文章目录
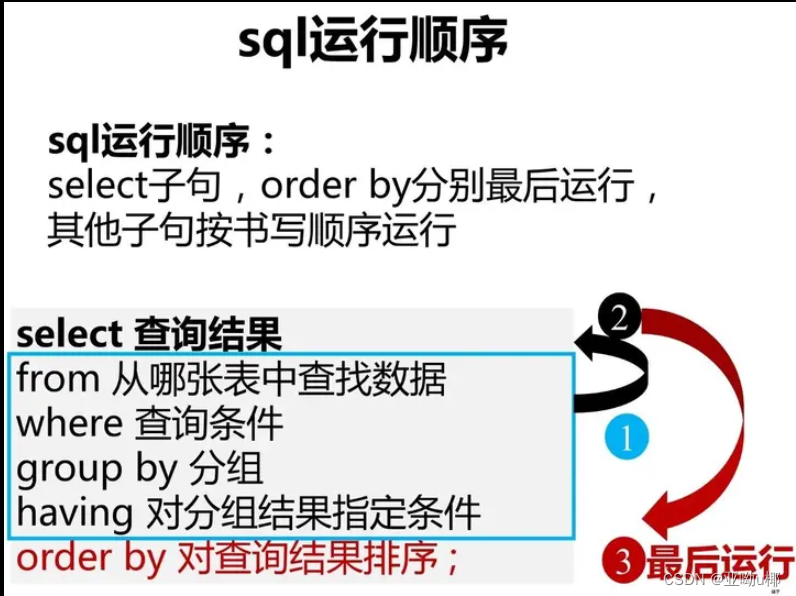
1. SELECT from world
- Read the notes about this table. Observe the result of running this SQL command to show the name, continent and population of all countries.
SELECT name, continent, population FROM world;
- How to use WHERE to filter records. Show the name for the countries that have a population of at least 200 million. 200 million is 200000000, there are eight zeros.
SELECT name FROM world
WHERE population >= 200000000;
- Give the name and the per capita GDP for those countries with a population of at least 200 million.
SELECT name, gdp/population
FROM world
WHERE population >= 200000000;
- Show the name and population in millions for the countries of the continent ‘South America’. Divide the population by 1000000 to get population in millions.
SELECT name, population/1000000
FROM world
WHERE continent = "South America";
- Show the name and population for France, Germany, Italy
SELECT name, population
FROM world
WHERE name IN ("France", "Germany", "Italy");
- Show the countries which have a name that includes the word ‘United’
SELECT name FROM world
WHERE name LIKE "%United%";
- Two ways to be big: A country is big if it has an area of more than 3 million sq km or it has a population of more than 250 million.
Show the countries that are big by area or big by population. Show name, population and area.
SELECT name, population, area
FROM world
WHERE population > 250000000 OR area > 3000000;
- Exclusive OR (XOR). Show the countries that are big by area (more than 3 million) or big by population (more than 250 million) but not both. Show name, population and area.
- Australia has a big area but a small population, it should be included.
- Indonesia has a big population but a small area, it should be included.
- China has a big population and big area, it should be excluded.
- United Kingdom has a small population and a small area, it should be excluded.
SELECT name, population, area
FROM world
WHERE population > 250000000 XOR area > 3000000;
- Show the name and population in millions and the GDP in billions for the countries of the continent ‘South America’. Use the ROUND function to show the values to two decimal places.
For South America show population in millions and GDP in billions both to 2 decimal places.
SELECT name, ROUND(population/1000000,2), ROUND(gdp/1000000000,2)
FROM world
WHERE continent = "South America";
- Show the name and per-capita GDP for those countries with a GDP of at least one trillion (1000000000000; that is 12 zeros). Round this value to the nearest 1000.
Show per-capita GDP for the trillion dollar countries to the nearest $1000.
SELECT name, ROUND(gdp/population,-3)
FROM world
WHERE gdp > 1000000000000;
- Greece has capital Athens.
Each of the strings ‘Greece’, and ‘Athens’ has 6 characters.
Show the name and capital where the name and the capital have the same number of characters.
- You can use the LENGTH function to find the number of characters in a string
SELECT name, capital
FROM world
WHERE LENGTH(capital) = LENGTH(name);
- The capital of Sweden is Stockholm. Both words start with the letter ‘S’.
Show the name and the capital where the first letters of each match. Don’t include countries where the name and the capital are the same word.
- You can use the function LEFT to isolate the first character.
- You can use <> as the NOT EQUALS operator.
SELECT name, capital
FROM world
WHERE LEFT(name,1)=LEFT(capital,1) and name<>capital;
- Equatorial Guinea and Dominican Republic have all of the vowels (a e i o u) in the name. They don’t count because they have more than one word in the name.
Find the country that has all the vowels and no spaces in its name.
- You can use the phrase name NOT LIKE
'%a%'
to exclude characters from your results. - The query shown misses countries like Bahamas and Belarus because they contain at least one ‘a’.
SELECT name
FROM world
WHERE name NOT LIKE "% %" AND name LIKE "%a%" AND name LIKE '%o%'
AND name LIKE '%e%' AND name LIKE '%i%' AND name LIKE '%u%';
2. SELECT from Nobel Tutorial
- Change the query shown so that it displays Nobel prizes for 1950.
SELECT yr, subject, winner
FROM nobel
WHERE yr = 1950;
- Show who won the 1962 prize for Literature.
SELECT winner
FROM nobel
WHERE yr = 1962 AND subject = 'Literature';
- Show the year and subject that won ‘Albert Einstein’ his prize.
SELECT yr, subject
FROM nobel
WHERE winner = "Albert Einstein";
- Give the name of the ‘Peace’ winners since the year 2000, including 2000.
SELECT winner
FROM nobel
WHERE yr >= 2000 AND subject = "Peace";
- Show all details (yr, subject, winner) of the Literature prize winners for 1980 to 1989 inclusive.
// 方法1:
SELECT yr, subject, winner
FROM nobel
WHERE yr BETWEEN 1980 AND 1989 AND subject = "Literature";
// 方法2:
SELECT yr, subject, winner
FROM nobel
WHERE yr>=1980 AND yr<=1989 AND subject = "Literature";
- Show all details of the presidential winners:
- Theodore Roosevelt
- Woodrow Wilson
- Jimmy Carter
- Barack Obama
SELECT *
FROM nobel
WHERE winner in ("Theodore Roosevelt","Woodrow Wilson","Jimmy Carter","Barack Obama");
- Show the winners with first name John.
SELECT winner
FROM nobel
WHERE winner LIKE "John %";
- Show the year, subject, and name of Physics winners for 1980 together with the Chemistry winners for 1984.
SELECT yr, subject, winner
FROM nobel
WHERE (subject="Physics" AND yr=1980) OR (subject="Chemistry" AND yr=1984);
- Show the year, subject, and name of winners for 1980 excluding Chemistry and Medicine.
SELECT yr, subject, winner
FROM nobel
WHERE yr=1980 AND subject<>"Chemistry" AND subject<>"Medicine";
- Show year, subject, and name of people who won a ‘Medicine’ prize in an early year (before 1910, not including 1910) together with winners of a ‘Literature’ prize in a later year (after 2004, including 2004)
SELECT yr, subject, winner
FROM nobel
WHERE (subject="Medicine" AND yr<1910) OR (subject="Literature" AND yr>=2004);
- Find all details of the prize won by PETER GRÜNBERG
SELECT *
FROM nobel
WHERE winner LIKE "PETER GR_NBERG";
- Find all details of the prize won by EUGENE O’NEILL
SELECT *
FROM nobel
WHERE winner = "Eugene O'Neill";
- Knights in order
List the winners, year and subject where the winner starts with Sir. Show the the most recent first, then by name order.
SELECT winner, yr, subject
FROM nobel
WHERE winner LIKE "Sir%"
ORDER BY yr DESC, winner;
- The expression subject IN (‘Chemistry’,‘Physics’) can be used as a value - it will be 0 or 1.
Show the 1984 winners and subject ordered by subject and winner name; but list Chemistry and Physics last.
select winner, subject
from nobel
where yr=1984
order by subject in ('Chemistry','Physics'),subject,winner;
3. SELECT in SELECT
- List each country name where the population is larger than that of ‘Russia’.
select name
from world
where population>(select population
from world
where name='Russia');
- Show the countries in Europe with a per capita GDP greater than ‘United Kingdom’.
SELECT name FROM world
WHERE continent="Europe" and gdp/population > (SELECT gdp/population
FROM world WHERE name="United Kingdom");
- List the name and continent of countries in the continents containing either Argentina or Australia. Order by name of the country.
SELECT name, continent FROM world
WHERE continent in (SELECT continent FROM world
WHERE name IN("Argentina","Australia"))
ORDER BY name;
- Which country has a population that is more than Canada but less than Poland? Show the name and the population.
// 注意:sql中没有连续比较!!!
select name,population
from world
where population>(select population from world where name='Canada')
and population<(select population from world where name ='Poland');
- Germany (population 80 million) has the largest population of the countries in Europe. Austria (population 8.5 million) has 11% of the population of Germany.
Show the name and the population of each country in Europe. Show the population as a percentage of the population of Germany.
select name,
concat(round(population/(select population from world
where name='Germany')*100),'%')
from world
where continent='Europe';
- Which countries have a GDP greater than every country in Europe? [Give the name only.] (Some countries may have NULL gdp values)
SELECT name FROM world
WHERE gdp > ALL(SELECT gdp FROM world
WHERE continent="Europe" AND gdp>0);
【关联子查询】:https://zhuanlan.zhihu.com/p/41844742
7. Find the largest country (by area) in each continent, show the continent, the name and the area:
// 方法1:
select continent,name,area
from world as x
where x.area=(select max(y.area)
from world as y
where x.continent=y.continent);
// 方法2:
SELECT continent, name, area
FROM world
WHERE area IN (SELECT MAX(area)
FROM world
GROUP BY continent);
// 方法3:
select continent,name,area
from world as x
where x.area>=ALL(select y.area
from world as y
where x.continent=y.continent);
- List each continent and the name of the country that comes first alphabetically.
select continent,name
from world x
where x.name<=all(select name from world y
where x.continent=y.continent);
- Find the continents where all countries have a population <= 25000000. Then find the names of the countries associated with these continents. Show name, continent and population.
SELECT name, continent, population
FROM world w JOIN
(SELECT continent
FROM world
GROUP BY continent
HAVING max(pop) < 250000000
) c
ON w.continent = c.continent;
//方法2
SELECT name,continent,population
FROM world x
WHERE 25000000 >= ALL (SELECT population FROM world y
WHERE x.continent=y.continent AND y.population>0)
- Some countries have populations more than three times that of any of their neighbours (in the same continent). Give the countries and continents.
SELECT name, continent FROM world as x
WHERE population/3 > ALL(SELECT population FROM world as y
WHERE y.continent=x.continent AND x.name!=y.name);
4. SUM and COUNT
- Show the total population of the world.
SELECT SUM(population)
FROM world;
- List all the continents - just once each.
SELECT DISTINCT(continent)
FROM world
- Give the total GDP of Africa
SELECT SUM(gdp) FROM world
WHERE continent="Africa";
- How many countries have an area of at least 1000000
SELECT COUNT(name) FROM world
WHERE area >= 1000000;
- What is the total population of (‘Estonia’, ‘Latvia’, ‘Lithuania’)
SELECT SUM(population)
FROM world
WHERE name IN ('Estonia', 'Latvia', 'Lithuania');
- For each continent show the continent and number of countries.
SELECT continent, COUNT(name) FROM world
GROUP BY continent;
- For each continent show the continent and number of countries with populations of at least 10 million.
SELECT continent, COUNT(name)
FROM world
WHERE population >= 10000000
GROUP BY continent;
- List the continents that have a total population of at least 100 million.
SELECT continent
FROM world
GROUP BY continent
HAVING SUM(population) >= 100000000;
More Exercise
- Show the total number of prizes awarded.
nobel(yr, subject, winner)
SELECT COUNT(winner) FROM nobel;
- List each subject - just once
// 方法01:
SELECT subject
FROM nobel
GROUP BY subject;
// 方法02:
SELECT DISTINCT(subject)
FROM nobel
- Show the total number of prizes awarded for Physics.
nobel(yr, subject, winner)
SELECT COUNT(subject)
FROM nobel
WHERE subject="Physics";
- For each subject show the subject and the number of prizes.
SELECT subject, COUNT(subject) as num
FROM nobel
GROUP BY subject;
- For each subject show the first year that the prize was awarded.
SELECT subject, min(yr)
FROM nobel
GROUP BY subject;
- For each subject show the number of prizes awarded in the year 2000.
SELECT subject, COUNT(winner) AS num
FROM nobel
WHERE yr=2000
GROUP BY subject;
- Show the number of different winners for each subject.
SELECT subject, COUNT(DISTINCT(winner)) as num
FROM nobel
GROUP BY subject;
- For each subject show how many years have had prizes awarded.
SELECT subject, COUNT(DISTINCT(yr))
FROM nobel
GROUP BY subject;
- Show the years in which three prizes were given for Physics.
SELECT yr
FROM nobel
WHERE subject="Physics"
GROUP BY yr
HAVING COUNT(subject)=3;
- Show winners who have won more than once.
SELECT winner
FROM nobel
GROUP BY winner
HAVING COUNT(winner)>1;
- Show winners who have won more than one subject.
//区别第11题,当时做错了
SELECT winner
FROM nobel
GROUP BY winner
HAVING COUNT(DISTINCT(subject))>1;
- Show the year and subject where 3 prizes were given. Show only years 2000 onwards.
SELECT yr, subject
FROM nobel
WHERE yr>=2000
GROUP BY yr,subject
HAVING COUNT(winner)=3;
5. JOIN
- 几种join之间的关系图:
- The first example shows the goal scored by a player with the last name ‘Bender’. The * says to list all the columns in the table - a shorter way of saying matchid, teamid, player, gtime
Modify it to show the matchid and player name for all goals scored by Germany. To identify German players, check for: teamid = ‘GER’
SELECT matchid, player FROM goal
WHERE teamid="GER";
- From the previous query you can see that Lars Bender’s scored a goal in game 1012. Now we want to know what teams were playing in that match.
Notice in the that the column matchid in the goal table corresponds to the id column in the game table. We can look up information about game 1012 by finding that row in the game table.
Show id, stadium, team1, team2 for just game 1012.
SELECT id,stadium,team1,team2
FROM game
WHERE id=1012;
- Modify it to show the player, teamid, stadium and mdate for every German goal.
SELECT player, teamid, stadium, mdate
FROM game JOIN goal ON (id=matchid)
WHERE teamid = "GER";
- Use the same JOIN as in the previous question.
Show the team1, team2 and player for every goal scored by a player called Marioplayer LIKE 'Mario%'
.
//Method 1:
SELECT team1, team2, player
FROM goal, game
WHERE game.id = goal.matchid AND player LIKE "Mario%";
//Method 2:
SELECT ga.team1, ga.team2, go.player
FROM game ga JOIN goal go ON (ga.id=go.matchid)
WHERE go.player LIKE "Mario%";
- The table eteam gives details of every national team including the coach. You can JOIN goal to eteam using the phrase goal JOIN eteam on teamid=id.
Show player, teamid, coach, gtime for all goals scored in the first 10 minutes gtime<=10.
//Method 1:
SELECT player, teamid, coach, gtime
FROM goal, eteam
WHERE goal.teamid = eteam.id AND gtime<=10;
//Method 2:
SELECT player, teamid, coach, gtime
FROM goal g JOIN eteam e ON (e.id=g.teamid)
WHERE g.gtime<=10;
- To JOIN game with eteam you could use either
game JOIN eteam ON (team1=eteam.id) or game JOIN eteam ON (team2=eteam.id).
Notice that because id is a column name in both game and eteam you must specify eteam.id instead of just id.
List the the dates of the matches and the name of the team in which ‘Fernando Santos’ was the team1 coach.
SELECT mdate, teamname
FROM game JOIN eteam ON (game.team1=eteam.id)
WHERE coach = "Fernando Santos";
- List the player for every goal scored in a game where the stadium was ‘National Stadium, Warsaw’.
SELECT player
FROM goal JOIN game ON (game.id=goal.matchid)
WHERE stadium = "National Stadium, Warsaw";
- The example query shows all goals scored in the Germany-Greece quarterfinal.
Instead show the name of all players who scored a goal against Germany.
SELECT DISTINCT(player)
FROM goal JOIN game ON (goal.matchid=game.id)
WHERE teamid <> "GER" AND (team1="GER" OR team2="GER");
- Show teamname and the total number of goals scored.
SELECT teamname, COUNT(teamid)
FROM eteam JOIN goal ON (eteam.id=goal.teamid)
GROUP BY eteam.teamname;
- Show the stadium and the number of goals scored in each stadium.
SELECT stadium, COUNT(game.id) AS NumofGame
FROM game JOIN goal ON (game.id=goal.matchid)
GROUP BY game.stadium;
- For every match involving ‘POL’, show the matchid, date and the number of goals scored.
SELECT matchid, mdate, COUNT(teamid) num
FROM game JOIN goal ON (game.id=goal.matchid)
WHERE team1="POL" OR team2="POL"
GROUP BY matchid, mdate;
- For every match where ‘GER’ scored, show matchid, match date and the number of goals scored by ‘GER’.
SELECT matchid, mdate, COUNT(teamid) as num
FROM goal JOIN game ON (game.id=goal.matchid)
WHERE teamid="GER"
GROUP BY matchid;
List every match with the goals scored by each team as shown. This will use “CASE WHEN” which has not been explained in any previous exercises.
Notice in the query given every goal is listed. If it was a team1 goal then a 1 appears in score1, otherwise there is a 0. You could SUM this column to get a count of the goals scored by team1. Sort your result by mdate, matchid, team1 and team2.
SELECT mdate,
team1,
SUM(CASE WHEN team1 = teamid THEN 1 ELSE 0 END) AS score1,
team2,
SUM(CASE WHEN team2 = teamid THEN 1 ELSE 0 END) AS score2
FROM game a LEFT JOIN goal b ON a.id = b.matchid
GROUP BY mdate, team1,team2
ORDER BY mdate, matchid, team1, team2;
More Exercise
- 两张表是这样的:
How to do joins.
The phraseFROM album JOIN track ON album.asin=track.album
represents the join of the tablesalbum
andtrack
. This JOIN has one row for every track. In addition to the track fields (album
,disk
,posn
andsong
) it includes the details of the correspondingalbum
(title
,artist
…).
- Find the title and artist who recorded the song ‘Alison’.
SELECT a.title, a.artist
FROM album AS a JOIN track AS t ON (a.asin=t.album)
WHERE t.song="Alison";
- Which artist recorded the song ‘Exodus’?
SELECT a.artist
FROM album AS a JOIN track AS t ON (a.asin=t.album)
WHERE t.song="Exodus";
- Show the song for each track on the album ‘Blur’
SELECT song
FROM album AS a JOIN track AS t ON (a.asin=t.album)
WHERE t.album=(SELECT asin FROM album WHERE title="Blur");
- For each album show the title and the total number of track.
SELECT title, COUNT(*)
FROM album JOIN track ON (asin=album)
GROUP BY title;
- For each album show the title and the total number of tracks containing the word ‘Heart’ (albums with no such tracks need not be shown).
SELECT title, COUNT(*)
FROM album AS a JOIN track AS t ON (a.asin=t.album)
WHERE song LIKE "%Heart%"
GROUP BY title;
- A “title track” is where the song is the same as the title. Find the title tracks.
SELECT song
FROM album AS a JOIN track AS t ON (a.asin=t.album)
WHERE a.title=t.song;
- An “eponymous” album is one where the title is the same as the artist (for example the album ‘Blur’ by the band ‘Blur’). Show the eponymous albums.
SELECT title
FROM album
WHERE title=artist;
- Find the songs that appear on more than 2 albums. Include a count of the number of times each shows up.
//有时候对,有时候错
SELECT track.song, COUNT(album.title)
FROM album JOIN track ON album.asin = track.album
GROUP BY track.song
HAVING COUNT(DISTINCT album.title) > 2;
- A “good value” album is one where the price per track is less than 50 pence. Find the good value album - show the title, the price and the number of tracks.
SELECT album.title, album.price, COUNT(*) AS number_of_tracks
FROM album JOIN track ON album.asin = track.album
GROUP BY album.title
HAVING (album.price / COUNT(*)) < 0.5;
- Wagner’s Ring cycle has an imposing 173 tracks, Bing Crosby clocks up 101 tracks.
List albums so that the album with the most tracks is first. Show the title and the number of tracks.
Where two or more albums have the same number of tracks you should order alphabetically.
SELECT album.title, COUNT(*) AS number_of_tracks
FROM album JOIN track ON album.asin = track.album
GROUP BY album.asin
ORDER BY COUNT(*) DESC;
6. More JOIN operations
- List the films where the yr is 1962 [Show id, title].
SELECT id,title
FROM movie
WHERE yr=1962;
- Give year of ‘Citizen Kane’.
SELECT yr
FROM movie
WHERE title = "Citizen Kane";
- List all of the Star Trek movies, include the id, title and yr (all of these movies include the words Star Trek in the title). Order results by year.
SELECT id, title, yr
FROM movie
WHERE title LIKE "%Star Trek%"
ORDER BY yr;
- What id number does the actor ‘Glenn Close’ have?
SELECT id
FROM actor
WHERE name="Glenn Close";
- What is the id of the film ‘Casablanca’
SELECT id
FROM movie
WHERE title="Casablanca";
- Obtain the cast list for ‘Casablanca’.
what is a cast list?
The cast list is the names of the actors who were in the movie.
Use movieid=11768, (or whatever value you got from the previous question)
SELECT name
FROM casting JOIN actor ON (casting.actorid=actor.id)
WHERE casting.movieid=11768;
- Obtain the cast list for the film ‘Alien’
SELECT name
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE movie.title="Alien";
- List the films in which ‘Harrison Ford’ has appeared
SELECT title
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE actor.name="Harrison Ford";
- List the films where ‘Harrison Ford’ has appeared - but not in the starring role. [Note: the ord field of casting gives the position of the actor. If ord=1 then this actor is in the starring role].
SELECT title
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE casting.ord<>1 AND actor.name="Harrison Ford";
- List the films together with the leading star for all 1962 films.
SELECT title, name
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE movie.yr=1962 AND casting.ord=1;
- Which were the busiest years for ‘Rock Hudson’, show the year and the number of movies he made each year for any year in which he made more than 2 movies.
SELECT yr, COUNT(yr) as num
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE actor.name='Rock Hudson'
GROUP BY yr
HAVING COUNT(yr) > 2
ORDER BY num DESC;
- List the film title and the leading actor for all of the films ‘Julie Andrews’ played in.
SELECT title, name
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE casting.ord=1 AND movieid IN (SELECT movieid FROM casting JOIN actor ON (actorid=id) WHERE name = 'Julie Andrews');
- Obtain a list, in alphabetical order, of actors who’ve had at least 15 starring roles.
SELECT DISTINCT(actor.name) as actor
FROM movie
JOIN casting ON (movie.id=casting.movieid)
JOIN actor ON (casting.actorid=actor.id)
WHERE casting.ord=1
GROUP BY actor.name
HAVING COUNT(actor.name) >= 15
ORDER BY actor.name;
- List the films released in the year 1978 ordered by the number of actors in the cast, then by title.
SELECT movie.title, COUNT(casting.actorid)
FROM movie
JOIN casting ON (movie.id=casting.movieid)
WHERE movie.yr=1978
GROUP BY movie.title
ORDER BY COUNT(casting.actorid) DESC, title;
- List all the people who have worked with ‘Art Garfunkel’.
SELECT DISTINCT name FROM casting
JOIN actor ON actorid = actor.id
WHERE name != 'Art Garfunkel' AND movieid IN (
SELECT movieid
FROM movie
JOIN casting ON movieid = movie.id
JOIN actor ON actorid = actor.id
WHERE actor.name = 'Art Garfunkel'
);
7. Using NULL
Introduction to NULL:https://sqlzoo.net/wiki/Selecting_NULL_values.
- List the teachers who have NULL for their department.
Why we cannot use =
You might think that the phrasedept=NULL
would work here but it doesn’t - you can use the phrasedept IS NULL
SELECT name
FROM teacher
WHERE dept IS NULL;
- Note the INNER JOIN misses the teachers with no department and the departments with no teacher.
SELECT teacher.name, dept.name
FROM teacher INNER JOIN dept ON (teacher.dept=dept.id);
- Use a different JOIN so that all teachers are listed.
SELECT t.name, d.name
FROM teacher AS t LEFT JOIN dept AS d ON (t.dept=d.id);
- Use a different JOIN so that all departments are listed.
SELECT t.name, d.name
FROM teacher AS t RIGHT JOIN dept AS d ON (t.dept=d.id);
Using the COALESCE function: https://sqlzoo.net/wiki/COALESCE
- Use COALESCE to print the mobile number. Use the number ‘07986 444 2266’ if there is no number given. Show teacher name and mobile number or ‘07986 444 2266’
SELECT name, COALESCE(mobile, '07986 444 2266')
FROM teacher;
- Use the COALESCE function and a LEFT JOIN to print the teacher name and department name. Use the string ‘None’ where there is no department.
SELECT t.name, COALESCE(d.name, "None")
FROM teacher AS t LEFT JOIN dept AS d ON (t.dept=d.id);
- Use COUNT to show the number of teachers and the number of mobile phones.
SELECT COUNT(id), COUNT(mobile)
FROM teacher;
- Use COUNT and GROUP BY dept.name to show each department and the number of staff. Use a RIGHT JOIN to ensure that the Engineering department is listed.
SELECT dept.name ,COUNT(teacher.name)
FROM teacher RIGHT OUTER JOIN dept ON teacher.dept = dept.id
GROUP BY dept.name;
Using CASE: https://sqlzoo.net/wiki/CASE
- Use CASE to show the name of each teacher followed by ‘Sci’ if the teacher is in dept 1 or 2 and ‘Art’ otherwise.
SELECT teacher.name,
CASE
WHEN teacher.dept IN (1, 2)
THEN 'Sci'
ELSE 'Art'
END
FROM teacher;
- Use CASE to show the name of each teacher followed by ‘Sci’ if the teacher is in dept 1 or 2, show ‘Art’ if the teacher’s dept is 3 and ‘None’ otherwise.
SELECT teacher.name,
CASE
WHEN teacher.dept IN (1, 2)
THEN 'Sci'
WHEN teacher.dept = 3
THEN 'Art'
ELSE 'None'
END
FROM teacher;
8. Self JOIN
More details about database:
- How many stops are in the database.
SELECT COUNT(id)
FROM stops;
- Find the id value for the stop ‘Craiglockhart’
SELECT id
FROM stops
WHERE name='Craiglockhart';
- Give the id and the name for the stops on the ‘4’ ‘LRT’ service.
SELECT
stops.id,
stops.name
FROM
stops JOIN route ON (stops.id=route.stop)
WHERE
num = 4
AND route.company="LRT"
//Without ORDER BY, it will be correct but typographical error !!!
ORDER BY route.pos;
- The query shown gives the number of routes that visit either London Road (149) or Craiglockhart (53). Run the query and notice the two services that link these stops have a count of 2. Add a HAVING clause to restrict the output to these two routes.
SELECT
company,
num,
COUNT(*)
FROM
route
WHERE s
top=149 OR stop=53
GROUP BY
company, num
HAVING COUNT(*) = 2;
- Execute the self join shown and observe that b.stop gives all the places you can get to from Craiglockhart, without changing routes. Change the query so that it shows the services from Craiglockhart to London Road.
SELECT
a.company,
a.num,
a.stop,
b.stop
FROM route a
JOIN route b ON (a.company=b.company AND a.num=b.num)
WHERE
a.stop=53
AND b.stop = (SELECT id
FROM stops
WHERE name = 'London Road');
- The query shown is similar to the previous one, however by joining two copies of the stops table we can refer to stops by name rather than by number. Change the query so that the services between ‘Craiglockhart’ and ‘London Road’ are shown. If you are tired of these places try ‘Fairmilehead’ against ‘Tollcross’
SELECT
a.company,
a.num,
stopa.name,
stopb.name
FROM
route a
JOIN route b ON (a.company=b.company AND a.num=b.num)
JOIN stops as stopa ON (a.stop=stopa.id)
JOIN stops as stopb ON (b.stop=stopb.id)
WHERE
stopa.name='Craiglockhart'
and stopb.name = 'London Road';
- Give a list of all the services which connect stops 115 and 137 (‘Haymarket’ and ‘Leith’).
// Method 1:
SELECT
a.company,
a.num
FROM
route a
JOIN route b ON (a.num=b.num AND a.company=b.company)
WHERE
a.stop=115 AND b.stop=137
GROUP BY num;
// Method 2:
SELECT
a.company,
a.num
FROM
route a, route b
WHERE a.num = b.num AND (a.stop = 115 AND b.stop = 137)
GROUP BY num;
- Give a list of the services which connect the stops ‘Craiglockhart’ and ‘Tollcross’.
// Method 1:
SELECT
a.company,
a.num
FROM
route a
JOIN route b ON (a.company = b.company AND a.num = b.num)
JOIN stops stopa ON a.stop = stopa.id
JOIN stops stopb ON b.stop = stopb.id
WHERE
stopa.name = 'Craiglockhart'
AND stopb.name = 'Tollcross';
// Method 2:
SELECT
a.company,
a.num
FROM
route a
JOIN route b ON (a.num=b.num AND a.company=b.company)
WHERE
a.stop=(SELECT id FROM stops WHERE name="Craiglockhart")
AND b.stop=(SELECT id FROM stops WHERE name="Tollcross");
- Give a distinct list of the stops which may be reached from ‘Craiglockhart’ by taking one bus, including ‘Craiglockhart’ itself, offered by the LRT company. Include the company and bus no. of the relevant services.
SELECT
name,
a.company,
a.num
FROM
stops
JOIN route a ON (stops.id=a.stop)
JOIN route b ON (a.num=b.num AND a.company=b.company)
WHERE
b.stop=(SELECT id FROM stops WHERE name="Craiglockhart");
- 注意这种错误的问题!
- Find the routes involving two buses that can go from Craiglockhart to Lochend.
Show the bus no. and company for the first bus, the name of the stop for the transfer,and the bus no. and company for the second bus.
SELECT
a.num,
a.company,
stopb.name,
c.num,
c.company
FROM
route a
JOIN route b ON (a.company = b.company AND a.num = b.num)
JOIN (route c JOIN route d ON (c.company = d.company AND c.num = d.num))
JOIN stops stopa ON a.stop = stopa.id
JOIN stops stopb ON b.stop = stopb.id
JOIN stops stopc ON c.stop = stopc.id
JOIN stops stopd ON d.stop = stopd.id
WHERE
stopa.name = 'Craiglockhart'
AND stopd.name = 'Sighthill'
AND stopb.name = stopc.name
ORDER BY
LENGTH(a.num), b.num, stopb.name, LENGTH(c.num), d.num;
9. Window function
窗口函数的介绍-01: https://blog.csdn.net/qq_41805514/article/details/81772182
窗口函数的介绍-02: https://sqlite.org/windowfunctions.html
- Show the lastName, party and votes for the constituency ‘S14000024’ in 2017.
SELECT lastName, party, votes
FROM ge
WHERE constituency="S14000024" AND yr=2017
ORDER BY votes DESC;
- You can use the RANK function to see the order of the candidates. If you RANK using (ORDER BY votes DESC) then the candidate with the most votes has rank 1.
Show the party and RANK for constituency S14000024 in 2017. List the output by party.
// 注意:什么时候使用PARTITION BY!!!
SELECT party,
votes,
RANK() OVER (ORDER BY votes DESC) AS rank
FROM ge
WHERE constituency = 'S14000024' AND yr = 2017
GROUP BY party;
- 【partition by和group by的区别】:group by 和 partition by 都有分组统计的功能,但是partition by并不具有group by的汇总功能。partition by统计的每一条记录都存在,而group by将所有的记录汇总成一条记录(类似于distinct EmpDepartment 去重)。partition by可以和聚合函数结合使用,同时具有其他高级功能。(相关博客:https://blog.csdn.net/dwt1415403329/article/details/87835383)
- The 2015 election is a different PARTITION to the 2017 election. We only care about the order of votes for each year.
Use PARTITION to show the ranking of each party in S14000021 in each year. Include yr, party, votes and ranking (the party with the most votes is 1).
SELECT yr, party, votes,
RANK() OVER (PARTITION BY yr ORDER BY votes DESC) AS RANK
FROM ge
WHERE constituency = 'S14000021'
ORDER BY party, yr ASC;
- Edinburgh constituencies are numbered S14000021 to S14000026.
Use PARTITION BY constituency to show the ranking of each party in Edinburgh in 2017. Order your results so the winners are shown first, then ordered by constituency.
Submit SQLRestore default.
SELECT constituency, party, votes,
RANK() OVER (PARTITION BY constituency ORDER BY votes DESC) AS rank
FROM ge
WHERE constituency IN ('S14000021','S14000022','S14000023','S14000024','S14000025','S14000026') AND yr = 2017
ORDER BY rank, constituency ASC;
- You can use SELECT within SELECT to pick out only the winners in Edinburgh.
Show the parties that won for each Edinburgh constituency in 2017.
SELECT constituency,party
FROM (SELECT constituency,party, votes,
RANK() OVER (PARTITION BY constituency ORDER BY votes DESC) as posn
FROM ge
WHERE constituency BETWEEN 'S14000021' AND 'S14000026'
AND yr = 2017) AS pk
WHERE pk.posn=1;
- You can use COUNT and GROUP BY to see how each party did in Scotland. Scottish constituencies start with ‘S’.
Show how many seats for each party in Scotland in 2017.
SELECT party, COUNT(RANK)
FROM (SELECT constituency,party, votes,
RANK() OVER (PARTITION BY yr,constituency ORDER BY votes DESC) AS RANK
FROM ge
WHERE constituency LIKE 'S%'
AND yr = 2017
ORDER BY constituency, votes DESC) a
WHERE RANK = 1
GROUP BY party
ORDER BY party ASC;