先从一个图像开始:
可以通过键盘的上下左右按键来控制移动方向
'''设置窗口—>设置初始速度speed—>加载图片—>得到图片矩形位置—>根据speed移动改变position—>填充背景position
加载图片(把photo放到screen)—>更新整个待显示的 Surface 对象到屏幕上'''
#导入pygame包
import pygame
import sys
#初始化pygame
pygame.init()
#窗口大小
size=width,height=800,600
#设置窗口,初始化一个准备显示的窗口或屏幕,set_mode(resolution=(0,0), flags=0, depth=0) -> Surface
screen=pygame.display.set_mode(size)
pygame.display.set_caption('你好')
#默认偏移量,越大,每次move就移动的多,可看作速度
speed=[1,1]
#加载图片,返回的是surface对象
photo1=pygame.image.load('企鹅.png')
#得到图片的矩形框,返回Rect对象
position=photo1.get_rect()
while True:
for event in pygame.event.get():
#如果事件类型是退出,就退出程序
if event.type==pygame.QUIT:
sys.exit()
'''通过按键,改变偏移量speed'''
#如果事件类型是键盘按键(key 模块)
if event.type==pygame.KEYDOWN:#不想用pygame.则可以在前面加from pygame.locals import *
#如果按下左键,左移
if event.key==pygame.K_LEFT:
speed=[-1,0]
#如果按下右键,右移
if event.key==pygame.K_RIGHT:
speed=[1,0]
#如果按下上键,上移
if event.key==pygame.K_UP:
speed=[0,-1]
#如果按下下键,下移
if event.key==pygame.K_DOWN:
speed=[0,1]
#根据speed移动
position=position.move(speed)
'''
#到边界时不动
if position.left<=0 or position.right>=width or position.top<=0 or position.bottom>=height :
speed=[0,0]
'''
'''移动后,会出界,所以设置将出界时的一些操作,下次循环以这个speed来移动'''
#出左右界,改变speed,也就是对speed列表第0个位置取反,而第1个位置不变,使得反向移动
if position.left<=0 or position.right>=width:
speed[0]=-speed[0]
#出上下界,对speed列表第1个位置取反,而第0个位置不变,使得反向移动
if position.top<=0 or position.bottom>=height :
speed[1]=-speed[1]
#填充背景
screen.fill((255,255,255))
#加载图片,把photo1放到screen
screen.blit(photo1,position)
#更新整个待显示的 Surface 对象到屏幕上
pygame.display.flip()
#pygame.time.delay(10)
pygame.time.Clock().tick(200)
效果如图:
三个走起!一只企鹅可以控制,另外两只自由移动
步骤看似有点重复,但是为下面多个图像起到铺垫作用
import pygame#导入pygame包
import sys
#初始化pygame
pygame.init()
#窗口大小
size=width,height=800,600
#设置窗口,初始化一个准备显示的窗口或屏幕,set_mode(resolution=(0,0), flags=0, depth=0) -> Surface
screen=pygame.display.set_mode(size)
pygame.display.set_caption('企鹅碰碰碰')
#默认偏移量,越大,每次move就移动的多,可看作速度
speed=[1,1]
speed1=[2,2]
speed2=[3,3]
#加载图片,返回的是surface对象
photo=pygame.image.load('企鹅.png')
photo1=pygame.image.load('企鹅.png')
photo2=pygame.image.load('企鹅.png')
#得到图片的矩形框,返回Rect对象
position=photo.get_rect()
position1=photo1.get_rect()
position2=photo2.get_rect()
while True:
for event in pygame.event.get():
#如果事件类型是退出,就退出程序
if event.type==pygame.QUIT:
sys.exit()
'''通过按键,改变偏移量speed'''
#如果事件类型是键盘按键(key 模块)
if event.type==pygame.KEYDOWN:#不想用pygame.则可以在前面加from pygame.locals import *
#如果按下左键,左移
if event.key==pygame.K_LEFT:
speed=[-1,0]
#如果按下右键,右移
if event.key==pygame.K_RIGHT:
speed=[1,0]
#如果按下上键,上移
if event.key==pygame.K_UP:
speed=[0,-1]
#如果按下下键,下移
if event.key==pygame.K_DOWN:
speed=[0,1]
#根据speed移动
position=position.move(speed)
position1=position1.move(speed1)
position2=position2.move(speed2)
'''
#到边界时不动
if position.left<=0 or position.right>=width or position.top<=0 or position.bottom>=height :
speed=[0,0]
'''
'''移动后,会出界,所以设置将出界时的一些操作,下次循环以这个speed来移动'''
#出左右界,改变speed,也就是对speed列表第0个位置取反,而第1个位置不变,使得反向移动
if position.left<=0 or position.right>=width:
speed[0]=-speed[0]
#出上下界,对speed列表第1个位置取反,而第0个位置不变,使得反向移动
if position.top<=0 or position.bottom>=height :
speed[1]=-speed[1]
if position1.left<=0 or position1.right>=width:
speed1[0]=-speed1[0]
#出上下界,对speed列表第1个位置取反,而第0个位置不变,使得反向移动
if position1.top<=0 or position1.bottom>=height :
speed1[1]=-speed1[1]
if position2.left<=0 or position2.right>=width:
speed2[0]=-speed2[0]
#出上下界,对speed列表第1个位置取反,而第0个位置不变,使得反向移动
if position2.top<=0 or position2.bottom>=height :
speed2[1]=-speed2[1]
#填充背景
screen.fill((255,255,255))
#加载图片,把photo放到screen
screen.blit(photo,position)
screen.blit(photo1,position1)
screen.blit(photo2,position2)
#更新整个待显示的 Surface 对象到屏幕上
pygame.display.flip()
#pygame.time.delay(10)
pygame.time.Clock().tick(200)
效果如图:
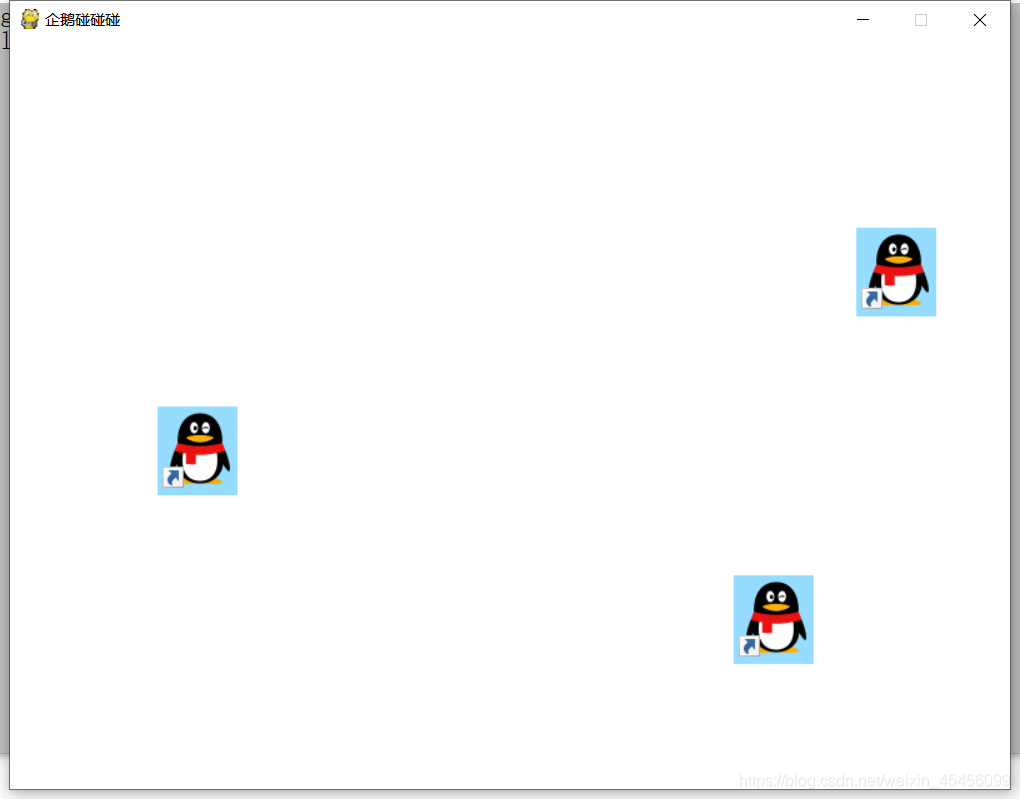
接下来变成多个图像:一只可控,其他按程序自由移动
这个的关键在于怎么把字符串当作变量名使用 ,下面再介绍
'''设置窗口—>设置初始速度speed—>加载图片—>得到图片矩形位置—>根据speed移动改变position—>填充背景position
加载图片(把photo放到screen)—>更新整个待显示的 Surface 对象到屏幕上'''
#导入pygame包
import pygame
import sys
#初始化pygame
pygame.init()
#窗口大小
size=width,height=1000,800
#设置窗口,初始化一个准备显示的窗口或屏幕,set_mode(resolution=(0,0), flags=0, depth=0) -> Surface
screen=pygame.display.set_mode(size)
pygame.display.set_caption('企鹅碰碰碰!')
#默认偏移量,,越大,每次move就移动的多,可看作速度
speedlist=[[0,0],[1,1],[2,2],[3,3],[4,4],[5,5],[6,6],[7,7],[8,8],[9,9],[10,10]]
num=-1
for speed in speedlist:
num+=1
#得到初始速度speedNum
locals()['speed'+str(num)]=speed
#得到photoNum
locals()['photo'+str(num)]=pygame.image.load('企鹅.png')
#得到positionNum
locals()['position'+str(num)]=locals()['photo'+str(num)].get_rect()
while True:
for event in pygame.event.get():
#如果事件类型是退出,就退出程序
if event.type==pygame.QUIT:
sys.exit()
'''通过按键,改变偏移量speed'''
#如果事件类型是键盘按键(key 模块)
if event.type==pygame.KEYDOWN:#不想用pygame.则可以在前面加from pygame.locals import *
#如果按下左键,左移
if event.key==pygame.K_LEFT:
speed0=[-1,0]
#如果按下右键,右移
if event.key==pygame.K_RIGHT:
speed0=[1,0]
#如果按下上键,上移
if event.key==pygame.K_UP:
speed0=[0,-1]
#如果按下下键,下移
if event.key==pygame.K_DOWN:
speed0=[0,1]
#初始化为-1
num=-1
for speed in speedlist:
num+=1
#根据speed移动改变position
locals()['position'+str(num)]=locals()['position'+str(num)].move(locals()['speed'+str(num)])#positionNum=positionNum.move(speedNum)
#出左右界,改变speed,也就是对speed列表第0个位置取反,而第1个位置不变,使得反向移动
if locals()['position'+str(num)].left<=0 or locals()['position'+str(num)].right>=width:
locals()['speed'+str(num)][0]=-locals()['speed'+str(num)][0]
#出上下界,对speed列表第1个位置取反,而第0个位置不变,使得反向移动
if locals()['position'+str(num)].top<=0 or locals()['position'+str(num)].bottom>=height :
locals()['speed'+str(num)][1]=-locals()['speed'+str(num)][1]
#填充一次背景
screen.fill((255,255,255))
num=-1
#将所有待显示的放到缓存
for speed in speedlist:
num+=1
screen.blit(locals()['photo'+str(num)],locals()['position'+str(num)])
#一次性更新整个待显示缓存到屏幕上
pygame.display.flip()
#如果太快可以选择以下两种之一延迟
#pygame.time.delay(10)
#pygame.time.Clock().tick(200)
更改列表。添加元素即可添加企鹅数量,和速度
speedlist=[[0,0],[1,1],[2,2],[3,3],[4,4],[5,5],[6,6],[7,7],[8,8],[9,9],[10,10]]
效果如图:
下面来举个例子,怎么将字符串当作变量名来使用
1,先上个错误的示范,花了好久才解决的
报错,can’t assign to operator 无法分配给运算符
2,再来个正确的操作,在想用做变量的字符串前面加个locals()[],即变量名为locals()[字符串]
3,如果想通过循环得到一系列变量,可以通字符串加法来得到尾部,这样得到的是字符串,可以用来open文件当作文件名,循环创建多个文件,因为是字符串所以可以直接print输出,还是因为是字符串,所以不能直接当作变量名。
如:
speedlist=([0,0],[1,1],[2,2],[3,3])
num=-1
for speed in speedlist:
num+=1
print(num)
locals()['speed'+str(num)]=speed
with open ('speed'+str(num)+'.txt','w') as f:
f.write('6666')
print('speed'+str(num))
print(speed0)
print(speed1)
print(speed2)
得到结果如下图: