Here you can find first example of backbone.js and php.
In this Example 2, we will improve Example 1 by:
- Use collection of backbone.js ( collection is ordered sets of models)
- Using mysql, PDO we will retrieve message list
So first of all create a new directory “example_2” in our project directory “php-backbone”, create a new html file “index.html” in this new “example_2” directory. and write following boilerplate html code in this new “index.html” file.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
<!DOCTYPEhtml>
<html>
<head>
<title>php backbone.js example 2</title>
</head>
<body>
<!-- JavaScript -->
<scriptsrc="../js/jquery-2.0.3.min.js"></script>
<scriptsrc="../js/underscore-min.js"></script>
<scriptsrc="../js/backbone-min.js"></script>
<scriptsrc="../js/example_2.js"></script>
</body>
</html>
|
We have already included “example_2.js” in our “example_2/index.html”, now we need to create this js file in “js” directory. So create “example_2.js” file in js directory.
Now add “MessageModel” model in this file like below
1
2
3
4
5
6
7
8
|
varMessageModel = Backbone.Model.extend({
defaults: {
code: 0,
message:"Test Message"
}
});
|
add “MessageRouter” router and create an object of this router and start history like below
1
2
3
4
5
6
7
8
9
10
11
12
13
|
varMessageRouter = Backbone.Router.extend({
routes: {
"":"displayMessages"
},
displayMessages:function() {
}
});
varmessageRouter =newMessageRouter();
Backbone.history.start();
|
now we have to check that our model and router are working well or not, in “displayMessages” function create object of model and log its message value.
1
2
3
4
|
displayMessages:function() {
varmsg =newMessageModel();
console.log(msg.toJSON());
}
|
It should display output “Test Message” browser console.
Now we will add backbone.js collection, it is just an ordered sets of models. so write code in “example_2.js” like below.
1
2
3
|
varMessageCollection = Backbone.Collection.extend({
model: MessageModel
});
|
To test this “MessageCollection”, we will create some object of “MessageModel” and pass the list of model to collection and use a underscore.js iterator “each” function to retrieve data of “MessageCollection”.
So modify the “displayMessages” function like below.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
displayMessages:function() {
varmsg1 =newMessageModel({ code:"001", message:"Registration Successfully "});
varmsg2 =newMessageModel({ code:"002", message:"Registration Failed"});
varmsg3 =newMessageModel({ code:"003", message:"Login Successfully"});
varmsg4 =newMessageModel({ code:"003", message:"Login Failed"});
varmessageCollection =newMessageCollection([ msg1, msg2, msg3, msg4]);
_.each(messageCollection.models,function(msg) {
console.log("code => "+ msg.get("code") +", message => "+ msg.get("message"));
});
}
|
Output in the console will like below.
code => 001, message => Registration Successfully
code => 002, message => Registration Failed
code => 003, message => Login Successfully
code => 003, message => Login Failed
Now we will work for view. so at first in “/example_2/index.html” we will add a div for contain message list and add a template for render each message item.
so add below code in index.html.
1
2
3
4
5
6
|
<divid="messageList"></div>
<!-- Templates -->
<scripttype="text/template"id="tpl-message-item">
<ahref="#"id="<%= code %>"><%= message %></a>
</script>
|
In “js/example_2.js” file we will create two views, one for message list and another for message item. So add below two views in this file.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
varMessageListView = Backbone.View.extend({
tagName:"ul",
render:function(eventName) {
_.each(this.model.models,function(msg) {
$(this.el).append(newMessageListItemView({model:msg}).render().el);
},this);
returnthis;
}
});
varMessageListItemView = Backbone.View.extend({
tagName:"li",
template:_.template($('#tpl-message-item').html()),
render:function(eventName) {
$(this.el).html(this.template(this.model.toJSON()));
returnthis;
}
});
|
And change the function “displayMessages” like below.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
displayMessages:function() {
varmsg1 =newMessageModel({ code:"001", message:"Registration Successfully "});
varmsg2 =newMessageModel({ code:"002", message:"Registration Failed"});
varmsg3 =newMessageModel({ code:"003", message:"Login Successfully"});
varmsg4 =newMessageModel({ code:"003", message:"Login Failed"});
varmessageCollection =newMessageCollection([msg1, msg2, msg3, msg4]);
varmessageListView =newMessageListView({model:messageCollection});
$('#messageList').html(messageListView.render().el);
}
|
From browser access “example_2”, then you will get output in our browser like below.
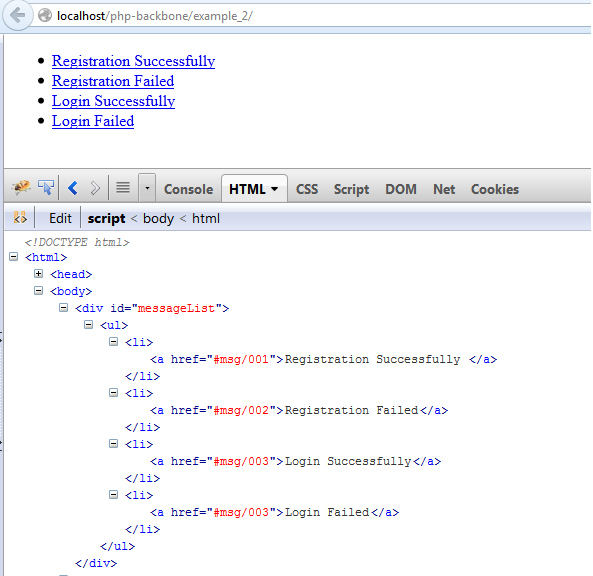
PHP Backbone Output
Now we have to a simple thing that retrieve message list from mysql database by php and display it, our model, controller, view and router all are all most ready. so in our mysql database create a new table and insert new data using below script.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
CREATETABLEIFNOTEXISTS `messages` (
`id`int(11)NOTNULLAUTO_INCREMENT,
`code`int(11)NOTNULL,
`message`varchar(255)NOTNULL,
PRIMARYKEY(`id`)
) ENGINE=InnoDB DEFAULTCHARSET=utf8 AUTO_INCREMENT=8;
INSERTINTO`messages` (`id`, `code`, `message`)VALUES
(1, 100,'Continue'),
(2, 101,'Switching Protocols'),
(3, 102,'Processing'),
(4, 200,'OK'),
(5, 201,'Created'),
(6, 203,'Non-Authoritative Information'),
(7, 204,'No Content');
|
You will also find this sql script with source “db/messages.sql”.
Then create a new php file “example_2.php” in api and write code like below.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
getMessages();
functiongetMessages() {
$sql="select * FROM messages ORDER BY code";
try{
$db= getConnection();
$stmt=$db->query($sql);
$messages=$stmt->fetchAll(PDO::FETCH_OBJ);
$db= null;
echojson_encode($messages);
}catch(PDOException$e) {
echo'{"error":{"text":'.$e->getMessage() .'}}';
}
}
functiongetConnection() {
$dbhost="localhost";// Your Host Name, For Me It localhost
$dbuser="root"; // User Name, For Me It root
$dbpass="root"; // Password, For Me It root
$dbname="test"; // Database Name, For Me It test
$dbh=newPDO("mysql:host=$dbhost;dbname=$dbname",$dbuser,$dbpass);
$dbh->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
return$dbh;
}
|
Change $dbhost, $dbuser, $dbpass, $dbname as yours.
In above “getConnection()” function, its simple, we just connect to the database and return the handler using PDO.
In “getMessages” function we just retrieve messages as object and return the json result set.
In example_2.js we have to add the url to the Collection class “MessageCollection ” like below.
1
2
3
4
5
6
|
varMessageCollection = Backbone.Collection.extend({
model: MessageModel,
url:"../api/example_2.php"
});
|
Now we have to fetch messages, So change the “displayMessages” function like below.
1
2
3
4
5
6
7
8
9
10
11
|
displayMessages:function() {
varmessageCollection =newMessageCollection();
varmessageListView =newMessageListView({model:messageCollection});
messageCollection.fetch({
success:function() {
$('#messageList').html(messageListView.render().el);
}
});
}
|
So if you access this example “example_2” then we will get output like below.
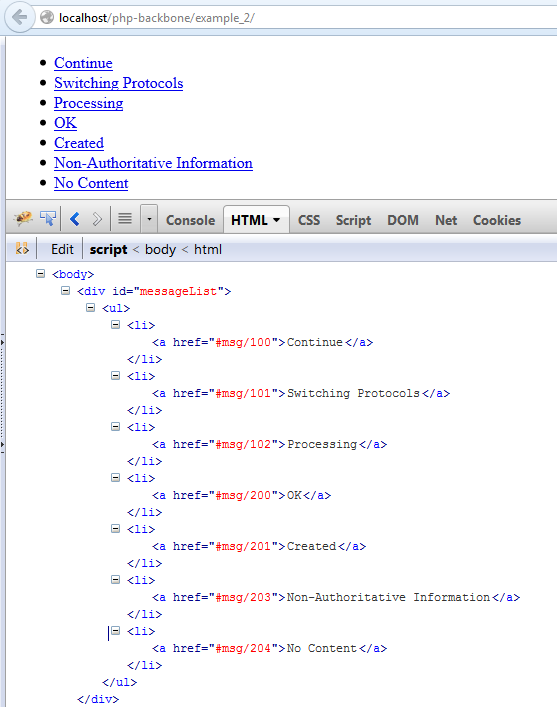
PHP Backbone Output
If you have any question, feel free to ask in comment.