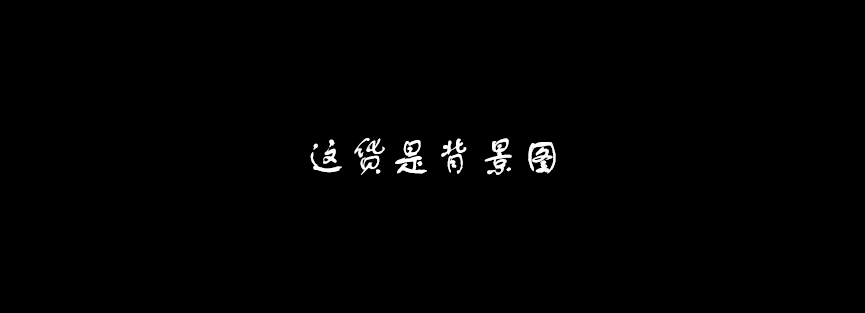
在学习完servlet与jsp技术后,我们可以先来做一个小练习,来实现第一个网页与后台和数据库之间的交叉练习;实现
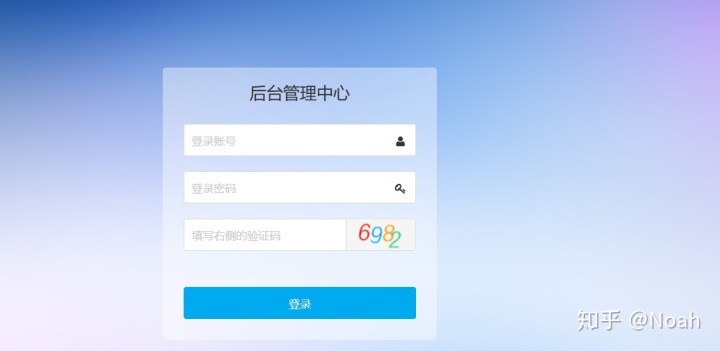
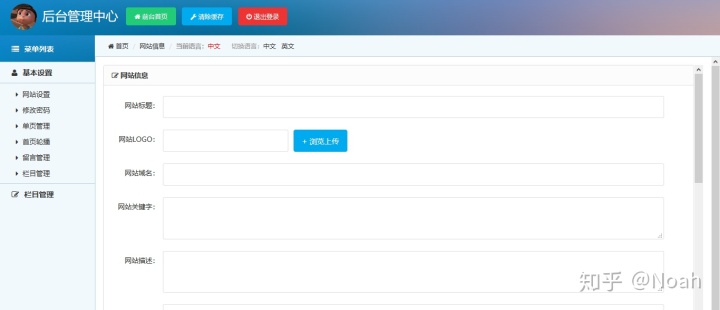
简单的来说要达到三个需求:
- 实现用户登录
- 实现用户退出
- 实现用户注册
简单来说就是要进行这样的分析:
- 用户登录:根据用户名和密码查询用户信息。查到则登录成功,查不到则登录失败。
- 用户退出:销毁session
- 用户注册:将用户注册信息插入数据库
首先呢我们要在数据库中创建一个表叫做t_user
建表语句与插入语句可以这样写:
create table t_user1(
uid INT auto_increment,
uname VARCHAR(20) not null,
pwd VARCHAR(20) not null,
age int(3) not null,
sex VARCHAR(10) not null,
birthday date not null,
PRIMARY key(uid)
)
insert into t_user1 values(default,'皮皮','123',18,'男','2009-1-01')
然后我们就可以构建这个项目了:
我们要实现mvc编程思想:所以要实现自己的业务逻辑分层,建好自己的包,
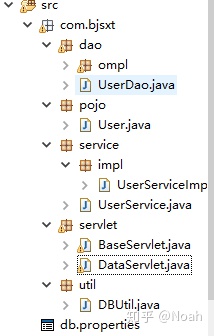
实现分层,我们就是对具体类的编写:首先要编写实体类User
public class User {
private int uid;
private String uname;
private String pwd;
private String sex;
private int age;
private String birthday;
这个user实体没有截完正,里面还应该有共有取值赋值方法,与tostring方法,带参与无参构造方法。
BaseServlet处理请求信息的编写
ckage com.bjsxt.servlet;
import java.io.IOException;
import java.lang.reflect.Method;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class BaseServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// TODO Auto-generated method stub
//设置编码格式
req.setCharacterEncoding("utf-8");
resp.setContentType("text/html;charset=utf-8");
//获取请求数据
String methodName=req.getParameter("method");
//处理请求数据(动态的根据方法名调用方法---反射)
//反射获取方法所在的类的类对象
System.out.println(methodName);
//反射获取要调用的对象
try {
Class cla=this.getClass();
Method m=cla.getMethod(methodName, HttpServletRequest.class,HttpServletResponse.class);
m.invoke(this, req,resp);
} catch (NoSuchMethodException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
处理方法的DataServlet编写
ackage com.bjsxt.servlet;
import java.io.IOException;
import java.lang.reflect.Method;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import com.bjsxt.pojo.User;
import com.bjsxt.service.UserService;
import com.bjsxt.service.impl.UserServiceImpl;
public class DataServlet extends BaseServlet {
//登陆数据处理方法
public void userLogin(HttpServletRequest req,HttpServletResponse resp) throws IOException{
//获取请求信息
String uname = req.getParameter("uname");
String pwd = req.getParameter("pwd");
//处理请求信息
System.out.println(uname+" "+pwd);
//处理业务对象
UserService us=new UserServiceImpl();
User u = us.getUserInfoService(uname,pwd);
System.out.println("jieguo"+u);
//创建或者获取seession对象
HttpSession session = req.getSession();
//处理响应结果
//重定向
if(u!=null){
//登陆成功
session.setAttribute("user", u);
resp.sendRedirect("/13project/main.jsp");
}else{
//登陆失败
session.setAttribute("flag", "loginFalse");
resp.sendRedirect("/13project/login.jsp");
}
}
//退出数据处理方法
public void userOut(HttpServletRequest req,HttpServletResponse resp) throws IOException{
//获取请求信息
//处理请求信息
HttpSession session =req.getSession();
session.invalidate();
//响应处理结果
resp.sendRedirect("/13project/login.jsp");
}
//注册数据处理方法
public void userReg(HttpServletRequest req,HttpServletResponse resp) throws IOException{
//获取请求信息
String uname = req.getParameter("uname");
String pwd = req.getParameter("pwd");
String sex = req.getParameter("sex");
int age = Integer.parseInt(req.getParameter("age"));
String birthday = req.getParameter("birthday");
//处理请求信息
//获取业务层对象
UserService us=new UserServiceImpl();
int i=us.regUserInfoService(uname,pwd,sex,age,birthday);
//处理注册
//处理响应结果
//重定向
if(i>0){
resp.sendRedirect("/13project/login.jsp");
}else{
resp.sendRedirect("/13project/reg.jsp");
}
}
}
业务类接口userService
package com.bjsxt.service;
import com.bjsxt.pojo.User;
public interface UserService {
//用户登陆
User getUserInfoService(String uname, String pwd);
int regUserInfoService(String uname, String pwd, String sex, int age,
String birthday);
}
业务实现类UserServiceImpl
package com.bjsxt.service.impl;
import com.bjsxt.dao.UserDao;
import com.bjsxt.dao.ompl.UserDaoImpl;
import com.bjsxt.pojo.User;
import com.bjsxt.service.UserService;
public class UserServiceImpl implements UserService {
//创建Dao对象
UserDao ud = new UserDaoImpl();
@Override
public User getUserInfoService(String uname, String pwd) {
// 处理业务
return ud.getUserInfoDao(uname,pwd);
}
@Override
public int regUserInfoService(String uname, String pwd, String sex,
int age, String birthday) {
return ud.regUserInfoDao(uname,pwd,sex,age,birthday);
}
}
底层dao接口
package com.bjsxt.dao;
import com.bjsxt.pojo.User;
public interface UserDao {
//查询用户信息
User getUserInfoDao(String uname, String pwd);
int regUserInfoDao(String uname, String pwd, String sex, int age,
String birthday);
}
dao的实现类
package com.bjsxt.dao.ompl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.bjsxt.dao.UserDao;
import com.bjsxt.pojo.User;
import com.bjsxt.util.DBUtil;
public class UserDaoImpl implements UserDao{
@Override
public User getUserInfoDao(String uname, String pwd) {
// 声明jdbc变量
Connection conn=null;
PreparedStatement ps=null;
ResultSet rs=null;
User u=null;
try {
//创建连接
conn = DBUtil.getConnection();
//创建sql语句
String sql = "select * from t_user where uname=? and pwd=?";
//创建sql命令对象
ps = conn.prepareStatement(sql);
//占位符命令
ps.setString(1, uname);
ps.setString(2, pwd);
//执行sql
rs=ps.executeQuery();
//遍历
while(rs.next()){
u = new User();
u.setUid(rs.getInt("uid"));
u.setUname(rs.getString("uname"));
u.setPwd(rs.getString("pwd"));
u.setSex(rs.getString("sex"));
u.setAge(rs.getInt("age"));
u.setBirthday(rs.getString("birthday"));
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, ps, conn);
}
//返回结果
return u;
}
@Override
public int regUserInfoDao(String uname, String pwd, String sex, int age,
String birthday) {
//创建sql语句
String sql="insert into t_user values(default,?,?,?,?,?)";
return DBUtil.executeDML(sql, uname,pwd,sex,age,birthday);
}
}
还有页面实现的jsp文件
登陆页面login.jsp等,具体的源码奉上
链接: https://pan.baidu.com/s/1KtOPiGX24P2gNKl7TJVs9w 提取码: 24ua