2.4 Maven的简单CURD练习
2.4.1 数据结构准备
数据库 : userdb
表 : user_info
数据要求 : 添加不少于200条 能够删除 更新 和 分页即可
2.4.2 大体思路
创建项目 > 创建包 > 导入jar > 创建实体类 > 创建DAO > 创建Service > 配置 Spring > 测试
初始化maven项目
<properties>
<!-- maven项目整体编码 -->
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- 项目编译的时候,源码(.java)使用哪个版本JDK -->
<maven.compiler.source>1.7</maven.compiler.source>
<!-- 项目编译的时候,可执行文件(.class)使用哪个版本JDK -->
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
修改了maven项目的一些初始化配置需要更新maven项目
更新流程 :
1) 在某个maven项目上点击右键 选择 "maven" > "Update Maven Project"
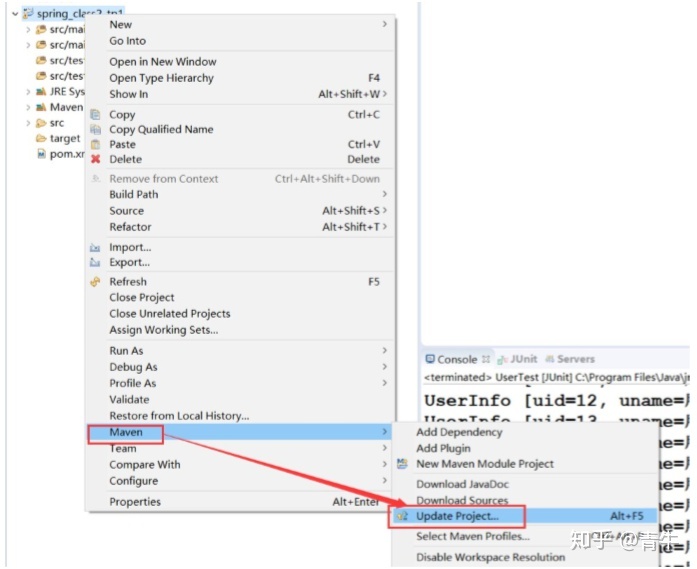
2) 在弹出的对话框点击 "OK" 即可
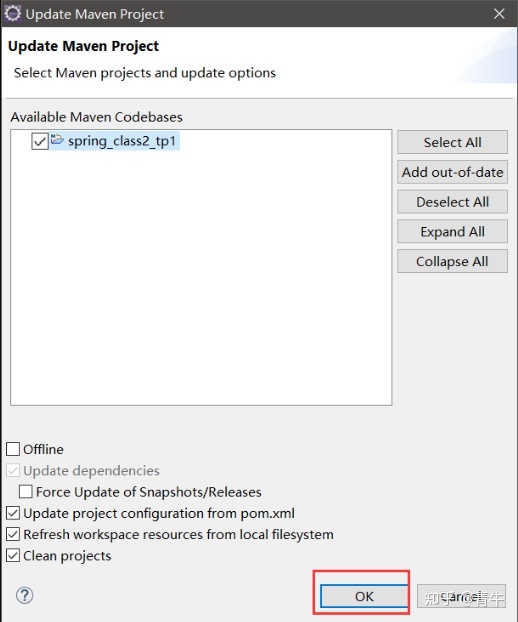
我们更新项目的主要目的就是为了解决maven项目初始化jdk版本的问题
更新前
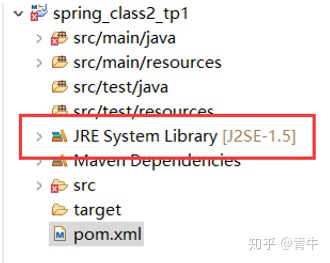
更新后
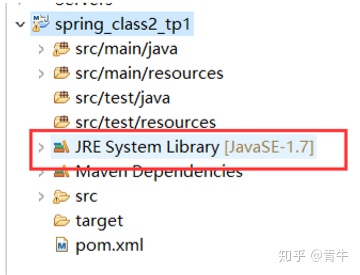
导入jar包
<dependencies>
<!-- 数据库相关jar包 -->
<dependency>
<groupId>commons-dbutils</groupId>
<artifactId>commons-dbutils</artifactId>
<version>1.6</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.9</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.6</version>
</dependency>
<!-- 单元测试相关jar包 -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<!-- JSTL和EL表达式jar包 -->
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<!-- Spring相关jar包 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
</dependencies>
2.4.3 具体实现
实体类
package com.hnxy.entity;
import java.util.Date;
/**
* 以OOP思想为基础
* @author My
*
*/
public class UserInfo {
// 私有属性
private Integer uid; // 主键ID
private String uname; // 客户姓名
private Date regDate; // 注册时间
private Double money; // 用户余额
// 共有方法
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public String getUname() {
return uname;
}
public void setUname(String uname) {
this.uname = uname;
}
public Date getRegDate() {
return regDate;
}
public void setRegDate(Date regDate) {
this.regDate = regDate;
}
public Double getMoney() {
return money;
}
public void setMoney(Double money) {
this.money = money;
}
@Override
public String toString() {
return "UserInfo [uid=" + uid + ", uname=" + uname + ", regDate=" + regDate + ", money=" + money + "]";
}
}
DAO层接口
package com.hnxy.dao;
import java.util.List;
import com.hnxy.entity.UserInfo;
/**
* 用户表的DAO层接口
* 减少类与类之间的耦合
* @author My
*
*/
public interface UserDAO {
/**
* 查询数据总条数
* @return
* @throws Exception
*/
public int findUserByPageListCount()throws Exception;
/**
* 分页查询数据
* @param start
* @param size
* @return
* @throws Exception
*/
public List<UserInfo> findUserByPageList(Integer start,Integer size)throws Exception;
/**
* 按ID查询
* @param uid
* @return
* @throws Exception
*/
public UserInfo findUserByID(Integer uid)throws Exception;
/**
* 添加
* @param user
* @return
* @throws Exception
*/
public int insertUser(UserInfo user)throws Exception;
/**
* 更新
* @param user
* @return
* @throws Exception
*/
public int updateUser(UserInfo user)throws Exception;
/**
* 删除
* @param user
* @return
* @throws Exception
*/
public int deleteUser(UserInfo user)throws Exception;
}
DAO层接口实现类
package com.hnxy.dao.impl;
import java.util.List;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import com.hnxy.dao.UserDAO;
import com.hnxy.entity.UserInfo;
/**
* UserDAO接口的实现类
* @author My
*
*/
public class UserDAOImpl implements UserDAO {
// 创建SQL的执行对象
private QueryRunner qr;
public void setQr(QueryRunner qr) {
this.qr = qr;
}
public int findUserByPageListCount() throws Exception {
// 创建方法的返回值
int totalCount = 0;
// 编写SQL语句
String sql = "select count(*) from v1";
// 占位符赋值
// 执行
Number num = qr.query(sql, new ScalarHandler<Number>(1));
// 处理返回值
totalCount = num.intValue();
// 返回
return totalCount;
}
public List<UserInfo> findUserByPageList(Integer start, Integer size) throws Exception {
// 创建方法的返回值
List<UserInfo> list = null;
// 创建SQL语句
String sql = "select * from v1 limit ?,?";
// 占位符赋值
Object[] params = {start,size};
// 执行
list = qr.query(sql, new BeanListHandler<UserInfo>(UserInfo.class),params);
// 返回
return list;
}
public UserInfo findUserByID(Integer uid) throws Exception {
// 创建方法的返回值
UserInfo user = null;
// 创建SQL语句
String sql = "select * from v1 where uid = ?";
// 占位符赋值
Object[] params = {uid};
// 执行
user = qr.query(sql, new BeanHandler<UserInfo>(UserInfo.class),params);
// 返回
return user;
}
public int insertUser(UserInfo user) throws Exception {
// 创建方法的返回值
int count = 0;
// 创建SQL语句
String sql = "insert into user_info values (null,?,?,?)";
// 占位符赋值
Object[] params = {user.getUname(),user.getRegDate(),user.getMoney()};
// 执行
count = qr.update(sql,params);
// 返回
return count;
}
public int updateUser(UserInfo user) throws Exception {
// 创建方法的返回值
int count = 0;
// 创建SQL语句
String sql = "update user_info set u_name=?,u_regdate=?,u_money=? where u_id = ?";
// 占位符赋值
Object[] params = {user.getUname(),user.getRegDate(),user.getMoney(),user.getUid()};
// 执行
count = qr.update(sql,params);
// 返回
return count;
}
public int deleteUser(UserInfo user) throws Exception {
// 创建方法的返回值
int count = 0;
// 创建SQL语句
String sql = "delete from user_info where u_id = ?";
// 占位符赋值
Object[] params = {user.getUid()};
// 执行
count = qr.update(sql,params);
// 返回
return count;
}
}
Service层接口
package com.hnxy.service;
import java.util.List;
import com.hnxy.entity.UserInfo;
/**
* 用户表的业务层接口
* @author My
*
*/
public interface UserService {
/**
* 查询数据总条数
* @return
* @throws Exception
*/
public int findUserByPageListCount()throws Exception;
/**
* 分页查询数据
* @param pageIndex 页码 dao start = service (pageIndex-1)*pageSize
* @param pageSize 每页展示多少条 dao size = service pageSize
* @return
* @throws Exception
*/
public List<UserInfo> findUserByPageList(Integer pageIndex,Integer pageSize)throws Exception;
/**
* 按ID查询
* @param uid
* @return
* @throws Exception
*/
public UserInfo findUserByID(Integer uid)throws Exception;
/**
* 添加
* @param user
* @return
* @throws Exception
*/
public int insertUser(UserInfo user)throws Exception;
/**
* 更新
* @param user
* @return
* @throws Exception
*/
public int updateUser(UserInfo user)throws Exception;
/**
* 删除
* @param user
* @return
* @throws Exception
*/
public int deleteUser(UserInfo user)throws Exception;
}
Service层接口实现类
package com.hnxy.service.impl;
import java.util.List;
import com.hnxy.dao.UserDAO;
import com.hnxy.entity.UserInfo;
import com.hnxy.service.UserService;
/**
* 业务层实现类
* @author My
*
*/
public class UserServiceImpl implements UserService {
// 创建DAO层接口
private UserDAO userDAO;
public void setUserDAO(UserDAO userDAO) {
this.userDAO = userDAO;
}
public int findUserByPageListCount() throws Exception {
return userDAO.findUserByPageListCount();
}
public List<UserInfo> findUserByPageList(Integer pageIndex, Integer pageSize) throws Exception {
List<UserInfo> list = null;
if(null != pageIndex && null != pageSize){
if(pageIndex > 0 && pageSize > 0){
list = userDAO.findUserByPageList((pageIndex-1)*pageSize, pageSize);
}
}
// 返回
return list;
}
public UserInfo findUserByID(Integer uid) throws Exception {
return userDAO.findUserByID(uid);
}
public int insertUser(UserInfo user) throws Exception {
return userDAO.insertUser(user);
}
public int updateUser(UserInfo user) throws Exception {
return userDAO.updateUser(user);
}
public int deleteUser(UserInfo user) throws Exception {
return userDAO.deleteUser(user);
}
}
SpringIOC实现
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 需要一个数据源对象 -->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://127.0.0.1:3306/userdb?characterEncoding=UTF-8" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<!-- 创建SQL的执行对象 -->
<bean id="qr" class="org.apache.commons.dbutils.QueryRunner" scope="prototype">
<!-- 构造函数创建对象 -->
<constructor-arg name="ds" ref="dataSource" />
</bean>
<!-- 创建一个DAO层对象 -->
<bean id="userDAO" class="com.hnxy.dao.impl.UserDAOImpl">
<property name="qr" ref="qr" />
</bean>
<!-- 创建一个Service层对象 -->
<bean id="userService" class="com.hnxy.service.impl.UserServiceImpl">
<property name="userDAO" ref="userDAO" />
</bean>
</beans>
测试类
package com.hnxy.test;
import java.util.Date;
import java.util.List;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.hnxy.entity.UserInfo;
import com.hnxy.service.UserService;
public class UserTest {
// 创建spring配置文件读取对象
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
// 根据类型获取业务层对象
UserService service = context.getBean(UserService.class);
public void addTest()throws Exception{
// 添加
UserInfo user = new UserInfo();
user.setUname("陈丽娟");
user.setRegDate(new Date());
user.setMoney(0.01);
int count = service.insertUser(user);
System.out.println(count);
}
public void updateTest()throws Exception{
// 修改ID为100的数据 金额改成50000
UserInfo user = service.findUserByID(100);
if(null != user){
user.setMoney(50000D);
int count = service.updateUser(user);
System.out.println(count);
}else{
System.out.println("没找到!");
}
}
public void deleteTest()throws Exception{
// 删除ID为99的数据
UserInfo user = service.findUserByID(99);
if(null != user){
int count = service.deleteUser(user);
System.out.println(count);
}else{
System.out.println("没找到!");
}
}
@Test
public void queryTest()throws Exception{
// 分页展示
int pageIndex = 2;
int pageSize = 10;
int totalCount = service.findUserByPageListCount();
// 计算总页数
int totalPgae = totalCount%pageSize==0?totalCount/pageSize:totalCount/pageSize+1;
System.out.println("总条数:"+totalCount+"总页数:"+totalPgae);
// 分页展示
List<UserInfo> list = service.findUserByPageList(pageIndex, pageSize);
// 展示
for (UserInfo userInfo : list) {
System.out.println(userInfo);
}
}
}