打开的括号推入堆栈,并在遇到闭合括号时通过回溯来解决。 如果您的方程式为12*(5+6)
,则将其推入堆栈直到找到第二个右括号。 然后弹出,直到第一个开放式括号出来,然后简化5+6
并再次推入堆栈。 最后,堆栈将不包含在括号内,您可以使用BODMAS简化最终堆栈。 您可以在这里查看我的实现。
Now the two classes are complete. The first class will parse items, the second class will be used by the first if the input string is an expression.
现在,这两个课程已经完成。 如果输入字符串是表达式,则第一类将解析项目,第二类将由第一类使用。
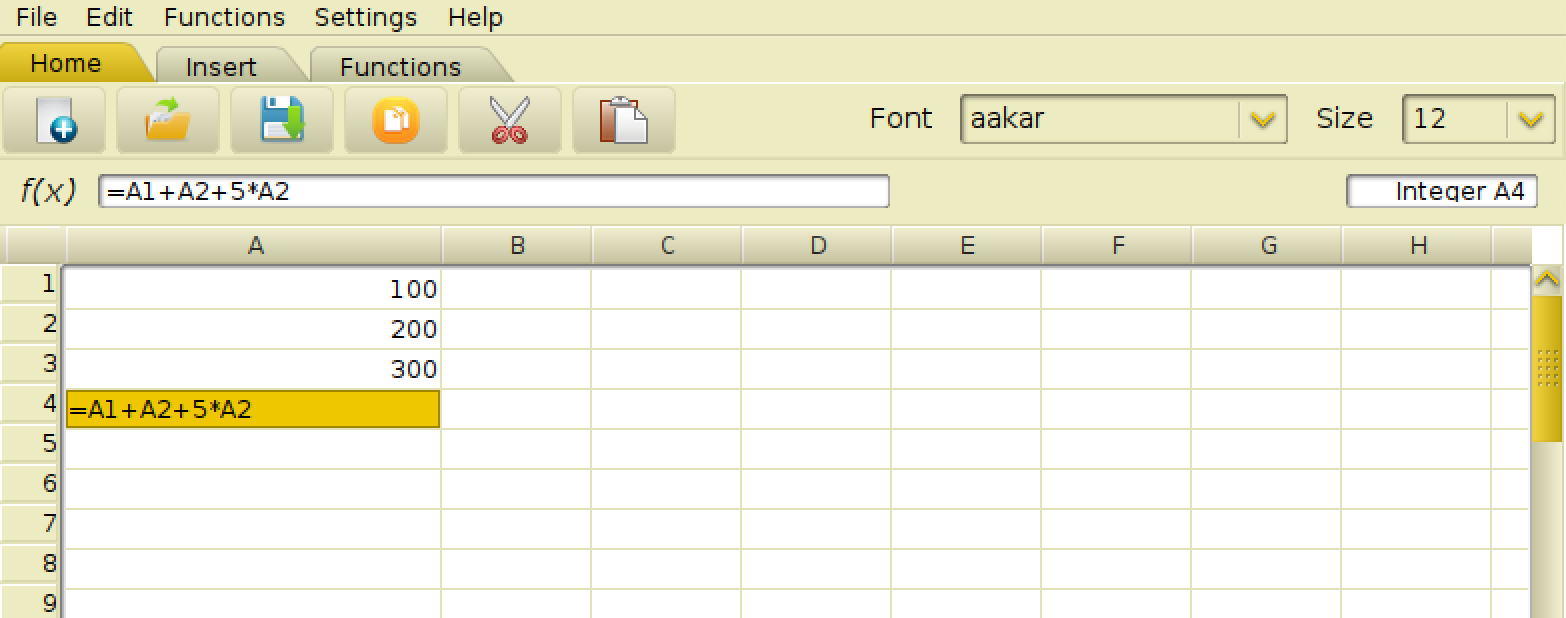
第二个任务单元类 (Second Task-Cell Class)
Being an apprentice my cell implementation was not perfect or appropriate. However, the ideal method is to do the implementation of a proper generics. If you’re new (like me back then) you can use strings with datatype in separate classes. Here’s how I’d organise the classes now;
作为学徒,我的单元实现并不完美或不合适。 但是,理想的方法是执行适当的泛型 。 如果您是新手(就像我一样),可以在单独的类中使用具有数据类型的字符串。 这是我现在组织课程的方式;
Cell<E> <- used to render cells, a generic class
AbstractDataType <- store shared data (user input string, etc)
DataType extends AbstractDataType <- store data (an example)
FloatType extends AbstractDataType <- floating point data storage
Here E could be the datatype of the cell found by the parser (Parser could return Class of the proper datatype in generics). We use inheritance to share the common attributes that any cell should have (user input string, cell colour if any and more that’ll discuss later). The AbstractDataType class demonstrates the abstraction. You will never create classes of AbstractDataType since it makes no sense.
这里E可能是解析器找到的单元格的数据类型(Parser可以返回泛型中适当数据类型的Class)。 我们使用继承来共享任何单元格应具有的公共属性(用户输入字符串,单元格颜色(如有)以及稍后将讨论的更多内容)。 AbstractDataType类演示了抽象 。 您将永远不会创建AbstractDataType类,因为它没有任何意义。
In our operations, we will be using AbstractDatatype dataT = new DataType()
which is polymorphism. This will enable us to add and subtract cells when cell referencing comes into play. All fields in the cells are Encapsulated and are only mutated using getters and setters.
在我们的操作中,我们将使用AbstractDatatype dataT = new DataType()
,它是多态的 。 这将使我们能够在单元格引用起作用时添加和减去单元格。 单元格中的所有字段都是封装的 ,仅使用getter和setter进行突变。
Now we have covered all the primary OOP concepts and on how to implement an Algorithm!
现在,我们已经涵盖了所有主要的OOP概念以及如何实现算法!
This wasn’t a simple task for any of the students who were quite new to programming, let alone JAVA.
对于任何一个刚开始编程的学生来说,这都不是一件容易的事,更不用说JAVA了。
第三任务用户界面 (Third Task-User Interface)
This is something that’s quite difficult to explain over a text. Hence I’ll share the look and feel of my implementation. However, NetBeans was a simple tool that enabled proper GUI implementation. I believe it is no longer used in practice since we have Electron/Qt like frameworks.
这是很难在文本上解释的东西。 因此,我将分享实现的外观。 但是,NetBeans是启用正确GUI实现的简单工具。 我相信,由于我们拥有类似Electron / Qt的框架,因此不再在实践中使用它。
JAVA has inbuilt GUI components and they are quite straightforward once you read the docs. Reading docs was an important skill which I believe I gathered from the very same course.
JAVA具有内置的GUI组件,一旦您阅读了文档,它们就非常简单。 阅读文档是一项重要技能,我相信我从同一门课程中学到的。
电子表格程序的关键UI组件 (The key UI component for the Spreadsheet program)
- The sheet view (Board of cells) 图纸视图(单元板)
- Menus for saving, copying, pasting, etc 保存,复制,粘贴等菜单
- Cell editor (Where you see the original equation or piece of text of the cell) 单元格编辑器(在其中看到单元格的原始方程式或一段文本)
- Active cell indicator (Shows which cell you have clicked on eg: A1) 活动单元格指示器(显示您单击的单元格,例如:A1)
Following was my GUI implementation. Note that I have used a theme (Apparently the only good theme that has proper rendering on non-windows platforms).
以下是我的GUI实现。 请注意,我使用了一个主题(显然,这是在非Windows平台上具有正确呈现的唯一好的主题)。
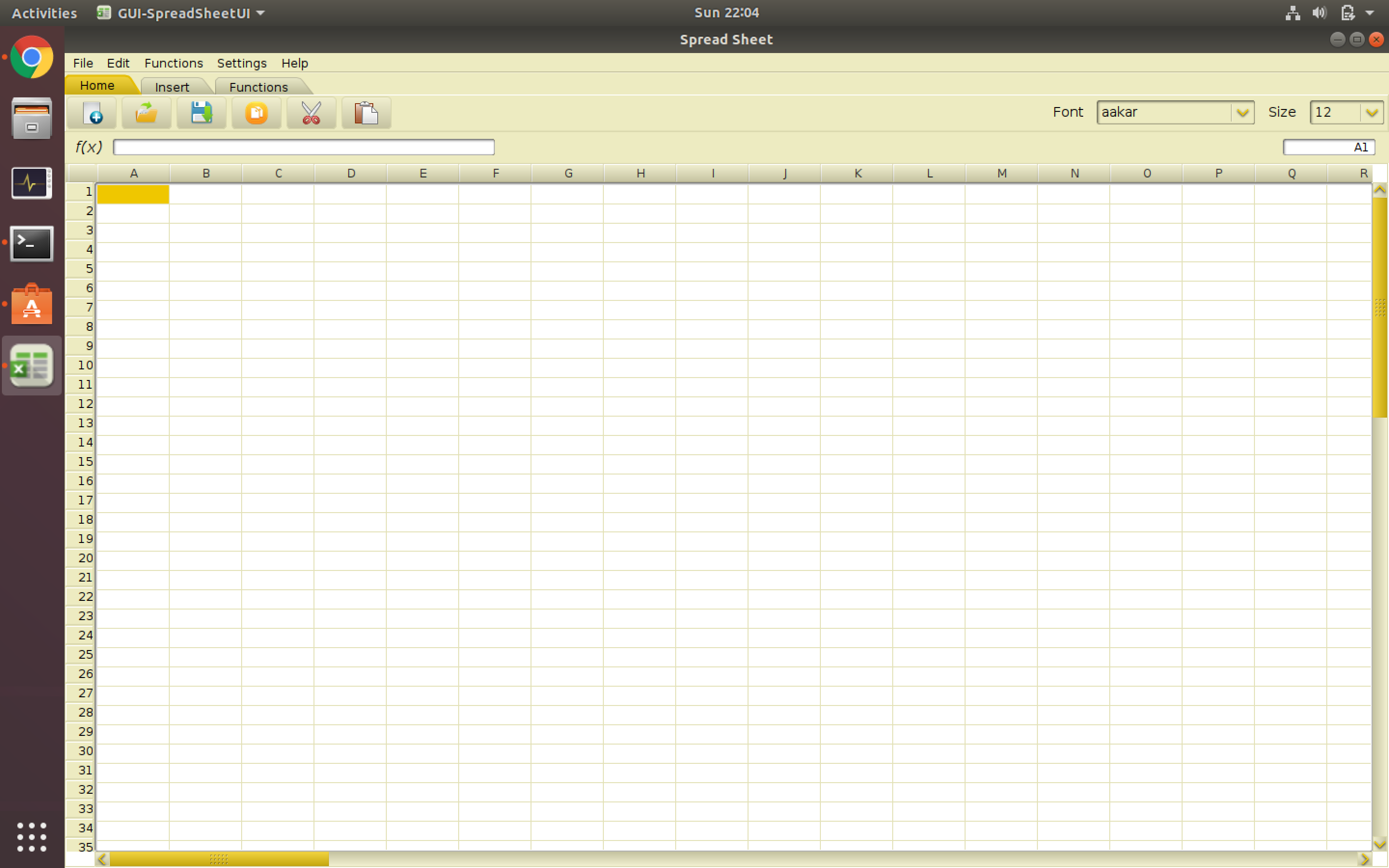
单元格引用 (Cell Referencing)
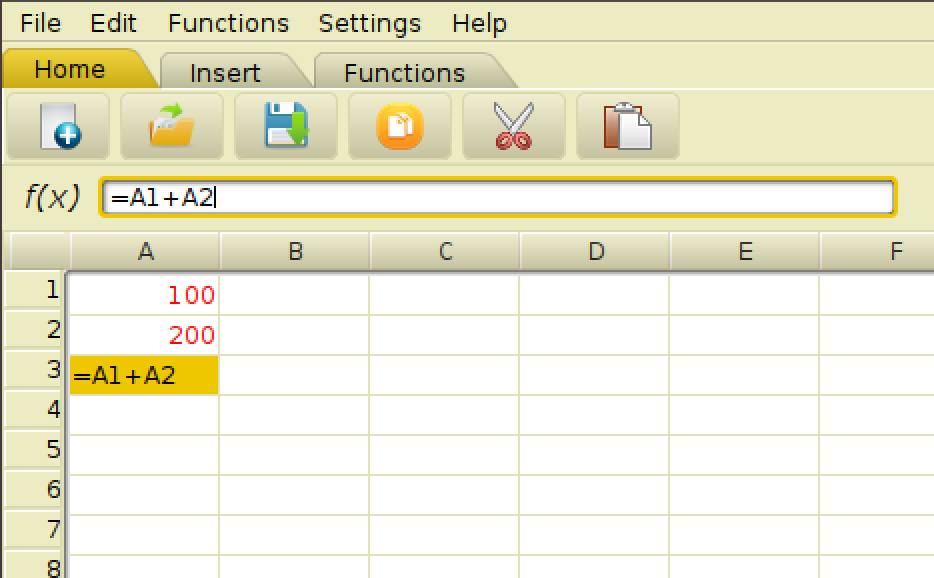
This was a feature I grabbed from the MS Excel program. Pretty neat feature!
这是我从MS Excel程序中获取的功能。 相当整齐的功能!
JAVA has many libraries that motivated us to go above and beyond for this particular project. I have added a few such screenshots to motivate my beloved readers and future engineers!
JAVA有许多库激励着我们超越这个特定项目。 我添加了一些这样的屏幕截图来激发我心爱的读者和未来的工程师!
绘图(无J图表库) (Graphing (J-free-chart Library))
JFreeChart library provides a wide array of graphing capabilities. I have used a few to make the program a bit elegant.
JFreeChart库提供了多种图形功能。 我使用了一些程序使程序变得更优雅。
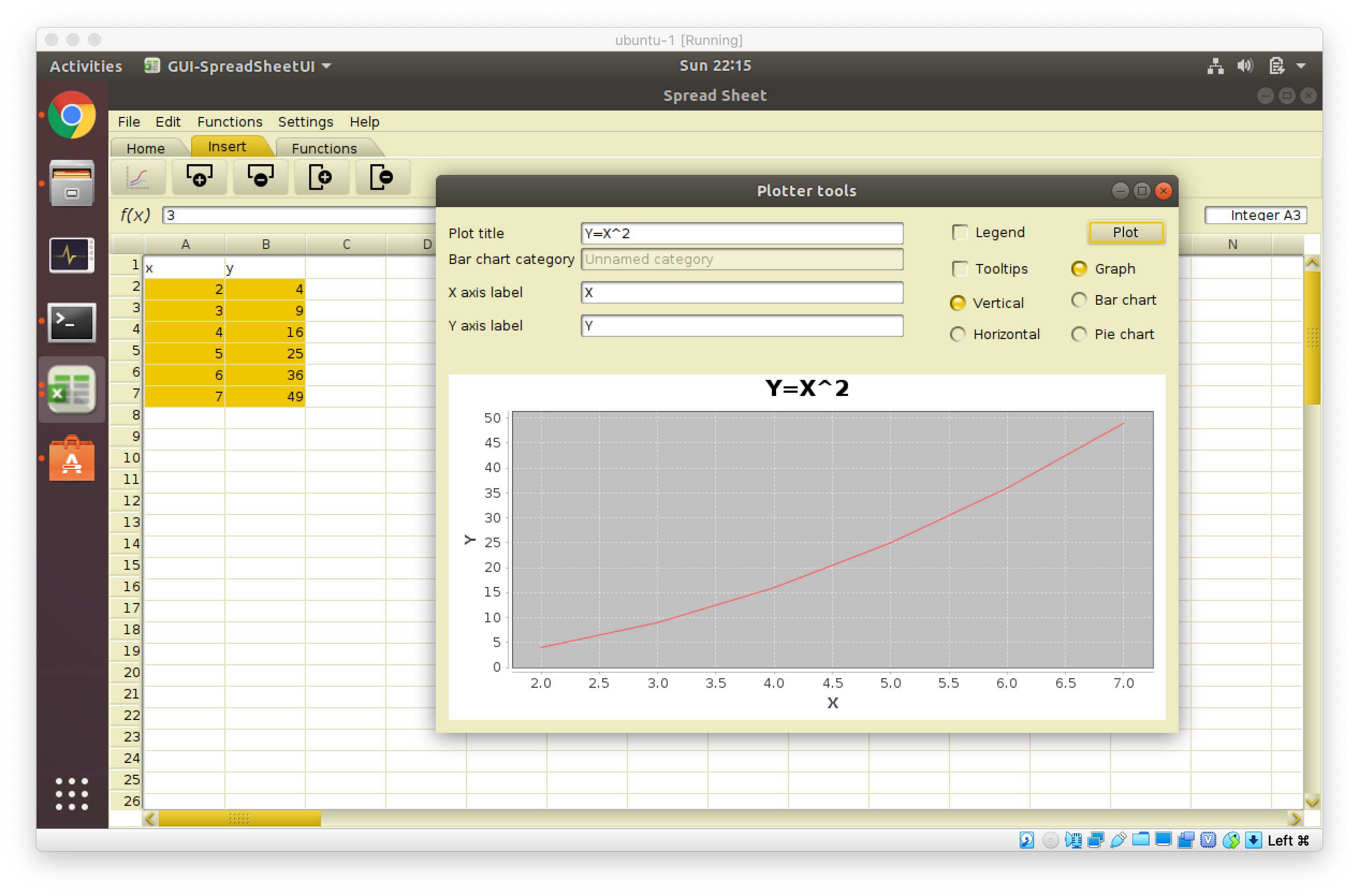
使用OpenCSV保存(序列化) (Saving (Serializing) with OpenCSV)
One of the most common ways of exporting spreadsheet data is using the CSV format. In this program, I used OpenCSV library to handle CSV file parsing.
导出电子表格数据的最常见方法之一是使用CSV格式。 在此程序中,我使用OpenCSV库处理CSV文件解析。
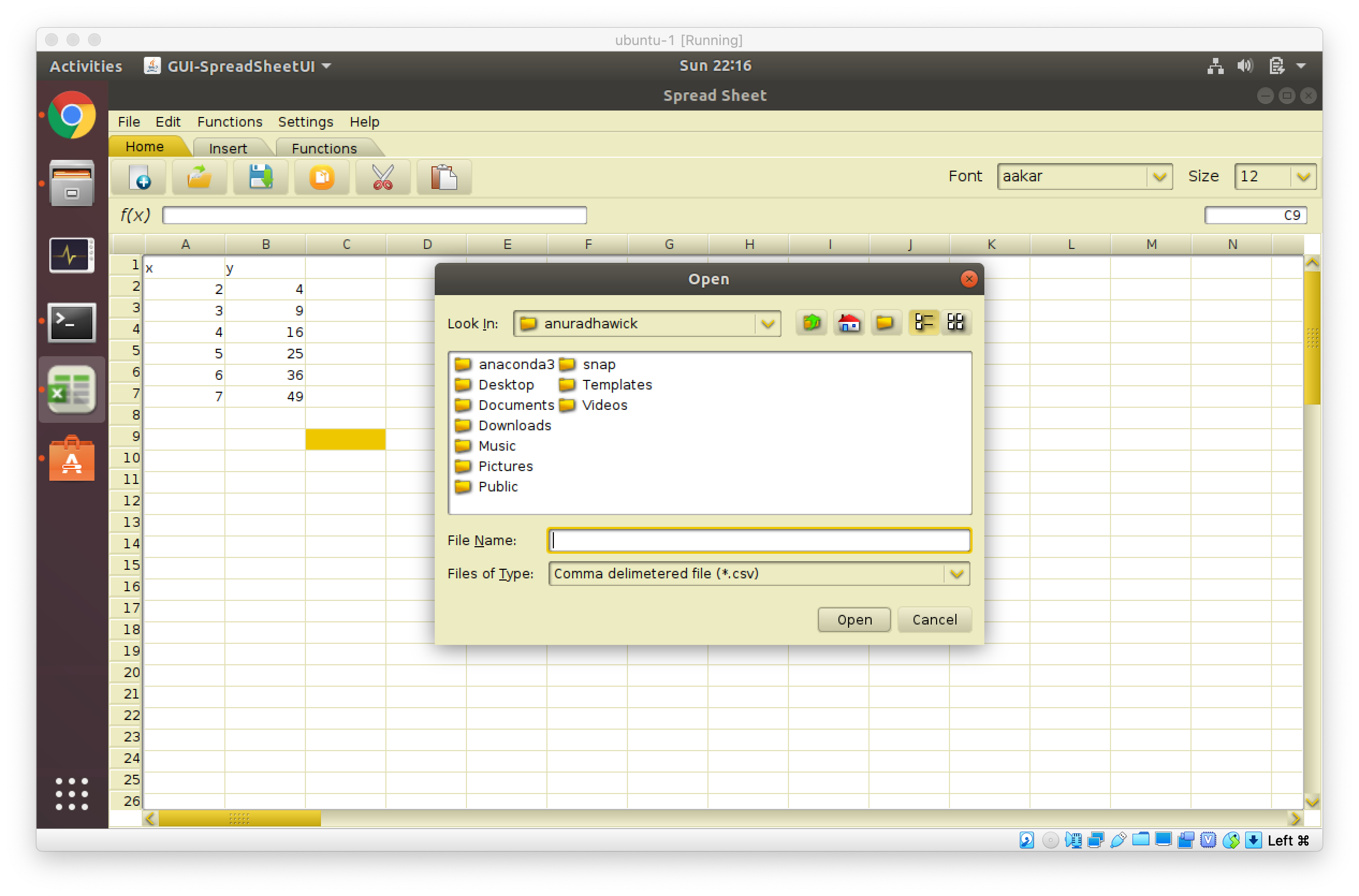
实现功能和有用的数据结构/设计模式 (Implementing Features and Useful Data Structures/Design Patterns)
单元格引用 (Cell Referencing)
This can be implemented in multiple ways.
这可以以多种方式实现。
Observer Design Pattern — Here the observers would be the cells that refer to other cells. We add such cells into the array of observing cells within the cells being observed. Changes are pushed as updates. Note that JAVA has an observable interface which makes the implementation sleek.
观察者设计模式 -这里的观察者将是引用其他单元的单元。 我们将此类细胞添加到正在观察的细胞内的观察细胞阵列中。 更改将作为更新推送。 请注意,JAVA有一个可观察的接口,使实现更加流畅。
Mediator Pattern — In this implementation, we have one cell coordinator that takes care of changes and updates. A HashMap object could be used to store possible referees and references.
中介器模式 —在此实现中,我们有一个单元协调器来处理更改和更新。 HashMap对象可用于存储可能的裁判和引用。
Special Notes — It is essential to look for cyclic dependencies. Otherwise, the program might hit with StackOverflows due to never-ending reference cycles.
特殊说明-寻找循环依赖关系至关重要。 否则,由于永无休止的引用周期,程序可能会遇到StackOverflows。
撤销重做 (Undo/Redo)
Though I did not implement this in my program, this can be achieved by using the Memento Design pattern. This could either be implemented as chained object references or as a stack of actions.
尽管我没有在程序中实现此功能,但可以使用Memento Design模式来实现。 这可以实现为链接的对象引用,也可以实现为动作堆栈。
对当代的最后思考 (Final Thoughts for Present Times)
This project was carried out before 6 years in 2014!. Since the times have made us move on from desktop GUIs, a web-based project would make more sense. Let me share a few projects that might help a budding software engineer to build confidence in programming.
该项目在2014年之前进行了6年! 由于时代已使我们从桌面GUI继续前进,因此基于Web的项目更有意义。 让我分享一些项目,这些项目可能会帮助刚起步的软件工程师树立对编程的信心。
- Library management program 图书馆管理程序
- A voting program for office use (for decision making) 办公室使用的投票程序(用于决策)
- A web-based baby monitor (for those who love to deal with image processing, etc) 基于Web的婴儿监视器(适用于喜欢处理图像处理等的人)
- A buying and selling program for some commodity (cars, homes, etc) 一些商品(汽车,房屋等)的买卖计划
Hope you enjoyed reading this. My sole thought of showing this project is to motivate young fellows to do a project through to its completion. Give it a go and share your project with me as well. Cheers!
希望您喜欢阅读本文。 我展示这个项目的唯一想法是激励年轻人完成一个项目。 快去与我分享您的项目。 干杯!
Give it a run!
放手吧!
git clone https://github.com/anuradhawick/SpreadSheet.git
cd SpreadSheet/dist/
java -jar SpreadSheet.jar
:)
:)
翻译自: https://towardsdatascience.com/how-to-have-a-solid-start-in-software-engineering-158dd9aa02c8
java扎实书籍