笔者在做硬件工程师的工作过程中,发现对于EMC工程师来说,为了滤掉高频辐射信号,常常会使用无源的低通滤波器,其中包括RC低通滤波和LC低通滤波。
- RC低通滤波
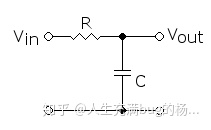
其截止频率的计算公式为:
2. LC低通滤波
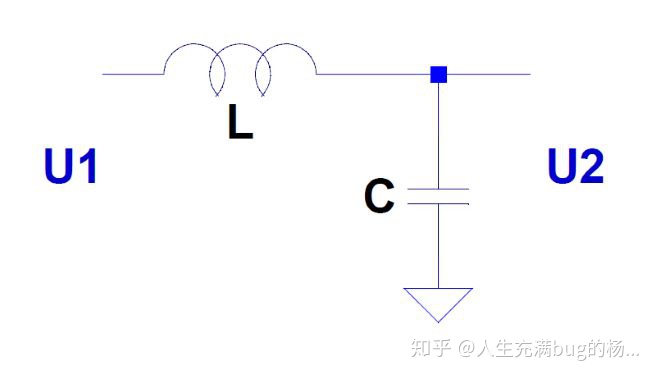
其截止频率的计算公式为:
对于高频信号(例如1GMHz以上的)来讲,常常需要使用到各种pF,nH级别的电容电感进行无源滤波,有绝对值很大的指数,但是电脑自带的计算器有比较呆板,所以常常会有计算错误的情况发生,于是笔者就旨在设计一款专门运用于高频无源滤波调试的开源脚本来进行计算RLC的参数:
3. RC滤波计算界面脚本
# RC_Filter
import tkinter as tk
import tkinter.messagebox
import math
window = tk.Tk()
window.title('RC Filter Calculator')
window.geometry('450x300')
#Var Definition
R_insert_var=tk.DoubleVar()
C_insert_var=tk.DoubleVar()
F_insert_var=tk.DoubleVar()
#function
#You know C and cutoff frequency,so you want to calculate R.
def calculate_R():
C_insert=C_insert_var.get()
F_insert=F_insert_var.get()
if C_insert>0 and F_insert>0:
R_calculation=float('%0.4f'%(1/(2*math.pi*F_insert*C_insert)*1e6));
tk.messagebox.showinfo(title='Result',message='You need a {} Ω resistor'.format(R_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of capacity and goal frequency.');
#You know R and cutoff frequency,so you want to calculate C.
def calculate_C():
R_insert=R_insert_var.get()
F_insert=F_insert_var.get()
if R_insert>0 and F_insert>0:
C_calculation=float('%0.4f'%(1/(2*math.pi*F_insert*R_insert)*1e6));
tk.messagebox.showinfo(title='Result',message='You need a {} pF capacitor'.format(C_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of resistance and goal frequency.');
#You know L and C,so you want to calculate cutoff frequency.
def calculate_F():
R_insert=R_insert_var.get()
C_insert=C_insert_var.get()
if R_insert>0 and C_insert>0:
F_calculation=float('%0.4f'%(1/(2*math.pi*C_insert*R_insert)*1e6));
tk.messagebox.showinfo(title='Result',message='The cutoff frequency calculation result is {} MHz'.format(F_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of resistancen and capacity.');
#Widget pack
l_R = tk.Label(window, text='Please insert the resistance value', font=('Arial', 12), width=30, height=2)
l_R.place(x=-5,y=20,anchor="nw")
R_insert=tk.Entry(window,textvariable=R_insert_var)
R_insert.place(x=255,y=30)
l_C = tk.Label(window, text='Please insert the capacity value', font=('Arial', 12), width=30, height=2)
l_C.place(x=-5,y=80,anchor="nw")
C_insert=tk.Entry(window,textvariable=C_insert_var)
C_insert.place(x=255,y=90)
l_F = tk.Label(window, text='Please insert the goal frequency', font=('Arial', 12), width=30, height=2)
l_F.place(x=-5,y=140,anchor="nw")
F_insert=tk.Entry(window,textvariable=F_insert_var)
F_insert.place(x=255,y=150)
b_R=tk.Button(window,text='Calculate R',command=calculate_R)
b_R.place(x=60,y=200,anchor="nw")
b_C=tk.Button(window,text='Calculate C',command=calculate_C)
b_C.place(x=160,y=200,anchor="nw")
b_F=tk.Button(window,text='Calculate Frequency',command=calculate_F)
b_F.place(x=260,y=200,anchor="nw")
l_ohm = tk.Label(window, text='Ω', font=('Arial', 12), width=5, height=2)
l_ohm.place(x=380,y=20,anchor="nw")
l_pf = tk.Label(window, text='pf', font=('Arial', 12), width=5, height=2)
l_pf.place(x=380,y=80,anchor="nw")
l_MHz = tk.Label(window, text='MHz', font=('Arial', 12), width=5, height=2)
l_MHz.place(x=380,y=140,anchor="nw")
window.mainloop()
4. LC滤波计算界面脚本
#LC_Filter
import tkinter as tk
import tkinter.messagebox
from math import*
window = tk.Tk()
window.title('LC Filter Calculator')
window.geometry('450x300')
#Var Definition
L_insert_var=tk.DoubleVar()
C_insert_var=tk.DoubleVar()
F_insert_var=tk.DoubleVar()
#function
def calculate_L():
C_insert=C_insert_var.get()
F_insert=F_insert_var.get()
if C_insert>0 and F_insert>0:
L_calculation=pow(1/(2*pi*sqrt(C_insert)*F_insert),2)*1e9
L_calculation=float('%0.4f'%L_calculation);
tk.messagebox.showinfo(title='Result',message='You need a {} nH inductor'.format(L_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of capacity and goal frequency.');
def calculate_C():
L_insert=L_insert_var.get()
F_insert=F_insert_var.get()
if L_insert>0 and F_insert>0:
C_calculation=pow(1/(2*pi*sqrt(L_insert)*F_insert),2)*1e9
C_calculation=float('%0.4f'%C_calculation);
tk.messagebox.showinfo(title='Result',message='You need a {} pF capacitor'.format(C_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of inductance and goal frequency.');
def calculate_F():
L_insert=L_insert_var.get()
C_insert=C_insert_var.get()
if L_insert>0 and C_insert>0:
F_calculation=1/(2*pi*sqrt(L_insert*1e-9*C_insert*1e-12)*1e6)
F_calculation=float('%0.4f'% F_calculation);
tk.messagebox.showinfo(title='Result',message='The cutoff frequency calculation result is {} MHz'.format(F_calculation))
else:
tk.messagebox.showerror(title='Error',message=' Please insert valid value of inductance and capacity.');
#Widget pack
l_L = tk.Label(window, text='Please insert the inductance value', font=('Arial', 12), width=30, height=2)
l_L.place(x=-5,y=20,anchor="nw")
L_insert=tk.Entry(window,textvariable=L_insert_var)
L_insert.place(x=255,y=30)
l_C = tk.Label(window, text='Please insert the capacity value', font=('Arial', 12), width=30, height=2)
l_C.place(x=-5,y=80,anchor="nw")
C_insert=tk.Entry(window,textvariable=C_insert_var)
C_insert.place(x=255,y=90)
l_F = tk.Label(window, text='Please insert the goal frequency', font=('Arial', 12), width=30, height=2)
l_F.place(x=-5,y=140,anchor="nw")
F_insert=tk.Entry(window,textvariable=F_insert_var)
F_insert.place(x=255,y=150)
b_L=tk.Button(window,text='Calculate L',command=calculate_L)
b_L.place(x=60,y=200,anchor="nw")
b_C=tk.Button(window,text='Calculate C',command=calculate_C)
b_C.place(x=160,y=200,anchor="nw")
b_F=tk.Button(window,text='Calculate Frequency',command=calculate_F)
b_F.place(x=260,y=200,anchor="nw")
l_nH = tk.Label(window, text='nH', font=('Arial', 12), width=5, height=2)
l_nH.place(x=380,y=20,anchor="nw")
l_pf = tk.Label(window, text='pf', font=('Arial', 12), width=5, height=2)
l_pf.place(x=380,y=80,anchor="nw")
l_MHz = tk.Label(window, text='MHz', font=('Arial', 12), width=5, height=2)
l_MHz.place(x=380,y=140,anchor="nw")
window.mainloop()
5. 界面展示
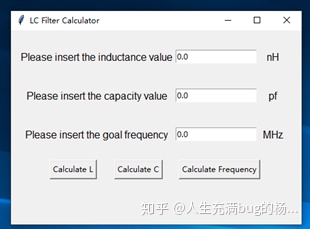
如图,当你需要滤波的通路上已经有68nH的电感,你需要滤除掉300MHz的噪声,这个时候你就可以输入你设定的值,然后点击“Calculate C” 就会得到一个结果如图所示:
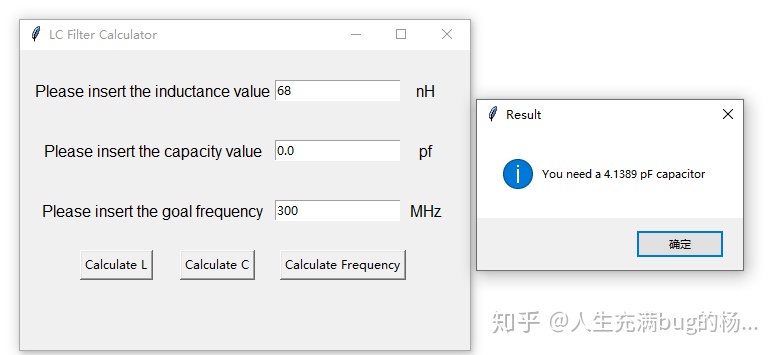
同理可运用在RC滤波计算。