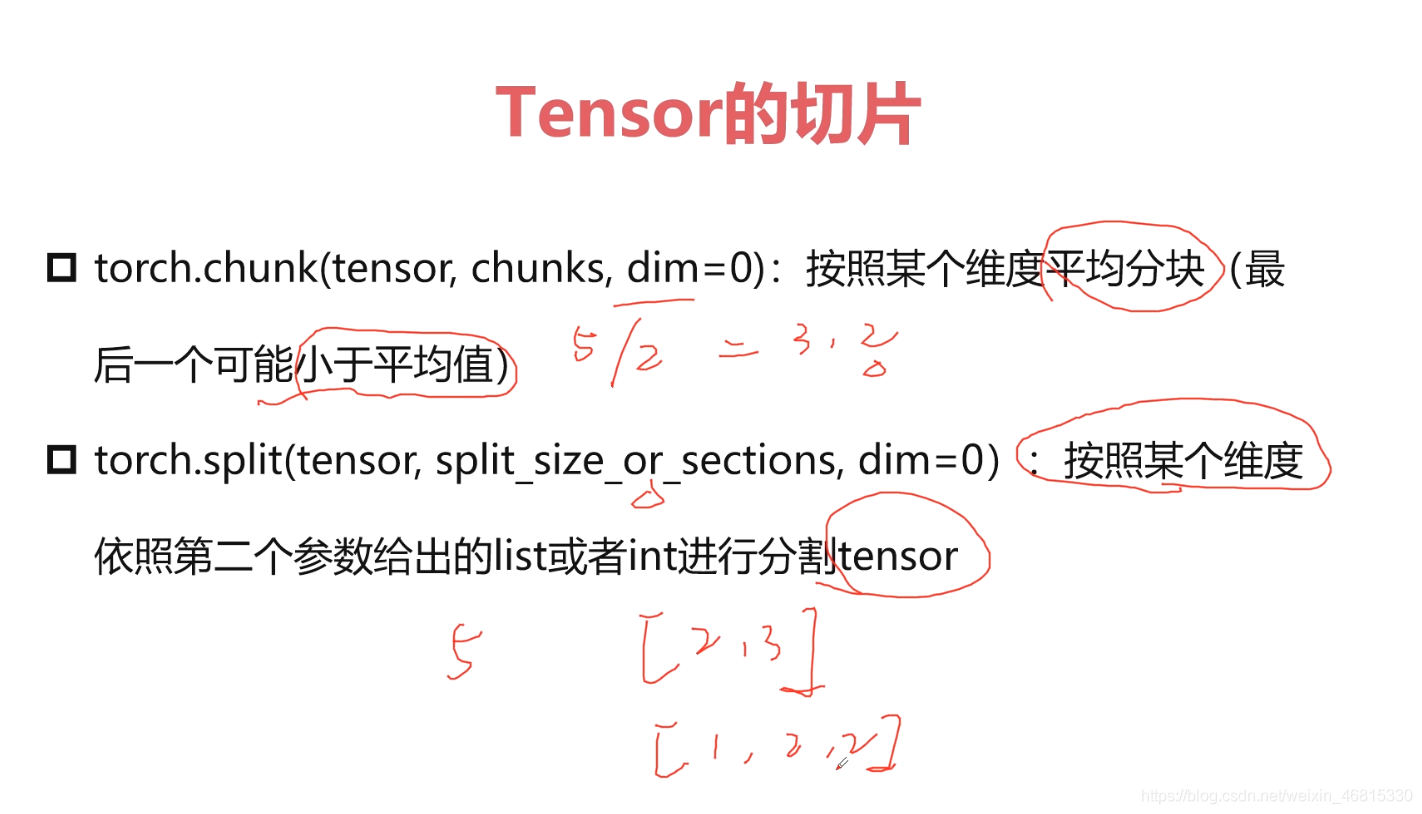
import torch
a = torch.rand((10,4))
print(a)
out = torch.chunk(a,2,dim = 0)
print(out[0],out[0].shape)
print(out[1],out[1].shape)
tensor([[0.4780, 0.9652, 0.8689, 0.6855],
[0.6050, 0.6120, 0.8252, 0.4419],
[0.8837, 0.2002, 0.0820, 0.7129],
[0.7875, 0.6649, 0.1209, 0.1815],
[0.5611, 0.5992, 0.1595, 0.8397],
[0.3782, 0.1737, 0.2253, 0.7230],
[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153],
[0.9524, 0.3471, 0.6847, 0.8776],
[0.4589, 0.3072, 0.7128, 0.6409]])
tensor([[0.4780, 0.9652, 0.8689, 0.6855],
[0.6050, 0.6120, 0.8252, 0.4419],
[0.8837, 0.2002, 0.0820, 0.7129],
[0.7875, 0.6649, 0.1209, 0.1815],
[0.5611, 0.5992, 0.1595, 0.8397]]) torch.Size([5, 4])
tensor([[0.3782, 0.1737, 0.2253, 0.7230],
[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153],
[0.9524, 0.3471, 0.6847, 0.8776],
[0.4589, 0.3072, 0.7128, 0.6409]]) torch.Size([5, 4])
out = torch.chunk(a,2,dim = 1)
print(out[0],out[0].shape)
print(out[1],out[1].shape)
tensor([[0.4780, 0.9652],
[0.6050, 0.6120],
[0.8837, 0.2002],
[0.7875, 0.6649],
[0.5611, 0.5992],
[0.3782, 0.1737],
[0.5463, 0.1684],
[0.6934, 0.2580],
[0.9524, 0.3471],
[0.4589, 0.3072]]) torch.Size([10, 2])
tensor([[0.8689, 0.6855],
[0.8252, 0.4419],
[0.0820, 0.7129],
[0.1209, 0.1815],
[0.1595, 0.8397],
[0.2253, 0.7230],
[0.7635, 0.9761],
[0.9594, 0.8153],
[0.6847, 0.8776],
[0.7128, 0.6409]]) torch.Size([10, 2])
out = torch.split(a,2,dim=0)
print(out)
(tensor([[0.4780, 0.9652, 0.8689, 0.6855],
[0.6050, 0.6120, 0.8252, 0.4419]]), tensor([[0.8837, 0.2002, 0.0820, 0.7129],
[0.7875, 0.6649, 0.1209, 0.1815]]), tensor([[0.5611, 0.5992, 0.1595, 0.8397],
[0.3782, 0.1737, 0.2253, 0.7230]]), tensor([[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153]]), tensor([[0.9524, 0.3471, 0.6847, 0.8776],
[0.4589, 0.3072, 0.7128, 0.6409]]))
out = torch.split(a,3,dim=0)
print(out)
print(len(out))
(tensor([[0.4780, 0.9652, 0.8689, 0.6855],
[0.6050, 0.6120, 0.8252, 0.4419],
[0.8837, 0.2002, 0.0820, 0.7129]]), tensor([[0.7875, 0.6649, 0.1209, 0.1815],
[0.5611, 0.5992, 0.1595, 0.8397],
[0.3782, 0.1737, 0.2253, 0.7230]]), tensor([[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153],
[0.9524, 0.3471, 0.6847, 0.8776]]), tensor([[0.4589, 0.3072, 0.7128, 0.6409]]))
4
tensor([[0.4780, 0.9652, 0.8689, 0.6855],
[0.6050, 0.6120, 0.8252, 0.4419],
[0.8837, 0.2002, 0.0820, 0.7129]]) torch.Size([3, 4])
tensor([[0.7875, 0.6649, 0.1209, 0.1815],
[0.5611, 0.5992, 0.1595, 0.8397],
[0.3782, 0.1737, 0.2253, 0.7230]]) torch.Size([3, 4])
tensor([[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153],
[0.9524, 0.3471, 0.6847, 0.8776]]) torch.Size([3, 4])
tensor([[0.4589, 0.3072, 0.7128, 0.6409]]) torch.Size([1, 4])
out = torch.split(a,[1,3,6],dim=0)
for t in out:
print(t,t.shape)
tensor([[0.4780, 0.9652, 0.8689, 0.6855]]) torch.Size([1, 4])
tensor([[0.6050, 0.6120, 0.8252, 0.4419],
[0.8837, 0.2002, 0.0820, 0.7129],
[0.7875, 0.6649, 0.1209, 0.1815]]) torch.Size([3, 4])
tensor([[0.5611, 0.5992, 0.1595, 0.8397],
[0.3782, 0.1737, 0.2253, 0.7230],
[0.5463, 0.1684, 0.7635, 0.9761],
[0.6934, 0.2580, 0.9594, 0.8153],
[0.9524, 0.3471, 0.6847, 0.8776],
[0.4589, 0.3072, 0.7128, 0.6409]]) torch.Size([6, 4])