swagger生成api
Do you have an application landscape with different clients that have different responsibilities? For instance, you have a web page and an app with different users and different access rights to your API. If you happen to have very many different calls, it gets tricky for the developers to keep track of which call is meant for which application and users. In this article, I present my solution that helped the developers in my team a lot.
您是否有一个具有不同职责的不同客户的应用程序环境? 例如,您有一个网页和一个应用程序,它们具有不同的用户和对API的不同访问权限。 如果碰巧有很多不同的调用,那么开发人员就很难跟踪哪个调用是针对哪个应用程序和用户的。 在本文中,我介绍了我的解决方案,该解决方案对团队中的开发人员有很大帮助。
In case you are a bit lost and/or didn’t read my previous article about Generating Swagger Code for your flutter app, I described how to set up Swagger in your AspNet Core application to easily generate the client code for your flutter app. Of course, you can substitute the backend or the client technology with virtually anything you want.
万一您迷路了和/或没有阅读我以前的有关为 Flutter应用程序生成Swagger代码的文章,我描述了如何在AspNet Core应用程序中设置Swagger,以轻松地为您的Flutter应用程序生成客户端代码。 当然,您可以用几乎任何您想要的东西替代后端或客户端技术。
为什么我要拆分我的API? (Why exactly would I want to split my API?)
Let’s say, we have a large backend application that offers a collection of services for several different client types (mobile apps, desktop apps, websites, …). It is most likely that not every offered call is meant for every client. Now, if you generate your Client API code using the swagger definition, it would make sense to only generate the client API code for the calls that are actually needed. Reducing the swagger definition to only the required calls has a lot of advantages:
假设我们有一个大型的后端应用程序,它为几种不同的客户端类型(移动应用程序,桌面应用程序,网站等)提供服务集合。 很有可能不是每个提供的呼叫都针对每个客户。 现在,如果您使用swagger定义生成客户端API代码,则仅为实际需要的调用生成客户端API代码就很有意义。 将swagger定义减少为仅所需的调用具有很多优点:
- We get rid of unnecessary boilerplate in the client code. 我们摆脱了客户端代码中不必要的样板。
- It reduces app size. 它减小了应用程序的大小。
- It accelerates client app programming because developers do not have to crawl through unsupported APIs. 由于开发人员不必通过不受支持的API进行爬网,因此可以加快客户端应用程序编程的速度。
- By only providing allowed APIs, it actually improves data security because developers are less likely to use incorrect calls that transfer unnecessary data. Obviously, it does not replace a proper rights & roles or data security concept. 通过仅提供允许的API,它实际上提高了数据安全性,因为开发人员不太可能使用不正确的调用来传输不必要的数据。 显然,它不能代替适当的权利和角色或数据安全性概念。
Is there a catch? Obviously, you have to make sure that the definitions are always up to date. Otherwise, the generated code does not contain the required APIs, some parameters might be outdated, and so on. Additionally, if you release new API versions frequently, my approach might not scale so well due to the generated overhead — depending on your project and API size.
有收获吗? 显然,您必须确保定义始终是最新的。 否则,生成的代码将不包含必需的API,某些参数可能已过时,等等。 此外,如果您频繁发布新的API版本,由于产生的开销,我的方法可能无法很好地扩展-取决于您的项目和API的大小。
开始之前的一点免责声明 (A little disclaimer before we start)
The solution I present here is, to be honest, not perfect, and actually exploits a feature that was meant to be used differently. However, the functionality I am looking for is not supported — at least not to my knowledge and research. On top of that: Different solutions are available and I will briefly look into them in order to draw a complete picture for you of why I personally went for this specific solution.
老实说,我在这里提出的解决方案并不完美,实际上是利用了原本打算用于不同用途的功能。 但是,我所寻找的功能不受支持-至少在我的知识和研究范围内。 最重要的是:有不同的解决方案可用,我将简要地研究它们,以便为您绘制一张完整的图片,说明我亲自选择该特定解决方案的原因。
Let's get into it.
让我们开始吧。
In my backend projects, I don’t introduce too many different API versions. From time to time a new API version comes up, which means that I introduce version 3 next to version 2, and sometimes there is still a deprecated version 1. So most of the time, there is nothing fancy going on. AspNet Core and Swagger API versioning, on the other hand, support declaring minor version numbers, which I rarely see being used. In the sample Github project and multiple professional projects, I exploit the minor version feature to split my API. For instance, API version 2.0 includes all routes tagged with version 2, while only a portion of the API (which I require for a specific client) is also mapped to 2.1, or alternatively, explicitly excluded. If there are even more applications, they will be mapped to 2.2, 2.3, and so on.
在我的后端项目中,我没有介绍太多不同的API版本。 有时会出现一个新的API版本,这意味着我在版本2之后介绍了版本3,有时仍然不推荐使用版本1。因此,在大多数情况下,没有任何幻想。 另一方面,AspNet Core和Swagger API版本控制支持声明次要版本号,我很少看到使用它。 在示例Github项目和多个专业项目中,我利用次要版本功能拆分了我的API。 例如,API版本2.0包含标记有版本2的所有路由,而仅API的一部分(我需要特定的客户端)也映射到2.1,或者明确地排除在外。 如果有更多的应用程序,它们将被映射到2.2、2.3等。
The Swagger UI for the Flutter client and, respectively, the Flutter Swagger definitions will not include the Delete operation.
Flutter客户端的Swagger UI和Flutter Swagger定义将分别不包含Delete操作。
But what if you are already using minor versions in your projects? Even if you are using minor versions, you could reserve certain numbers for specific topics. Something you will never ever reach — depending on your project you can define some constants like:
但是,如果您已经在项目中使用了次要版本,该怎么办? 即使您使用的是次要版本,也可以为特定主题保留一定数量。 您永远无法达到的目标-根据您的项目,您可以定义一些常量,例如:
For my approach to work, it is necessary to adjust Swagger’s version pattern from “‘v’V” to “‘v’VV”. A single capital “V” only allows us to introduce new major versions. In order to have an additional version within your major version, you would have to add minor versions to be included as well. Respectively, if you are already working with minor versions, you can try to add a third version parameter and use the status to split the API. However, AspNetCore’s API Versioning is not exactly supporting this out of the box and you will probably need some complex refactoring or a different API versioning framework.
对于我的工作方式,有必要将Swagger的版本模式从“'v'V”调整为“'v'VV”。 单个大写的“ V”仅允许我们引入新的主要版本。 为了在主版本中包含其他版本,您还必须添加次要版本。 分别,如果您已经在使用次要版本,则可以尝试添加第三个版本参数,并使用状态来拆分API。 但是,AspNetCore的API版本控制并不能完全支持此功能,您可能需要进行一些复杂的重构或使用其他API版本控制框架。
What this does is to break the API into multiple smaller APIs that exist next to the original API. But since we do not introduce any new controllers or anything like that, it really is just another route mapping to the already existing controllers. The following image illustrates this behavior.
这样做是为了将API分成原始API旁边存在的多个较小的API。 但是,由于我们没有引入任何新的控制器或类似的东西,它实际上只是映射到现有控制器的另一条路由。 下图说明了此行为。
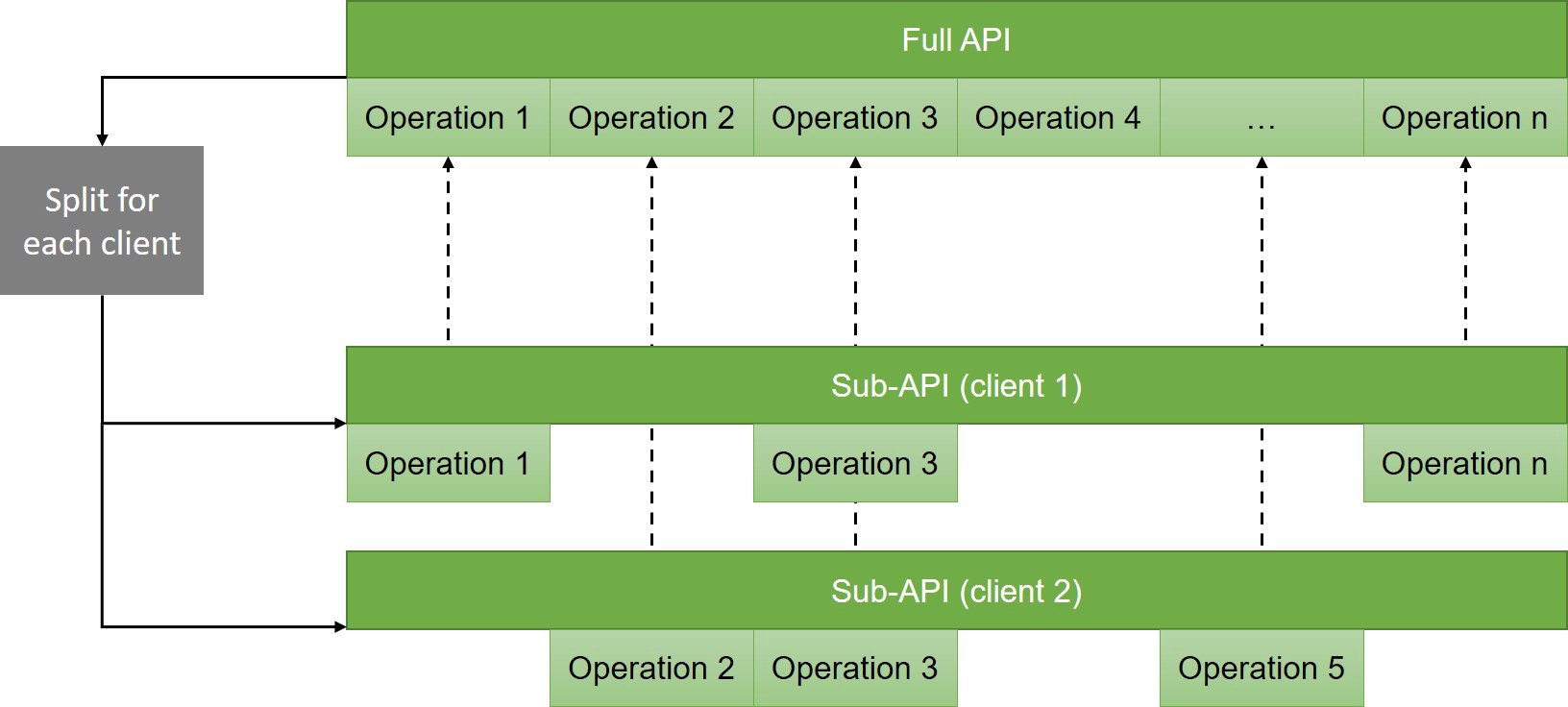
It is totally possible to customize the swagger page for each “Sub-API”. In the following example, I provide a custom description that explains what it is used for. This is how you can provide a custom name for the dropdown in the swagger UI:
完全可以为每个“ Sub-API”自定义页面。 在下面的示例中,我提供了一个自定义描述,说明了它的用途。 这是您可以在招摇的UI中为下拉菜单提供自定义名称的方法:
And this small sample illustrates how you can adjust the description for a specific client API:
这个小样本说明了如何调整特定客户端API的描述:
检索规范文件需要做什么? (What needs to be done to retrieve the specification files?)
Actually, you can have your developers download the files directly from the Swagger UI.
实际上,您可以让开发人员直接从Swagger UI下载文件。
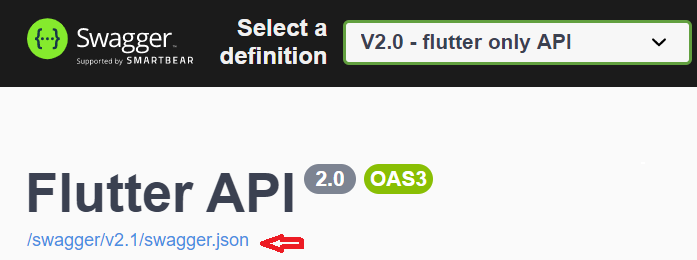
After downloading the specific file, the swagger API code can be generated according to the guidelines provided in my article Generating Swagger Code for your flutter app. But it can already be useful even if you do not use it for API code generation because it tells your developers which calls are supposed to be used by them.
下载特定文件后,可以根据我的文章“为您的flutter应用程序生成Swagger代码”中提供的准则生成swagger API代码。 但是,即使您不将其用于API代码生成,它也已经很有用,因为它会告诉开发人员应该由他们使用哪些调用。
您谈论的替代方案呢? (What about the alternatives you talked about?)
While I was looking for possible solutions I stumbled upon a few promising solutions that unfortunately came with some kind of drawback.
在寻找可能的解决方案时,我偶然发现了一些有前途的解决方案,但不幸的是存在一些缺陷。
Initially, I was hoping for support using the Swashbuckle Swagger package or the Swagger-api codegen feature. I looked into somehow assigning custom tags to the operations. However, this would result in two problems. Firstly, the Swagger UI groups operations by tags, which means the Swagger UI would no longer be as useful as it is now (e.g. grouping by controller). Secondly, it would require manual manipulation of the swagger definition. The codegen configuration does not support an out-of-the-box filtering approach — and even if it did — it would be a hell of a configuration effort. However, if this is what you want, you could e.g. look into forking the codegen project and provide a configuration that takes a configuration file as a parameter that contains a list of operations.
最初,我希望使用Swashbuckle Swagger软件包或Swagger-api代码生成功能获得支持。 我研究了如何为操作分配自定义标签。 但是,这将导致两个问题。 首先,Swagger UI 通过标签对操作进行分组,这意味着Swagger UI将不再像现在那样有用(例如,按控制器分组)。 其次,这需要对摇摇欲坠的定义进行手动操作。 代码生成配置不支持即用型过滤方法,即使支持,也是如此。 但是,如果这是您想要的,则可以例如研究派生codegen项目并提供一个配置,该配置将配置文件作为包含操作列表的参数。
I found an API splitter tool that post-processes the output and splits the swagger definition into multiple smaller files. This is basically what we want but the tool appears to be very old and I honestly never bothered to check if it supports the new OpenAPI Specification format. Mostly because I disliked the idea of becoming dependent on a seemingly abandoned third-party tool. Furthermore, this tool is not providing multiple swagger definitions but really just splits one working definition into multiple files. This means that a single file cannot be used for Swagger API code generation because the dependencies (e.g. models) cannot be resolved and the meta-information is not present.
我找到了一个API拆分器工具 ,该工具可以对输出进行后处理,并将swagger定义拆分为多个较小的文件。 基本上这就是我们想要的,但是该工具似乎已经很旧了,老实说,我从来没有费心检查它是否支持新的OpenAPI规范格式。 主要是因为我不喜欢依赖看似被抛弃的第三方工具的想法。 此外,该工具不提供多种定义,而是仅将一个工作定义拆分为多个文件。 这意味着不能将单个文件用于Swagger API代码生成,因为无法解析依赖性(例如模型)并且不存在元信息。
A full example is available in my sample GitHub project. Feel free to comment below if you have any remaining questions. If you liked this content or if you want more content like this, I would appreciate it if you have a look here.
我的示例GitHub项目中提供了完整的示例。 如果您还有其他疑问,请在下面发表评论。 如果您喜欢这些内容,或者想要更多类似的内容,请在此处查看 。
swagger生成api