Dubbo是一款高性能、轻量级的开源java RPC框架,提供了三大核心能力:面向接口的远程方法调用、智能容错和负载均衡,以及服务自动注册和发现。
服务提供者创建过程:
- 创建mavenweb工程:服务的提供者
- 创建一个实体bean查询的结果,并且实现Serializable(Dubbo通信采用的二进制序列化)
- 提供一个服务接口xxxx
- 实现这个服务接口xxxxImpl
- 配置dubbo服务提供者的核心配置文件
- 声明dubbo服务提供者的名称:保证唯一性
- 声明dubbo使用的协议和端口号
- 暴露服务,使用直连方式
- 添加监听器
服务提供者代码结构:
User
public class User implements Serializable {
private Integer id;
private String username;
private Integer age;
...
}
UserServiceImpl
public class UserServiceImpl implements UserService {
@Override
public User queryUserById(Integer id) {
// 假装是从数据库查出来的
User user = new User();
user.setId(id);
user.setUsername("lisi");
user.setAge(23);
return user;
}
}
dubbo_userservice_provider.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://dubbo.apache.org/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd">
<!-- 服务提供者声明名称:必须保证服务名称的唯一性,它的名称是dubbo内部使用的唯一标识-->
<dubbo:application name="001-link-userservice-provider" />
<!-- 访问服务协议的名称及端口号,dubbo官方推荐使用的是dubbo协议,端口号默认为20880-->
<!-- name指定协议名称,port指定协议的端口号-->
<dubbo:protocol name="dubbo" port="20880"/>
<!-- 暴露服务接口->dubbo:service
interface:暴露服务接口的全限定名
ref:接口引用的实现类在Spring容器中的标识
registry:如果不是用注册中心,则值为N/A
-->
<dubbo:service interface="cn.youkee.service.UserService" ref="userService" registry="N/A"/>
<!-- 将接口的实现类加载到spring容器中-->
<bean id="userService" class="cn.youkee.service.Impl.UserServiceImpl" />
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:dubbo_userservice_provider.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app >
消费者为了知道提供者到底提供什么服务,就需要引入提供者的jar包,这样才能知道有什么方法可以调。所以需要先把提供者pom文件中的打包方式给注释掉(打包之后再去掉注释,因为后续还要打成war包放tomcat上),按jar的方式进行打包,打包之后在中央仓库就有对应的jar包(如图所示),然后消费者就可以在自己的pom文件中引入提供者的依赖。
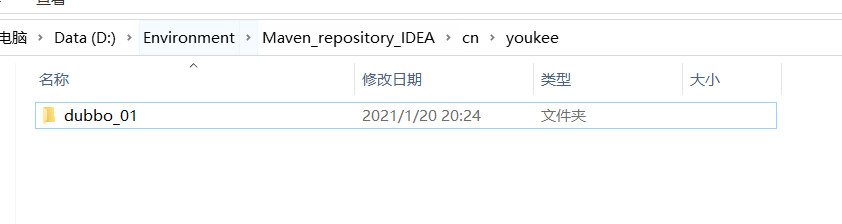
因为是在本地测试,所以配置提供者和消费者的tomcat的时候要注意端口问题,不然因为端口冲突,只有一个tomcat能启动。
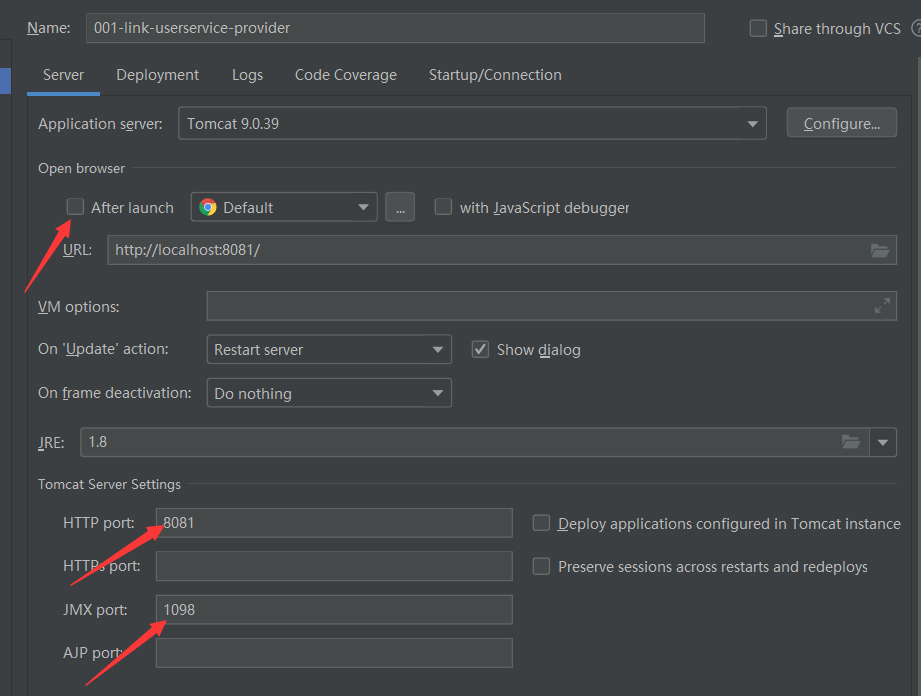
服务消费者创建过程:
- 创建mavenweb工程:服务的消费者
- 配置dubbo核心配置文件
- 编写controller
- 配置中央调度器(DispatcherServlet)
服务消费者代码结构:
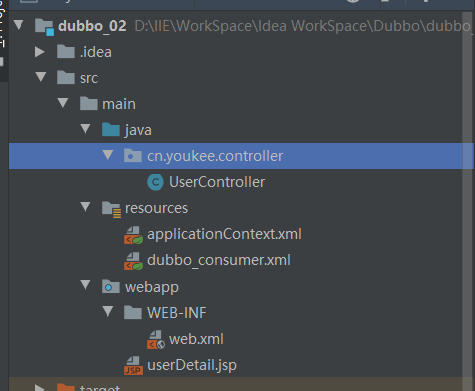
UserController
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping(value = "/user")
public String userDetail(Model model, Integer id) {
User user = userService.queryUserById(id);
model.addAttribute("user", user);
return "userDetail";
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 扫描组件-->
<context:component-scan base-package="cn.youkee.controller" />
<!-- 配置注解驱动-->
<mvc:annotation-driven />
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/" />
<property name="suffix" value=".jsp"/>
</bean>
</beans>
dubbo_consumer.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://dubbo.apache.org/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://dubbo.apache.org/schema/dubbo http://dubbo.apache.org/schema/dubbo/dubbo.xsd">
<!-- 声明服务消费者的名称:保证唯一性-->
<dubbo:application name="002-link-consumer"/>
<!--
引用远程服务接口:
id:远程服务接口对象的名称
interface:调用远程接口的全限定类名
url:访问服务接口的地址
registry:不是用注册中心,值为N/A
-->
<dubbo:reference id="userService"
interface="cn.youkee.service.UserService"
url="dubbo://localhost:20880"
registry="N/A"/>
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<servlet>
<servlet-name>dispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml, classpath:dubbo_consumer.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>dispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app >
userDetail.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>用户详情</title>
</head>
<body>
<h1>用户详情</h1>
<div>用户标识:${user.id}</div>
<div>用户姓名:${user.username}</div>
</body>
</html>
消费者的tomcat用默认的端口就好,不会冲突了。然后先启动服务提供者的tomcat,再启动服务消费者的tomcat,在浏览器访问user,记得带id,最后效果如图:
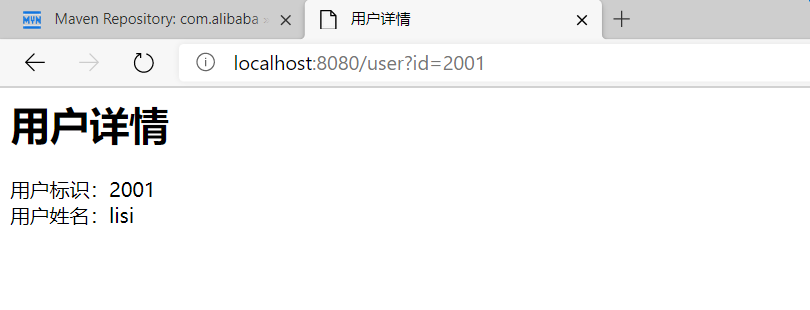
在控制台日志也可以看到dubbo的连接信息,因为是两台服务器都是在本地,所以ip都一样。
我们在UserController中并没有去new一个UserServiceImpl类,因为这样做就没有意义了(虽然有jar包,完全可以这么做)。这里只是演示的直连的方式,还没有注册中心,所以才需要打jar包,后续引入注册中心后,提供者向注册中心暴露接口和实体bean对象就行了,那个时候只能通过RPC调用,就不可能new一个实现类。