二、Pygame最小开发框架
这一节包括五部分内容,如下:
- Pygame简介与安装
- Pygame最小开发框架及最小游戏
- 壁球小游戏(展示型)与图像的基本使用
- 壁球小游戏(节奏型)与屏幕的帧率设置
- 壁球小游戏(操控型)与键盘的基本使用
1.Pygame简介与安装
直接用图说话:
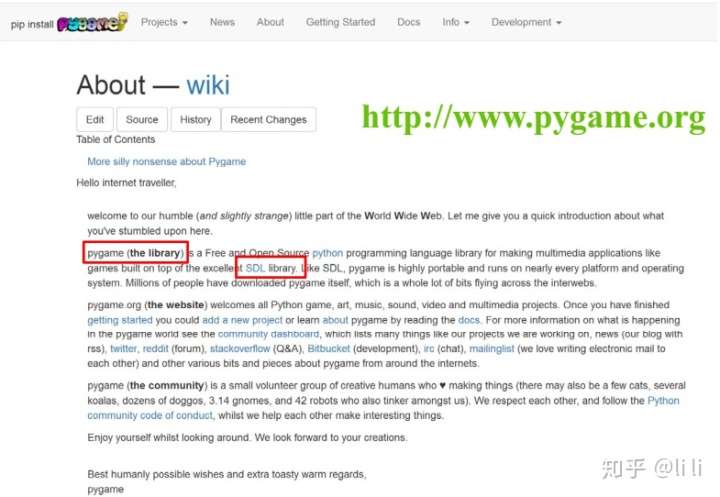
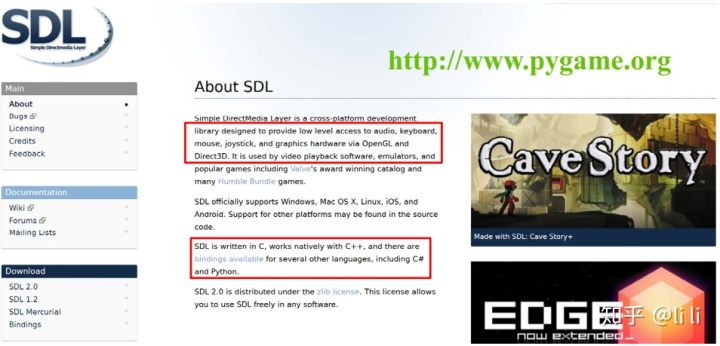
SDL(Simple DirectMedia Layer)是一套开放源代码的跨平台多媒体开发库,使用C语言写成。SDL提供了数种控制图像、声音、输出入的函数,让开发者只要用相同或是相似的代码就可以开发出跨多个平台(Linux、Windows、Mac OS X等)的应用软件。目前SDL多用于开发游戏、模拟器、媒体播放器等多媒体应用领域。
pygame (the library) is a Free and Open Sourcepythonprogramming language library for making multimedia applications like games built on top of the excellentSDLlibrary. Like SDL, pygame is highly portable and runs on nearly every platform and operating system. Millions of people have downloaded pygame itself, which is a whole lot of bits flying across the interwebs.
Pygame的安装:
pip install pygame
安装完成后的小测试:
(py3NetworkProgramming) C:UsersAdministratorEnvspy3NetworkProgrammingLibsite-packagespygameexamples>python aliens.py
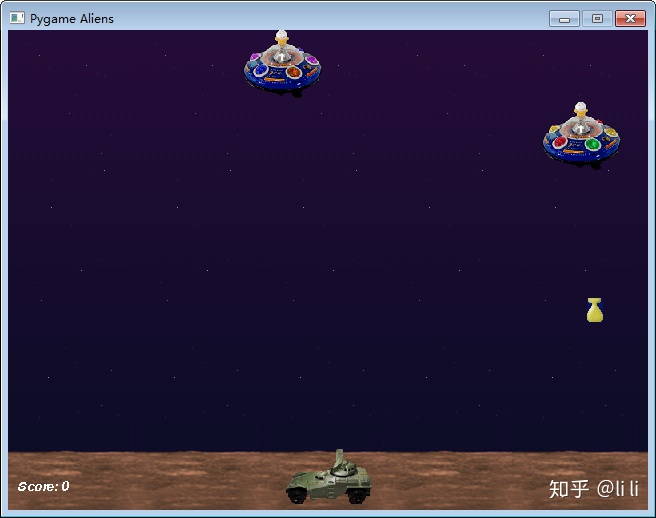
对Pygame的理解:
Python最经典的2D游戏开发第三方库,也支持3D游戏开发
• Pygame适合用于游戏逻辑验证、游戏入门及系统演示验证
• Pygame是一种游戏开发引擎,基本逻辑具有参考价值
• Pygame有些"过时",但永远"不过时"
• 使用Pygame可以开发出优秀的游戏!
对于学习的态度,我喜欢作者下面的配图和文字:
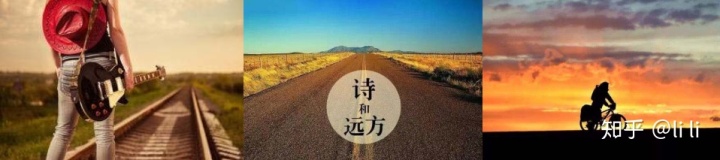
无论学什么专业,从事什么工作,在世界的哪里坚守着
即使我们不能仗剑天涯,即使要面对眼前的苟且
请学习Pygame,用技术让自己的心灵来趟"诗和远方"的旅行吧
2.Pygame最小开发框架及最小游戏
直接上图和代码:
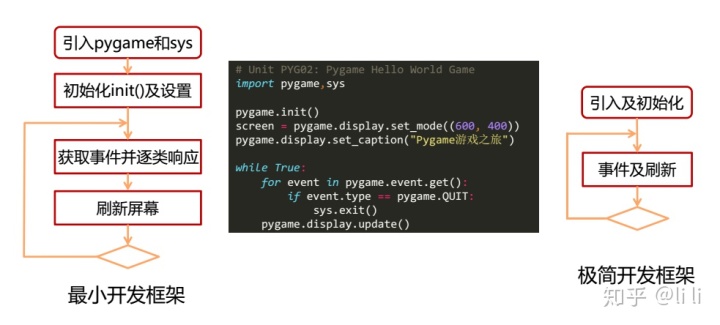
代码:
# Unit PYG02: Pygame Hello World Game
import sys
import pygame
pygame.init()
screen = pygame.display.set_mode((600, 400))
pygame.display.set_caption("Pygame游戏之旅")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
pygame.display.update()
代码说明:
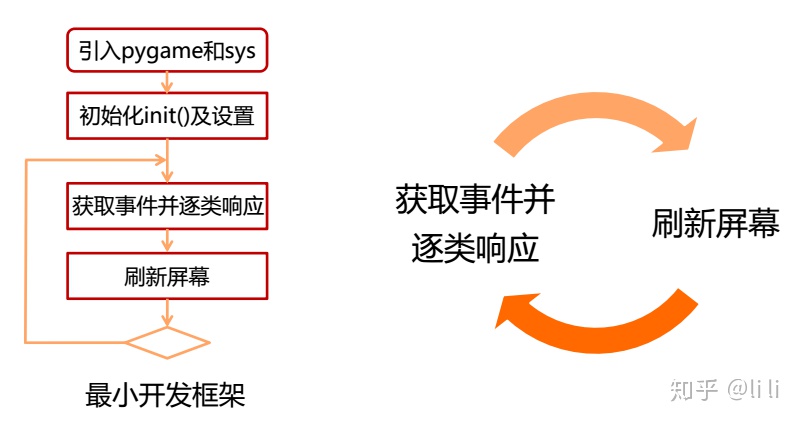
- sys是Python的标准库
- sys提供Python运行时环境变量的操控
- sys.exit()用于退出结束游戏并退出
pygame.init()
- 对Pygame内部各功能模块进行初始化创建及变量设置,默认调用
pygame.display.set_mode(size)
- 初始化显示窗口,第一个参数size是一个二值元组,分别表示窗口的宽度和高度
pygame.display.set_caption(title)
- 设置显示窗口的标题内容,参数title是一个字符串类型
while True:
- 无限循环,直到Python运行时退出结束
pygame.event.get()
- 从Pygame的事件队列中取出事件,并从队列中删除该事件,例如:键盘按下是一个事件。
event.type
- 获得事件类型,并逐类响应
pygame.QUIT
- 是Pygame中定义的退出事件常量
pygame.display.update()
- 对显示窗口进行更新,默认窗口全部重绘
3.壁球小游戏(展示型)与图像的基本使用
壁球小游戏(展示型)的关键要素
- 壁球:游戏需要一个壁球,通过图片引入
- 壁球运动:壁球要能够上下左右运动
- 壁球反弹:壁球要能够在上下左右边缘反弹
素材下载位置:https://python123.io/PY15/PYG02-ball.gif
代码:
# Unit PYG02: Pygame Wall Ball Game version 1 展示型
import sys
import pygame
pygame.init()
size = width, height = 600, 400
speed = [1,1]
BLACK = 0, 0, 0
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Pygame壁球")
ball = pygame.image.load("PYG02-ball.gif")
ballrect = ball.get_rect()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
ballrect = ballrect.move(speed[0], speed[1])
if ballrect.left < 0 or ballrect.right > width:
speed[0] = - speed[0]
if ballrect.top < 0 or ballrect.bottom > height:
speed[1] = - speed[1]
screen.fill(BLACK)
screen.blit(ball, ballrect)
pygame.display.update()
代码说明:
pygame.image.load(filename)
- 将filename路径下的图像载入游戏,支持JPG、PNG、 GIF(非动画)等13种常用图片格式
Surface对象 ball.get_rect()
- Pygame使用内部定义的Surface对象表示所有载入的图像,其中.get_rect()方法返回一个覆盖图像的矩形Rect对象
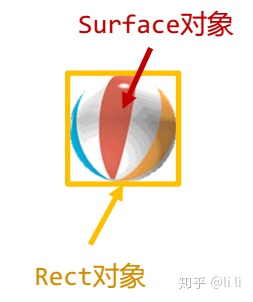
Rect对象
- Rect对象有一些重要属性,例如:top,bottom,left,right 表示上下左右width,height 表示宽度、高度
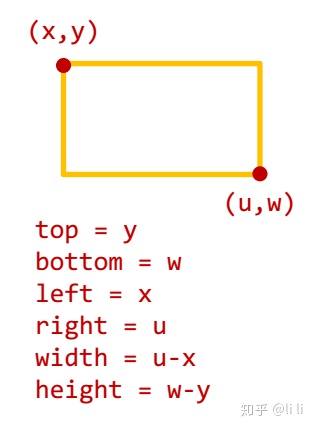
ballrect.move(x,y)
- 矩形移动一个偏移量(x,y),即在横轴方向移动x像素,纵轴方向移动y像素,xy为整数
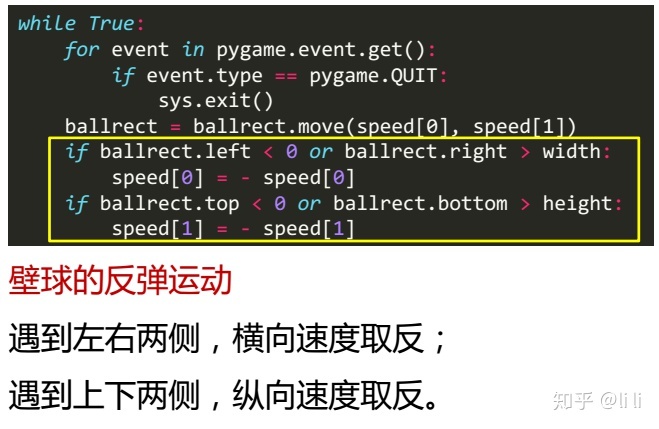
screen.fill(color)
- 显示窗口背景填充为color颜色,采用RGB色彩体系。由于壁球不断运动,运动后原有位置将默认填充白色,因此需要不断刷新背景色
screen.blit(src, dest)
- 将一个图像绘制在另一个图像上,即将src绘制到dest位置上。通过Rect对象引导对壁球的绘制。
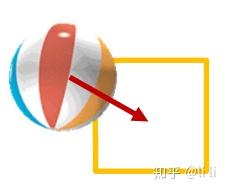
4.壁球小游戏(节奏型)与屏幕的帧率设置
需求:
- 壁球可以按照一定速度运动
从需求到实现的关键要素:
- 壁球速度:如何控制壁球的运动速度呢?
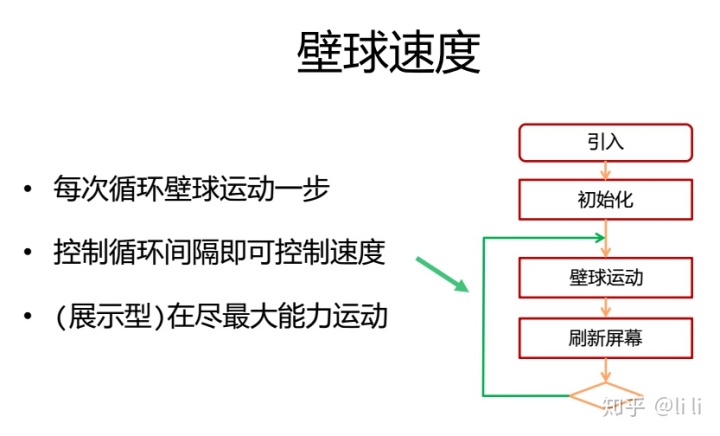
代码:
# Unit PYG02: Pygame Wall Ball Game version 2 节奏型
import pygame
import sys
pygame.init()
size = width, height = 600, 400
speed = [1,1]
BLACK = 0, 0, 0
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Pygame壁球")
ball = pygame.image.load("PYG02-ball.gif")
ballrect = ball.get_rect()
fps = 300
fclock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
ballrect = ballrect.move(speed[0], speed[1])
if ballrect.left < 0 or ballrect.right > width:
speed[0] = - speed[0]
if ballrect.top < 0 or ballrect.bottom > height:
speed[1] = - speed[1]
screen.fill(BLACK)
screen.blit(ball, ballrect)
pygame.display.update()
fclock.tick(fps)
代码说明:
pygame.time.Clock()
- 创建一个Clock对象,用于操作时间
clock.tick(framerate)
- 控制帧速度,即窗口刷新速度,例如:clock.tick(100)表示每秒钟100次帧刷新视频中每次展示的静态图像称为帧
5.壁球小游戏(操控型)与键盘的基本使用
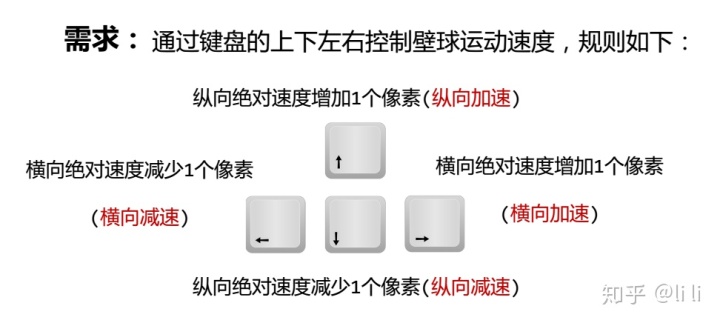
从需求到实现的关键要素:
- 键盘使用:如何获取键盘的操作事件
- 速度调节:根据对应按键调节壁球运动速度
代码:
# Unit PYG02: Pygame Wall Ball Game version 3 操控型
import pygame
import sys
pygame.init()
size = width, height = 600, 400
speed = [1,1]
BLACK = 0, 0, 0
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Pygame壁球")
ball = pygame.image.load("PYG02-ball.gif")
ballrect = ball.get_rect()
fps = 300
fclock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
speed[0] = speed[0] if speed[0] == 0 else (abs(speed[0]) - 1)*int(speed[0]/abs(speed[0]))
elif event.key == pygame.K_RIGHT:
speed[0] = speed[0] + 1 if speed[0] > 0 else speed[0] - 1
elif event.key == pygame.K_UP:
speed[1] = speed[1] + 1 if speed[1] > 0 else speed[1] - 1
elif event.key == pygame.K_DOWN:
speed[1] = speed[1] if speed[1] == 0 else (abs(speed[1]) - 1)*int(speed[1]/abs(speed[1]))
ballrect = ballrect.move(speed)
if ballrect.left < 0 or ballrect.right > width:
speed[0] = - speed[0]
if ballrect.top < 0 or ballrect.bottom > height:
speed[1] = - speed[1]
screen.fill(BLACK)
screen.blit(ball, ballrect)
pygame.display.update()
fclock.tick(fps)
代码说明:
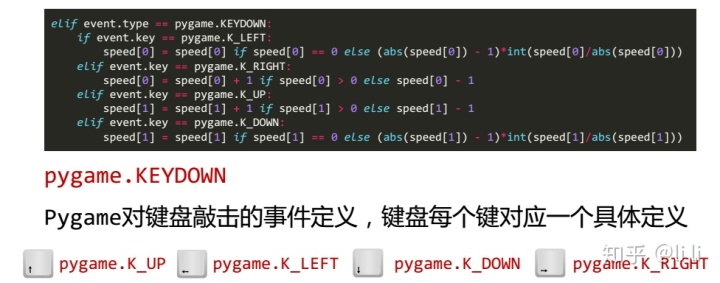
运行效果:
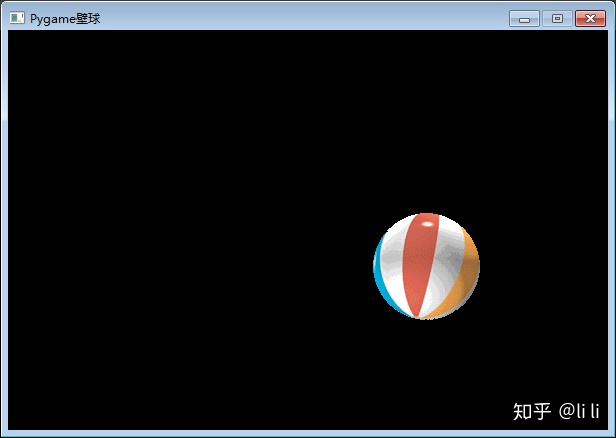