/**/
//复数计算器
#include<iostream>
#include<cmath>
#include<string>
#include<fstream>
#include<ctime>
using namespace std;
#define EPS 1e-5//定义精度常数
namespace NameCComplex{//定义命名空间
struct User
{
string szName;//用户名
int nTime=0;//使用次数
int nTest=0;//测试次数
double dlAve;//平均成绩
int nAdd=0;//加法次数
int nSub=0;
int nMul=0;
double dlScore[3];//三次测试得分
}user;
class CComplex
{
friend istream& operator>>(istream &in, CComplex& com);//提取符重载
friend ostream& operator<<(ostream &out, CComplex& com);//插入符重载
public:
CComplex(double real = 0, double image = 0)
{
this->real = real; this->image = image;
}
CComplex& operator+ (CComplex& com) { return CComplex(real + com.real, image + com.image); }
CComplex& operator- (CComplex& com) { return CComplex(real - com.real, image - com.image); }
CComplex& operator* (CComplex& com) { return CComplex(real*com.real - image*com.image, real*com.image + image*com.real); }
CComplex& operator+= (CComplex& com)
{
real += com.real; image += com.image; return *this;
}
CComplex& operator-= (CComplex& com)
{
real -= com.real; image -= com.image; return *this;
}
CComplex& operator*= (CComplex& com)
{
double nreal = real*com.real - image*com.image;
double nimage = real*com.image + image*com.real;
real = nreal; image = nimage;
return *this;
}
CComplex& operator++ () { ++real; ++image; return *this; }//前置++
CComplex& operator-- () { --real; --image; return *this; }
CComplex& operator++ (int) { return CComplex(real++, image++); }//后置++
CComplex& operator-- (int){ return CComplex(real--, image--); }
double mod() { return sqrt(real*real + image*image); }
bool operator> (CComplex& com) { return mod() > com.mod(); }
bool operator< (CComplex& com) { return mod() < com.mod(); }
bool operator!= (CComplex& com) { return real != com.real||image != com.image; }
bool operator== (CComplex& com) { return real == com.real&&image == com.image; }
private:
double real, image;
};
ostream& operator<<(ostream &out, CComplex& com)//插入符重载
{
if (fabs(com.image) < EPS)//虚部为0
out << com.real;
else if (fabs(com.real) < EPS)//实部为0
out << com.image << "i";
else if (com.image > 0)
out << com.real << "+" << com.image << "i";
else
out << com.real << com.image << "i";
return out;
}
istream& operator >> (istream &in, CComplex& com)//提取符重载
{
cout << "输入复数:";
string s; cin >> s;
int n = 0, sign = 1;//n为从字符串中提取的数字,sign是n的符号
com.image = com.real = 0;
for (int i = 0; i < s.size(); i++)
{
if ((s[i] >= '0'&&s[i] <= '9') || (s[i] == '-') || (s[i] == '+') || (s[i] == 'i'));
else
{
cout << "error" << endl; return in;
}
}
for (int i = 0; i < s.size();)
{
if (s[i] == '-')
{
s
C++程序设计实践指导——第二章 样例讲解
最新推荐文章于 2022-07-06 09:01:43 发布
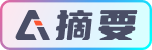