一、需求
在我们程序中,需要同时跑多个脚本程序。
比如以下两个脚本:
-- 1.lua
while true do
print("1: trun on led")
wait_sec(15)
print("1: trun off led")
wait_sec(10)
end
-- 2.lua
count = 0
while true do
print('2: count = ' .. count)
count = count + 1
wait_sec(5)
end
我希望这两个脚本可以在我们的程序中同时运行。然而,我们的程序是基于事件驱动的单线程编程模式。所以,我们不希望为每一个Lua脚本的运行开一个独立的线程。
说明:作者的Lua版本是 5.4。下面的例子在不同的版本上,可能会有不同。
二、思路
之前没有找到合适的方式,打开使用我们自己的协程模块实现上述功能。
为每一个脚本创建一个协程,在我们注册的回调函数中使用 schedule->yield() 切换协程。
后面在网上找到 lua 自带的协程可以被使用。
lua_resume() 与 lua_yield()
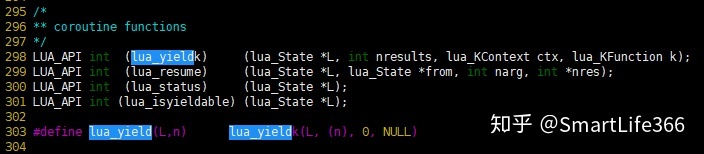
lua_resume 的参数说明:
- L,表示要恢复的协程
- from,表示是哪个协程在 resume 它。如果没有,否填NULL
- narg,表示在栈中压了多少个参数
- nres,表示再次从 lua_resume() 返回时,协程通过 co.yield() 传了多少个参数
lua_yieldk 的参数说明:
- L,表示是哪个协程要切出
- nresults,表示 yield() 传了多少个参数进去
- ctx,继续执行的上下文
- k,继续执行的函数
关于 ctx 与 k 的原因,见: Lua 5.2 如何实现 C 调用中的 Continuation
Lua手册:https://www.lua.org/manual/5.3/manual.html
三、实现
3.1 参考网上demo
Demo例子:
//! main.c
#include <stdio.h>
#include <lua.h>
#include <lualib.h>
#include <lauxlib.h>
static int Stop(lua_State* L);
lua_State* CreateCoroutine(lua_State* gL, const char* corName);
int main()
{
lua_State *L = luaL_newstate();
luaL_openlibs(L);
luaL_dofile(L,"corTest.lua");
lua_State* newL = CreateCoroutine(L,"CorT