Building an API is a rewarding way to allow data to flow between apps and create compelling functionality in your latest project without having to start from scratch.
构建API是一种奖励方式,可让数据在应用程序之间流动,并在最新项目中创建引人注目的功能,而无需从头开始。
Newcomers to the world of APIs will definitely need a little advice and guidance to make sure that their fledgling efforts are a success. To that end, here are some essential tips to follow if you want to write APIs in Javascript.
API领域的新手肯定会需要一些建议和指导,以确保他们的起步努力是成功的。 为此,如果要使用Javascript编写API,请遵循以下一些基本技巧。
1.选择理想的框架 (1. Choose the perfect framework)
Once you have got a handle on the API definition and understand exactly what this tech can be used to achieve, you need to select a framework that makes sense for your needs.
掌握了API定义并准确了解了该技术可以实现的功能后,您需要选择一个适合您需求的框架。
To create a browser-based app, your best bet for building a Node.js RESTful API will be either Express or Koa. They can accommodate features for making life easier for end-users, as well as assisting with aspects like rendering.
要创建基于浏览器的应用,构建Node.js RESTful API的最佳选择是Express或Koa 。 它们可以容纳一些功能,以使最终用户的生活更加轻松,并在渲染等方面提供帮助。
If you are solely focused on API services in isolation, then Restify should be the framework at the top of the agenda. Popular platforms including Netflix are partly reliant on it, so it is a tried and tested option.
如果您仅专注于隔离的API服务 ,那么Restify应该是首要任务。 包括Netflix在内的流行平台在一定程度上依赖于它,因此它是一个久经考验的选择。
2.控制用户请求量 (2. Control user request volumes)
Performance can suffer if your API is bombarded by requests from a single user, so rate limiting is important to prevent this.
如果您的API受到来自单个用户的请求的攻击,则性能可能会受到影响,因此限制速率对于防止这种情况很重要。
You can use these headers to determine the number of requests remaining for a given user:
您可以使用以下标头来确定给定用户的剩余请求数:
X-Rate-Limit-Limit — Puts a cap on requests made within a specific timeframe.
X-Rate-Limit- Limit-对特定时间范围内的请求设置上限。
X-Rate-Limit-Remaining — marks the remaining number of requests available in a given span.
X-Rate-Limit-Remaining-标记给定跨度中可用的剩余请求数。
X-Rate-Limit-Reset — sets the point at which a reset of the rate limit will occur.
X-Rate-Limit-Reset ( X速率限制重设) —设置速率限制重设的点。
The good news is that the aforementioned frameworks will either let you use rate-limiting natively or through the use of commonly available plugins. One thing to keep in mind is that the timeframe can vary from provider to provider, ranging from a few minutes to an hour or more.
好消息是,上述框架将使您可以本机使用速率限制,也可以通过使用常用插件来使用。 要记住的一件事是,时间范围可能因提供商而异,从几分钟到一个小时甚至更长。
3.现有技能可能会有所帮助 (3. Existing skills can be helpful)
The nouns with which you are familiar from when you go to learn Javascript or harness basic HTTP can be applied in an API creation context. The main aspect to remember here is that resources are identified by nouns, and nothing more.
学习Java脚本或利用基本HTTP时熟悉的名词可以应用在API创建上下文中。 这里要记住的主要方面是资源是用名词标识的,仅此而已。
For example, if you wanted to build an API which manages user information, from adding them to an app to erasing their profiles, then these are some of the nouns you might want to use:
例如,如果您想构建一个用于管理用户信息的API,从将用户信息添加到应用程序到擦除其个人资料,那么以下是您可能要使用的一些名词:
Create fresh users with either POST /user or PUT /user:/id.
使用POST / user或PUT / user:/ id创建新用户。
Retrieve user information with GET /user or GET /user/:id.
使用GET / user或GET / user /:id检索用户信息。
Edit user data via PATCH /user/:id.
通过PATCH / user /:id编辑用户数据。
Erase a user using DELETE /user/:id.
使用DELETE / user /:id擦除用户。
Careful use of nouns such as POST, PUT, GET, PATCH and DELETE will allow you to apply existing skills to API building.
仔细使用诸如POST,PUT,GET,PATCH和DELETE之类的名词,您可以将现有技能应用于API的构建。
4.使用HTTP标头实现元数据的发送 (4. Sending metadata is achieved using HTTP headers)
HTTP headers can be harnessed to distribute data relating to everything from pagination and rate-limiting to authentication for security purposes.
为了安全起见,可以利用HTTP标头分发与从分页和速率限制到身份验证的所有内容有关的数据。
Custom metadata can be set within headers, just make sure to use a header name as a prefix which is unique to your app so that conflicts do not arise.
可以在标头中设置自定义元数据,只需确保将标头名称用作您的应用程序唯一的前缀,以免发生冲突。
Say that your app is called “HyperGas”, for example. You could use the prefix HyperGas in headers in the following ways:
假设您的应用程序名为“ HyperGas ”。 您可以通过以下方式在标头中使用前缀HyperGas:
HyperGas-Identity-Account-ID
HyperGas-Identity-Account-ID
HyperGas-Networking-Host-Name
HyperGas网络主机名
HyperGas-Object-Storage-Policy
HyperGas对象存储策略
Also, make sure that the headers you use do not exceed the 80KB limit that is currently imposed. This exists to counteract DDoS attacks, as well as to improve performance.
另外,请确保您使用的标头不超过当前强加的80KB限制 。 这是为了抵制DDoS攻击以及提高性能 。
5.利用状态码使您受益 (5. Leverage status codes to your advantage)
HTTP is your friend once again when it comes to status codes, so long as you set them in the standard way.
只要您以标准方式设置HTTP,HTTP就会再次成为您的朋友。
Here are some examples of codes that should be issued in response to a given error:
以下是一些应针对给定错误而发出的代码示例:
2xx when there are no complications.
2xx时无并发症。
3xx if the requested resource is not present in the indicated location.
如果请求的资源不在指定的位置,则为3xx 。
4xx if a client error is to blame for a failed request.
如果客户端错误归咎于失败的请求,则为4xx 。
5xx if the API is at fault.
如果API错误,则为5xx 。
If you have chosen Restify as your framework, an HTTP status code can be set like so:
如果您选择Restify作为框架,则可以这样设置HTTP状态代码:
res.status(201)
If Express is more your speed, then this is the code setting method of choice:
如果Express可以提高您的速度,则可以选择以下代码设置方法:
res.status(500).send({error: ‘Internal server error happened’}
6.适当测试您的API (6. Test your API appropriately)
To put your API through its paces once built, it makes sense to adopt a black-box approach to testing so that you know that it is resilient and robust.
为了使您的API能够在构建后立即步入正轨,有必要采用黑盒方法进行测试,以便您知道它具有弹性和健壮性。
There are modules, like supertest, intended to streamline this process, and with the help of test runner like mocha you can implement the following experiment:
有一些模块(例如supertest )旨在简化此过程,在像mocha的测试运行程序的帮助下,您可以实施以下实验:
const request = require(‘supertest’)
describe(‘GET /user/:id’, function() {
it(‘returns a user’, function() {
// newer mocha versions accepts promises as well
return request(app)
.get(‘/user’)
.set(‘Accept’, ‘application/json’)
.expect(200, {
id: ‘1’,
name: ‘John Doe’
}, done)
})
})
Tests should generally be formatted to avoid making guesses about the current configuration of the system in question, but it makes sense to add data to the target database specifically for the purposes of testing to help with these trial runs.
通常应该对测试进行格式化,以避免猜测有关系统的当前配置,但是将数据添加到目标数据库是有意义的,特别是为了进行测试以帮助这些试运行。
7.迎合其他用户 (7. Cater to other users)
You will want your API to be accessible and adaptable to other developers and coders, so aim to document its inner workings as you go along and distribute this information far and wide.
您将希望您的API可以被其他开发人员和编码人员访问并适应,因此请在进行操作时记录其内部工作情况,并广泛地分发此信息。
If you need help with this, a service like API Blueprint can be a great way of streamlining the process of writing documentation to go along with your API.
如果您需要帮助,可以使用API Blueprint之类的服务来简化与API一起编写文档的过程。
8.使HTTP请求成为条件 (8. Make HTTP requests conditional)
Taking advantage of conditional requests means that you can implement manipulations on the fly according to the HTTP header that has been used. This will give you greater control over the way that requests get processed.
利用条件请求意味着您可以根据已使用的HTTP标头即时执行操作。 这样可以更好地控制请求的处理方式。
The headers themselves can determine the outcome based on factors like the last time the data was modified. Here are the headers to deploy:
标头本身可以根据诸如上次修改数据之类的因素确定结果。 以下是要部署的标头:
Last-Modified — this specified the point at which a change was made.
Last-Modified ( 上次修改时间) -这指定了进行更改的时间点。
Etag — signifying the entity tag.
Etag —表示实体标签。
If-Modified-Since — can be combined with the first header in this list.
If-Modified-Since-可以与该列表中的第一个标头结合使用。
If-None-Match — usually paired with the entity tag.
If-None-Match-通常与实体标签配对。
9.应该使用使用JWT的无状态身份验证 (9. Stateless authentication using JWT should be used)
A JSON Web Token (JWT) can be used for the purposes of authentication since it matches the need for REST APIs to be stateless. JWTs need a header, payload and signature to provide authentication, although bear in mind that no encryption is involved.
JSON Web令牌 ( JWT )可用于身份验证,因为它符合REST API无状态的要求。 JWT需要标头,有效负载和签名来提供身份验证,尽管要记住,不涉及加密。
Here is how to add this kind of authentication when building an API:
以下是在构建API时如何添加这种身份验证的方法:
const koa = require(‘koa’)
const jwt = require(‘koa-jwt’)
const app = koa()
app.use(jwt({
secret: ‘secret-key’
}))
// Protected middleware
app.use(function *(){
// content of the token will be available on this.state.user
this.body = {
secret: ‘21’
}
})
You will also need to make sure that the token is provided as part of the Authorization header, like so:
您还需要确保令牌是作为Authorization标头的一部分提供的,如下所示:
curl — header “Authorization: Bearer eyJhbGciOiRWOSzI1NiIsInR5cC64IkpXVCJ9.eyJzqWIiOiIxZjM0NTY1ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYsqaW4iOnRydWV9.TJVA95OrM7E2cBab30RMHrHDcEfxjoYZgeUYGQh7HgQ” example.com
Endeavor to use HTTPS to ensure secure connectivity is established between all API endpoints, in addition to using this authentication mode.
除了使用此身份验证模式外,还要努力使用HTTPS来确保在所有API端点之间建立安全连接。
10.紧跟API开发 (10. Stay on top of API developments)
Perhaps most important of all it makes sense to keep your ear to the ground and ensure that you are looped into the news and developments that are shaping APIs at the moment. Fresh query languages like Facebook’s GraphQL and Netflix’s Falcor that have emerged recently are an example of why this is important.
也许最重要的是,保持警惕,并确保您了解当前正在形成API的新闻和开发。 最近出现的新鲜查询语言,例如Facebook的GraphQL和Netflix的Falcor,就是为什么这很重要的一个例子。
结论 (Conclusion)
With hard work and a dash of luck, your API building endeavors should be fruitful and fulfilling. You need to be prepared to learn from experience as well as by paying attention to the input of seasoned experts in this arena.
经过艰苦的工作和一点运气,您的API构建工作应该是富有成果和充实的。 您需要准备从经验中学习,也要注意该领域经验丰富的专家的意见。
使用Bit共享和管理可重用JS组件 (Share & Manage Reusable JS Components with Bit)
Use Bit (Github) to share, document, and manage reusable components from different projects. It’s a great way to increase code reuse, speed up development, and build apps that scale.
使用Bit ( Github )共享,记录和管理来自不同项目的可重用组件。 这是增加代码重用,加速开发并构建可扩展应用程序的好方法。
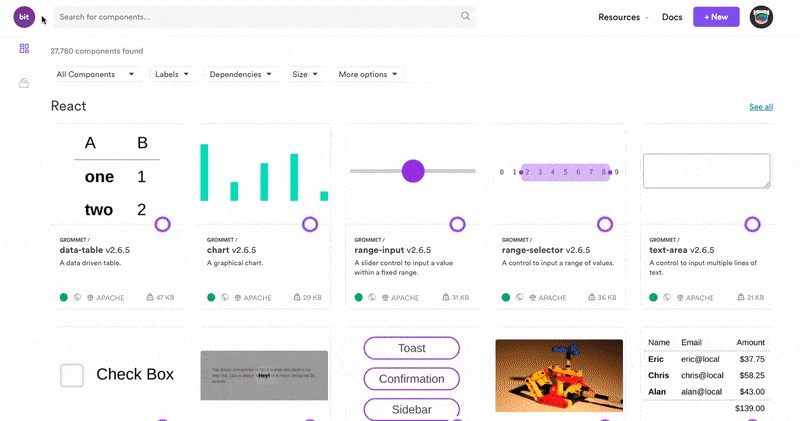
翻译自: https://blog.bitsrc.io/10-tips-for-building-an-api-9ec335601d59