周一到周五,每天一篇,北京时间早上7点准时更新~
跟着我们一起一天天进步,一步一步变成业界大佬。当别人在炫技术的时候,我们来手把手教你那些技术怎么实现。
As images represent large regions of memory and we have just explained how to write directly into images from your shaders, you may have guessed that we’ll now explain the memory barrier types that you can use to synchronize access to that memory. Just as with buffers and atomic counters, you can call
一个图片代表的是一大片的内存区域,我们之前已经讲过如何从shader中往图片里写数据了,你可能已经猜到了,我们现在开始要讲解关于这部分内容的memory barrier了。就跟buffer object和atomic counter一样, 你可以调用:
glMemoryBarrier(GL_SHADER_IMAGE_ACCESS_BIT);
You should call glMemoryBarrier() with the GL_SHADER_IMAGE_ACCESS_BIT set when something has written to an image that you want read from images later—including other shaders.Similarly, a version of the GLSL memoryBarrier() function, memoryBarrierImage(), ensures that operations on images from inside your shader are completed before it returns.
这里,你应该在调用该传入的参数是GL_SHADER_IMAGE_ACCESS_BIT来保证你先写入数据,然后后面的其他逻辑才能开始读取。同样的,在glsl中,也有对应版本的memoryBarrier函数,memoryBarrierImage()保证了你在 shader中对图片的操作完成了之后才返回。
Texture Compression(纹理压缩)
Textures can take up an incredible amount of space. Modern games can easily use a gigabyte or more of texture data in a single level. That’s a lot of data! Where do you put it all? Textures are an important part of making rich, realistic, and impressive scenes, but if you can’t load all of the data onto the GPU, your rendering will be slow, if not impossible. One way to deal with storing and using a large amount of texture data is to compress the data. Compressed textures have two major benefits. First, they reduce the amount of storage space required for image data. Although the texture formats supported by OpenGL are generally not compressed as aggressively as in formats such as JPEG, they do provide substantial space benefits. The second (and possibly more important) benefit is that, because the graphics processor needs to read less data when fetching from a compressed texture, less memory bandwidth is required when compressed textures are used.
纹理可能会占用相当一部分的存储空间。很多游戏很容易就使用超过1GB甚至更多的图片数据。纹理是使得你的场景变得更丰富、更加的真实和有带入感的手段,如果你不能把所有的纹理都放到GPU上去,那么这样势必会 影响你的渲染速度。一个可以用来减少纹理图片存储空间的做法就是使用压缩数据。压缩纹理数据主要有两个方面的好处,第一他们可以减少存储空间,第二因为在纹理采样的时候,GPU可以读取更少的数据,这样可以减小 数据总线带宽的压力。
OpenGL supports a number of compressed texture formats. All OpenGL implementations support at least the compression schemes listed in Table 5.13.
OpenGL支持很多压缩纹理的格式,表5.13中展示了所有的OpenGL实现中最少需要支持的压缩纹理的格式清单。
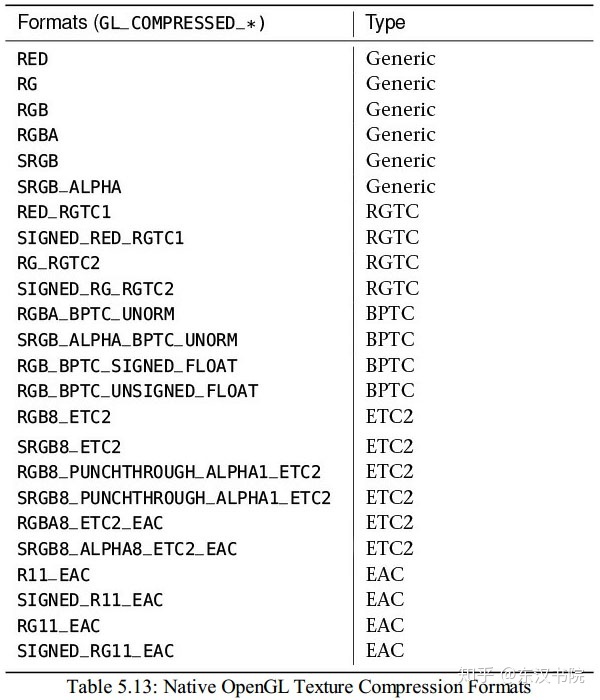
The first six formats listed in Table 5.13 are generic and allow the OpenGL driver to decide which compression mechanism to use. As a result, your driver can use the format that best suits the current conditions. The catch is that the choice is implementation specific; although your code will work on many platforms, the result of rendering with them might not be the same.
表5.13中的前6个格式是通用的,并且可以允许OpenGL的驱动去决定使用哪一个压缩的策略。所以,你的驱动可以选择当前状态下,最好的一种格式。所以这是由具体的GPU厂商的实现决定的,尽管你的代码可以在很多平台 上跑,但是运行出来的结果不一定完全一样。
The RGTC (Red–Green Texture Compression) format breaks a texture image into 4 × 4 texel blocks, compressing the individual channels within each block using a series of codes. This compression mode works only for one- and two-channel signed and unsigned textures, and only for certain texel formats. You don’t need to worry about the exact compression scheme unless you are planning on writing a compressor. Just note that the space savings from using RGTC is 50%.
RGTC格式会讲一个图片干成4x4的像素小块,每一个小块为单位去进行数据的压缩。这种压缩格式只能适用于一个通道或者2个通道的有符号或者无符号数据类型的图片,并且只能适用于一些特定的像素格式。 你不需要担心具体的压缩算法,你只需要知道的是这种格式可以减少50%的内存开销。
The BPTC (Block Partitioned Texture Compression) format also breaks textures up into blocks of 4 × 4 texels, each represented as 128 bits (16 bytes) of data in memory. The blocks are encoded using a rather complex scheme that essentially comprises a pair of endpoints and a representation of the position on a line between those two endpoints. It allows the endpoints to be manipulated to generate a variety of values as output for each texel. The BPTC formats are capable of compressing 8-bit per-channel normalized data and 32-bit per-channel floating-point data. The compression ratio for BPTC formats ranges from 25% for RGBA floating-point data to 33% for RGB 8-bit data.
BPTC格式会把纹理干成一些4x4的像素块,每个像素块占128比特。这些数据块使用了非常复杂的编码算法,主要是压缩两端的点并且用两端的点去计算端点之间连线上的位置。它允许使用端点数据去计算一系列的值来作为 纹理像素的值。BPTC格式可以适用于每个通道8比特以及每个通道32比特的浮点数据的压缩。对于32位的RGBA浮点数据的图片,该算法的压缩率为25%,对于8比特的RGB数据来说,压缩率为33%。
Ericsson Texture Compression (ETC2) and Ericsson Alpha Compression (EAC)6 are low-bandwidth formats that are also available in OpenGL ES 3.0. They are designed for extremely low bit-per-pixel applications such as those found in mobile devices, which have substantially less memory bandwidth than the high-performance GPUs found in desktop and workstation computers.
ETC2和EAC格式是低带宽格式,OpenGLES3.0中也可用。它们主要是为了移动设备而设计的,它们消耗的带宽比较小,所以表现出了非常好的性能。
Your implementation may also support other compressed formats, such as S3TC8 and ETC1. You should check for the availability of formats not required by OpenGL before attempting to use them. The best way to do so is to check for support of the related extension. For example, if your implementation of OpenGL supports the S3TC format, it will advertise the GL_EXT_texture_compression_s3tc extension string.
你的GPU或许也支持S3TC8和ETC1格式。你应该先检查一下是否支持这样的格式,然后再去使用。最好的检查是否支持该格式的方式就是去检查相关的扩展是否存在。比如果如果你的GPU支持S3TC,那么你的扩展格式中会出现 GL_EXT_texture_compression_s3tc这个字符串。
本日的翻译就到这里,明天见,拜拜~~
第一时间获取最新桥段,请关注东汉书院以及图形之心公众号
东汉书院,等你来玩哦