ASE用Python面向对象编写的程序接口,内置了过渡态搜索、声子谱等功能,可以外接CP2K、VASP、Gaussian等软件进行能量计算。
官网对它的设计目标有着如下介绍:
· Easy to use:
Setting up an atomistic total energy calculation or molecular dynamics simulation with ASE is simple and straightforward. ASE can be used via a graphical user interface, Command line tool and the Python language. Python scripts are easy to follow (see What is Python? for a short introduction). It is simple for new users to get access to all of the functionality of ASE.
· Flexible:
Since ASE is based on the Python scripting language it is possible to perform very complicated simulation tasks without any code modifications. For example, a sequence of calculations may be performed with the use of simple “for-loop” constructions. There exist ASE modules for performing many standard simulation tasks.
· Customizable:
The Python code in ASE is structured in modules intended for different purposes. There are ase.calculators for calculating energies, forces and stresses, ase.md and ase.optimize modules for controlling the motion of atoms, constraints objects and filters for performing nudged-elastic-band calculations etc. The modularity of the object-oriented code make it simple to contribute new functionality to ASE.
· Pythonic:
It fits nicely into the rest of the Python world with use of the popular NumPy package for numerical work (see Numeric arrays in Python for a short introduction). The use of the Python language allows ASE to be used both interactively as well as in scripts.
· Open to participation:
The CAMPOS Atomic Simulation Environment is released under the GNU Lesser General Public License version 2.1 or any later version. See the files COPYING and COPYING.LESSER which accompany the downloaded files, or see the license at GNU’s web server at http://www.gnu.org/licenses/. Everybody is invited to participate in using and developing the code
在Windows下,下载ase-3.17.0-py3-none-any.whl,通过pip安装。
在linux下,建议安装Anaconda,下载解压,python setup.py,安装时保持网络连接
vi .bash_rc 添加Python和ASE环境变量:
export PYTHONPATH=<path-to-ase-package>:$PYTHONPATH
export PATH=<path-to-ase-command-line-tools>:$PATH
使用VASP还需要添加VASP和赝势的环境变量:
export ASE_VASP_COMMAND="mpiexec vasp_std"
export VASP_PP_PATH=$HOME/vasp/mypps
mypps目录下应该有三个文件夹:
The pseudopotentials are expected to be in:
LDA: $VASP_PP_PATH/potpaw/
PBE: $VASP_PP_PATH/potpaw_PBE/
PW91: $VASP_PP_PATH/potpaw_GGA/
ASE内置有Effective Medium Theory (EMT)模型,可以快速地计算能量。
A semi-empirical effective medium theory for metals and alloyswww.sciencedirect.com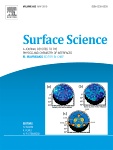
安装好后运行命令"ase test"测试安装包的结果
运行一下Tutorials中的一些例子,官网给定基本上都是emt的例子。
1.计算N原子在Cu(111)表面的吸附能,测试内置的emt能否运行
from ase import Atoms
from ase.calculators.emt import EMT
from ase.constraints import FixAtoms
from ase.optimize import QuasiNewton
from ase.build import fcc111, add_adsorbate
h = 1.85
d = 1.10
slab = fcc111('Cu', size=(4, 4, 2), vacuum=10.0)
slab.set_calculator(EMT()) #设置计算方法为EMT
e_slab = slab.get_potential_energy()
molecule = Atoms('2N', positions=[(0., 0., 0.), (0., 0., d)])
molecule.set_calculator(EMT())
e_N2 = molecule.get_potential_energy()
add_adsorbate(slab, molecule, h, 'ontop')
constraint = FixAtoms(mask=[a.symbol != 'N' for a in slab])
slab.set_constraint(constraint)
dyn = QuasiNewton(slab, trajectory='N2Cu.traj')
dyn.run(fmax=0.05)
print('Adsorption energy:', e_slab + e_N2 - slab.get_potential_energy())
2. VASP计算带隙
from ase.build import bulk
from ase.calculators.vasp import Vasp2
import os
si = bulk("Cu")
mydir = 'bandstructure' # Directory where we will do the calculations
# Make self-consistent ground state
calc = Vasp2(kpts=(8, 8, 8),pp='PBE',directory=mydir)
si.set_calculator(calc)
si.get_potential_energy() # Run the calculation
# Non-SC calculation along band path
kpts = {'path': 'WGX', # The BS path
'npoints': 30} # Number of points along the path
calc.set(isym=0, # Turn off kpoint symmetry reduction
icharg=11, # Non-SC calculation
kpts=kpts)
# Run the calculation
si.get_potential_energy()
# Load the calculator from the VASP output files
calc_load = Vasp2(restart=True, directory=mydir)