目录
1 . rear指向队尾指针后一个位置 牺牲一个存储空间来区分队空和队满
2 . rear 指向队尾指针后一个位置 增设 size 判断队满 / 空
3 . rear 指向队尾指针后一个位置 增设 tag 判断
4 . rear 指向队尾 牺牲一个存储空间来区分队空和队满
5 . rear 指向队尾 增设 size 判断队满 / 空
一 . 队列的顺序存储结构
1 . rear指向队尾指针后一个位置 牺牲一个存储空间来区分队空和队满
#include <iostream>
// rear指向队尾指针后一个位置 牺牲一个存储空间来区分队空和队满
using namespace std;
#define MaxSize 10//队列元素的最大个数
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
} SqQueue;
//初始化队列
void InitQueue(SqQueue &Q) {
//初始时 队头、队尾指针指向 0
Q.front = Q.rear = 0;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if(Q.front == Q.rear) { //队列为空
return true;
} else {
return false;
}
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if((Q.rear + 1) % MaxSize == Q.front) {
return true;
} else {
return false;
}
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if(QueueFull(Q)) {
return false;
}
Q.data[Q.rear] = x; // x 插入队尾
Q.rear = (Q.rear + 1) % MaxSize; //队尾指针加 1 取模
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if(QueueEmpty(Q)) { //队列为空
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if(QueueEmpty(Q)) { //队列为空
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素个数
int GetQueueLength(SqQueue &Q) {
int len = 0;
len = (Q.rear + MaxSize - Q.front) % MaxSize;
return len;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 10; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-1 代码运行结果:
2 . rear 指向队尾指针后一个位置 增设 size 判断队满 / 空
#include <iostream>
// rear 指向队尾指针后一个位置 增设 size 判断队满/空
//每次入队 size + 1
//每次出队 size - 1
using namespace std;
#define MaxSize 10//队列元素的最大个数
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
int size;//队列当前长度
} SqQueue;
//初始化队列
void InitQueue(SqQueue &Q) {
//初始时 队头、队尾指针指向 0
Q.front = Q.rear = Q.size = 0;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if(Q.size == 0) { //队列为空
return true;
} else {
return false;
}
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if(Q.size == MaxSize) {
return true;
} else {
return false;
}
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if(QueueFull(Q)) {
return false;
}
Q.data[Q.rear] = x; // x 插入队尾
Q.rear = (Q.rear + 1) % MaxSize; //队尾指针加 1 取模
Q.size++;
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if(QueueEmpty(Q)) { //队列为空
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
Q.size--;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if(QueueEmpty(Q)) { //队列为空
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素个数
int GetQueueLength(SqQueue &Q) {
return Q.size;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 10; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-2 代码运行结果:
3 . rear 指向队尾指针后一个位置 增设 tag 判断
#include <iostream>
// rear 指向队尾指针后一个位置 增设 tag 判断
//每次出队操作成功时 令 tag = 0 只有出队操作 才会导致队空
//队空条件 front == rear && tag == 0
//每次入队操作成功时 令 tag = 1 只有入队操作 才会导致队满
//队满条件 front == rear && tag == 1
using namespace std;
#define MaxSize 10
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
int tag;
} SqQueue;
//初始化
void InitQueue(SqQueue &Q) {
Q.front = Q.rear = Q.tag = 0;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if (Q.front == Q.rear && Q.tag == 0) {
return true;
}
return false;
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if (Q.front == Q.rear && Q.tag == 1) {
return true;
}
return false;
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if (QueueFull(Q)) {//队满
return false;
}
Q.data[Q.rear] = x;
Q.rear = (Q.rear + 1) % MaxSize;
Q.tag = 1;
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
Q.tag = 0;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素的个数
int GetQueueLength(SqQueue Q) {
if(QueueEmpty(Q)) {
return 0;
}
if(QueueFull(Q)) {
return MaxSize;
}
return (Q.rear + MaxSize - Q.front) % MaxSize;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 10; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-3 代码运行结果:
4 . rear 指向队尾 牺牲一个存储空间来区分队空和队满
#include <iostream>
// rear 指向队尾 牺牲一个存储空间来区分队空和队满
//队空条件 (Q.rear + 1) % MaxSize == Q.front
//队满条件 (Q.rear + 2) % MaxSize == Q.front
using namespace std;
#define MaxSize 10
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
} SqQueue;
//初始化
void InitQueue(SqQueue &Q) {
Q.front = 0;
Q.rear = MaxSize - 1;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if ((Q.rear + 1) % MaxSize == Q.front) {
return true;
}
return false;
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if ((Q.rear + 2) % MaxSize == Q.front) {
return true;
}
return false;
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if (QueueFull(Q)) {//队满
return false;
}
Q.rear = (Q.rear + 1) % MaxSize;
Q.data[Q.rear] = x;
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素的个数
int GetQueueLength(SqQueue Q) {
if(QueueEmpty(Q)) {
return 0;
}
if(QueueFull(Q)) {
return MaxSize - 1;
}
return (Q.rear + MaxSize + 1 - Q.front) % MaxSize;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 4; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-4 代码运行结果:
5 . rear 指向队尾 增设 size 判断队满 / 空
#include <iostream>
// rear 指向队尾 增设 size 判断队满/空
//每次入队 size + 1
//每次出队 size - 1
using namespace std;
#define MaxSize 10
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
int size;
} SqQueue;
//初始化
void InitQueue(SqQueue &Q) {
Q.front = 0;
Q.rear = MaxSize - 1;
Q.size = 0;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if (Q.size == 0) {
return true;
}
return false;
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if (Q.size == MaxSize) {
return true;
}
return false;
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if (QueueFull(Q)) {//队满
return false;
}
Q.rear = (Q.rear + 1) % MaxSize;
Q.data[Q.rear] = x;
Q.size++;
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
Q.size--;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素的个数
int GetQueueLength(SqQueue Q) {
return Q.size;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 10; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-5 代码运行结果:
6 . rear 指向队尾 增设 tag 判断
#include <iostream>
// rear 指向队尾 增设 tag 判断
//每次出队操作成功时 令 tag = 0 只有出队操作 才会导致队空
//队空条件 Q.front == (Q.rear + 1) % MaxSize && Q.tag == 0
//每次入队操作成功时 令 tag = 1 只有入队操作 才会导致队满
//队满条件 Q.front == (Q.rear + 1) % MaxSize && Q.tag == 1
using namespace std;
#define MaxSize 10
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//用静态数组存放队列元素
int front, rear;//队头指针和队尾指针
int tag;
} SqQueue;
//初始化
void InitQueue(SqQueue &Q) {
Q.front = 0;
Q.rear = MaxSize - 1;
Q.tag = 0;
}
//判断队列是否为空
bool QueueEmpty(SqQueue Q) {
if (Q.front == (Q.rear + 1) % MaxSize && Q.tag == 0) {
return true;
}
return false;
}
//判断队列是否已满
bool QueueFull(SqQueue Q) {
if (Q.front == (Q.rear + 1) % MaxSize && Q.tag == 1) {
return true;
}
return false;
}
//入队
bool EnQueue(SqQueue &Q, ElemType x) {
if (QueueFull(Q)) {//队满
return false;
}
Q.rear = (Q.rear + 1) % MaxSize;
Q.data[Q.rear] = x;
Q.tag = 1;
return true;
}
//出队
bool DeQueue(SqQueue &Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MaxSize;
Q.tag = 0;
return true;
}
//获得队头元素的值
bool GetHead(SqQueue Q, ElemType &x) {
if (QueueEmpty(Q)) {
return false;
}
x = Q.data[Q.front];
return true;
}
//队列元素的个数
int GetQueueLength(SqQueue Q) {
if(QueueEmpty(Q)) {
return 0;
}
if(QueueFull(Q)) {
return MaxSize;
}
return (Q.rear + MaxSize + 1 - Q.front) % MaxSize;
}
void test() {
SqQueue Q;
InitQueue(Q);
for (int i = 1; i < 10; i++) {
EnQueue(Q, i);
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
if (!EnQueue(Q, 10)) {
cout << "Is Full,Insertion Failed!" << endl;
} else {
cout << "Inserted successfully!" << endl;
}
cout << "GetQueueLength:" << GetQueueLength(Q) << endl;
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
1-6 代码运行结果: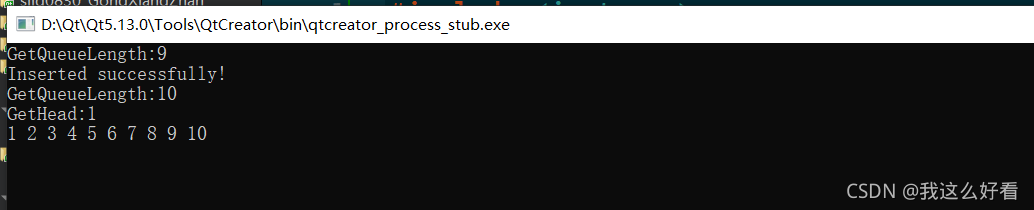
二 . 队列的链式存储
1 . 链式队列 (带头结点)
#include <iostream>
//链式队列 (带头结点)
using namespace std;
typedef int ElemType;
typedef struct LinkNode { //链式队列结点
ElemType data;
struct LinkNode *next;
} LinkNode;
typedef struct { //链式队列
LinkNode *front, *rear; //链式队列的队头和队尾指针
} LinkQueue;
//初始化
void InitQueue(LinkQueue &Q) {
Q.front = Q.rear = (LinkNode *)malloc(sizeof(LinkNode));//建立头结点
Q.front->next = NULL;//初始为空
}
//判断队列为空
bool QueueEmpty(LinkQueue Q) {
if(Q.front == Q.rear) {
return true;
} else {
return false;
}
}
//入队
bool EnQueue(LinkQueue &Q, ElemType x) {
LinkNode *s = (LinkNode *)malloc(sizeof(LinkNode));
if(!s) { //空间申请失败
return false;
}
//创建新结点插入队尾
s->data = x;
s->next = NULL;
Q.rear->next = s;
Q.rear = s;
}
//出队
bool DeQueue(LinkQueue &Q, ElemType &x) {
if(QueueEmpty(Q)) { //空队
return false;
}
LinkNode *p = Q.front->next;
x = p->data;
Q.front->next = p->next;
if(Q.rear == p) { //队列最后一个元素
Q.front = Q.rear;
}
free(p);
return true;
}
//获得队头元素
bool GetHead(LinkQueue Q, ElemType &x) {
if(QueueEmpty(Q)) { //空队
return false;
}
x = Q.front->next->data;
return true;
}
void test() {
LinkQueue Q;
InitQueue(Q);
for (int i = 0; i < 10; i++) {
EnQueue(Q, i);
}
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while(!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
2-1 代码运行结果: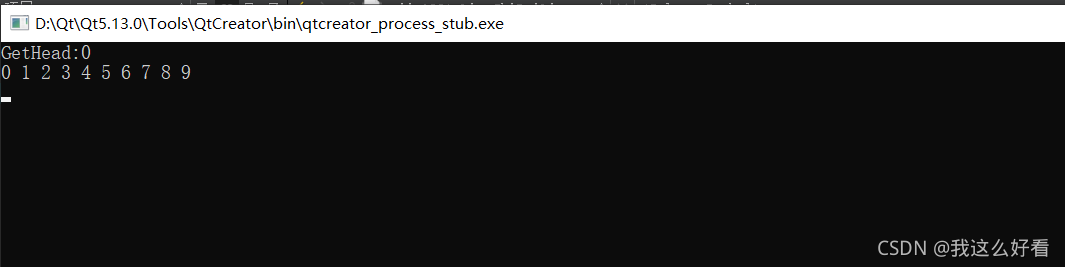
2 . 链式队列 (不带头结点)
#include <iostream>
//链式队列 (不带头结点)
using namespace std;
typedef int ElemType;
typedef struct LinkNode { //链式队列结点
ElemType data;
struct LinkNode *next;
} LinkNode;
typedef struct { //链式队列
LinkNode *front, *rear; //链式队列的队头和队尾指针
} LinkQueue;
//初始化
void InitQueue(LinkQueue &Q) {
Q.front = Q.rear = NULL; //初始为空
}
//判断队列为空
bool QueueEmpty(LinkQueue Q) {
if(Q.front == NULL) {
return true;
} else {
return false;
}
}
//入队
bool EnQueue(LinkQueue &Q, ElemType x) {
LinkNode *s = (LinkNode *)malloc(sizeof(LinkNode));
if(!s) { //空间申请失败
return false;
}
//创建新结点插入队尾
s->data = x;
s->next = NULL;
if(QueueEmpty(Q)) {
Q.front = Q.rear = s;
} else {
Q.rear->next = s;
Q.rear = s;
}
return true;
}
//出队
bool DeQueue(LinkQueue &Q, ElemType &x) {
if(QueueEmpty(Q)) { //空队
return false;
}
LinkNode *p = Q.front;
x = p->data;
if(Q.rear == p) { //队列最后一个元素
Q.front = Q.rear = NULL;
} else {
Q.front = p->next;
}
free(p);
return true;
}
//获得队头元素
bool GetHead(LinkQueue Q, ElemType &x) {
if(QueueEmpty(Q)) { //空队
return false;
}
x = Q.front->data;
return true;
}
void test() {
LinkQueue Q;
InitQueue(Q);
for (int i = 0; i < 10; i++) {
EnQueue(Q, i);
}
ElemType x;
GetHead(Q, x);
cout << "GetHead:" << x << endl;
while(!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
2-2 代码运行结果:
3 . 双端队列
//双端队列 //1. 输入受限的双端队列 可以两端删除 但只可一端输入 //2. 输出受限的双端队列 可以两端输入 但只可一端删除 //双端队列具有栈的所有功能 //!!!考点 //判断输出序列的合法性