TensorFlow2.0 前向传播 – 测试集正确率统计
1.导入与设置
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import datasets
print(tf.__version__)
2.加载数据集
(x, y), (x_test, y_test) = datasets.mnist.load_data()
print(x.shape)
print(y.shape)
print(x_test.shape)
print(y_test.shape)
3.图片预处理
x = tf.convert_to_tensor(x, dtype = tf.float32)
y = tf.convert_to_tensor(y, dtype = tf.int32)
print(x.dtype)
print(y.dtype)
x_test = tf.convert_to_tensor(x_test, dtype = tf.float32)
y_test = tf.convert_to_tensor(y_test, dtype = tf.int32)
print(x_test.dtype)
print(y_test.dtype)
print(tf.reduce_min(x), tf.reduce_max(x))
print(tf.reduce_min(y), tf.reduce_max(y))
x = x / 255.0
x_test = x_test / 255.0
print(tf.reduce_min(x), tf.reduce_max(x))
print(tf.reduce_min(x_test), tf.reduce_max(x_test))
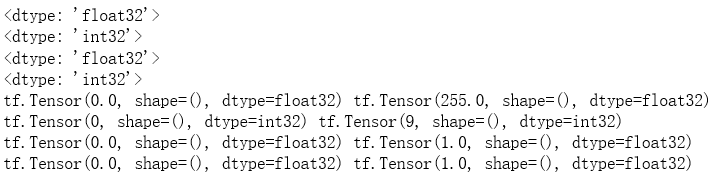
4.数据集制作 变量初始化
train_db = tf.data.Dataset.from_tensor_slices((x, y)).batch(128)
test_db = tf.data.Dataset.from_tensor_slices((x_test, y_test)).batch(128)
train_iter = iter(train_db)
sample = next(train_iter)
print("batch:", sample[0].shape, sample[1].shape)
test_iter = iter(test_db)
test_sample = next(test_iter)
print("test batch:", test_sample[0].shape, test_sample[1].shape)
lr = 1e-3
w1 = tf.Variable(tf.random.truncated_normal([784, 256], stddev=0.1))
b1 = tf.Variable(tf.zeros([256]))
w2 = tf.Variable(tf.random.truncated_normal([256, 128], stddev=0.1))
b2 = tf.Variable(tf.zeros([128]))
w3 = tf.Variable(tf.random.truncated_normal([128, 10], stddev=0.1))
b3 = tf.Variable(tf.zeros([10]))
5.数据集制作 测试集 准确率统计
for epoch in range(10):
for step, (x, y) in enumerate(train_db):
x = tf.reshape(x, [-1, 28*28])
with tf.GradientTape() as tape:
h1 = x @ w1 + tf.broadcast_to(b1, [x.shape[0], 256])
h1 = tf.nn.relu(h1)
h2 = h1 @ w2 + b2
h2 = tf.nn.relu(h2)
out = h2 @ w3 + b3
y_onehot = tf.one_hot(y, depth=10)
loss = tf.square(y_onehot - out)
loss = tf.reduce_mean(loss)
grads = tape.gradient(loss, [w1, b1, w2, b2, w3, b3])
w1.assign_sub(lr*grads[0])
b1.assign_sub(lr*grads[1])
w2.assign_sub(lr*grads[2])
b2.assign_sub(lr*grads[3])
w3.assign_sub(lr*grads[4])
b3.assign_sub(lr*grads[5])
if step % 100 == 0:
print("epoch=", epoch, "step=", step, " loss:", float(loss))
total_correct = 0
total_num = 0
for step, (x, y) in enumerate(test_db):
x = tf.reshape(x, [-1, 28*28])
h1 = tf.nn.relu(x @ w1 + b1)
h2 = tf.nn.relu(h1 @ w2 + b2)
out = h2 @ w3 + b3
prob = tf.nn.softmax(out, axis=1)
pred = tf.argmax(prob, axis=1)
pred = tf.cast(pred, dtype=tf.int32)
correct = tf.cast(tf.equal(pred, y), dtype=tf.int32)
correct_sum = tf.reduce_sum(correct)
total_correct += correct_sum
total_num += x.shape[0]
acc = total_correct / total_num
print("acc:", acc.numpy())
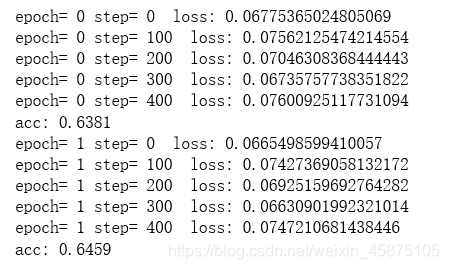