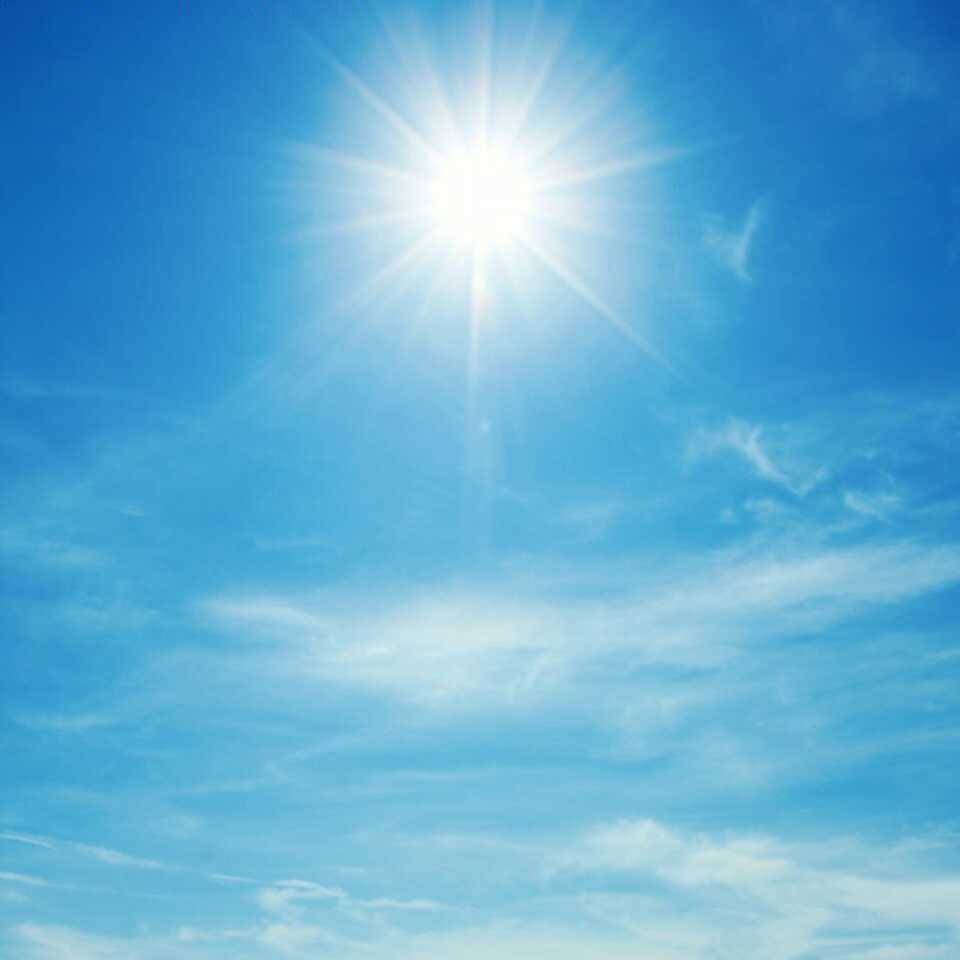
一、Goods 类:
package com.sxt.shichao5.copy;
public class Goods {
private String brand;
private String name;
private boolean isFlag;//用于标识是否有商品,假设true表示当前有商品,false表示当前没有商品
public Goods() {
super();
}
public Goods(String brand, String name) {
super();
this.brand = brand;
this.name = name;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public synchronized void set(String brand,String name){
if(isFlag){//相当于isFlag==true,表示当前有商品,
try {
super.wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}//当生产者线程被唤醒后从wait()之后的代码开始执行
}
//开始生产商品
this.setBrand(brand);
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
this.setName(name);
System.out.println("生产者生产了"+this.getBrand()+"---------------->"+this.getName());
//通知消费者
super.notify();//唤醒当前等待线程,就是消费者
this.isFlag = true;
}
public synchronized void get(){
if(!isFlag){
try {
super.wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
try {
Thread.sleep(300);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("消费者取走了"+this.getBrand()+"------------->"+this.getName());
//通知生产者
super.notify();//唤醒生产者
this.isFlag = false;
}
}
二、生产者Producter 类:
package com.sxt.shichao5.copy;
public class Producter implements Runnable{
private Goods goods;
public Producter(Goods goods) {
super();
this.goods = goods;
}
@Override
public void run() {
// TODO Auto-generated method stub
//开始生产商品
for(int i = 0;i<10;i++){
if(i%2!=0){//奇数时生产旺仔小馒头,偶数时生产娃哈哈矿泉水
goods.set("旺仔", "小馒头");
}else{
goods.set("娃哈哈", "矿泉水");
}
}
}
}
三、消费者类Costomer :
package com.sxt.shichao5.copy;
public class Costomer implements Runnable{
private Goods goods;
public Costomer(Goods goods) {
super();
this.goods = goods;
}
@Override
public void run() {
for(int i = 0;i<10;i++){
goods.get();
}
}
}
四、测试类Test :
package com.sxt.shichao5.copy;
public class Test {
public static void main(String[] args) {
// TODO Auto-generated method stub
Goods goods = new Goods();
Producter p = new Producter(goods);
Costomer c = new Costomer(goods);
Thread t1 = new Thread(p);
Thread t2 = new Thread(c);
//开启线程
t1.start();
t2.start();
}
}
五、运行结果图:
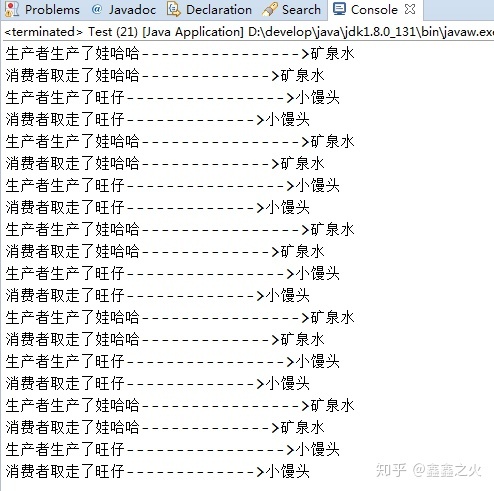