职工管理系统项目
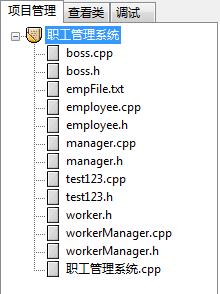
worker.h
#pragma once
#include <iostream>
#include <string>
using namespace std;
class Worker
{
public:
virtual void showInfo() = 0;
virtual string getDeptName() = 0;
int m_Id;
string m_Name;
int m_DeptId;
};
employee.h
#pragma once
#include <iostream>
using namespace std;
#include "worker.h"
class Employee: public Worker
{
public:
Employee(int id, string name, int dId);
virtual void showInfo();
virtual string getDeptName();
};
employee.cpp
#include "employee.h"
Employee::Employee(int id, string name, int dId)
{
this->m_Id = id;
this->m_Name = name;
this->m_DeptId = dId;
}
void Employee::showInfo()
{
cout<<"职工编号: "<<this->m_Id
<<"\t职工姓名: "<<this->m_Name
<<"\t岗位: "<<this->getDeptName()
<<"\t岗位职责: 完成经理交给的任务"<<endl;
}
string Employee::getDeptName()
{
return string("员工");
}
manager.h
#pragma once
#include <iostream>
using namespace std;
#include "worker.h"
class Manager:public Worker
{
public:
Manager(int id, string name, int dId);
virtual void showInfo();
virtual string getDeptName();
};
manager.cpp
#include "manager.h"
Manager::Manager(int id, string name, int dId)
{
this->m_Id = id;
this->m_Name = name;
this->m_DeptId = dId;
}
void Manager::showInfo()
{
cout<<"职工编号: "<<this->m_Id
<<"\t职工姓名: "<<this->m_Name
<<"\t岗位: "<<this->getDeptName()
<<"\t岗位职责: 完成老板交给的任务,并下发任务给员工"<<endl;
}
string Manager::getDeptName()
{
return string("经理");
}
boss.h
#pragma once
#include <iostream>
using namespace std;
#include "worker.h"
class Boss: public Worker
{
public:
Boss(int id, string name, int dId);
virtual void showInfo();
virtual string getDeptName();
};
boss.cpp
#include "boss.h"
Boss::Boss(int id, string name, int dId)
{
this->m_Id = id;
this->m_Name = name;
this->m_DeptId = dId;
}
void Boss::showInfo()
{
cout<<"职工编号: "<<this->m_Id
<<"\t职工姓名: "<<this->m_Name
<<"\t岗位: "<<this->getDeptName()
<<"\t岗位职责: 管理公司所有事务"<<endl;
}
string Boss::getDeptName()
{
return string("总裁");
}
test123.h
#include "worker.h"
#include "employee.h"
#include "manager.h"
#include "boss.h"
void test();
test123.cpp
#include "test123.h"
void test()
{
Worker * worker = NULL;
worker = new Employee(1,"张三",1);
worker->showInfo();
delete worker;
worker = new Manager(2,"李四",2);
worker->showInfo();
delete worker;
worker = new Boss(3,"王五",3);
worker->showInfo();
delete worker;
}
workerManager.h
#pragma once
#include <iostream>
using namespace std;
#include <string>
#include "worker.h"
#include "employee.h"
#include "manager.h"
#include "boss.h"
#include <fstream>
#define FILENAME "empFile.txt"
class WorkerManager
{
public:
WorkerManager();
void Show_Menu();
void Add_Emp();
void save();
void ExitSystem();
int m_EmpNum;
Worker ** m_EmpArray;
bool m_FileIsEmpty;
int get_EmpNum();
void init_Emp();
void Show_Emp();
void Del_Emp();
int IsExist(int id);
void Mod_Emp();
void Find_Emp();
void Sort_Emp();
void Clean_File();
~WorkerManager();
};
workerManager.cpp
#include "workerManager.h"
WorkerManager::WorkerManager()
{
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
ifstream ifs;
ifs.open(FILENAME, ios::in);
this->m_FileIsEmpty=false;
if(!ifs.is_open())
{
this->m_EmpNum=0;
this->m_FileIsEmpty=true;
this->m_EmpArray=NULL;
ifs.close();
return;
}
char ch;
ifs>>ch;
if(ifs.eof())
{
this->m_EmpNum=0;
this->m_FileIsEmpty=true;
this->m_EmpArray=NULL;
ifs.close();
return;
}
int num=this->get_EmpNum();
this->m_EmpNum=num;
this->m_EmpArray=new Worker * [this->m_EmpNum];
init_Emp();
}
void WorkerManager::Show_Menu()
{
cout<<"*****************************************"<<endl;
cout<<"********* 欢迎使用职工管理系统!*********"<<endl;
cout<<"********* 0.退出管理程序 *********"<<endl;
cout<<"********* 1.增加职工信息 *********"<<endl;
cout<<"********* 2.显示职工信息 *********"<<endl;
cout<<"********* 3.删除离职职工 *********"<<endl;
cout<<"********* 4.修改职工信息 *********"<<endl;
cout<<"********* 5.查找职工信息 *********"<<endl;
cout<<"********* 6.按照编号排序 *********"<<endl;
cout<<"********* 7.清空所有文档 *********"<<endl;
cout<<"*****************************************"<<endl;
cout<<endl;
}
void WorkerManager::Add_Emp()
{
cout<<"请输入增加职工数量: "<<endl;
int addNum = 0;
cin>>addNum;
if(addNum>0)
{
int newSize = this->m_EmpNum + addNum;
Worker ** newSpace = new Worker * [newSize];
if(this->m_EmpNum != NULL)
{
for(int i=0; i<this->m_EmpNum;i++)
{
newSpace[i] = this->m_EmpArray[i];
}
}
for(int i=0;i<addNum;i++)
{
int id;
string name;
int dSelect;
cout<<"请输入第 "<<i+1<<" 个新职工编号:"<<endl;
cin>>id;
cout<<"请输入第 "<<i+1<<" 个新职工姓名:"<<endl;
cin>>name;
cout<<"请选择该职工的岗位:"<<endl;
cout<<"1. 普通职工"<<endl;
cout<<"2. 经理"<<endl;
cout<<"3. 老板"<<endl;
cin>>dSelect;
Worker * worker = NULL;
switch(dSelect)
{
case 1:
worker = new Employee(id,name,1);
break;
case 2:
worker = new Manager(id,name,2);
break;
case 3:
worker = new Boss(id,name,3);
break;
default:
break;
}
newSpace[this->m_EmpNum+i] = worker;
}
delete[] this->m_EmpArray;
this->m_EmpArray = newSpace;
this->m_EmpNum = newSize;
this->m_FileIsEmpty=false;
cout<<"成功添加 "<<addNum<<" 名新职工!"<<endl;
this->save();
}
else
{
cout<<"输入有误"<<endl;
}
system("pause");
system("cls");
}
void WorkerManager::save()
{
ofstream ofs;
ofs.open(FILENAME, ios::out);
for(int i=0;i<this->m_EmpNum;i++)
{
ofs<<this->m_EmpArray[i]->m_Id<<" "
<<this->m_EmpArray[i]->m_Name<<" "
<<this->m_EmpArray[i]->m_DeptId<<endl;
}
ofs.close();
}
int WorkerManager:: get_EmpNum()
{
ifstream ifs;
ifs.open(FILENAME,ios::in);
int id;
string name;
int dId;
int num=0;
while(ifs>>id&&ifs>>name&&ifs>>dId)
{
num++;
}
ifs.close();
return num;
}
void WorkerManager::init_Emp()
{
ifstream ifs;
ifs.open(FILENAME,ios::in);
int id;
string name;
int dId;
int index=0;
while(ifs>>id&&ifs>>name&&ifs>>dId)
{
Worker * worker = NULL;
if(dId==1)
{
worker=new Employee(id,name,dId);
}
else if(dId==2)
{
worker=new Manager(id,name,dId);
}
else
{
worker=new Boss(id,name,dId);
}
this->m_EmpArray[index]=worker;
index++;
}
}
void WorkerManager::Show_Emp()
{
if(this->m_FileIsEmpty)
{
cout<<"文件不存在或者记录为空!"<<endl;
}
else
{
for(int i=0;i<m_EmpNum;i++)
{
this->m_EmpArray[i]->showInfo();
}
}
system("pause");
system("cls");
}
void WorkerManager::Del_Emp()
{
if(this->m_FileIsEmpty)
{
cout<<"文件不存在或者记录为空!"<<endl;
}
else
{
cout<<"请输入想要删除的职工的编号: "<<endl;
int id=0;
cin>>id;
int index=this->IsExist(id);
if(index!=-1)
{
for(int i=index;i<this->m_EmpNum-1;i++)
{
this->m_EmpArray[i]=this->m_EmpArray[i+1];
}
this->m_EmpNum--;
this->save();
cout<<"删除成功!"<<endl;
}
else
{
cout<<"删除失败,未找到该职工!"<<endl;
}
}
system("pause");
system("cls");
}
int WorkerManager::IsExist(int id)
{
int index=-1;
for(int i=0;i<this->m_EmpNum;i++)
{
if(this->m_EmpArray[i]->m_Id==id)
{
index=i;
break;
}
}
return index;
}
void WorkerManager::Mod_Emp()
{
if(this->m_FileIsEmpty)
{
cout<<"文件不存在或者记录为空!"<<endl;
}
else
{
cout<<"请输入修改职工的编号:"<<endl;
int id;
cin>>id;
int ret = this->IsExist(id);
if(ret != -1)
{
delete this->m_EmpArray[ret];
int newId = 0;
string newName = "";
int dSelect = 0;
cout<<"查到:"<<id<<" 号职工,请输入新职工号:"<<endl;
cin>>newId;
cout<<"请输入新姓名:"<<endl;
cin>>newName;
cout<<"请选择该职工的岗位:"<<endl;
cout<<"1. 普通职工"<<endl;
cout<<"2. 经理"<<endl;
cout<<"3. 老板"<<endl;
cin>>dSelect;
Worker * worker = NULL;
switch(dSelect)
{
case 1:
worker = new Employee(newId,newName,dSelect);
break;
case 2:
worker = new Manager(newId,newName,dSelect);
break;
case 3:
worker = new Boss(newId,newName,dSelect);
break;
default:
break;
}
this->m_EmpArray[ret] = worker;
cout<<"修改成功!"<<this->m_EmpArray[ret]->m_DeptId<<endl;
this->save();
}
else
{
cout<<"修改失败,查无此人。"<<endl;
}
}
system("pause");
system("cls");
}
void WorkerManager::Find_Emp()
{
if(this->m_FileIsEmpty)
{
cout<<"文件不存在或者记录为空!"<<endl;
}
else
{
cout<<"请输入查找的方式:"<<endl;
cout<<"1.按职工编号查找"<<endl;
cout<<"2.按姓名查找"<<endl;
int select = 0;
cin>>select;
if(select==1)
{
int id;
cout<<"请输入查找的职工编号:"<<endl;
cin>>id;
int ret = IsExist(id);
if(ret != -1)
{
cout<<"查找成功!该职工信息如下:"<<endl;
this->m_EmpArray[ret]->showInfo();
}
else
{
cout<<"查找失败,查无此人。"<<endl;
}
}
else if(select==2)
{
string name;
cout<<"请输入查找的姓名:"<<endl;
cin>>name;
bool flag=false;
for(int i=0;i<m_EmpNum;i++)
{
if(m_EmpArray[i]->m_Name==name)
{
cout<<"查找成功,职工编号为:"
<<m_EmpArray[i]->m_Id
<<" 号的信息如下:"<<endl;
flag = true;
this->m_EmpArray[i]->showInfo();
}
}
if(flag==false)
{
cout<<"查找失败,查无此人。"<<endl;
}
}
else
{
cout<<"输入选项有误。"<<endl;
}
}
system("pause");
system("cls");
}
void WorkerManager::Sort_Emp()
{
if(this->m_FileIsEmpty)
{
cout<<"文件不存在或者记录为空!"<<endl;
system("pause");
system("cls");
}
else
{
cout<<"请选择排序方式:"<<endl;
cout<<"1.按职工号进行升序"<<endl;
cout<<"2.按职工号进行降序"<<endl;
int select = 0;
cin>>select;
for(int i=0;i<m_EmpNum;i++)
{
int minOrMax = i;
for(int j=i+1; j<m_EmpNum; j++)
{
if(select==1)
{
if(m_EmpArray[minOrMax]->m_Id > m_EmpArray[j]->m_Id)
{
minOrMax = j;
}
}
else
{
if(m_EmpArray[minOrMax]->m_Id < m_EmpArray[j]->m_Id)
{
minOrMax = j;
}
}
}
if( i != minOrMax)
{
Worker * temp = m_EmpArray[i];
m_EmpArray[i] = m_EmpArray[minOrMax];
m_EmpArray[minOrMax] = temp;
}
}
cout<<"排序成功,排序后结果为:"<<endl;
this->save();
this->Show_Emp();
}
}
void WorkerManager::Clean_File()
{
cout<<"确认清空?"<<endl;
cout<<"1.确认"<<endl;
cout<<"2.返回"<<endl;
int select = 0;
cin>>select;
if(select==1)
{
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
if(this->m_EmpArray != NULL)
{
for(int i = 0; i < this->m_EmpNum; i++)
{
delete this->m_EmpArray[i];
this->m_EmpArray[i] = NULL;
}
this->m_EmpNum = 0;
delete[] this->m_EmpArray;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = true;
}
cout<<"清空成功!"<<endl;
}
system("pause");
system("cls");
}
void WorkerManager::ExitSystem()
{
cout<<"欢迎下次使用"<<endl;
system("pause");
exit(0);
}
WorkerManager::~WorkerManager()
{
if(this->m_EmpArray != NULL)
{
for(int i = 0; i < this->m_EmpNum; i++)
{
if(this->m_EmpArray[i] != NULL)
{
delete this->m_EmpArray[i];
}
}
delete[] this->m_EmpArray;
this->m_EmpArray = NULL;
}
}
职工管理系统.cpp
#include <iostream>
using namespace std;
#include "workerManager.h"
int main()
{
WorkerManager wm;
int choice = 0;
while (true)
{
wm.Show_Menu();
cout<<"请输入您的选择:"<<endl;
cin>>choice;
switch (choice)
{
case 0:
wm.ExitSystem();
break;
case 1:
wm.Add_Emp();
break;
case 2:
wm.Show_Emp();
break;
case 3:
wm.Del_Emp();
break;
case 4:
wm.Mod_Emp();
break;
case 5:
wm.Find_Emp();
break;
case 6:
wm.Sort_Emp();
break;
case 7:
wm.Clean_File();
break;
default:
system("cls");
break;
}
}
system("pause");
return 0;
}