面试题1
题目:编写代码,以给定值x为基准将链表分割成两部分,所有小于x的结点排在大于或等于x的结点之前 。
如下图:
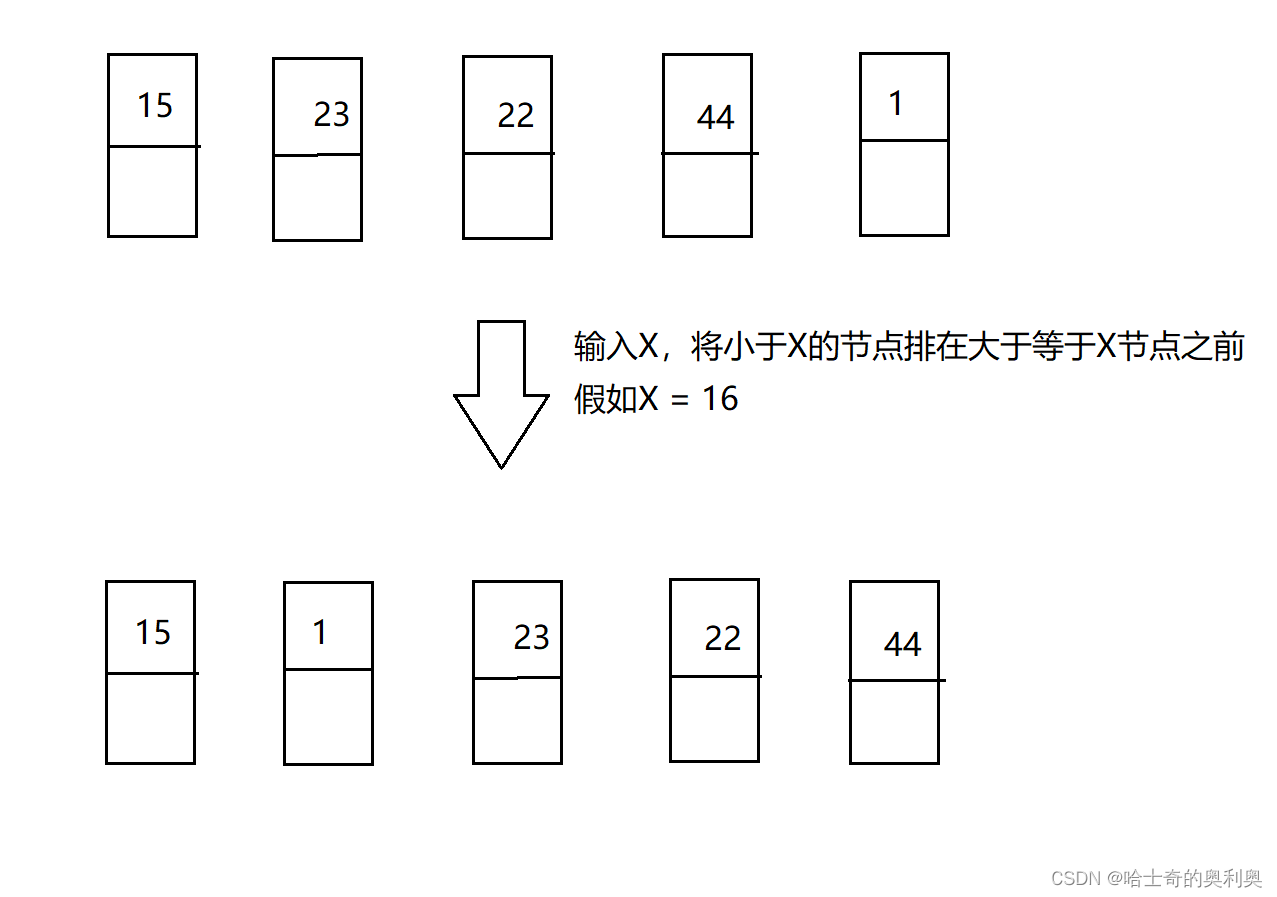
思路:1.定义两块空节点,以及cur节点用来遍历链表,进行与x进行比较,小于的放前面,大于的放后面
2.cur遍历完成将大于的部分插入到小于的部分后面
3.考虑特殊情况,如果都是比x大的(第二段为null)或者两段都为空(链表为空),就直接返回be(后部分的头节点)。
4.如果be不为空,需要将bs.next = null (防止next指向前面)
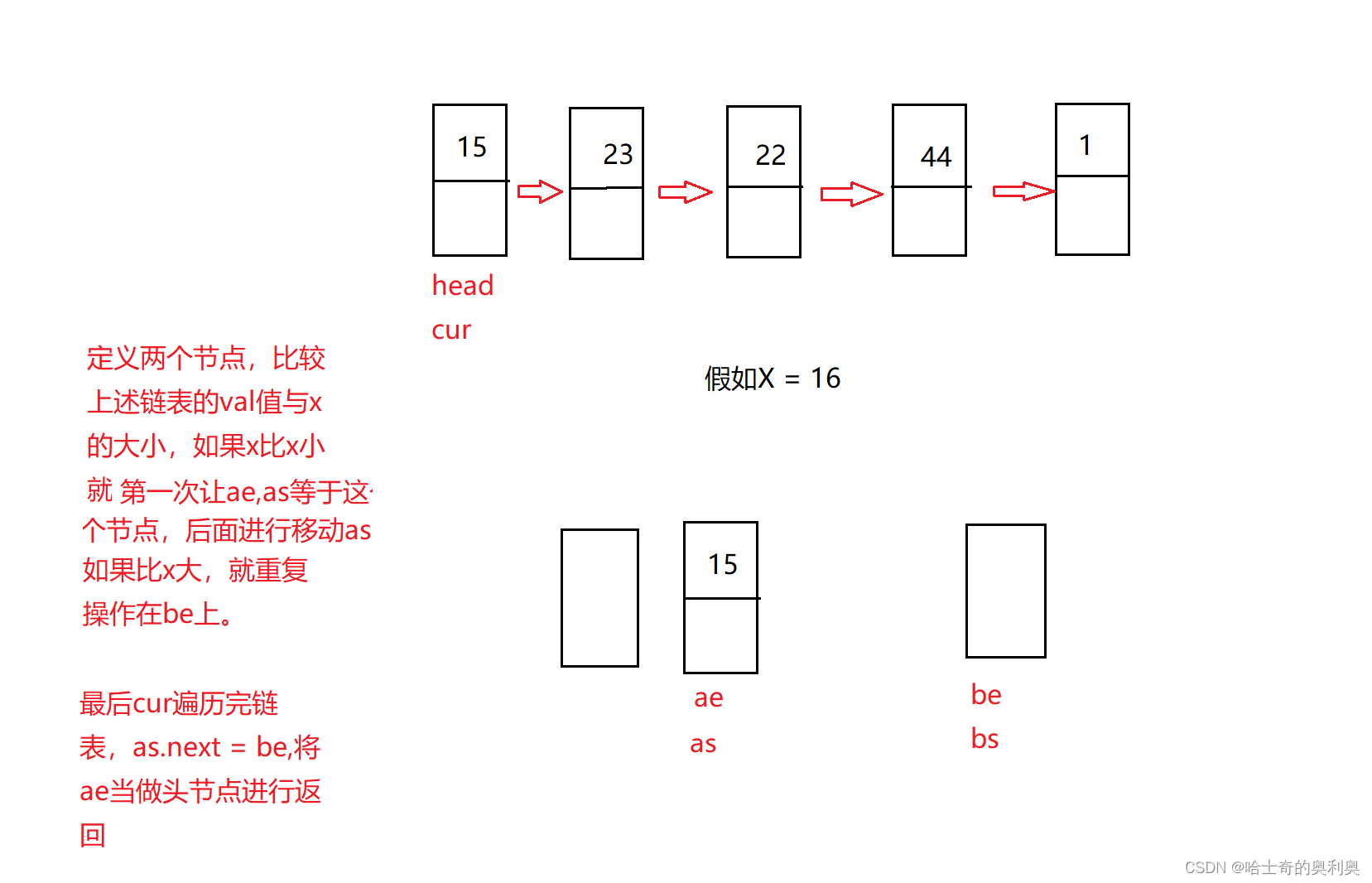
public class Partition {
public ListNode partition(ListNode pHead, int x) {
// write code here
ListNode ae = null;
ListNode as = null;
ListNode be = null;
ListNode bs = null;
ListNode cur = pHead;
while(cur != null){
if(cur.val < x){
if(ae == null){
ae = cur;
as = cur;
cur = cur.next;
}else{
as.next = cur;
as = as.next;
cur = cur.next;
}
}else{
if(be == null){
be = cur;
bs = cur;
cur = cur.next;
}else{
bs.next = cur;
bs = bs.next;
cur = cur.next;
}
}
}
if(as == null){
return be;
}
as.next = be;
if(be != null){
bs.next = null;
}
return ae;
}
}
面试题2
题目: 输入两个链表,找出它们的第一个公共结点
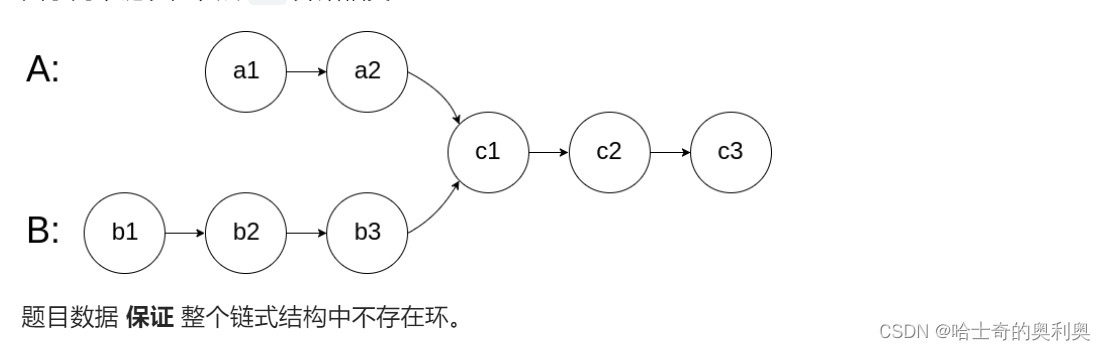
思路:
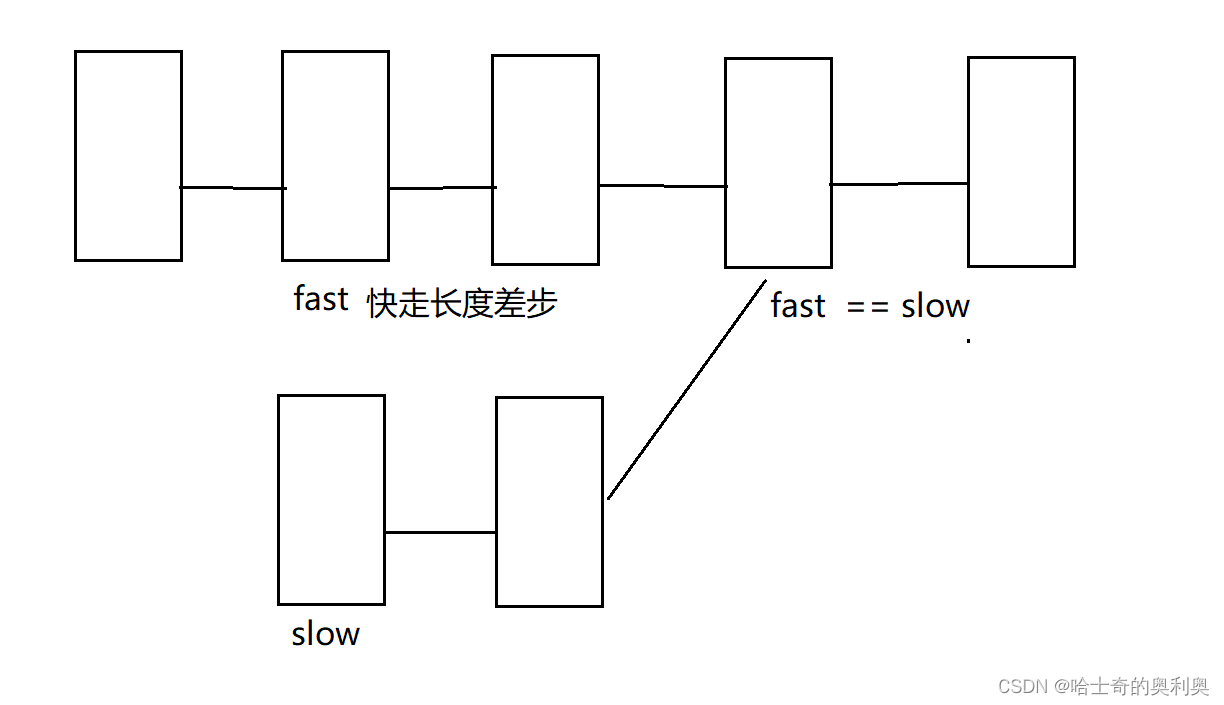
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
if(headA ==null || headB == null){
return null;
}
//1.分别求出长度
int LenA = 0;
int LenB = 0;
ListNode pl = headA;
ListNode ps = headB;
while(pl !=null){
LenA++;
pl = pl.next;
}
while(ps !=null){
LenB++;
ps = ps.next;
}
pl = headA;
ps = headB;
int len = LenA-LenB;
if(len<0){
pl = headB;
ps = headA;
len = LenB - LenA;
}
while(len != 0){
//让长来链表的指针快走长度差步
pl = pl.next;
len--;
}
while(pl != ps){
pl = pl.next;
ps = ps.next;
}
if(pl == null){
return null;
}
return pl;
}
}
代码解释:
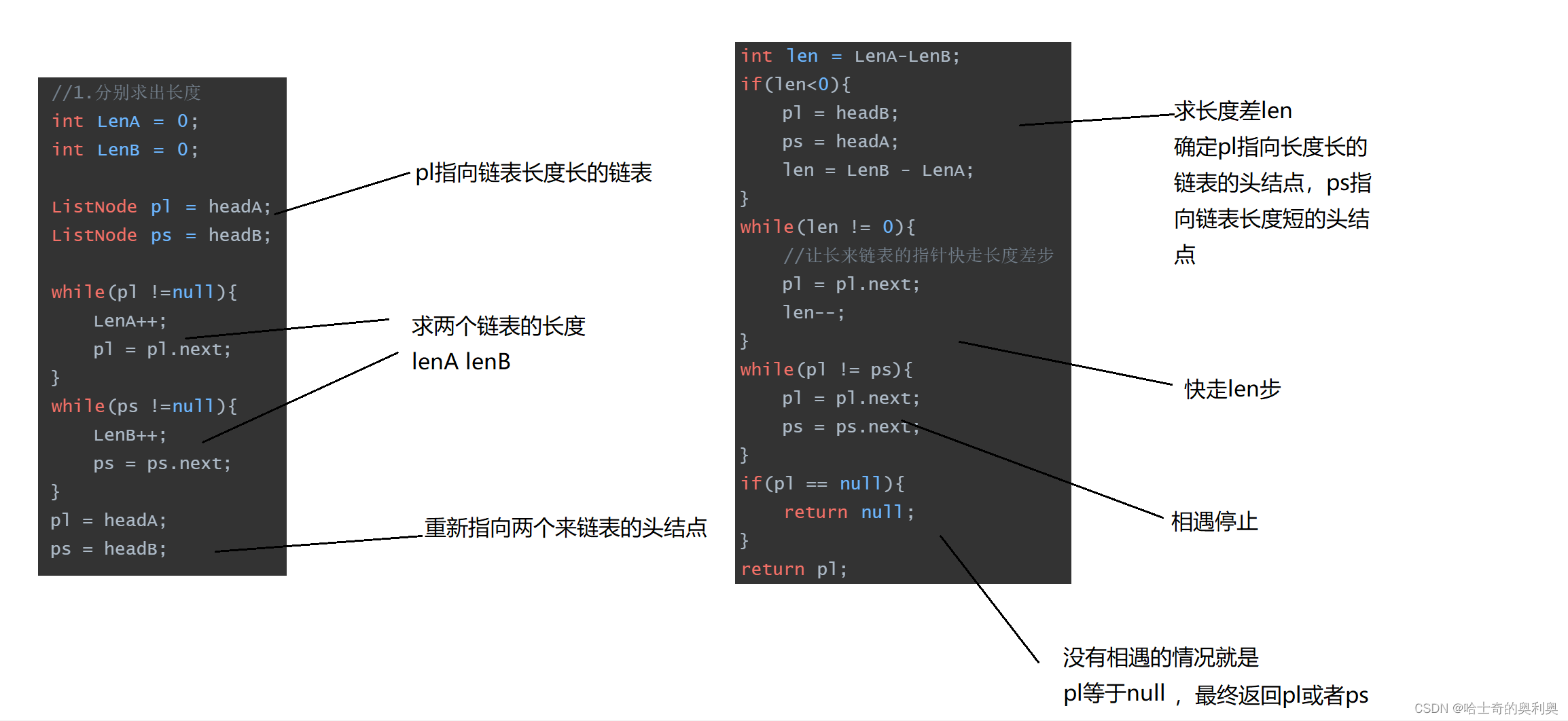
面试题3
题目:给定一个链表,判断链表中是否有环。
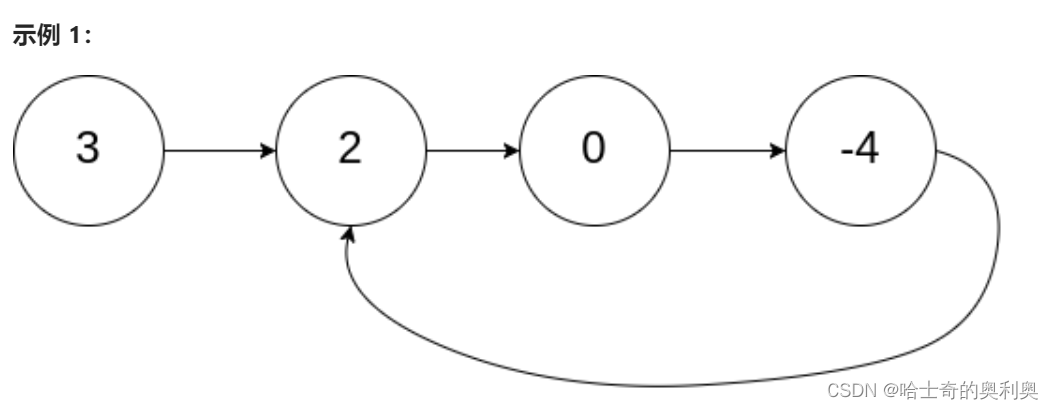
思路:定义快慢指针,fast走2步,slow走1步。slow== fast 返回true
public class Solution {
public boolean hasCycle(ListNode head) {
//快慢指针
ListNode fast = head;
ListNode slow = head;
while(fast!=null && fast.next !=null){
fast = fast.next.next;
slow = slow.next;
if (fast == slow){
return true;
}
}
return false;
}
}
思考:为什么不可以ast走3(大于2)步,slow走1步?
因为:这样有可能在有环的时候永远不会相遇
面试题4
题目:给定一个链表,返回链表开始入环的第一个节点。 如果链表无环,则返回 NULL
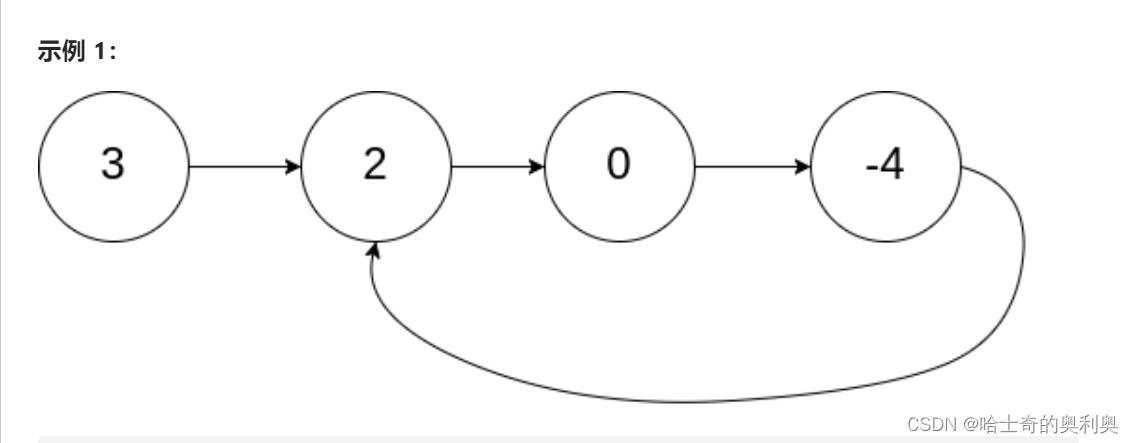
思路:
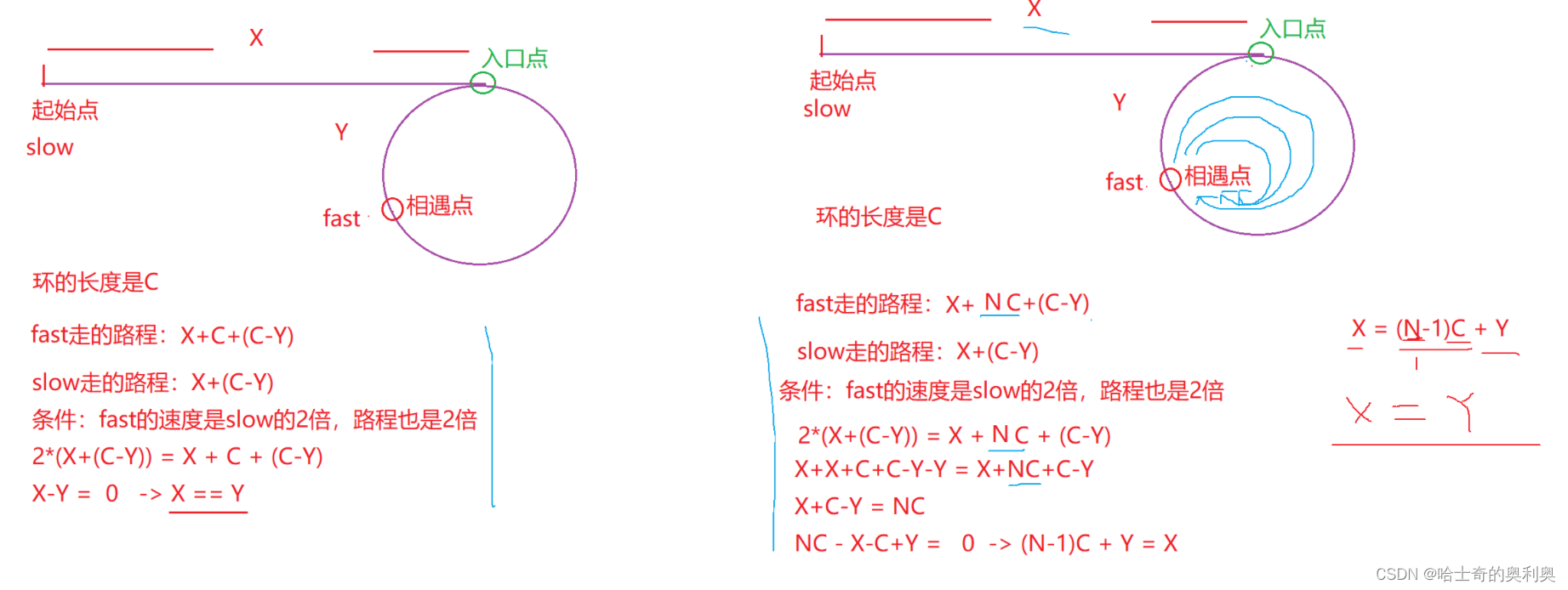
public class Solution {
public ListNode detectCycle(ListNode head) {
// 寻找相遇点,然后将slow指针重新指向head,然后下次相遇点就是入口点
//快慢指针
ListNode fast = head;
ListNode slow = head;
while(fast!=null && fast.next !=null){
fast = fast.next.next;
slow = slow.next;
if (fast == slow){
slow = head;
while(fast != slow){
fast = fast.next;
slow = slow.next;
}
return fast;
}
}
return null;
}
}