1.继承方式
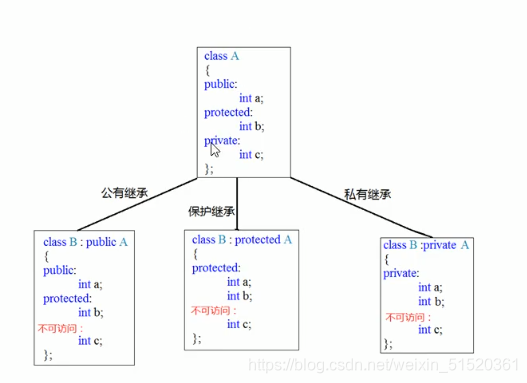
1.1公共继承
#include<string>
using namespace std;
class Base1
{
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son1 :public Base1
{
void func()
{
m_A = 10;
m_B = 10;
}
};
void test01()
{
Son1 s1;
s1.m_A = 100;
}
int main()
{
test01();
system("pause");
return 0;
}
1.2保护继承
class Base2
{
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son2 :protected Base2
{
public:
void func()
{
m_A = 10;
m_B = 10;
}
};
void test02()
{
Son2 s1;
}
1.3私有继承
class Base3
{
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son3 :private Base3
{
public:
void func()
{
m_A = 10;
m_B = 10;
}
};
class GrandSon3 :public Son3
{
public:
void func()
{
}
};
void test03()
{
Son3 s1;
}
2.继承中的对象模型
class Base
{
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son :public Base
{
public:
int m_D;
};
void test01()
{
cout << "size of Son=" << sizeof(Son) << endl;
}
3.继承中的同名成员处理
#include<iostream>
#include<string>
using namespace std;
class Base
{
public:
Base()
{
m_A = 100;
}
void func()
{
cout << "Base-func()调用" << endl;
}
void func(int a)
{
cout << "Base-func(int a)调用" << endl;
}
int m_A;
};
class Son :public Base
{
public:
Son()
{
m_A = 200;
}
void func()
{
cout << "Son-func()调用" << endl;
}
int m_A;
};
void test01()
{
Son s;
cout << "m_A= " << s.m_A << endl;
cout << "m_A= " << s.Base::m_A << endl;
}
void test02()
{
Son s;
s.func();
s.Base::func();
s.Base::func(100);
}
int main()
{
test01();
test02();
system("pause");
return 0;
}
4.继承中的同名静态成员处理方式
#include<iostream>
#include<string>
using namespace std;
class Base
{
public:
static int m_A;
static void func()
{
cout << "Base-static void func()" << endl;
}
static void func(int a)
{
cout << "Base-static void func(int a)" << endl;
}
};
int Base::m_A = 100;
class Son :public Base
{
public:
static int m_A;
static void func()
{
cout << "Son-static void func()" << endl;
}
};
int Son::m_A = 200;
void test01()
{
cout << "通过对象访问:" << endl;
Son s;
cout << "Son 下的m_A= " << s.m_A << endl;
cout << "Base 下的m_A= " << s.Base::m_A << endl;
cout << "通过类名访问:" << endl;
cout << "Son 下的m_A= " << Son::m_A << endl;
cout << "Base 下的m_A= " << Son::Base::m_A << endl;
}
void test02()
{
cout << "通过对象访问:" << endl;
Son s;
s.func();
s.Base::func();
cout << "通过类名访问:" << endl;
Son::func();
Son::Base::func();
Son::Base::func(100);
}
int main()
{
test02();
system("pause");
return 0;
}