import numpy as np
使用数组进行面向数组编程
- 向量化:利用数组表达式来代替显式循环的方法
- 速度会快
1~2
个量级 广播机制
是向量化的一种应用
np.meshgrid(arr_1, arr_2)
points = np.arange(-5, 5, 0.01)
small_points_1 = np.array([1, 2, 3])
small_points_2 = np.array([7, 8])
np.meshgrid(small_points_1, small_points_2)
[array([[1, 2, 3],
[1, 2, 3]]),
array([[7, 7, 7],
[8, 8, 8]])]
大型例子
x_s, y_s = np.meshgrid(points, points)
y_s
array([[-5. , -5. , -5. , ..., -5. , -5. , -5. ],
[-4.99, -4.99, -4.99, ..., -4.99, -4.99, -4.99],
[-4.98, -4.98, -4.98, ..., -4.98, -4.98, -4.98],
...,
[ 4.97, 4.97, 4.97, ..., 4.97, 4.97, 4.97],
[ 4.98, 4.98, 4.98, ..., 4.98, 4.98, 4.98],
[ 4.99, 4.99, 4.99, ..., 4.99, 4.99, 4.99]])
z = np.sqrt(x_s**2 + y_s**2)
z
array([[7.07106781, 7.06400028, 7.05693985, ..., 7.04988652, 7.05693985,
7.06400028],
[7.06400028, 7.05692568, 7.04985815, ..., 7.04279774, 7.04985815,
7.05692568],
[7.05693985, 7.04985815, 7.04278354, ..., 7.03571603, 7.04278354,
7.04985815],
...,
[7.04988652, 7.04279774, 7.03571603, ..., 7.0286414 , 7.03571603,
7.04279774],
[7.05693985, 7.04985815, 7.04278354, ..., 7.03571603, 7.04278354,
7.04985815],
[7.06400028, 7.05692568, 7.04985815, ..., 7.04279774, 7.04985815,
7.05692568]])
用matplotlib来尝试一下
import matplotlib.pyplot as plt
plt.imshow(z, cmap=plt.cm.gray); plt.colorbar()
plt.title("Image plot of $\sqrt{x^2 + y^2}$ for a grid of values")
plt.draw()
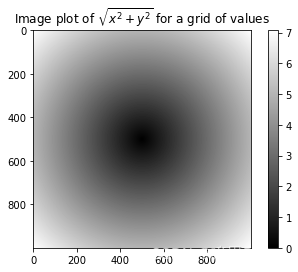
plt.close('all')
将条件逻辑作为数组操作
np.where()
是 x if CONDITION else y
的向量化
表示
x_arr = np.array([1.1, 1.2, 1.3, 1.4, 1.5])
y_arr = np.array([2.1, 2.2, 2.3, 2.4, 2.5])
cond = np.array([True, False, True, True, False])
- 需求为:当 cond 为 True 时,取
x_arr
中元素,反之 y_arr
result = np.where(cond, x_arr, y_arr)
result
array([1.1, 2.2, 1.3, 1.4, 2.5])
- 随机生成
4*4
矩阵,将所有的正值
替换为+2
,负值
替换为-2
matrix_4_4 = np.random.randn(4, 4)
matrix_4_4
array([[-0.31057548, -0.33117123, -0.32092367, -0.83744854],
[ 0.65508715, -1.09744727, -0.28864518, 1.87957568],
[ 0.13691023, 1.27582489, -0.73059565, -1.25889417],
[ 0.94719682, 1.22910758, -1.44860514, 0.63306565]])
cond = matrix_4_4 > 0
result = np.where(cond, 2, -2)
result
array([[-2, -2, -2, -2],
[ 2, -2, -2, 2],
[ 2, 2, -2, -2],
[ 2, 2, -2, 2]])
数学和统计方法
聚合
函数,可以np.sum(arr)
也可以使用arr.sum()
arr = np.random.randn(5, 4)
arr
array([[-6.15103319e-01, 1.94935393e-01, 1.07466373e+00,
5.60804143e-01],
[-1.29562464e+00, -9.50808332e-01, -6.91278641e-01,
-1.90757357e-03],
[ 2.14085181e+00, -5.65517145e-03, 2.99332159e-01,
-4.74249005e-01],
[-1.46233648e+00, 7.16732377e-01, -1.02124467e-01,
3.93163196e-02],
[-9.34729945e-01, 3.65241170e-01, -5.98246162e-02,
-1.46758290e-01]])
arr.mean()
-0.06742616905151418
np.mean(arr)
-0.06742616905151418
arr.sum()
-1.3485233810302835
test = np.array([[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3]])
print(test.mean(axis = 0))
print(test.mean(axis = 1))
[2. 2. 2. 2.]
[1. 2. 3.]
np.cumsum()
:从0开始元素累积和np.cumprod()
:从1开始元素累积积
arr = np.array([[1, 1, 2],
[3, 4, 5],
[6, 7, 8]])
arr
array([[1, 1, 2],
[3, 4, 5],
[6, 7, 8]])
arr.cumsum(axis=0)
array([[ 1, 1, 2],
[ 4, 5, 7],
[10, 12, 15]])
arr.cumprod(axis=1)
array([[ 1, 1, 2],
[ 3, 12, 60],
[ 6, 42, 336]])
from PIL import Image
Image.open('./numpy_statistic.jpeg')
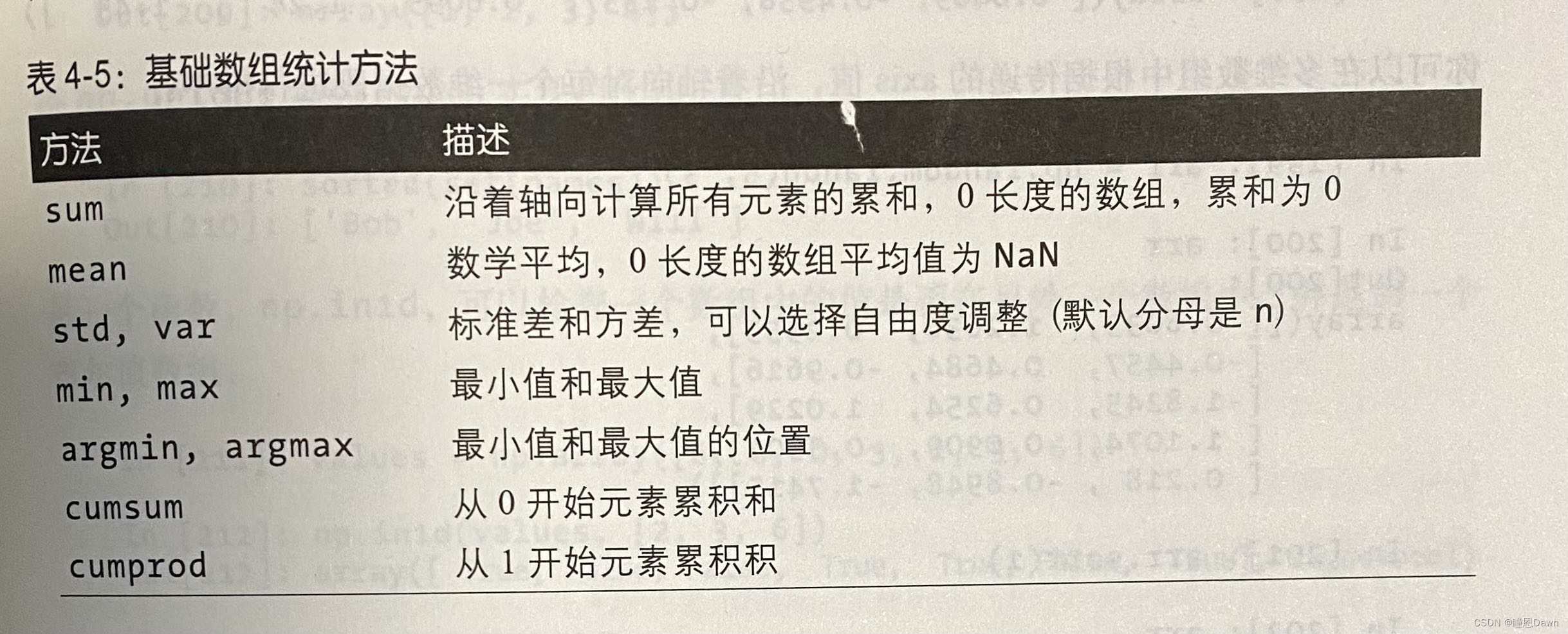
BOOL数组的方法
bool
值会被强制定为1 or 0
- 可以用来计算非0个数
arr = np.random.randn(100)
(arr>0).sum()
49
np.any()
和 np.all()
- any 是
至少
有一个True嘛? - all 是
全是
True嘛?
bools = np.array([False, False, True, False])
bools.any()
True
bools.all()
False
排序
np.sort()
返回的是排好序数组拷贝,不会原数组改变顺序- 根据某一列进行排序,会出现在pandas中
arr = np.random.randn(5, 3)
arr
array([[-0.71284458, -0.59405487, 1.20906567],
[-1.25745017, 0.90948517, -0.24589889],
[-0.18246322, -1.85378669, -1.14372438],
[ 0.19665606, -2.42751683, 1.75191219],
[ 0.07381909, -0.27235433, -0.74304361]])
np.sort(arr, 0)
array([[-1.25745017, -2.42751683, -1.14372438],
[-0.71284458, -1.85378669, -0.74304361],
[-0.18246322, -0.59405487, -0.24589889],
[ 0.07381909, -0.27235433, 1.20906567],
[ 0.19665606, 0.90948517, 1.75191219]])
唯一值与其他集合逻辑
np.unique()
返回数组中唯一值的排序好的数组
names = np.array(['Bob', 'Joe', 'Will', 'Bob', 'Will', 'Joe', 'Joe'])
names
array(['Bob', 'Joe', 'Will', 'Bob', 'Will', 'Joe', 'Joe'], dtype='<U4')
np.unique(names)
array(['Bob', 'Joe', 'Will'], dtype='<U4')
np.in1d([], [])
可以检查一个数组是否在另一个数组中,返回一个bool
数组
values = np.array([6, 0, 0, 3, 2, 5, 6])
np.in1d(values, [2, 3, 6])
array([ True, False, False, True, True, False, True])
Image.open('./List_set.jpeg')
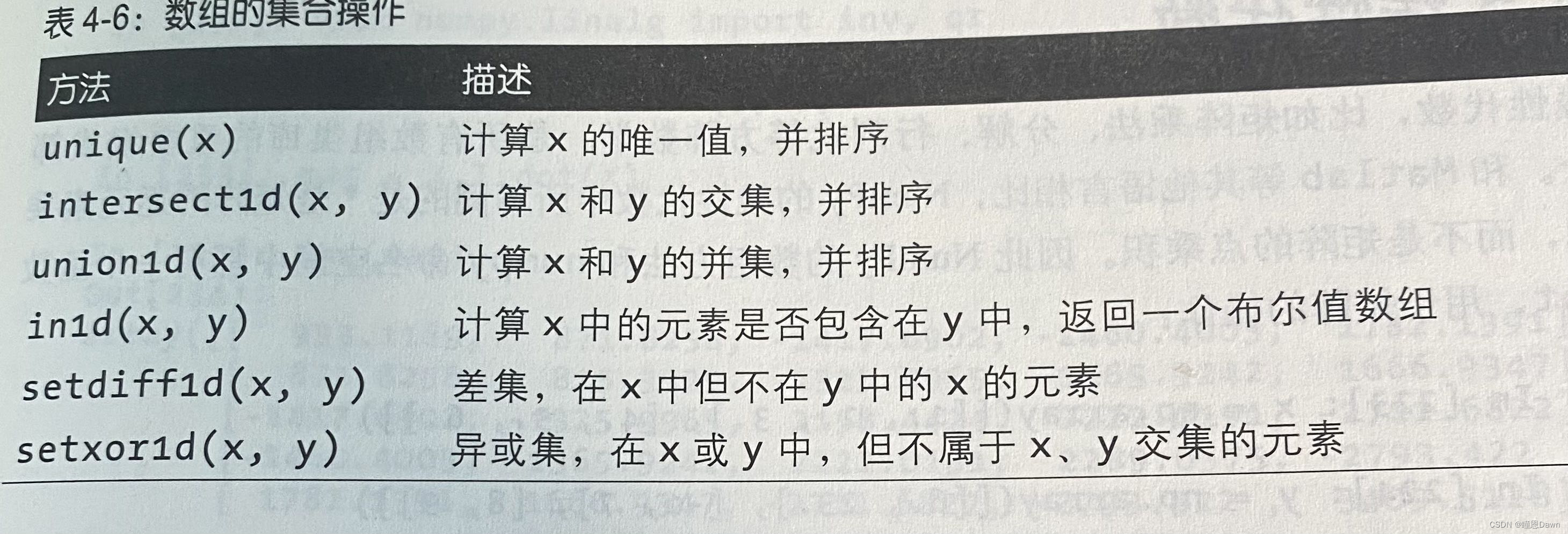