1,结构图:
controller层代码:
package com.xiexin.controller;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.xiexin.bean.Student;
import com.xiexin.bean.StudentExample;
import com.xiexin.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@RestController //方法 @Responbady 结合体
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
private Student student;
//mybatis 就是操作数库增删查改
//全查
@RequestMapping("/findAll")
public Map findAll() {
List<Student> students = studentService.findAll();
Map map = new HashMap();
map.put("code", 0);
map.put("msg", "成功");
map.put("data", students);
return map;
}
//根据id查
@RequestMapping("/findById")
public Map findById(Integer studentId) {
Student student = studentService.findById(studentId);
Map map = new HashMap();
map.put("code", 0);
map.put("msg", "成功");
map.put("data", student);
return map;
}
@RequestMapping("/findByParam")
public Map findByParam(Student student) {
List<Student> students = studentService.findByParam(student);
Map map = new HashMap();
map.put("code", 0);
map.put("msg", "成功");
map.put("data", students);
return map;
}
// @RequestMapping("/findOther")
// public Map findOther() {
// List<Student> students = studentService.selectByExample(null);
// Map map = new HashMap();
// map.put("code", 0);
// map.put("msg", "失败");
// map.put("data", students);
// return map;
// }
@RequestMapping("/findOneById")
public Map findOneById(Integer studentId) {
Student student = studentService.selectByPrimaryKey(studentId);
Map map = new HashMap();
map.put("code", 0);
map.put("msg", "失败");
map.put("data", student);
return map;
}
//动态sql查询
@RequestMapping("/findByParamKuangJia")
public Map findByParamKuangJia(Student student){
StudentExample example = new StudentExample();
StudentExample.Criteria criteria = example.createCriteria();
if (null !=student.getClssIdf() && !"".equals(student.getClssIdf())) {
criteria.andClssIdfEqualTo(student.getClssIdf());
}
if (null !=student.getStudentAge() && !"".equals(student.getStudentAge())) {
criteria.andStudentAgeLessThan(student.getStudentAge());
}
if (null !=student.getStudentName() && !"".equals(student.getStudentName())) {
criteria.andStudentNameEqualTo(student.getStudentName());
}
if (null != student.getStudentPassword() && !"".equals(student.getStudentPassword())) {
criteria.andStudentPasswordEqualTo(student.getStudentPassword());
}
if (null != student.getStudentMoney() && !"".equals(student.getStudentMoney())) {
criteria.andStudentMoneyEqualTo(student.getStudentMoney());
}
List<Student> students = studentService.findByExample(example);
Map codeMap = new HashMap<>();
if (students.size()==0){
codeMap.put("code",400);
codeMap.put("msg","查询失败");
return codeMap;
}else {
codeMap.put("code",0);
codeMap.put("msg","查询成功");
codeMap.put("data",students);
return codeMap;
}
}
//分页查询 带参数,layui版
@RequestMapping("/selectAllPage")
public Map selectAllPage(Student student,Integer page,
@RequestParam(value = "limit",required = false,defaultValue = "5") Integer pageSize){
//pageHelper Mybatis 插件 做分页
String studentName = student.getStudentName();
String studentAge = student.getStudentAge();
StudentExample example = new StudentExample();
StudentExample.Criteria criteria = example.createCriteria();
if (null !=student.getStudentAge() && !"".equals(student.getStudentAge())) {
int i = Integer.parseInt(studentAge);
if (i==17){
criteria.andStudentAgeLessThanOrEqualTo(studentAge);
}else if (i==18){
criteria.andStudentAgeGreaterThan(studentAge);
}
}
if (null !=student.getStudentName() && !"".equals(student.getStudentName())) {
criteria.andStudentNameEqualTo(studentName);
}
if (null!=student.getStudentTime()&&!"".equals(student.getStudentTime())){
criteria.andStudentTimeEqualTo(student.getStudentTime());
}
PageHelper.startPage(page, pageSize);
List<Student> students = studentService.selectByExample(example);
PageInfo pageInfo = new PageInfo(students);
Map codemap = new HashMap();
codemap.put("code",0);
codemap.put("msg","请求成功");
//layui所用到的接口
codemap.put("data",pageInfo.getList());
codemap.put("count",pageInfo.getTotal());
return codemap;
}
//根据id删除
@RequestMapping("/deleteByPrimaryKey")
public Map deleteByPrimaryKey(Integer studentId){
int students = studentService.deleteByPrimaryKey(studentId);
Map map = new HashMap();
if (students>0){
map.put("msg", "删除成功");
}else {
map.put("msg", "删除失败");
}
map.put("code", 0);
return map;
}
//批量删除
@RequestMapping("/deleteAll")
public Map deleteAll(@RequestParam(value="ids[]") List<Integer> ids){
for (Integer id : ids) {
this.deleteByPrimaryKey(id);
}
Map map = new HashMap();
map.put("code", 0);
map.put("msg", "删除成功");
return map;
}
//增加学生
@RequestMapping("/insertStudent")
public Map insertSelective(Student student) {
int i = studentService.insertSelective(student);
System.out.println("1");
Map codeMap = new HashMap();
if (i == 1) {
codeMap.put("code",0);
codeMap.put("msg","新增成功");
return codeMap;
}else{
codeMap.put("code",400);
codeMap.put("msg","新增失败");
return codeMap;
}
}
//根据时间查
@RequestMapping("/selectTimeAll")
public Map selectTimeAll(Student student){
System.out.println(1);
StudentExample studentExample = new StudentExample();
StudentExample.Criteria criteria = studentExample.createCriteria();实现曾
if (null !=student.getClssIdf() && !"".equals(student.getClssIdf())) {
criteria.andClssIdfEqualTo(student.getClssIdf());
}
if (null !=student.getStudentAge() && !"".equals(student.getStudentAge())) {
criteria.andStudentAgeLessThan(student.getStudentAge());
}
System.out.println(2);
List<Student> students = studentService.findByExample(studentExample);
Map codeMap = new HashMap<>();
codeMap.put("code", 0);
codeMap.put("msg","请求成功");
codeMap.put("data", students);
System.out.println("codemap"+codeMap);
return codeMap;
}
//修改
@RequestMapping("/updateByPrimaryKeySelective")
public Map updateByPrimaryKeySelective(Student student){
System.out.println(1);
int i = studentService.updateByPrimaryKeySelective(student);
Map codemap = new HashMap();
System.out.println(2);
if (i==1){
codemap.put("code", 0);
codemap.put("msg","修改成功");
System.out.println(3);
return codemap;
}else {
codemap.put("code", 400);
codemap.put("msg","修改失败");
return codemap;
}
}
//批量修改 接受前端money参数 给未成年人 修改 零花钱数
@RequestMapping("/updateMoney")
public Map updateMoney(Double money){
//1.构建未成年的条件
StudentExample example = new StudentExample();
StudentExample.Criteria criteria = example.createCriteria();
criteria.andStudentAgeLessThan("18");
Student student = new Student();
student.setStudentMoney(money);
int i = studentService.updateByExampleSelective(student, example);
Map codemap = new HashMap();
codemap.put("code", 0);
codemap.put("msg","修改成功");
System.out.println(3);
return codemap;
}
@RequestMapping("/updateTime")
public Map updateTime() throws ParseException {
StudentExample example = new StudentExample();
StudentExample.Criteria criteria = example.createCriteria();
String time1 = "2020-01-02";
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date parse = sdf.parse(time1);
criteria.andStudentTimeLessThan(parse);
Student student = new Student();
student.setStudentTime(parse);
Map codemap = new HashMap();
int i = studentService.updateByExampleSelective(student, example);
if (i>0){
codemap.put("code", 0);
codemap.put("msg","修改成功");
return codemap;
}else {
codemap.put("code", 400);
codemap.put("msg", "修改失败");
return codemap;
}
}
}
实现层代码:
package com.xiexin.service;
import com.xiexin.bean.Student;
import com.xiexin.bean.StudentExample;
import com.xiexin.dao.StudentDAO;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service("studentService")
public class StudentServiceImpl implements StudentService {
@Autowired
public StudentDAO studentDAO;
//全查
@Override
public List<Student> findAll() {
List<Student> studnts = studentDAO.findAll();
return studnts;
}
//根据id查
@Override
public Student findById(Integer studentId) {
Student student = studentDAO.findById(studentId);
return student;
}
//这个是使用生成器来实现全查效果
@Override
public List<Student> findByParam(Student student) {
List<Student> students = studentDAO.findByParam(student);
return students;
}
//调用框架查询
@Override
public List<Student> selectByExample(StudentExample example) {
List<Student> students = studentDAO.selectByExample(example);
return students;
}
//根据id查询
@Override
public Student selectByPrimaryKey(Integer studentId) {
Student student = studentDAO.selectByPrimaryKey(studentId);
return student;
}
@Override
public List<Student> findByExample(StudentExample example) {
List<Student> students = studentDAO.selectByExample(example);
return students;
}
//根据id删除
@Override
public int deleteByPrimaryKey(Integer studentId) {
int students = studentDAO.deleteByPrimaryKey(studentId);
return students;
}
@Override
public int insertSelective(Student record) {
int i = studentDAO.insertSelective(record);
return i;
}
//修改
@Override
public int updateByPrimaryKeySelective(Student record) {
int i = studentDAO.updateByPrimaryKeySelective(record);
return i;
}
//动态sql实现修改
@Override
public int updateByExampleSelective(Student record, StudentExample example) {
return studentDAO.updateByExampleSelective(record,example);
}
}
实现层看不明白的可以去看service层
service接口:
package com.xiexin.service;
import com.xiexin.bean.Student;
import com.xiexin.bean.StudentExample;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface StudentService {
//全查
List<Student> findAll();
//根据id查
Student findById(Integer studentId);
//where后跟and
List<Student> findByParam(Student student);
//调用StudentExample中的封装类
//全查
List<Student>selectByExample(StudentExample example);
//根据id查
Student selectByPrimaryKey(Integer studentId);
// 带参查询调用框架 仅限
List<Student> findByExample(StudentExample example);
//根据id删除
int deleteByPrimaryKey(Integer studentId);
//添加
int insertSelective(Student record);
//修改
int updateByPrimaryKeySelective(Student record);
//批量修改
int updateByExampleSelective(@Param("record") Student record, @Param("example") StudentExample example);//批量修改
}
dao层:
package com.xiexin.dao;
import com.xiexin.bean.Student;
import com.xiexin.bean.StudentExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
public interface StudentDAO {
long countByExample(StudentExample example);
int deleteByExample(StudentExample example);
int deleteByPrimaryKey(Integer studentId);
int insert(Student record);
int insertSelective(Student record);
//List<Student>selectByExample(StudentExample example);
//List<Student> findByExample(StudentExample example);
List<Student> selectByExample(StudentExample example);
Student selectByPrimaryKey(Integer studentId);
int updateByExampleSelective(@Param("record") Student record, @Param("example") StudentExample example);//批量修改
int updateByExample(@Param("record") Student record, @Param("example") StudentExample example);
int updateByPrimaryKeySelective(Student record);
int updateByPrimaryKey(Student record);
//以下自己写的
//全查
List<Student> findAll();
//根据id查
Student findById(Integer studentId);
// where 后的and查询
List<Student> findByParam(Student student);
}
dao.xml:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.xxx.dao.StudentDAO">
<resultMap id="BaseResultMap" type="com.xxx.bean.Student">
<id column="student_id" jdbcType="INTEGER" property="studentId"/>
<result column="student_name" jdbcType="VARCHAR" property="studentName"/>
<result column="student_age" jdbcType="VARCHAR" property="studentAge"/>
<result column="student_time" jdbcType="TIMESTAMP" property="studentTime"/>
<result column="student_account" jdbcType="VARCHAR" property="studentAccount"/>
<result column="student_password" jdbcType="VARCHAR" property="studentPassword"/>
<result column="student_money" jdbcType="DOUBLE" property="studentMoney"/>
<result column="clss_idf" jdbcType="INTEGER" property="clssIdf"/>
</resultMap>
<sql id="Example_Where_Clause">
<where>
<foreach collection="oredCriteria" item="criteria" separator="or">
<if test="criteria.valid">
<trim prefix="(" prefixOverrides="and" suffix=")">
<foreach collection="criteria.criteria" item="criterion">
<choose>
<when test="criterion.noValue">
and ${criterion.condition}
</when>
<when test="criterion.singleValue">
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue">
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue">
and ${criterion.condition}
<foreach close=")" collection="criterion.value" item="listItem" open="("
separator=",">
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Update_By_Example_Where_Clause">
<where>
<foreach collection="example.oredCriteria" item="criteria" separator="or">
<if test="criteria.valid">
<trim prefix="(" prefixOverrides="and" suffix=")">
<foreach collection="criteria.criteria" item="criterion">
<choose>
<when test="criterion.noValue">
and ${criterion.condition}
</when>
<when test="criterion.singleValue">
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue">
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue">
and ${criterion.condition}
<foreach close=")" collection="criterion.value" item="listItem" open="("
separator=",">
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Base_Column_List">
student_id, student_name, student_age, student_time, student_account, student_password,
student_money, clss_idf
</sql>
<select id="selectByExample" parameterType="com.xxx.bean.StudentExample" resultMap="BaseResultMap">
select
<if test="distinct">
distinct
</if>
<include refid="Base_Column_List"/>
from student
<if test="_parameter != null">
<include refid="Example_Where_Clause"/>
</if>
<if test="orderByClause != null">
order by ${orderByClause}
</if>
<if test="limit != null">
<if test="offset != null">
limit ${offset}, ${limit}
</if>
<if test="offset == null">
limit ${limit}
</if>
</if>
</select>
<select id="selectByPrimaryKey" parameterType="java.lang.Integer" resultMap="BaseResultMap">
select
<include refid="Base_Column_List"/>
from student
where student_id = #{studentId,jdbcType=INTEGER}
</select>
<delete id="deleteByPrimaryKey" parameterType="java.lang.Integer">
delete from student
where student_id = #{studentId,jdbcType=INTEGER}
</delete>
<delete id="deleteByExample" parameterType="com.xxx.bean.StudentExample">
delete from student
<if test="_parameter != null">
<include refid="Example_Where_Clause"/>
</if>
</delete>
<insert id="insert" parameterType="com.xxx.bean.Student">
insert into student (student_id, student_name, student_age,
student_time, student_account, student_password,
student_money, clss_idf)
values (#{studentId,jdbcType=INTEGER}, #{studentName,jdbcType=VARCHAR}, #{studentAge,jdbcType=VARCHAR},
#{studentTime,jdbcType=TIMESTAMP}, #{studentAccount,jdbcType=VARCHAR}, #{studentPassword,jdbcType=VARCHAR},
#{studentMoney,jdbcType=DOUBLE}, #{clssIdf,jdbcType=INTEGER})
</insert>
<insert id="insertSelective" parameterType="com.xxx.bean.Student">
insert into student
<trim prefix="(" suffix=")" suffixOverrides=",">
<if test="studentId != null">
student_id,
</if>
<if test="studentName != null">
student_name,
</if>
<if test="studentAge != null">
student_age,
</if>
<if test="studentTime != null">
student_time,
</if>
<if test="studentAccount != null">
student_account,
</if>
<if test="studentPassword != null">
student_password,
</if>
<if test="studentMoney != null">
student_money,
</if>
<if test="clssIdf != null">
clss_idf,
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides=",">
<if test="studentId != null">
#{studentId,jdbcType=INTEGER},
</if>
<if test="studentName != null">
#{studentName,jdbcType=VARCHAR},
</if>
<if test="studentAge != null">
#{studentAge,jdbcType=VARCHAR},
</if>
<if test="studentTime != null">
#{studentTime,jdbcType=TIMESTAMP},
</if>
<if test="studentAccount != null">
#{studentAccount,jdbcType=VARCHAR},
</if>
<if test="studentPassword != null">
#{studentPassword,jdbcType=VARCHAR},
</if>
<if test="studentMoney != null">
#{studentMoney,jdbcType=DOUBLE},
</if>
<if test="clssIdf != null">
#{clssIdf,jdbcType=INTEGER},
</if>
</trim>
</insert>
<select id="countByExample" parameterType="com.xxx.bean.StudentExample" resultType="java.lang.Long">
select count(*) from student
<if test="_parameter != null">
<include refid="Example_Where_Clause"/>
</if>
</select>
<update id="updateByExampleSelective" parameterType="map">
update student
<set>
<if test="record.studentId != null">
student_id = #{record.studentId,jdbcType=INTEGER},
</if>
<if test="record.studentName != null">
student_name = #{record.studentName,jdbcType=VARCHAR},
</if>
<if test="record.studentAge != null">
student_age = #{record.studentAge,jdbcType=VARCHAR},
</if>
<if test="record.studentTime != null">
student_time = #{record.studentTime,jdbcType=TIMESTAMP},
</if>
<if test="record.studentAccount != null">
student_account = #{record.studentAccount,jdbcType=VARCHAR},
</if>
<if test="record.studentPassword != null">
student_password = #{record.studentPassword,jdbcType=VARCHAR},
</if>
<if test="record.studentMoney != null">
student_money = #{record.studentMoney,jdbcType=DOUBLE},
</if>
<if test="record.clssIdf != null">
clss_idf = #{record.clssIdf,jdbcType=INTEGER},
</if>
</set>
<if test="_parameter != null">
<include refid="Update_By_Example_Where_Clause"/>
</if>
</update>
<update id="updateByExample" parameterType="map">
update student
set student_id = #{record.studentId,jdbcType=INTEGER},
student_name = #{record.studentName,jdbcType=VARCHAR},
student_age = #{record.studentAge,jdbcType=VARCHAR},
student_time = #{record.studentTime,jdbcType=TIMESTAMP},
student_account = #{record.studentAccount,jdbcType=VARCHAR},
student_password = #{record.studentPassword,jdbcType=VARCHAR},
student_money = #{record.studentMoney,jdbcType=DOUBLE},
clss_idf = #{record.clssIdf,jdbcType=INTEGER}
<if test="_parameter != null">
<include refid="Update_By_Example_Where_Clause"/>
</if>
</update>
<update id="updateByPrimaryKeySelective" parameterType="com.xxx.bean.Student">
update student
<set>
<if test="studentName != null">
student_name = #{studentName,jdbcType=VARCHAR},
</if>
<if test="studentAge != null">
student_age = #{studentAge,jdbcType=VARCHAR},
</if>
<if test="studentTime != null">
student_time = #{studentTime,jdbcType=TIMESTAMP},
</if>
<if test="studentAccount != null">
student_account = #{studentAccount,jdbcType=VARCHAR},
</if>
<if test="studentPassword != null">
student_password = #{studentPassword,jdbcType=VARCHAR},
</if>
<if test="studentMoney != null">
student_money = #{studentMoney,jdbcType=DOUBLE},
</if>
<if test="clssIdf != null">
clss_idf = #{clssIdf,jdbcType=INTEGER},
</if>
</set>
where student_id = #{studentId,jdbcType=INTEGER}
</update>
<update id="updateByPrimaryKey" parameterType="com.xxx.bean.Student">
update student
set student_name = #{studentName,jdbcType=VARCHAR},
student_age = #{studentAge,jdbcType=VARCHAR},
student_time = #{studentTime,jdbcType=TIMESTAMP},
student_account = #{studentAccount,jdbcType=VARCHAR},
student_password = #{studentPassword,jdbcType=VARCHAR},
student_money = #{studentMoney,jdbcType=DOUBLE},
clss_idf = #{clssIdf,jdbcType=INTEGER}
where student_id = #{studentId,jdbcType=INTEGER}
</update>
<!--全查-->
<select id="findAll" resultMap="BaseResultMap">
select * from student
</select>
<!--根据id查询-->
<select id="findOne" parameterType="int" resultMap="BaseResultMap">
select * from student where student_id = #{studentId}
</select>
<!--多参查询 where 后的and查询-->
<select id="findByParam" parameterType="com.xxx.bean.Student" resultMap="BaseResultMap">
<![CDATA[
select * from student where clss_idf = #{clssIdf} and student_age < #{studentAge}
]]></select>
</mapper>
dao层和dao.xml是由代码生成器生成,看不懂也没问题,直接添加或者调用就行
后台到前台的时间获取可以使用
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
这两个注解,具体详情以及使用方法自行百度
前台html代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="/res/layui-v2.5.6/layui/css/layui.css">
<script src="/res/layui-v2.5.6/layui/layui.js"></script>
</head>
<body>
<div>
<script type="text/html" id="insertOneHtml"> <!--οnsubmit="return false" 防止跳转-->
<form class="layui-form layui-form-pane" action="" lay-filter="insertForm" οnsubmit="return false" >
<div class="layui-form-item">
<label class="layui-form-label">学生账号</label>
<div class="layui-input-inline">
<input type="text" name="studentAccount" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生密码</label>
<div class="layui-input-inline">
<input type="text" name="studentPassword" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">真实姓名</label>
<div class="layui-input-inline">
<input type="text" name="studentName" placeholder="请输入" class="layui-input">
</div>
</div>
<!--// 明天作业:添加 学生得身份证号。 后台读取身份正好, 自动算出年龄 和 性别 , 以及学生得生日, 星座-->
<div class="layui-form-item">
<label class="layui-form-label">学生年龄</label>
<div class="layui-input-inline">
<input type="text" name="studentAge" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生资金</label>
<div class="layui-input-inline">
<input type="text" name="studentMoney" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生入学时间</label>
<div class="layui-input-inline">
<input name="studentTime" type="text" class="layui-input" id="test5" placeholder="yyyy-MM-dd HH:mm:ss">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生积分</label>
<div class="layui-input-inline">
<input type="text" name="studentMoney" placeholder="请输入" class="layui-input">
</div>
</div>
<button type="submit" class="layui-btn" lay-submit="" lay-filter="insertSubmit" id="insertSubmit" style="display: none">立即提交</button>
</form>
</script>
<!-- 修改的 弹窗html-->
<script type="text/html" id="updateUserHtml">
<form class="layui-form layui-form-pane" action="" lay-filter="updateForm" οnsubmit="return false" >
<div class="layui-form-item">
<label class="layui-form-label">学生id</label>
<div class="layui-input-inline">
<input type="text" name="studentId" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生账号</label>
<div class="layui-input-inline">
<input type="text" name="studentAccount" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生密码</label>
<div class="layui-input-inline">
<input type="text" name="studentPassword" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">真实姓名</label>
<div class="layui-input-inline">
<input type="text" name="studentName" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生年龄</label>
<div class="layui-input-inline">
<input type="text" name="studentAge" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生资金</label>
<div class="layui-input-inline">
<input type="text" name="studentMoney" placeholder="请输入" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生入学时间</label>
<div class="layui-input-inline">
<input name="studentTime" type="text" class="layui-input" id="test6" placeholder="yyyy-MM-dd HH:mm:ss">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">学生积分</label>
<div class="layui-input-inline">
<input type="text" name="studentMoney" placeholder="请输入" class="layui-input">
</div>
</div>
<!-- <button type="submit" class="layui-btn" lay-submit="" lay-filter="insertSubmit" id="updateSubmit" style="display: none">立即提交</button>-->
</form>
</script>
<form class="layui-form" action="" οnsubmit="return false">
<div class="layui-inline">
<label class="layui-form-label">名字</label>
<div class="layui-input-inline">
<input type="text" name="studentName" class="layui-input">
</div>
</div>
<div class="layui-inline">
<label class="layui-form-label">是否成年</label>
<div class="layui-input-inline">
<select name="studentAge">
<option value="">请选择选项</option>
<option value="18">成年</option>
<option value="17">未成年</option>
</select>
</div>
</div>
<div class="layui-inline">
<label class="layui-form-label">时间</label>
<div class="layui-input-inline">
<input type="text" name="studentTime" class="layui-input">
</div>
</div>
<div class="layui-inline">
<div class="layui-input-inline">
<button class="layui-btn" lay-submit lay-filter="formSearch">搜索</button>
<button type="reset" class="layui-btn layui-btn-primary">重置</button>
</div>
</div>
</form>
</div>
<table class="layui-hide" id="test" lay-filter="test"></table>
<script type="text/html" id="toolbarDemo">
<div class="layui-btn-container">
<button class="layui-btn layui-btn-sm" lay-event="getCheckData">获取选中行数据</button>
<button class="layui-btn layui-btn-sm" lay-event="getCheckLength">获取选中数目</button>
<button class="layui-btn layui-btn-sm" lay-event="isAll">验证是否全选</button>
<button class="layui-btn layui-btn-sm layui-btn-danger" lay-event="moreDel">批量删除</button>
<<button class="layui-btn layui-btn-sm layui-btn-warm" lay-event="insertOne">新增一个同学</button>
<button class="layui-btn layui-btn-sm layui-btn-warm" lay-event="moreUpdate">批量修改</button>
</div>
</script>
<script type="text/html" id="barDemo">
<a class="layui-btn layui-btn-xs" lay-event="edit">编辑</a>
<a class="layui-btn layui-btn-danger layui-btn-xs" lay-event="del">删除</a>
</script>
<script>
layui.use(['table', 'jquery', 'layer', 'form', 'layedit', 'laydate'], function () {
var table = layui.table;
var layer = layui.layer;
var $ = layui.jquery; // $
var form = layui.form;
var layedit = layui.layedit;
var laydate = layui.laydate;
form.on('submit(insertSubmit)' , function (data) {
console.log(data)
$.ajax({
url:"/student/insertStudent",
type:'POST',
data:data.field,
success:function (res) {
console.log(res)
},
dataType:'json'
});
});
form.on('submit(formSearch)', function(data){
console.log(data.field)
table.reload('test', {
where: { //设定异步数据接口的额外参数,任意设
studentName: data.field.studentName
,studentAge: data.field.studentAge
,studentTime: data.field.studentTime
}
,page: {
curr: 1 //重新从第 1 页开始
}
}); //只重载数据
return false;
});
table.render({
elem: '#test'
, url: '/student/selectAllPage'
, toolbar: '#toolbarDemo' //开启头部工具栏,并为其绑定左侧模板
, defaultToolbar: ['filter', 'exports', 'print', { //自定义头部工具栏右侧图标。如无需自定义,去除该参数即可
title: '提示'
, layEvent: 'LAYTABLE_TIPS'
, icon: 'layui-icon-tips'
}]
, title: '用户数据表'
, cols: [[
{type: 'checkbox', fixed: 'left'}
, {field: 'studentId', title: 'ID', width: 80, fixed: 'left', unresize: true, sort: true}
, {field: 'studentAccount', title: '用户名', width: 120, edit: 'text'}
, {field: 'studentPassword', title: '密码', width: 150, edit: 'text', sort: true}
, {field: 'studentName', title: '真实姓名', width: 100}
, {field: ' studentAge;\n', title: '年龄', width: 120, templet: function (res) {
if (res.studentAge >= 18) {
return " <span style='color: red'> 已成年 </span> "
} else if (res.studentAge <= 18) {
return " <span style='color: green'> 未成年 </span> "
}
}
}
, {field: 'studentTime', title: '创建时间', width: 180, sort: true}
, {field: 'studentMoney', title: '零花钱', width: 180, sort: true}
, {fixed: 'right', title: 'right', toolbar: '#barDemo', width: 150}
]]
, page: true
// , parseData: function (res) { //res 即为原始返回的数据
// var code = 0111;
// if (res.resultCode == 20000) {
// code = 0;
// } else {
// code = res.resultCode;
// }
// return {
// "code": code, //解析接口状态
// "msg": res.resultMsg, //解析提示文本
// "count": res.totalCount, //解析数据长度
// "data": res.resultData //解析数据列表
// };
// }
});
//头工具栏事件
table.on('toolbar(test)', function (obj) {
var checkStatus = table.checkStatus(obj.config.id);
switch (obj.event) {
case 'getCheckData':
var data = checkStatus.data;
layer.alert(JSON.stringify(data));
break;
case 'getCheckLength':
var data = checkStatus.data;
layer.msg('选中了:' + data.length + ' 个');
break;
case 'isAll':
layer.msg(checkStatus.isAll ? '全选' : '未全选');
break;
//自定义头工具栏右侧图标 - 提示
case 'LAYTABLE_TIPS':
layer.alert('这是工具栏右侧自定义的一个图标按钮');
break
//批量删除
case 'moreDel':
var data = checkStatus.data
// console.log(data)
var ids = new Array();
$.each(data,function (index,item) {
// console.log(item.studentId);
ids.push(item.studentId)
})
console.log(ids)
$.ajax({
url:"/student/deleteAll",
type:"post",
data:{"ids":ids},
success:function (res) {
console.log(res)
},
dataType:'json'
});
break;
//批量修改
case "moreUpdate":
var data = checkStatus.data
var list = new Array();
$.each(data,function (index,item) {
list.push(item.studentId);
})
console.log("list="+list)
layer.confirm("是否全部修改",function (index) {
$.ajax({
url:"/后台路径",
type:"post",
data:{"list":list},
success:function (res) {
console.log(res)
table.reload('test');
},
dataType:'json'
});
});
break;
case 'insertOne':
//弹窗!!
layer.open({
type: 1,
content: $("#insertOneHtml").html(),
title: "新增一个学员",
area: ['700px', '700px'],
success: function (layero, index) {
//日期时间选择器
laydate.render({
elem: '#test5'
, type: 'datetime'
});
},
btn: ['确认修改', '取消修改', '按钮三'],
yes: function (index, layero) {
$("#insertSubmit").click()
},
btn2: function (index, layero) {
//return false 开启该代码可禁止点击该按钮关闭
},
cancel: function () {
//右上角关闭回调
//return false 开启该代码可禁止点击该按钮关闭
}
});
break;
case "sousuo":
}
});
//监听行工具事件
table.on('tool(test)', function (obj) {
var data = obj.data;
console.log(data)
//console.log(obj)
if (obj.event === 'del') {
layer.confirm('真的删除行么', function (index) {
// obj.del();
// 发送 ajax 到 后台 进行 删除!!
$.ajax({
url: '/student/deleteByPrimaryKey',
type: 'post',
data: {'id': data.studentId},
success: function (res) {
console.log(res);
// res.code == 400 no ==0 ok
if (res.code == 0) {
layer.msg("删除成功")
obj.del();
} else {
layer.msg("删除成功")
}
},
dataType: 'json'
});
layer.close(index);
});
} else if (obj.event === 'edit') {
layer.open({
type: 1,
content: $("#updateUserHtml").html(),
title: "用户的修改",
area: ['700px', '700px'],
success: function (layero, index) {
console.log(layero, index);
form.val("updateForm",{
"studentId":data.studentId,
"studentName":data.studentName,
"studentPassword":data.studentPassword,
"studentAge":data.studentAge,
"studentAccount":data.studentAccount,
"studentMoney":data.studentMoney,
"studentTime":data.studentTime
});
form.render() // 当页面重新 更新的后, 一定记得重新渲染一下 layui, 最好 后台/前台清空缓存
//日期时间选择器
laydate.render({
elem: '#test6'
, type: 'datetime'
});
},
btn: ['确认修改', '取消修改', '按钮三'],
yes: function (index, layero) {
var data = form.val("updateForm");
console.log(data)
$.ajax({
url: '/student/updateByPrimaryKeySelective',
type: 'post',
data: data,
success: function (res) {
console.log(res)
if (res.code == 0) {
layer.msg("修改成功")
layer.close(index);
table.reload('test');
// window.location.href="/res/stude.html";
} else {
layer.msg("修改失败")
}
},
dataType: 'json'
});
},
btn2: function (index, layero) {
//按钮【按钮二】的回调
//return false 开启该代码可禁止点击该按钮关闭
},
cancel: function () {
//右上角关闭回调
alert("你点击了 右上角的关闭")
//return false 开启该代码可禁止点击该按钮关闭
}
});
}
})
});
</script>
</body>
</html>
本人属于前端页面废物一个,请大家谅解
批量修改:
这个是没太了解过,只能让大家看看,以后会不定时的更新(看我自学能力来说)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>使用工具批量的修改</title>
<script src="http://libs.baidu.com/jquery/2.0.0/jquery.min.js"></script>
</head>
<body>
<div>
<span>给未成年学生增加:<input type="text" id="money"><span>元</span>,</span><input type="button" value="增加" id="btnUpdate"></span>
</div>
<div>
<span>更改时间:<input type="text" id="update"></span><input type="button" value="修改" id="btnUpdateTime"></span>
</div>
<script>
$(function () {
$("#btnUpdate").click(function () {
$.ajax({
url:"/student/updateMoney",
type:"post",
data:{
"money":$("#money").val()
},
success:function (res) {
console.log(res)
},
dataType:"json"
});
});
$("#btnUpdateTime").click(function () {
var up= $("#update").val();
console.log(up)
$.ajax({
url:"/student/updateTime",
type:"post",
data:{
"update":$("#update").val()
},
success:function (res) {
console.log(res)
},
dataType:"json"
});
});
});
</script>
</body>
</html>
功能实现图:
全查:
搜索:
根据姓名搜索:
根据时间搜索:
根据是否成年搜索:
新增:
编辑(修改):
删除:
map传值的时候msg写错了,但是删除功能可以实现
批量删除:
没有回显,所有不直接刷新
新增: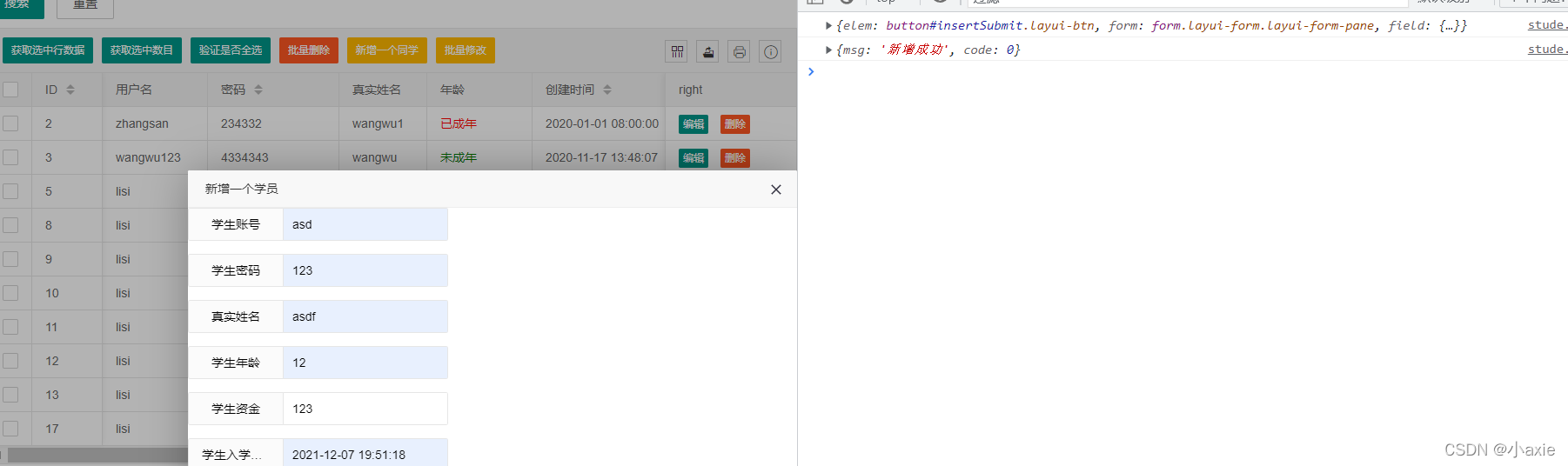