纯CSS3实现
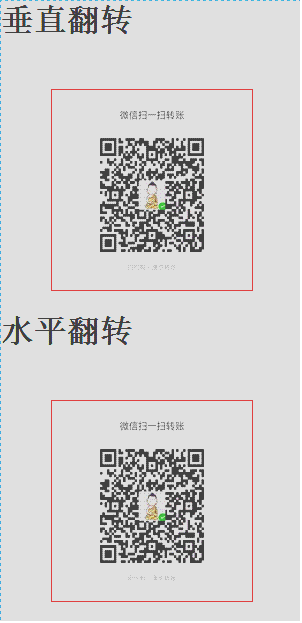
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
<style>
a {
display: block;
float: left;
margin: 10px 50px 10px 50px;
color: red;
border: 1px solid blue;
}
.a {
transition: All 1s ease-in-out;
width: 200px;
height: 200px;
background-color: #000000;
display: block;
text-align: center;
line-height: 200px;
font-size: 20px;
font-weight: bold;
}
.a:hover {
transform: rotate(360deg);
}
.b {
transition: All 0.4s ease-in-out;
width: 200px;
height: 200px;
background-color: #000000;
display: block;
text-align: center;
line-height: 200px;
font-size: 20px;
font-weight: bold;
}
.b:hover {
transform: scale(1.2);
}
.c {
transition: All 0.4s ease-in-out;
width: 200px;
height: 200px;
background-color: #000000;
display: block;
text-align: center;
line-height: 200px;
font-size: 20px;
font-weight: bold;
}
.c:hover {
transform: rotate(360deg) scale(1.2);
}
.d {
transition: All 0.4s ease-in-out;
width: 200px;
height: 200px;
background-color: #000000;
display: block;
text-align: center;
line-height: 200px;
font-size: 20px;
font-weight: bold;
}
.d:hover {
transform: translate(0, -10px);
}
.flip-container {
perspective: 1000;
clear: both;
border: 1px solid red;
margin-top: 50px;
margin-left: 50px;
}
.flip-container:hover .flipper {
transform: rotateY(-180deg);
}
.flip-container,
.front,
.back {
width: 200px;
height: 200px;
}
.flipper {
transition: 2s;
transform-style: preserve-3d;
position: relative;
}
.front,
.back {
backface-visibility: hidden;
position: absolute;
top: 0;
left: 0;
}
img {
width: 200px;
height: 200px;
overflow: hidden;
}
.front {
background: red;
z-index: 2;
}
.back {
background: blue;
transform: rotateY(-180deg);
}
.flip-container1 {
perspective: 1000;
border: 1px solid red;
margin-top: 50px;
margin-left: 50px;
}
.flip-container1:hover .flipper1 {
transform: rotateX(-180deg);
}
.flip-container1,
.front1,
.back1 {
width: 200px;
height: 200px;
}
.flipper1 {
transition: 2s;
transform-style: preserve-3d;
position: relative;
transform-origin: 100% 100px;
}
.front1,
.back1 {
backface-visibility: hidden;
position: absolute;
top: 0;
left: 0;
}
img {
width: 200px;
height: 200px;
overflow: hidden;
}
.front1 {
background: red;
z-index: 2;
}
.back1 {
background: blue;
transform: rotateX(-180deg);
}
</style>
</head>
<body>
<a class="a">360度旋转</a>
<a class="b">悬浮放大效果</a>
<a class="c">旋转放大</a>
<a class="d">上下移动</a>
<h1 style="clear: both;">垂直翻转</h1>
<div class="flip-container">
<div class="flipper">
<div class="front">
<img src="img/1.jpg" />
</div>
<div class="back">
<img src="img/2.jpg" />
</div>
</div>
</div>
<h1>水平翻转</h1>
<div class="flip-container1">
<div class="flipper1">
<div class="front1">
<img src="img/1.jpg" />
</div>
<div class="back1">
<img src="img/2.jpg" />
</div>
</div>
</div>
</body>
</html>
JS实现
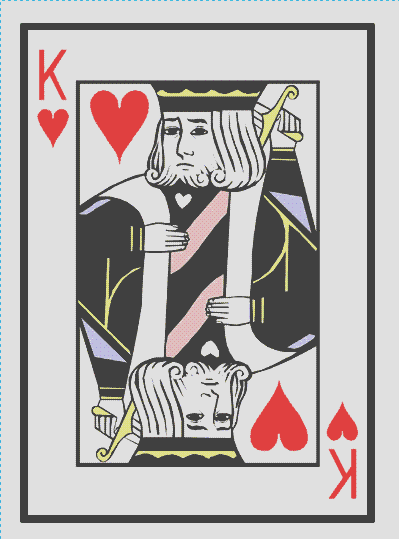
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<script src="js/jquery-2.1.0.min.js"></script>
<title>CSS3 翻转效果 </title>
<link rel="stylesheet" href="css/fanzhuan.css" type="text/css" />
<style>
#box {
width: 355px;
height: 500px;
margin: 30px auto;
position: relative;
border: 1px solid red;
}
.list {
position: absolute;
}
.list img {
border-radius: 2px;
}
</style>
<script>
$(function() {
var eleBack = null,
eleFront = null,
eleList = $(".list");
var funBackOrFront = function() {
eleList.each(function() {
if ($(this).hasClass("out")) {
eleBack = $(this);
} else {
eleFront = $(this);
}
});
};
funBackOrFront();
$("#box").hover(function() {
eleFront.addClass("out").removeClass("in");
setTimeout(function() {
eleBack.addClass("in").removeClass("out");
funBackOrFront();
}, 225);
return false;
});
})
</script>
</head>
<body>
<h1>必须给要翻转的添加flip类</h1>
<div id="box">
<span class="list flip out"><img src="img/puke-back.png" alt="纸牌正面" /></span>
<span class="list flip"><img src="img/puke-k.png" alt="纸牌背面" /> </span>
</div>
</body>
</html>
需要fanzhuan.css支持
.in {
-webkit-animation-timing-function: ease-out;
-webkit-animation-duration: 350ms;
animation-timing-function: ease-out;
animation-duration: 350ms;
}
.out {
-webkit-animation-timing-function: ease-in;
-webkit-animation-duration: 225ms;
animation-timing-function: ease-in;
animation-duration: 225ms;
}
@-webkit-keyframes fadein {
from { opacity: 0; }
to { opacity: 1; }
}
@keyframes fadein {
from { opacity: 0; }
to { opacity: 1; }
}
@-webkit-keyframes fadeout {
from { opacity: 1; }
to { opacity: 0; }
}
@keyframes fadeout {
from { opacity: 1; }
to { opacity: 0; }
}
.fade.out {
opacity: 0;
-webkit-animation-duration: 125ms;
-webkit-animation-name: fadeout;
animation-duration: 125ms;
animation-name: fadeout;
}
.fade.in {
opacity: 1;
-webkit-animation-duration: 225ms;
-webkit-animation-name: fadein;
animation-duration: 225ms;
animation-name: fadein;
}
.pop {
-webkit-transform-origin: 50% 50%;
transform-origin: 50% 50%;
}
.pop.in {
-webkit-transform: scale(1);
-webkit-animation-name: popin;
-webkit-animation-duration: 350ms;
transform: scale(1);
animation-name: popin;
animation-duration: 350ms;
opacity: 1;
}
.pop.out {
-webkit-animation-name: fadeout;
-webkit-animation-duration: 100ms;
animation-name: fadeout;
animation-duration: 100ms;
opacity: 0;
}
.pop.in.reverse {
-webkit-animation-name: fadein;
animation-name: fadein;
}
.pop.out.reverse {
-webkit-transform: scale(.8);
-webkit-animation-name: popout;
transform: scale(.8);
animation-name: popout;
}
@-webkit-keyframes popin {
from {
-webkit-transform: scale(.8);
opacity: 0;
}
to {
-webkit-transform: scale(1);
opacity: 1;
}
}
@keyframes popin {
from {
transform: scale(.8);
opacity: 0;
}
to {
transform: scale(1);
opacity: 1;
}
}
@-webkit-keyframes popout {
from {
-webkit-transform: scale(1);
opacity: 1;
}
to {
-webkit-transform: scale(.8);
opacity: 0;
}
}
@keyframes popout {
from {
transform: scale(1);
opacity: 1;
}
to {
transform: scale(.8);
opacity: 0;
}
}
@-webkit-keyframes slideinfromright {
from { -webkit-transform: translate3d(100%,0,0); }
to { -webkit-transform: translate3d(0,0,0); }
}
@keyframes slideinfromright {
from { transform: translateX(100%); }
to { transform: translateX(0); }
}
@-webkit-keyframes slideinfromleft {
from { -webkit-transform: translate3d(-100%,0,0); }
to { -webkit-transform: translate3d(0,0,0); }
}
@keyframes slideinfromleft {
from { transform: translateX(-100%); }
to { transform: translateX(0); }
}
@-webkit-keyframes slideouttoleft {
from { -webkit-transform: translate3d(0,0,0); }
to { -webkit-transform: translate3d(-100%,0,0); }
}
@keyframes slideouttoleft {
from { transform: translateX(0); }
to { transform: translateX(-100%); }
}
@-webkit-keyframes slideouttoright {
from { -webkit-transform: translate3d(0,0,0); }
to { -webkit-transform: translate3d(100%,0,0); }
}
@keyframes slideouttoright {
from { transform: translateX(0); }
to { transform: translateX(100%); }
}
.slide.out, .slide.in {
-webkit-animation-timing-function: ease-out;
-webkit-animation-duration: 350ms;
animation-timing-function: ease-out;
animation-duration: 350ms;
}
.slide.out {
-webkit-transform: translate3d(-100%,0,0);
-webkit-animation-name: slideouttoleft;
transform: translateX(-100%);
animation-name: slideouttoleft;
}
.slide.in {
-webkit-transform: translate3d(0,0,0);
-webkit-animation-name: slideinfromright;
transform: translateX(0);
animation-name: slideinfromright;
}
.slide.out.reverse {
-webkit-transform: translate3d(100%,0,0);
-webkit-animation-name: slideouttoright;
transform: translateX(100%);
animation-name: slideouttoright;
}
.slide.in.reverse {
-webkit-transform: translate3d(0,0,0);
-webkit-animation-name: slideinfromleft;
transform: translateX(0);
animation-name: slideinfromleft;
}
.slidefade.out {
-webkit-transform: translateX(-100%);
-webkit-animation-name: slideouttoleft;
-webkit-animation-duration: 225ms;
transform: translateX(-100%);
animation-name: slideouttoleft;
animation-duration: 225ms;
}
.slidefade.in {
-webkit-transform: translateX(0);
-webkit-animation-name: fadein;
-webkit-animation-duration: 200ms;
transform: translateX(0);
animation-name: fadein;
animation-duration: 200ms;
}
.slidefade.out.reverse {
-webkit-transform: translateX(100%);
-webkit-animation-name: slideouttoright;
-webkit-animation-duration: 200ms;
transform: translateX(100%);
animation-name: slideouttoright;
animation-duration: 200ms;
}
.slidefade.in.reverse {
-webkit-transform: translateX(0);
-webkit-animation-name: fadein;
-webkit-animation-duration: 200ms;
transform: translateX(0);
animation-name: fadein;
animation-duration: 200ms;
}
.slidedown.out {
-webkit-animation-name: fadeout;
-webkit-animation-duration: 100ms;
animation-name: fadeout;
animation-duration: 100ms;
}
.slidedown.in {
-webkit-transform: translateY(0);
-webkit-animation-name: slideinfromtop;
-webkit-animation-duration: 250ms;
transform: translateY(0);
animation-name: slideinfromtop;
animation-duration: 250ms;
}
.slidedown.in.reverse {
-webkit-animation-name: fadein;
-webkit-animation-duration: 150ms;
animation-name: fadein;
animation-duration: 150ms;
}
.slidedown.out.reverse {
-webkit-transform: translateY(-100%);
-webkit-animation-name: slideouttotop;
-webkit-animation-duration: 200ms;
transform: translateY(-100%);
animation-name: slideouttotop;
animation-duration: 200ms;
}
@-webkit-keyframes slideinfromtop {
from { -webkit-transform: translateY(-100%); }
to { -webkit-transform: translateY(0); }
}
@keyframes slideinfromtop {
from { transform: translateY(-100%); }
to { transform: translateY(0); }
}
@-webkit-keyframes slideouttotop {
from { -webkit-transform: translateY(0); }
to { -webkit-transform: translateY(-100%); }
}
@keyframes slideouttotop {
from { transform: translateY(0); }
to { transform: translateY(-100%); }
}
.slideup.out {
-webkit-animation-name: fadeout;
-webkit-animation-duration: 100ms;
animation-name: fadeout;
animation-duration: 100ms;
}
.slideup.in {
-webkit-transform: translateY(0);
-webkit-animation-name: slideinfrombottom;
-webkit-animation-duration: 250ms;
transform: translateY(0);
animation-name: slideinfrombottom;
animation-duration: 250ms;
}
.slideup.in.reverse {
-webkit-animation-name: fadein;
-webkit-animation-duration: 150ms;
animation-name: fadein;
animation-duration: 150ms;
}
.slideup.out.reverse {
-webkit-transform: translateY(100%);
-webkit-animation-name: slideouttobottom;
-webkit-animation-duration: 200ms;
transform: translateY(100%);
animation-name: slideouttobottom;
animation-duration: 200ms;
}
@-webkit-keyframes slideinfrombottom {
from { -webkit-transform: translateY(100%); }
to { -webkit-transform: translateY(0); }
}
@keyframes slideinfrombottom {
from { transform: translateY(100%); }
to { transform: translateY(0); }
}
@-webkit-keyframes slideouttobottom {
from { -webkit-transform: translateY(0); }
to { -webkit-transform: translateY(100%); }
}
@keyframes slideouttobottom {
from { transform: translateY(0); }
to { transform: translateY(100%); }
}
.viewport-flip {
-webkit-perspective: 1000;
perspective: 1000;
position: absolute;
}
.flip {
-webkit-backface-visibility: hidden;
-webkit-transform: translateX(0);
backface-visibility: hidden;
transform: translateX(0);
}
.flip.out {
-webkit-transform: rotateY(-90deg) scale(.9);
-webkit-animation-name: flipouttoleft;
-webkit-animation-duration: 175ms;
transform: rotateY(-90deg) scale(.9);
animation-name: flipouttoleft;
animation-duration: 175ms;
}
.flip.in {
-webkit-animation-name: flipintoright;
-webkit-animation-duration: 225ms;
animation-name: flipintoright;
animation-duration: 225ms;
}
.flip.out.reverse {
-webkit-transform: rotateY(90deg) scale(.9);
-webkit-animation-name: flipouttoright;
transform: rotateY(90deg) scale(.9);
animation-name: flipouttoright;
}
.flip.in.reverse {
-webkit-animation-name: flipintoleft;
animation-name: flipintoleft;
}
@-webkit-keyframes flipouttoleft {
from { -webkit-transform: rotateY(0); }
to { -webkit-transform: rotateY(-90deg) scale(.9); }
}
@keyframes flipouttoleft {
from { transform: rotateY(0); }
to { transform: rotateY(-90deg) scale(.9); }
}
@-webkit-keyframes flipouttoright {
from { -webkit-transform: rotateY(0) ; }
to { -webkit-transform: rotateY(90deg) scale(.9); }
}
@keyframes flipouttoright {
from { transform: rotateY(0); }
to { transform: rotateY(90deg) scale(.9); }
}
@-webkit-keyframes flipintoleft {
from { -webkit-transform: rotateY(-90deg) scale(.9); }
to { -webkit-transform: rotateY(0); }
}
@keyframes flipintoleft {
from { transform: rotateY(-90deg) scale(.9); }
to { transform: rotateY(0); }
}
@-webkit-keyframes flipintoright {
from { -webkit-transform: rotateY(90deg) scale(.9); }
to { -webkit-transform: rotateY(0); }
}
@keyframes flipintoright {
from { transform: rotateY(90deg) scale(.9); }
to { transform: rotateY(0); }
}
.viewport-turn {
-webkit-perspective: 200px;
perspective: 200px;
position: absolute;
}
.turn {
-webkit-backface-visibility: hidden;
-webkit-transform: translateX(0);
-webkit-transform-origin: 0;
backface-visibility :hidden;
transform: translateX(0);
transform-origin: 0;
}
.turn.out {
-webkit-transform: rotateY(-90deg) scale(.9);
-webkit-animation-name: flipouttoleft;
-webkit-animation-duration: 125ms;
transform: rotateY(-90deg) scale(.9);
animation-name: flipouttoleft;
animation-duration: 125ms;
}
.turn.in {
-webkit-animation-name: flipintoright;
-webkit-animation-duration: 250ms;
animation-name: flipintoright;
animation-duration: 250ms;
}
.turn.out.reverse {
-webkit-transform: rotateY(90deg) scale(.9);
-webkit-animation-name: flipouttoright;
transform: rotateY(90deg) scale(.9);
animation-name: flipouttoright;
}
.turn.in.reverse {
-webkit-animation-name: flipintoleft;
animation-name: flipintoleft;
}
@-webkit-keyframes flipouttoleft {
from { -webkit-transform: rotateY(0); }
to { -webkit-transform: rotateY(-90deg) scale(.9); }
}
@keyframes flipouttoleft {
from { transform: rotateY(0); }
to { transform: rotateY(-90deg) scale(.9); }
}
@-webkit-keyframes flipouttoright {
from { -webkit-transform: rotateY(0) ; }
to { -webkit-transform: rotateY(90deg) scale(.9); }
}
@keyframes flipouttoright {
from { transform: rotateY(0); }
to { transform: rotateY(90deg) scale(.9); }
}
@-webkit-keyframes flipintoleft {
from { -webkit-transform: rotateY(-90deg) scale(.9); }
to { -webkit-transform: rotateY(0); }
}
@keyframes flipintoleft {
from { transform: rotateY(-90deg) scale(.9); }
to { transform: rotateY(0); }
}
@-webkit-keyframes flipintoright {
from { -webkit-transform: rotateY(90deg) scale(.9); }
to { -webkit-transform: rotateY(0); }
}
@keyframes flipintoright {
from { transform: rotateY(90deg) scale(.9); }
to { transform: rotateY(0); }
}
.flow {
-webkit-transform-origin: 50% 30%;
transform-origin: 50% 30%;
}
.flow.out {
-webkit-transform: translateX(-100%) scale(.7);
-webkit-animation-name: flowouttoleft;
-webkit-animation-timing-function: ease;
-webkit-animation-duration: 350ms;
transform: translateX(-100%) scale(.7);
animation-name: flowouttoleft;
animation-timing-function: ease;
animation-duration: 350ms;
}
.flow.in {
-webkit-transform: translateX(0) scale(1);
-webkit-animation-name: flowinfromright;
-webkit-animation-timing-function: ease;
-webkit-animation-duration: 350ms;
transform: translateX(0) scale(1);
animation-name: flowinfromright;
animation-timing-function: ease;
animation-duration: 350ms;
}
.flow.out.reverse {
-webkit-transform: translateX(100%);
-webkit-animation-name: flowouttoright;
transform: translateX(100%);
animation-name: flowouttoright;
}
.flow.in.reverse {
-webkit-animation-name: flowinfromleft;
animation-name: flowinfromleft;
}
@-webkit-keyframes flowouttoleft {
0% { -webkit-transform: translateX(0) scale(1); }
60%, 70% { -webkit-transform: translateX(0) scale(.7); }
100% { -webkit-transform: translateX(-100%) scale(.7); }
}
@keyframes flowouttoleft {
0% { transform: translateX(0) scale(1); }
60%, 70% { transform: translateX(0) scale(.7); }
100% { transform: translateX(-100%) scale(.7); }
}
@-webkit-keyframes flowouttoright {
0% { -webkit-transform: translateX(0) scale(1); }
60%, 70% { -webkit-transform: translateX(0) scale(.7); }
100% { -webkit-transform: translateX(100%) scale(.7); }
}
@keyframes flowouttoright {
0% { transform: translateX(0) scale(1); }
60%, 70% { transform: translateX(0) scale(.7); }
100% { transform: translateX(100%) scale(.7); }
}
@-webkit-keyframes flowinfromleft {
0% { -webkit-transform: translateX(-100%) scale(.7); }
30%, 40% { -webkit-transform: translateX(0) scale(.7); }
100% { -webkit-transform: translateX(0) scale(1); }
}
@keyframes flowinfromleft {
0% { transform: translateX(-100%) scale(.7); }
30%, 40% { transform: translateX(0) scale(.7); }
100% { transform: translateX(0) scale(1); }
}
@-webkit-keyframes flowinfromright {
0% { -webkit-transform: translateX(100%) scale(.7); }
30%, 40% { -webkit-transform: translateX(0) scale(.7); }
100% { -webkit-transform: translateX(0) scale(1); }
}
@keyframes flowinfromright {
0% { transform: translateX(100%) scale(.7); }
30%, 40% { transform: translateX(0) scale(.7); }
100% { transform: translateX(0) scale(1); }
}