PAT1003紧急情况
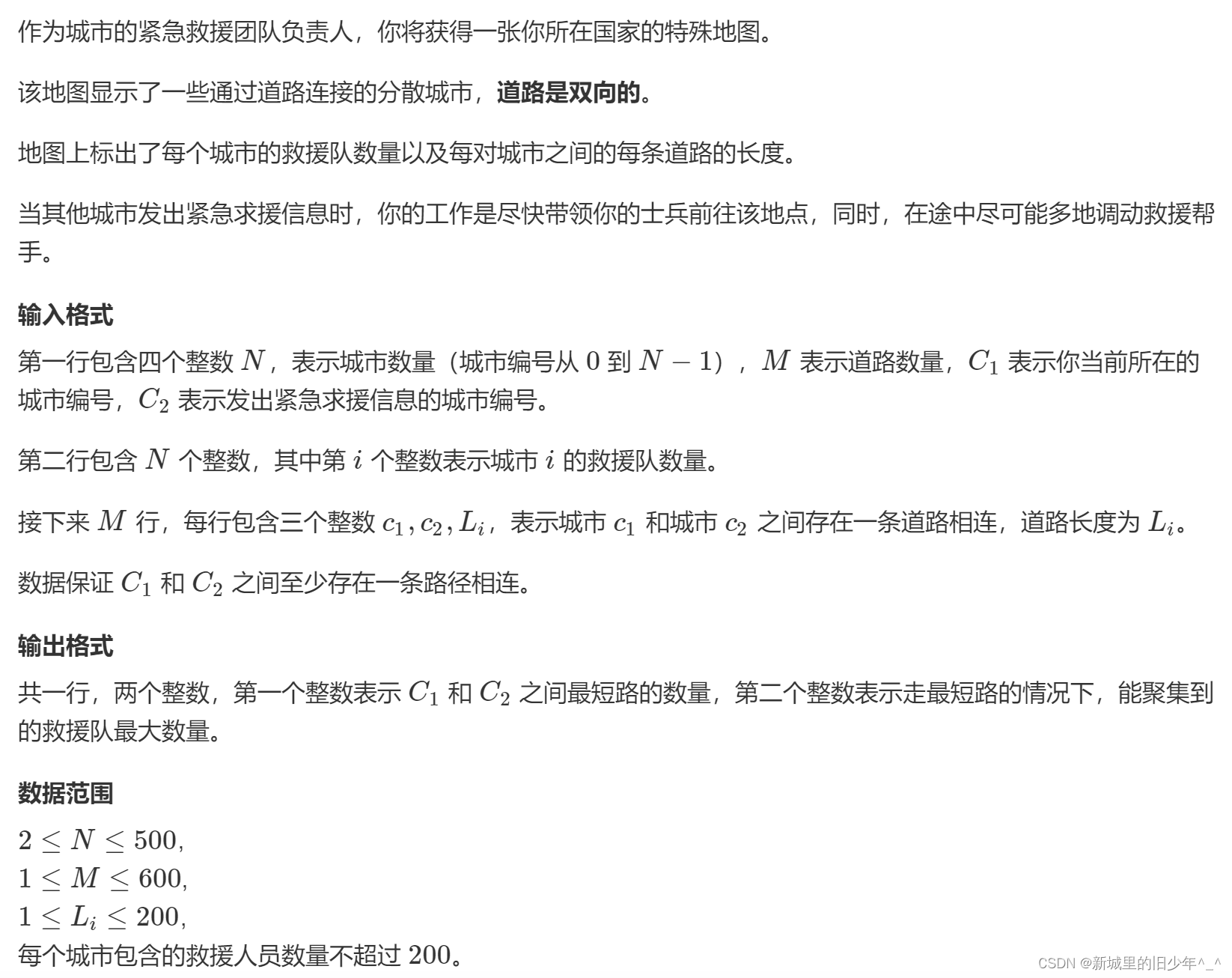
#include <algorithm>
#include <iostream>
#include <cstring>
using namespace std;
const int N = 510;
int n,m,s,t;
int g[N][N];
bool visit[N];
int w[N];
int dis[N],route_sum[N],weight_sum[N];
void dijkstra()
{
dis[s] = 0;
route_sum[s] = 1;
weight_sum[s] = w[s];
for(int i=0;i<n;i++){
int tt = -1;
for(int j=0;j<n;j++){
if(!visit[j] && (tt==-1 || dis[j] < dis[tt])) tt = j;
}
visit[tt] = true;
for(int j=0;j<n;j++){
if(dis[j] > dis[tt] + g[tt][j]){
dis[j] = dis[tt] + g[tt][j];
route_sum[j] = route_sum[tt];
weight_sum[j] = weight_sum[tt] + w[j];
}
else if(dis[j]==(dis[tt]+g[tt][j])){
route_sum[j] += route_sum[tt];
weight_sum[j] = max(weight_sum[j],weight_sum[tt]+w[j]);
}
}
}
}
int main()
{
memset(g,0x3f,sizeof g);
memset(dis,0x3f,sizeof dis);
cin >> n >> m >> s >> t;
for(int i=0;i<n;i++) cin >> w[i];
while(m--){
int a,b,c;
cin >> a >> b >> c;
g[a][b] = min(g[a][b],c);
g[b][a] = min(g[b][a],c);
}
dijkstra();
cout << route_sum[t] << " " << weight_sum[t] << endl;
return 0;
}
旅行计划
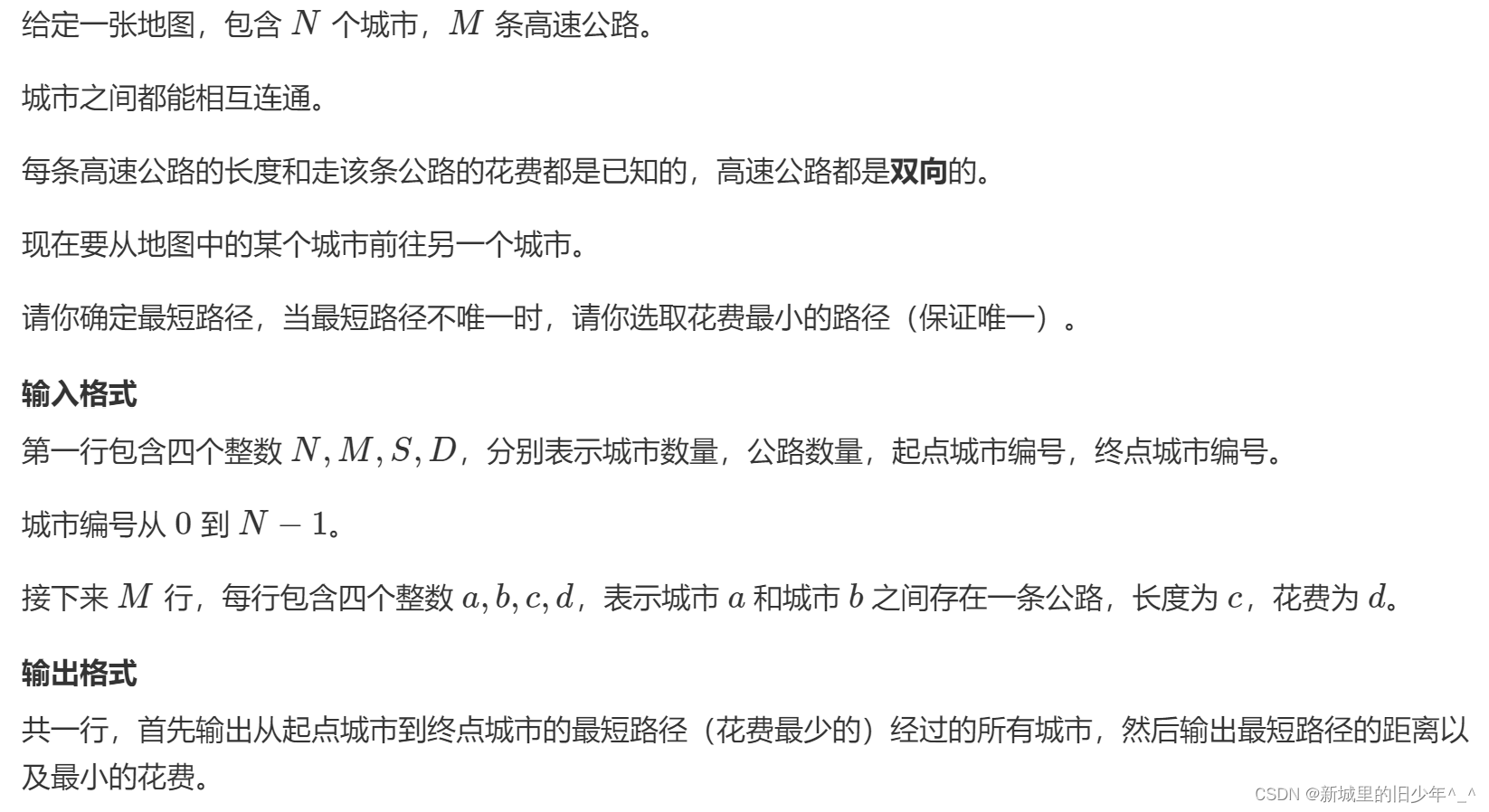
#include <algorithm>
#include <cstring>
#include <iostream>
#include <vector>
using namespace std;
const int N = 510;
int n,m,s,d;
int g[N][N],c[N][N];
int dis[N],cost[N];
int pre[N];
bool st[N];
int main()
{
memset(g,0x3f,sizeof g);
memset(c,0x3f,sizeof c);
memset(pre,-1,sizeof pre);
cin >> n >> m >> s >> d;
while(m--){
int a,b,x,y;
cin >> a >> b >> x >> y;
g[a][b] = g[b][a] = min(g[a][b],x);
c[a][b] = c[b][a] = min(c[a][b],y);
}
memset(dis,0x3f,sizeof dis);
memset(cost,0x3f,sizeof cost);
dis[s] = 0;
cost[s] = 0;
for(int i=0;i<n;i++){
int t = -1;
for(int j=0;j<n;j++){
if(!st[j] && (t==-1 || dis[j] < dis[t])) t = j;
}
st[t] = true;
for(int j=0;j<n;j++){
if(dis[j] > dis[t]+g[t][j]){
dis[j] = dis[t] + g[t][j];
cost[j] = cost[t] + c[t][j];
pre[j] = t;
}
else if(dis[j]==(dis[t]+g[t][j])){
if((cost[t]+c[t][j]) < cost[j]){
pre[j] = t;
cost[j] = cost[t] + c[t][j];
}
}
}
}
vector<int> res;
for(int i=d;i!=-1;i=pre[i]) res.push_back(i);
for(int i=res.size()-1;i>=0;i--) cout << res[i] << " ";
cout << dis[d] << " " << cost[d] << endl;
return 0;
}
团伙头目
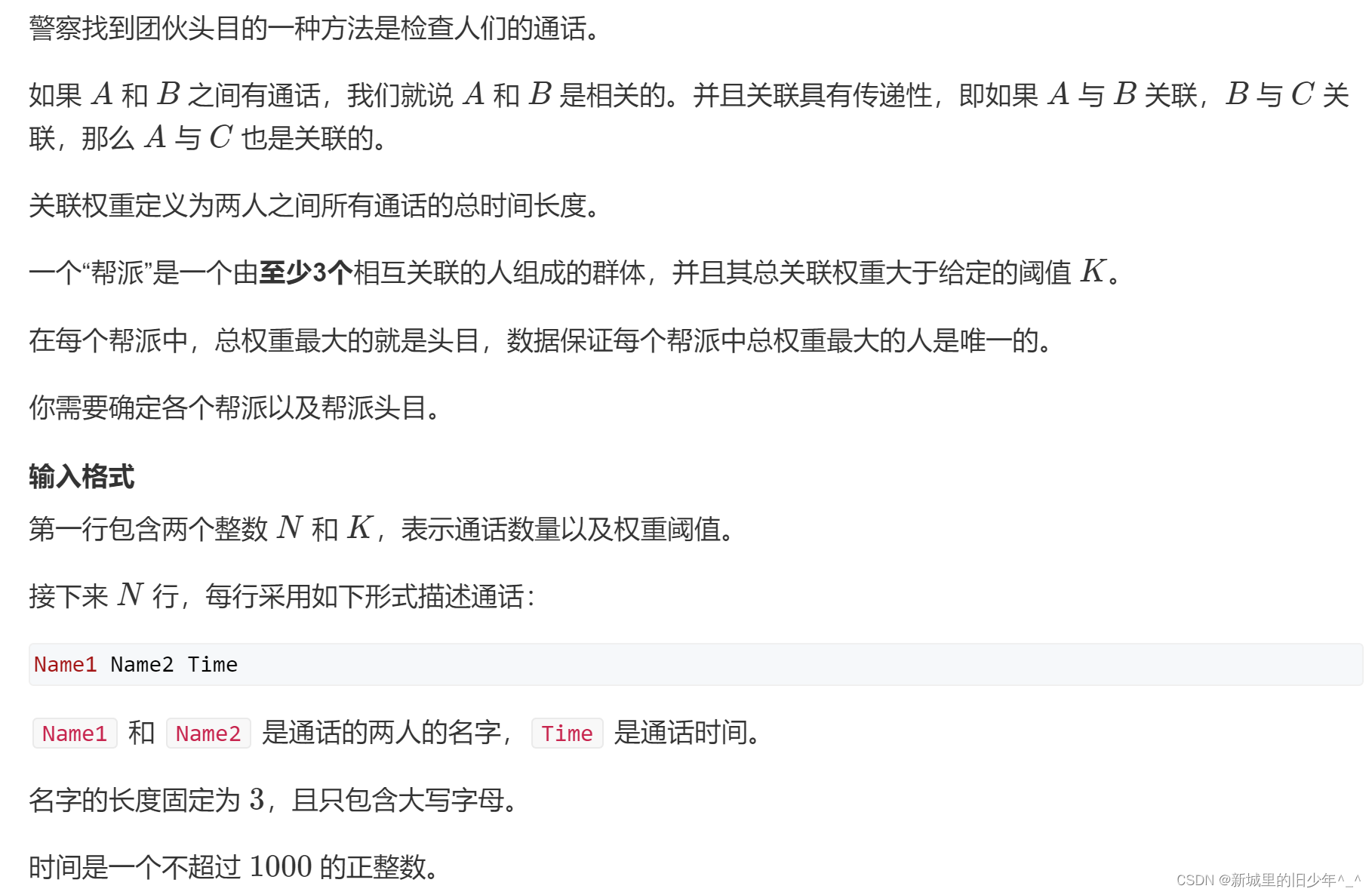
#include <cstring>
#include <iostream>
#include <vector>
#include <algorithm>
#include <map>
using namespace std;
const int N = 1010;
int n,k;
map<string,vector<pair<string,int>>> g;
map<string,int> total;
map<string,bool> st;
int dfs(string ver, vector<string>& nodes)
{
st[ver] = true;
nodes.push_back(ver);
int sum = 0;
for(auto next_node : g[ver]){
sum += next_node.second;
if(!st[next_node.first]) sum += dfs(next_node.first,nodes);
}
return sum;
}
int main()
{
cin >> n >> k;
while(n--){
string a,b;
int t;
cin >> a >> b >> t;
g[a].push_back({b,t});
g[b].push_back({a,t});
total[a] += t;
total[b] += t;
}
vector<pair<string,int>> res;
for(auto item:total){
string ver = item.first;
vector<string> nodes;
int sum = dfs(ver,nodes)/2;
if(nodes.size()>2 && sum > k )
{
string boss = nodes[0];
for(string node:nodes){
if(total[boss] < total[node]) boss = node;
}
res.push_back({boss,nodes.size()});
}
}
sort(res.begin(),res.end());
cout << res.size() << endl;
for(auto item:res){
cout << item.first << " " << item.second << endl;
}
return 0;
}
条条大路通罗马
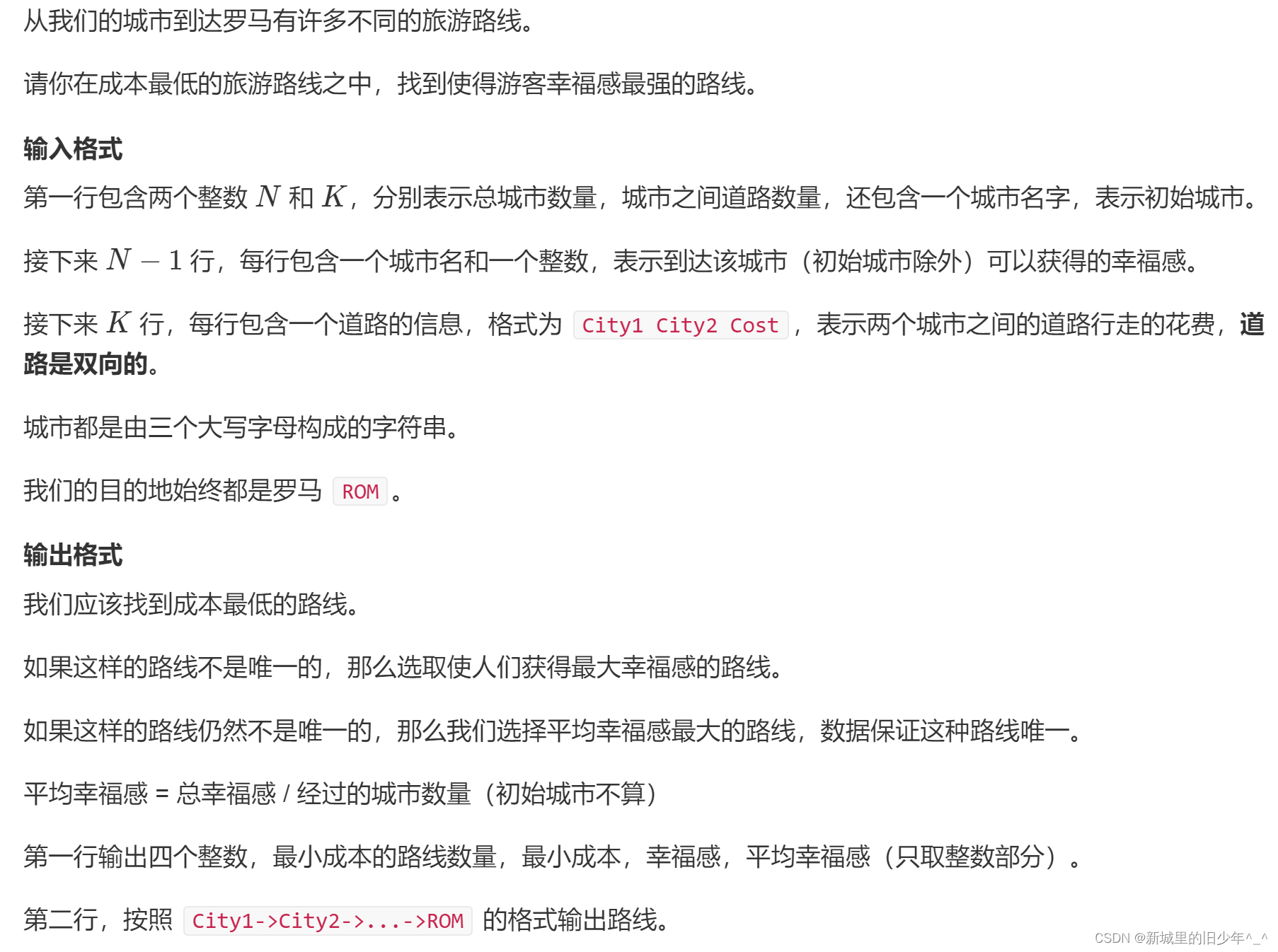
#include <iostream>
#include <algorithm>
#include <cstring>
#include <map>
using namespace std;
const int N = 210;
int n,k;
string city[N];
map<string,int> mp;
int w[N];
int g[N][N];
bool st[N];
int dis[N],route_num[N],cost[N],node_num[N],pre[N];
void dijkstra()
{
memset(dis,0x3f,sizeof dis);
dis[1] = 0;
route_num[1] = 1;
for(int i=0;i<n;i++){
int t = -1;
for(int j=1;j<=n;j++){
if(!st[j] && (t==-1 || dis[j] < dis[t])) t = j;
}
st[t] = true;
for(int j=1;j<=n;j++){
if(dis[j] > dis[t]+g[t][j]){
dis[j] = dis[t] + g[t][j];
route_num[j] = route_num[t];
cost[j] = cost[t] + w[j];
node_num[j] = node_num[t] + 1;
pre[j] = t;
}
else if(dis[j]==(dis[t]+g[t][j])){
route_num[j] += route_num[t];
if(cost[j] < cost[t]+w[j]){
cost[j] = cost[t] + w[j];
node_num[j] = node_num[t] + 1;
pre[j] = t;
}
else if(cost[j]==(cost[t]+w[j])){
if(node_num[j] > (node_num[t]+1)){
node_num[j] = node_num[t] + 1;
pre[j] = t;
}
}
}
}
}
}
int main()
{
cin >> n >> k >> city[1];
mp[city[1]] = 1;
w[1] = 0;
for(int i=2;i<=n;i++){
cin >> city[i] >> w[i];
mp[city[i]] = i;
}
memset(g,0x3f,sizeof g);
while(k--){
string a,b;
int num;
cin >> a >> b >> num;
g[mp[a]][mp[b]] = min(g[mp[a]][mp[b]],num);
g[mp[b]][mp[a]] = min(g[mp[b]][mp[a]],num);
}
dijkstra();
int t = mp["ROM"];
cout << route_num[t] << " " << dis[t] << " " << cost[t] << " " << cost[t]/node_num[t] << endl;
vector<int> res;
for(int i=t;i!=0;i=pre[i]) res.push_back(i);
cout << city[res[res.size()-1]];
for(int i=res.size()-2;i>=0;i--) cout << "->" << city[res[i]];
return 0;
}
在线地图
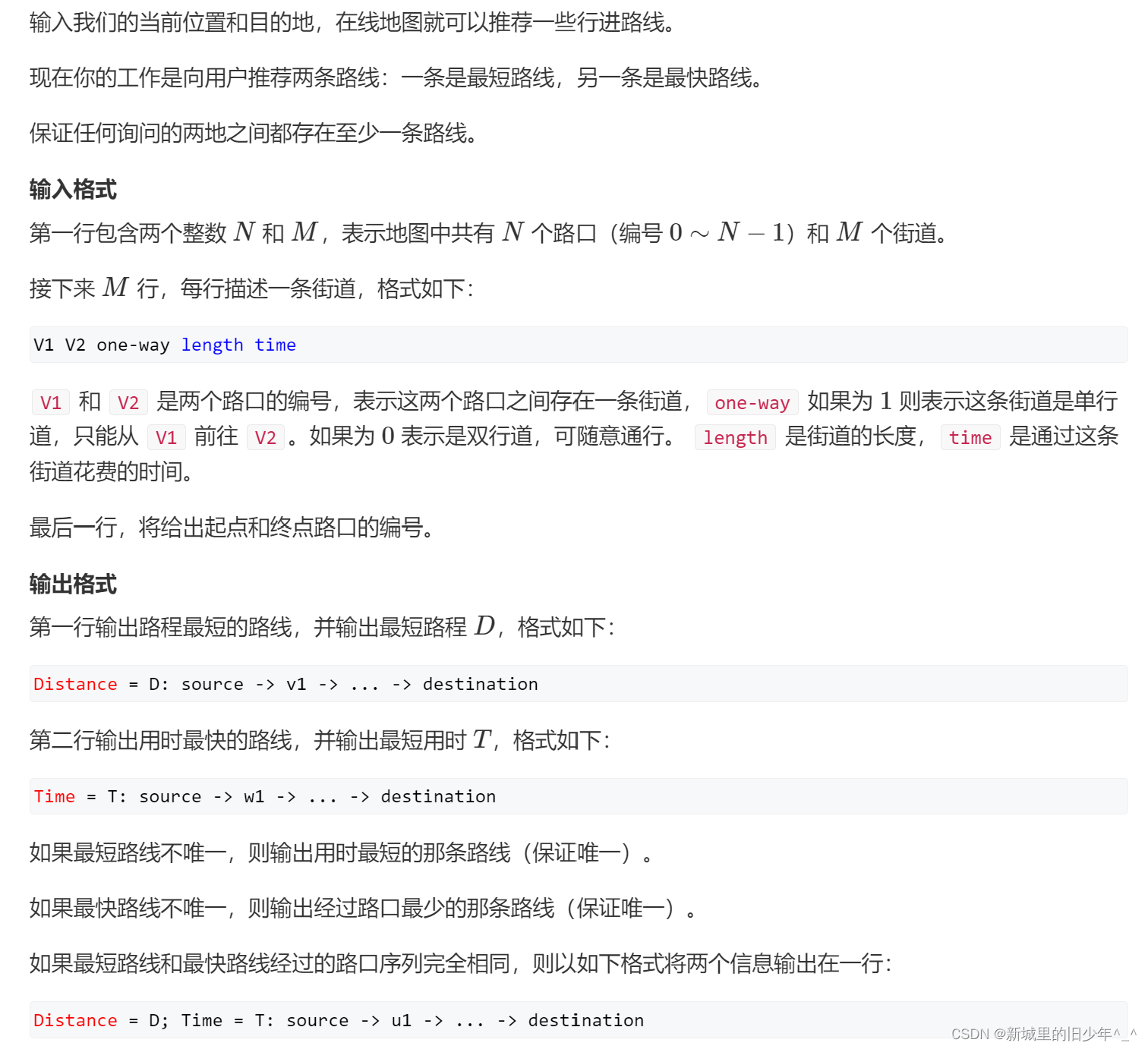
#include <algorithm>
#include <cstring>
#include <iostream>
#include <vector>
using namespace std;
const int N = 510;
int dis1[N],time1[N];
int time2[N],crossing[N];
int n,m,s,t;
int d1[N][N],d2[N][N];
int pre[N];
bool st[N];
void dijkstra1()
{
memset(dis1,0x3f,sizeof dis1);
memset(time1,0x3f,sizeof time1);
memset(st,0,sizeof st);
dis1[s] = 0;
time1[s] = 0;
for(int i=0;i<n;i++){
int t = -1;
for(int j=0;j<n;j++){
if(!st[j] && (t==-1 || dis1[j] < dis1[t] )) t = j;
}
st[t] = true;
for(int j=0;j<n;j++){
if(dis1[j] > (dis1[t] + d1[t][j])){
dis1[j] = dis1[t] + d1[t][j];
time1[j] = time1[t] + d2[t][j];
pre[j] = t;
}
else if(dis1[j]==(dis1[t]+d1[t][j]))
{
if(time1[j] > (time1[t] + d2[t][j])){
time1[j] = time1[t] + d2[t][j];
pre[j] = t;
}
}
}
}
}
void dijkstra2()
{
memset(time2,0x3f,sizeof time2);
memset(crossing,0x3f,sizeof crossing);
memset(st,0,sizeof st);
memset(pre,-1,sizeof pre);
time2[s] = 0;
crossing[s] = 0;
for(int i=0;i<n;i++){
int t = -1;
for(int j = 0; j < n; j++){
if(!st[j] && (t==-1 || time2[j] < time2[t])) t = j;
}
st[t] = true;
for(int j=0;j<n;j++){
if(time2[j] > (time2[t]+d2[t][j])){
time2[j] = time2[t] + d2[t][j];
crossing[j] = crossing[t] + 1;
pre[j] = t;
}
else if(time2[j] == (time2[t] + d2[t][j])){
if(crossing[j] > (crossing[t]+1)){
crossing[j] = crossing[t] + 1;
pre[j] = t;
}
}
}
}
}
int main()
{
memset(d1,0x3f,sizeof d1);
memset(d2,0x3f,sizeof d2);
cin >> n >> m;
while(m--){
int v1,v2,one_way,length,cost_time;
cin >> v1 >> v2 >> one_way >> length >> cost_time;
d1[v1][v2] = min(d1[v1][v2],length);
d2[v1][v2] = min(d2[v1][v2],cost_time);
if(!one_way){
d1[v2][v1] = min(d2[v2][v1],length);
d2[v2][v1] = min(d2[v2][v1],cost_time);
}
}
cin >> s >> t;
vector<int> path1;
dijkstra1();
for(int i=t;i!=s;i=pre[i]) path1.push_back(i);
path1.push_back(s);
vector<int> path2;
dijkstra2();
for(int i=t;i!=s;i=pre[i]) path2.push_back(i);
path2.push_back(s);
if(path1 != path2){
printf("Distance = %d: ",dis1[t]);
cout << path1[path1.size()-1];
for(int i=path1.size()-2;i>=0;i--) cout << " -> " << path1[i];
cout << endl;
printf("Time = %d: ",time2[t]);
cout << path2[path2.size()-1];
for(int i=path2.size()-2;i>=0;i--) cout << " -> " << path2[i];
}
else{
printf("Distance = %d; Time = %d: ",dis1[t],time2[t]);
cout << path1[path1.size()-1];
for(int i=path1.size()-2;i>=0;i--) cout << " -> " << path1[i];
}
return 0;
}
哈密顿回路
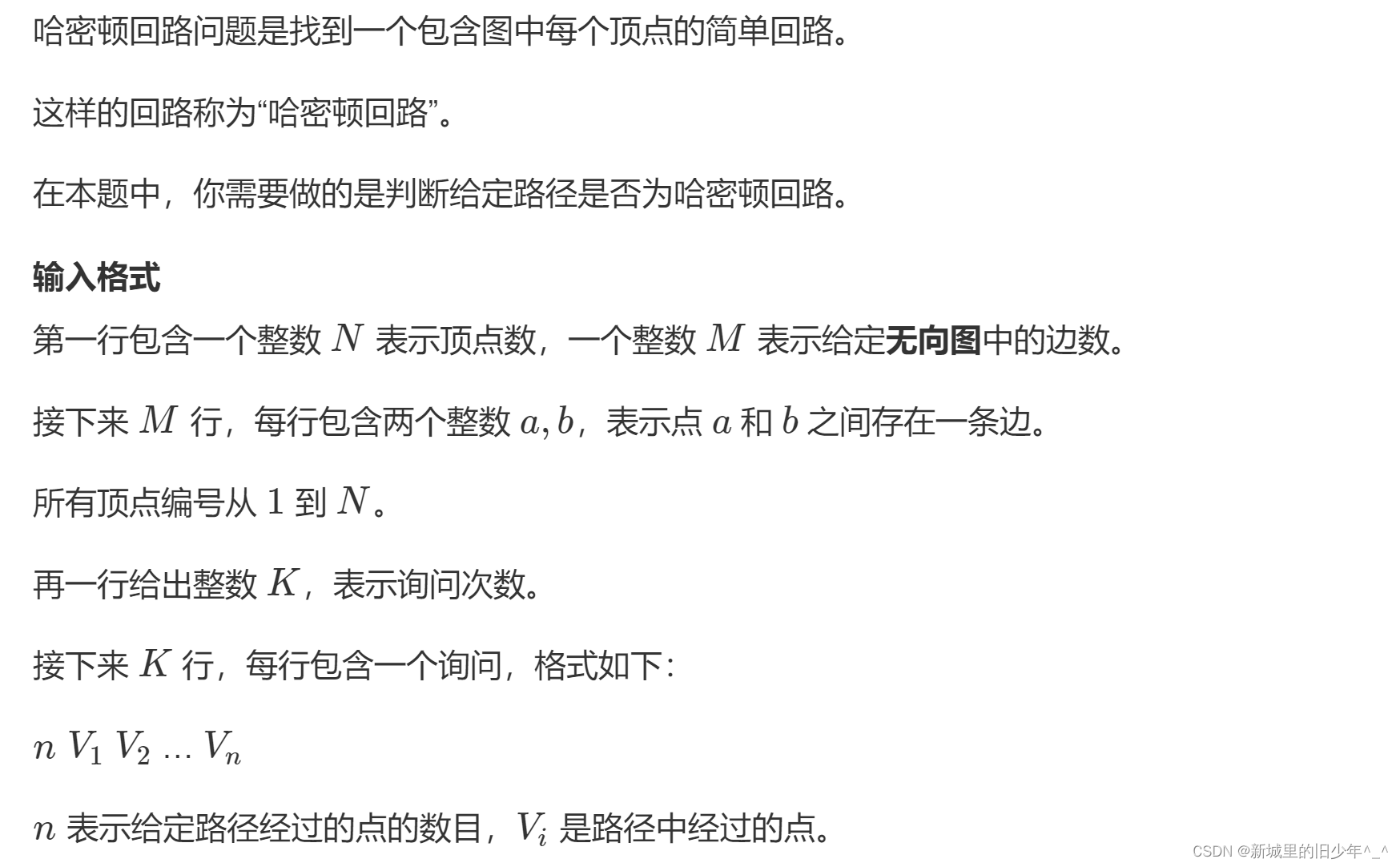
#include <algorithm>
#include <iostream>
#include <cstring>
using namespace std;
const int N = 210;
bool g[N][N];
int n,m;
int nodes[N];
bool st[N];
bool check(int node_num)
{
if(nodes[0] != nodes[node_num-1]) return false;
if(node_num != n+1) return false;
memset(st,0,sizeof st);
for(int i=0;i<n;i++){
st[nodes[i]] = true;
}
for(int i=1;i<=n;i++){
if(st[i]==false) return false;
}
for(int i=0;i<node_num-1;i++){
if(g[nodes[i]][nodes[i+1]] == false) return false;
}
return true;
}
int main()
{
cin >> n >> m;
while(m--)
{
int a,b;
cin >> a >> b;
g[a][b] = g[b][a] = true;
}
int k;
cin >> k;
while(k--)
{
int cnt;
cin >> cnt;
for(int i=0;i<cnt;i++) cin >> nodes[i];
if(check(cnt)) cout << "YES" << endl;
else cout << "NO" << endl;
}
return 0;
}
欧拉路径
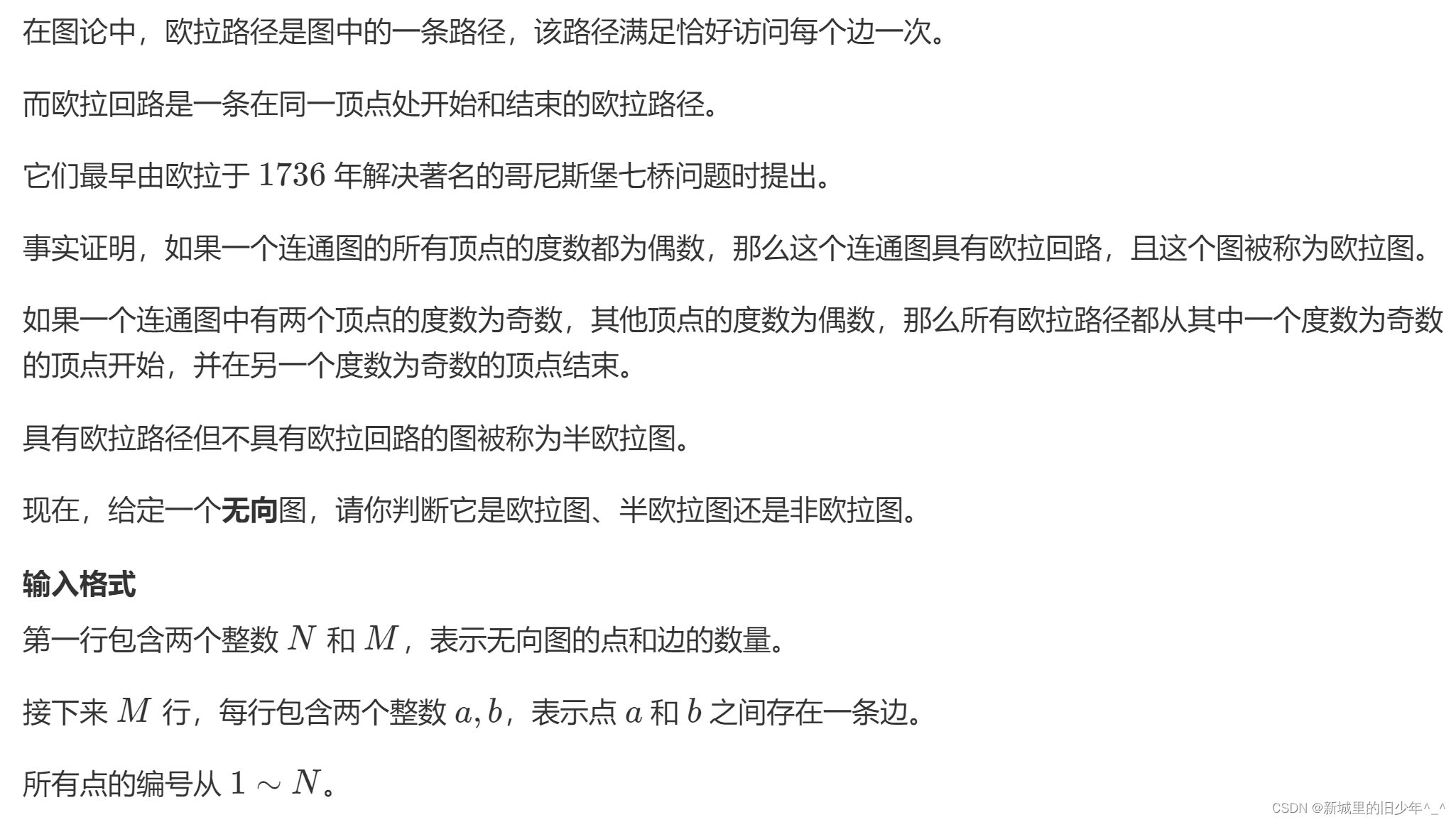
#include <algorithm>
#include <iostream>
#include <cstring>
using namespace std;
const int N = 510;
bool g[N][N];
int d[N];
int n,m;
bool st[N];
int res = 0;
void dfs(int u)
{
res++;
st[u] = true;
for(int i=1;i<=n;i++){
if(!st[i] && g[u][i]) dfs(i);
}
}
int main()
{
memset(st,0,sizeof st);
cin >> n >> m;
while(m--)
{
int a,b;
cin >> a >> b;
g[a][b] = g[b][a] = true;
d[a]++,d[b]++;
}
dfs(1);
int degree_odd = 0;
for(int i=1;i<=n;i++){
if(d[i]%2) degree_odd++;
}
for(int i=1;i<=n;i++) cout << d[i] << " ";
cout << endl;
if(res==n){
if(degree_odd==0) puts("Eulerian");
else if(degree_odd==2) puts("Semi-Eulerian");
else puts("Non-Eulerian");
}
else puts("Non-Eulerian");
return 0;
}