let colorArr = ['#FAD237', '#3469FC', '#9013FE', '#E5579A', '#34D129'];
let gradientArr = ['250,210,55', '52,105,252', '144,19,254', '229,87,154', '52,209,41'];
initLineChart();
// 图表调用并赋值
function initLineChart() {
let data1 = {
xAxisData: ['2012', '2013', '2014', '2015', '2016', '2017', '2018'],
seriesData: [{
name: '实际用电曲线',
data: [861, 559, 343, 604, 459, 728, 844]
}, {
name: '中长期聚合曲线',
data: [533, 454, 360, 770, 607, 328, 449]
}, {
name: '长期聚合曲线',
data: [461, 583, 421, 455, 515, 739, 583]
}]
};
basicLineChart1(this.$Echarts.init(document.getElementById('line1')), data1);
ladderLineChart(this.$Echarts.init(document.getElementById('line2')), data1);
setLineImgChart(this.$Echarts.init(document.getElementById('line3')), data1);
basicLineChart2(this.$Echarts.init(document.getElementById('line4')), data1);
}
/**
* 基础折线图
* @param chart:echart容器
* data: 折线图数据
*/
export function basicLineChart1(chart, chartData) {
let dataArr = [];
for(let i = 0; i < chartData.seriesData.length; i++) {
let obj = chartData.seriesData[i];
dataArr.push({
name: obj.name,
type: 'line',
symbolSize: 0,
smooth: true,
lineStyle: {
color: 'rgb(' + gradientArr[i] &
Echarts实现折线图效果
于 2023-07-07 15:28:06 首次发布
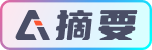