效果图
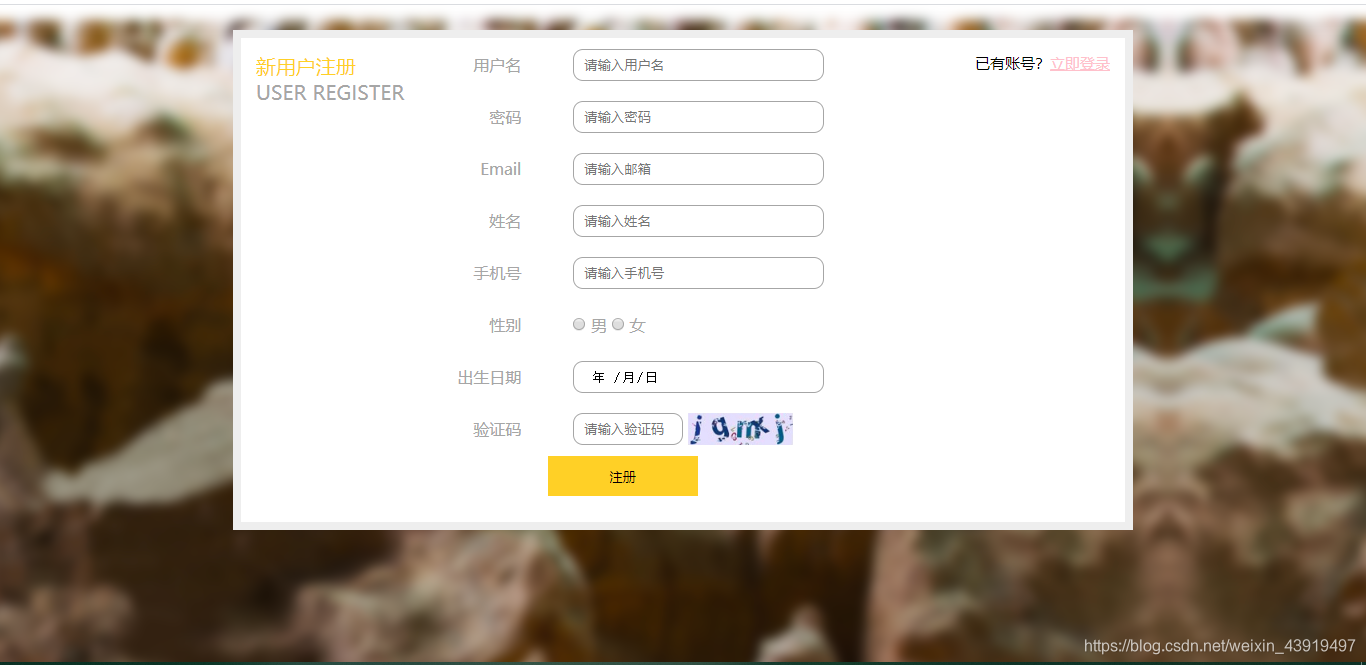
代码
<!DOCTYPE html>
<html lang="ch">
<head>
<meta charset="UTF-8">
<title>用户注册</title>
<style>
*{
margin: 0px;
padding: 0px;
box-sizing: border-box;
}
body{
background-image: url("img/register_bg.png");
padding-top: 25px;
}
.re_div{
border: 8px solid #EEEEEE;
width: 900px;
height: 500px;
background-color: white;
margin: auto;
}
.cLeft{
float: left;
margin: 15px;
}
#p1{
color: #FFD026;
font-size: 20px;
}
#p2{
color: #A6A6A6;
font-size: 20px;
}
.cCenter{
float: left;
}
.td_left{
width: 100px;
height: 50px;
text-align: right;
color: #A6A6A6;
}
.td_right{
padding-left: 50px;
color: #A6A6A6;
}
#username,#password,#email,#name,#tel,#birthday,#checkcode{
width: 251px;
height: 32px;
border: 1px solid #A6A6A6;
border-radius: 10px;
padding-left: 10px;
}
#checkcode{
width: 110px;
}
#cheImg{
height: 32px;
vertical-align: middle;
}
#btn_sub{
width: 150px;
height: 40px;
background-color: #FFD026;
border: 1px solid #FFD026;
}
.cRight{
font-size: 15px;
margin: 15px;
float: right;
}
.cRight p a{
color: pink;
}
</style>
</head>
<body>
<div class="re_div">
<div class="cLeft">
<p id="p1">新用户注册</p>
<p id="p2">USER REGISTER</p>
</div>
<div class="cCenter">
<table>
<tr>
<td class="td_left"><label for="username">用户名</label></td>
<td class="td_right"><input type="text" name="username" id="username" placeholder="请输入用户名"></td>
</tr>
<tr>
<td class="td_left"><label for="password">密码</label></td>
<td class="td_right"><input type="password" name="password" id="password" placeholder="请输入密码"></td>
</tr>
<tr>
<td class="td_left"><label for="email">Email</label></td>
<td class="td_right"><input type="email" name="email" id="email" placeholder="请输入邮箱"></td>
</tr>
<tr>
<td class="td_left"><label for="name">姓名</label></td>
<td class="td_right"><input type="text" name="name" id="name" placeholder="请输入姓名"></td>
</tr>
<tr>
<td class="td_left"><label for="tel">手机号</label></td>
<td class="td_right"><input type="text" name="tel" id="tel" placeholder="请输入手机号"></td>
</tr>
<tr>
<td class="td_left"><label>性别</label></td>
<td class="td_right">
<input type="radio" name="gender" value="male"> 男
<input type="radio" name="gender" value="female"> 女
</td>
</tr>
<tr>
<td class="td_left"><label for="birthday">出生日期</label></td>
<td class="td_right"><input type="date" name="birthday" id="birthday" placeholder="请输入出生日期"></td>
</tr>
<tr>
<td class="td_left"><label for="checkcode" >验证码</label></td>
<td class="td_right"><input type="text" name="checkcode" id="checkcode" placeholder="请输入验证码">
<img id="cheImg" src="img/verify_code.jpg">
</td>
</tr>
<tr>
<td colspan="2" align="center"><input id="btn_sub" type="submit" value="注册"></td>
</tr>
</table>
</div>
<div class="cRight">
<p id="p3">已有账号?<a href="#">立即登录</a></p>
</div>
</div>
</body>
</html>