Assume that you have basic knowledge on php, backbone.js, underscore.js and jquery.
We will use Firefox browser with firebug to test.
Actually here we will learn how to use model, view and router of backbone.js and fetch data from server using php.
First of all we will create directory structure.
1
2
3
4
5
6
7
|
|-> php-backbone
|-> js
|-> jquery-2.0.3.min.js
|-> underscore-min.js
|-> backbone-min.js
|-> api
|-> example_1
|
create above directory structure in you web_root. and put those js files in js directory (those will be found in source, link at bottom or you can get those by google).
now we will create a Boilerplate in example_1 directory named “index.html”, so create a new index.html file under example_1 directory and write below code in this file.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
<!DOCTYPEhtml>
<html>
<head>
<title>php backbone.js example 1</title>
</head>
<body>
<!-- JavaScript -->
<scriptsrc="../js/jquery-2.0.3.min.js"></script>
<scriptsrc="../js/underscore-min.js"></script>
<scriptsrc="../js/backbone-min.js"></script>
</body>
</html>
|
Backbone’s only hard dependency is Underscore.js ( >= 1.5.0). For RESTful persistence, history support via Backbone.Router and DOM manipulation with Backbone.View, include jQuery, and json2.js for older Internet Explorer support. (Mimics of the Underscore and jQuery APIs, such as Lo-Dash and Zepto, will also tend to work, with varying degrees of compatibility.)
Now create an new example_1.js file in js directory, and include it at bottom of all script to our Boilerplate index.html file, then our index.html file will look like below.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
<!DOCTYPEhtml>
<html>
<head>
<title>php backbone.js example 1</title>
</head>
<body>
<!-- JavaScript -->
<scriptsrc="../js/jquery-2.0.3.min.js"></script>
<scriptsrc="../js/underscore-min.js"></script>
<scriptsrc="../js/backbone-min.js"></script>
<scriptsrc="../js/example_1.js"></script>
</body>
</html>
|
and open it with you favorite editor.
In example_1.js, we will first create a “Message” model, and add a default property called “message” like below.
1
2
3
4
5
|
varMessageModel = Backbone.Model.extend({
defaults: {
message:"Text Message"
}
});
|
Now we will access our example_1 to test that is our model working or not. so urlhttp://localhost/php-backbone/example_1/ on FireFox (this url for mine as i follow above directory structure so your url can not be like it, so use your url to access example_1). Open Firebug console, create a object of MessageModel and access its message property like below.
1
2
|
varmsg =newMessageModel();
msg.get('message');
|
run this and will get output like below screenshot.
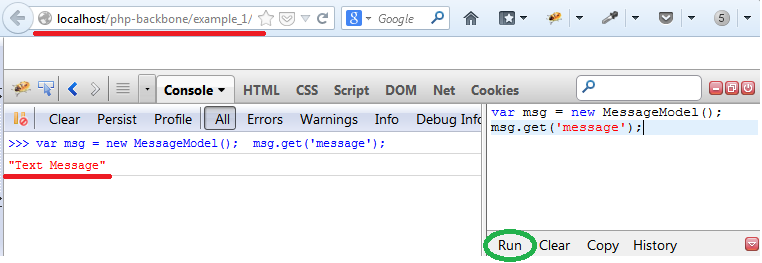
model default property access
After above model build backbone router like below.
1
2
3
4
5
6
7
8
9
10
11
12
|
varMessageRouter = Backbone.Router.extend({
routes:{
"":"displayMessage"
},
displayMessage:function() {
varmessageModel =newMessageModel();
console.log(messageModel.get("message"));
}
});
varmessageRouter =newMessageRouter();
Backbone.history.start();
|
here we build a router and added a route and assigned a function “displayMessage”, in this function we created a model object for “MessageModel” and print its “message” property in console.
to execute this router we created object of this router. then we start backbone history to ask backbone to monitoring hashchange events, and dispatching routes.
Now if you access this example_1 from browser then you will see output “Text Message” on firebug console.
To create View, first we need to change our index.html under example_1 directory.
in body tag, we will add a div tag in which we will display our message, and a template which will use to contain message text. so add below html come in body tag before script tags.
1
2
3
4
5
6
|
<divid="msg"></div>
<!-- Templates -->
<scripttype="text/template"id="tpl-hello-backbone">
<%= message %>
</script>
|
here in template “<%= … %>” used to interpolate variable message.
So our template is ready, now we will create backbone view. add below code in example_1.js after model code.
1
2
3
4
5
6
7
8
9
|
varMessageView = Backbone.View.extend({
template:_.template($('#tpl-hello-backbone').html()),
render:function(eventName) {
$(this.el).html(this.template(this.model.toJSON()));
returnthis;
}
});
|
here we assigned javascript template and created render function which used to render the view template from model data.
Now we have to call this render function from router function “displayMessage”. change this function as below.
1
2
3
4
5
|
displayMessage:function() {
varmessageModel =newMessageModel();
varmessageView =newMessageView({model:messageModel});
$('#msg').html(messageView.render().el);
}
|
here we created object of MessageView with our messageModel. and called view’s render function and put its output in our “#msg” div. there “el” is a DOM element which created from the view’s tagName, className, id and attributes properties, if specified. If not, el is an empty div.
Now if you access example_1 then you will see “Text Message” in browser.
Above we completed below steps::
1) Build directory structure
2) Created Boilerplate for backbone.js
3) Created backbone.js model
4) Created backbone.js router
5) Created backbone.js view
Now its time to get data from server (using php) and display it, we have already completed most of work, now we will just fetch data from server using our MessageModel.
Create “example_1.php” file in api directory and write one code in it like below.
1
2
|
<?php
echo'{"message":"Hello Backbonejs"}';
|
here we just echo a string followed by json structure.
Then we need modify our model little bit that just add “urlRoot” property with vlaue “../api/example_1.php”, so change our model like below.
1
2
3
4
5
6
|
varMessageModel = Backbone.Model.extend({
urlRoot :'../api/example_1.php',
defaults: {
message:"Text Message"
}
});
|
Then change the “displayMessage” function in backbone router like below.
1
2
3
4
5
6
7
8
9
10
|
displayMessage:function() {
varmessageModel =newMessageModel();
varmessageView =newMessageView({model:messageModel});
messageModel.fetch({
success:function() {
$('#msg').html(messageView.render().el);
}
});
}
|
here we only use “messageModel.fetch()” function to collect data for this model from server. and after successful fetch we are calling render function. now if you access exmaple_1 then you can see output “Hello Backbonejs” in your browser.
if you can see this text then Congratulation :) , if not then you can post comment, we will fix it together.
Here you can find Second example of backbone.js and php.