栈的容器实现
代码展示
#include <iostream>
using namespace std;
template<typename T>
class Stack
{
private:
int top;
T*bottom;
T*end;
public:
Stack(int size = 2) {
bottom = new T[size];
top = -1;
end = bottom+size;
}
~Stack()
{
delete[]bottom;
end=bottom= NULL;
}
Stack(const Stack &other)
{
int lenth =top;
int size = other.end - other.bottom;
this->bottom = new T[size];
memcpy(this->bottom,other.bottom,lenth*sizeof(T));
this->end = this->bottom+size;
this->top = this->top+size;
}
bool empty()
{
return top == -1;
}
bool full()
{
return this->top == this->size()-1;
}
void greater()
{
int size = this->end-this->bottom;
T*temp = new T[2*size];
memcpy(temp,this->bottom,size*sizeof(T));
delete []bottom;
bottom = temp;
end = bottom + 2*size;
}
void push_back(const T val)
{
if(this->full())
{
this->greater();
}
top++;
bottom[top] = val;
}
void pop_back()
{
if(this->empty())
{
return;
}
--top;
}
T fornt()const
{
return *bottom;
}
int size()
{
return end-bottom;
}
int lenth()
{
return top;
}
T &at(int index)
{
if((index<0) || index > this->lenth())
{
cout<<"访问越界"<<endl;
}
return bottom[index];
}
};
int main()
{
Stack<int>v;
for(int i=1; i<=20; i++)
{
v.push_back(i);
cout<<v.size()<<endl;
}
for(int i=0; i<20; i++)
{
cout<<v.at(i)<<" ";
}
cout<<endl;
cout << "Hello World!" << endl;
return 0;
}
运行结果
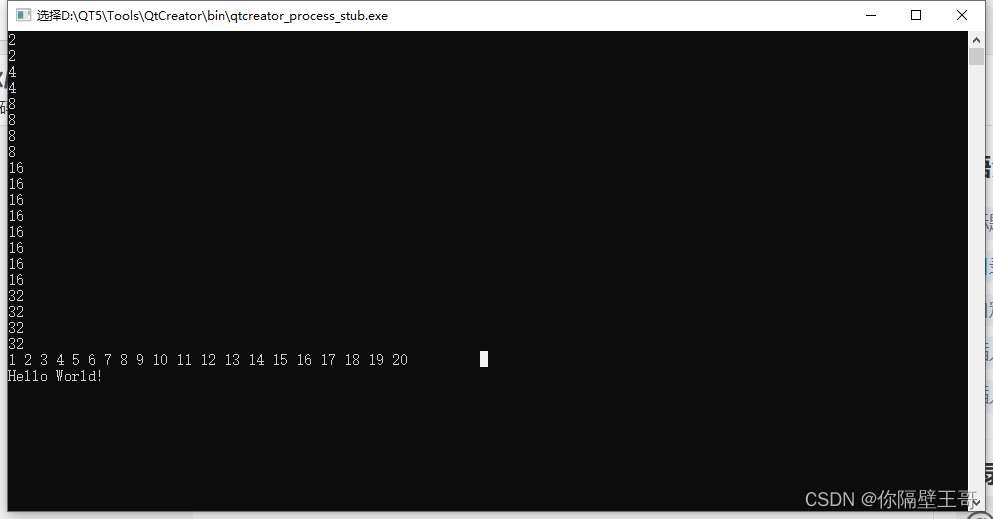
队列的容器实现
代码展示
#include <iostream>
#include <cstring>
using namespace std;
template<class T>
class myqueue {
private:
T*first;
int front;
int tail;
int size;
public:
myqueue()
{
size=20;
front=tail=0;
first = new T[size];
}
~myqueue()
{
delete[]first;
first=NULL;
}
myqueue(const myqueue &other)
{
int len = (tail+size-front)%size;
this->first = new T[size];
memcpy(this->first, other.first, len*sizeof(int));
}
bool empty()
{
return this->tail == this->front;
}
bool full()
{
return (this->tail+1)%size == this->front;
}
void greater()
{
T *temp = new T[2*size];
size=size*2;
memcpy(temp, this->first, size*sizeof (int));
delete []first;
first = temp;
}
void push_back( const T val)
{
if(this->full())
{
this->greater();
}
else
{
tail=(tail+1)%size;
first[tail]=val;
}
}
void pop_back()
{
if(this->empty())
{
return;
}
front=(front+1)%size;
}
T mfront() const
{
return first[front];
}
int msize()
{
return (tail+size-front)%size;
}
int len()
{
return size;
}
T &at(int index)
{
if(index<0 || index>(index+1)%size)
{
cout<<"访问越界"<<endl;
}
return first[index];
}
};
int main()
{
myqueue<int> v;
for(int i=1; i<=20; i++)
{
v.push_back(i);
cout<<v.msize()<<endl;
}
for(int i=1; i<20; i++)
{
cout<<v.at(i)<<" ";
}
cout<<endl;
return 0;
}
运行结果
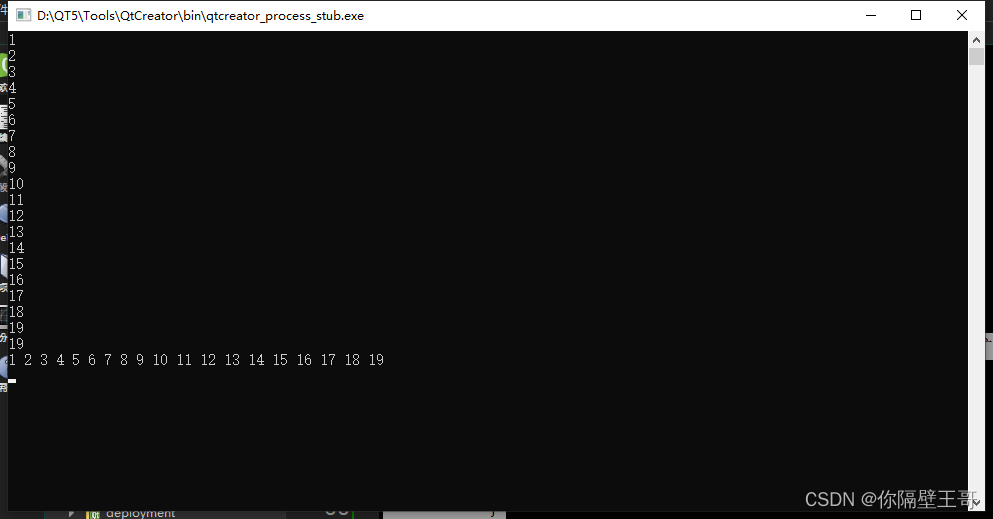